文本框控件
An auto-suggestion control saves your users some typing. It's a sort of combo-box that keys on the user input to automatically present a list of matching options. You've seen the functionaity on the Google main page: As you type, it displays a drop-down box that lists a set of suggestions. You can then click an item to have it auto-complete the input.
自动建议控件可以为您的用户节省一些输入时间。 这是一种组合框,可在用户输入上键入以自动显示匹配选项的列表。 您已经在Google主页上看到了功能:键入时,它会显示一个下拉框,其中列出了一组建议。 然后,您可以单击一个项目以使其自动完成输入。
Google's auto-suggestion box is very sophisticated, and I mention it only to give you the general idea. Today's simple example is much less ambitious. We'll discuss how to set up a text input box that is paired with a hidden listbox that's pre-filled with "suggestions" that have been read from from a server-side database. Then we'll look at some code to handle the U/I events --- and save your users some effort.
Google的自动提示框非常复杂,我提到它只是为了让您大致了解。 今天的简单例子远没有那么雄心勃勃。 我们将讨论如何设置一个文本输入框,该文本输入框与一个隐藏的列表框配对,该列表框预先填充了从服务器端数据库读取的“建议”。 然后,我们看一些代码来处理U / I事件---并节省用户的精力。
To follow along you'll need a PHP web-page and a database such as MySQL to obtain the list of values.
要继续学习,您将需要一个PHP网页和一个数据库(例如MySQL)来获取值列表。
2. Create a div with a width of 25% and set the visibility attribute to 'hidden' and a relatively short
2.创建一个宽度为25%的div ,并将可见性属性设置为“隐藏”且相对较短
height like around 100-150px and set the id attribute to something relevant like 'divSuggestions'
高度,例如100-150px左右,并将id属性设置为相关的内容,例如“ divSuggestions”
because we'll be referring to this div quite often via JavaScript's getElementById() function.
因为我们将经常通过JavaScript的getElementById()函数来引用该div。
Somewhere in the header section we need to create a JavaScript array variable that we'll use a little later. I'm calling this variable 'nameArray' because I'm looking up first and last names for my list of values.
在标头部分的某个位置,我们需要创建一个JavaScript数组变量,稍后我们将使用它。 我将这个变量称为“ nameArray”,因为我正在查找值列表的名字和姓氏。
<script type="text/javascript">
var nameArray = null;
</script>
s', we want the suggestions box to show all values from our table that begin with the letter 's'.
s ',我们希望“建议”框显示表中所有以字母“ s”开头的值。
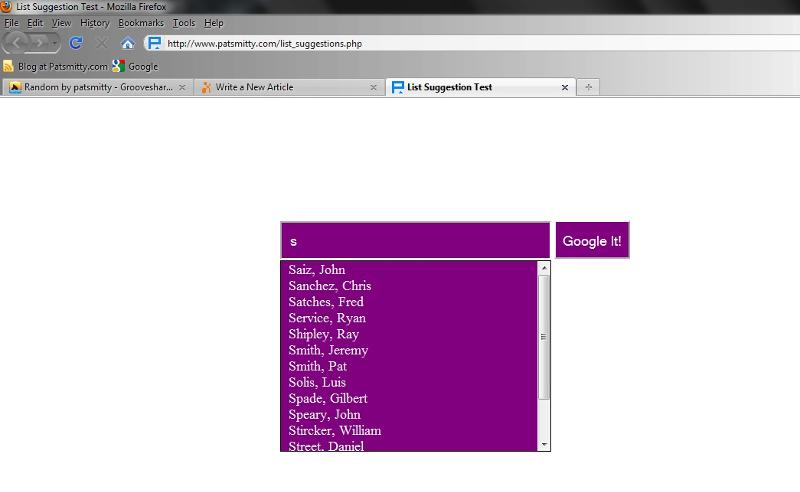
There are a lot of little things that we need to take into consideration when it comes to functionality. For this super-simple example we're going to display the box whenever a user begins to type. If the user types input to the point where there are not any matching values, the box disappears. The box will also disappear when the user erases all the text in the box (i.e. the text-box is empty).
在功能方面,我们需要考虑很多小事情。 对于这个超级简单的示例,我们将在用户开始输入内容时显示该框。 如果用户将输入键入到没有匹配值的点,则该框消失。 当用户擦除框中的所有文本时(即文本框为空),该框也将消失。
We will want to 'highlight' the suggestions when the user moves the mouse over them and add an event when the user clicks on a suggestion, the suggestions box disappears and the textbox is filled with the selected suggestion.
当用户将鼠标移到建议上方时,我们将要“突出显示”建议,并在用户单击建议时添加一个事件,建议框消失,并且文本框填充有所选建议。
Finally we will make the suggestions box disappear when the user clicks anywhere in the page outside of the suggestions box. All these little features makes for a nice, simple suggestions-box familiar to anyone wh's used the Google search box.
最后,当用户单击建议框之外的页面中的任意位置时,我们将使建议框消失。 所有这些小功能使使用Google搜索框的任何人都可以熟悉一个很好的简单建议框。
Let's get to JavaScript-ing and PHP-ing! Down at the bottom of the page just inside the </body> tag we'll create our javascript section. Inside the javascript tags we'll need to create 4 functions.
让我们开始JavaScript-ing和PHP-ing! 在页面底部</ body>标记内,我们将创建javascript部分。 在javascript标记内,我们需要创建4个函数。
We need to create a function that grabs the input text as the user types and determine if the suggestions box should show or not. Remember: 'divSuggestions' is the id of my textbox.
我们需要创建一个函数,该函数可以在用户键入时捕获输入文本,并确定建议框是否应显示。 记住:“ divSuggestions”是我的文本框的ID。
function doSuggestionBox(text) { // function that takes the text box's inputted text as an argument
var input = text; // store inputed text as a variable for later manipulation
// determine whether to display suggestion box or not
if (input == "") { // hide the suggestion box
document.getElementById('divSuggestions').style.visibility = 'hidden';
} else { // show the suggestion box
document.getElementById('divSuggestions').style.visibility = 'visible';
doSuggestions(text);
}
}
When a user clicks on a suggestion, we want to hide the suggestion box and display the selected suggestion in the text box (the ID of my text box is 'txtSearch').
当用户单击建议时,我们要隐藏建议框并在文本框中显示选定的建议(我的文本框的ID为“ txtSearch”)。
function doSelection(text) { // function that grabs the selected suggestion and hides the suggestions box and places the selected suggestion in the text box
var selection = text;
document.getElementById('divSuggestions').style.visibility = 'hidden'; // hides the suggestion box
document.getElementById("txtSearch").value = selection; // sets the text in the textbox to the selected suggestion
}
When a user moves the mouse over a suggestion, we want to highlight it. You can use whatever colors you want - I'm using (background/foreground) purple/white and white/black.
当用户将鼠标移到建议上方时,我们要突出显示该建议。 您可以使用任何想要的颜色-我使用的是(背景/前景)紫色/白色和白色/黑色。
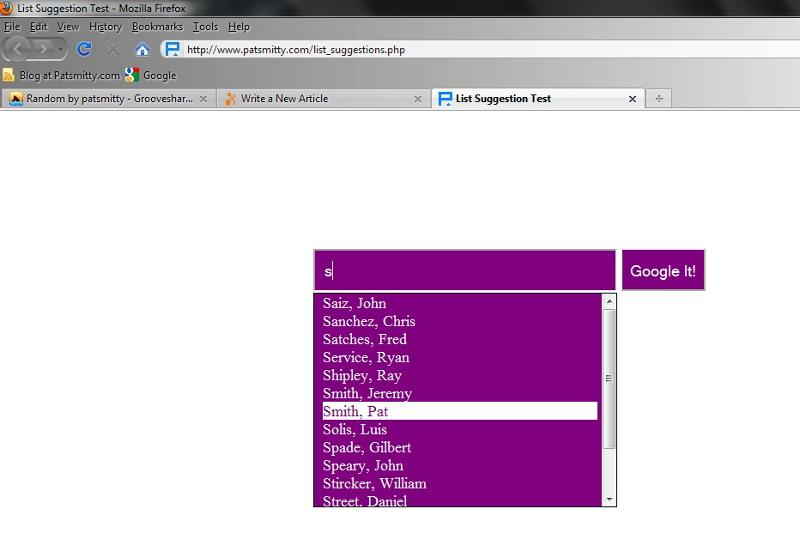
function changeBG(obj) { // when the mouse hovers over a suggestion, the suggestion is highlighted
element = document.getElementById(obj);
oldColor = element.style.backgroundColor;
if (oldColor == "purple" || oldColor == "") {
element.style.background = "white";
element.style.color = "purple";
element.style.cursor = "pointer";
} else {
element.style.background = "purple";
element.style.color = "white";
element.style.cursor = "default";
}
}
The following function actually creates the suggestions as individual divs to place into the suggestions box.
以下函数实际上将建议创建为单独的div,以放入建议框中。
function doSuggestions(text) { // actually displays the relevant suggestions in the suggestions box
var input = text;
var inputLength = input.toString().length;
var code = "";
var counter = 0;
// loop through our array of values to determine which ones are matching suggestions
while( counter < this.nameArray.length) {
var x = this.nameArray[counter]; // avoids retyping this code a bunch of times
// if statement that determines if the inputed text matches the value from our list of
// values - if true, adds the suggestion to the list
if(x.substr(0, inputLength).toLowerCase() == input.toLowerCase()) {
// The relative suggestion is a div that we'll place into the suggestions box. You can see
// how we're implementing a couple of the previous functions we created previously.
code += "<div id='" + x + "'onmouseover='changeBG(this.id);' onMouseOut='changeBG(this.id);' onclick='doSelection(this.innerHTML)'>" + x + "</div>";
}
counter += 1;
}
if (code == "") {
document.getElementById('divSuggestions').style.visibility='hidden';
}
document.getElementById('divSuggestions').innerHTML = code; // adds the suggestions to the suggestions box
document.getElementById('divSuggestions').style.overflow='auto'; // shows a vertical scroll bar if needed
}
Okay, we're almost done. All we need to do now is grab the list of values from our database. So at the top of our page just inside the <body> tag we'll add our <?php ?> section. Inside our php section we need to connect to the database and store the list of values in the JavaScript array that we created at the beginning. I called mine 'nameArray' because I'll be getting first and last names from my database.
好的,我们快完成了。 现在我们需要做的就是从数据库中获取值列表。 因此,在页面顶部<body>标记内,我们将添加<?php?>部分。 在我们的php部分中,我们需要连接到数据库并将值列表存储在我们最初创建JavaScript数组中。 我称自己的名字为“ nameArray”,因为我将从数据库中获取名字和姓氏。
mysql_connect("your database server", "username", "password") OR DIE ('Unable to connect to database! Please try again later.');
mysql_select_db('your database name');
$query = 'SELECT user_lname, user_fname FROM user ORDER BY user_lname ASC';
$result = mysql_query($query);
$counter = 0;
// write the values from the database into the javascript array
echo("<script type='text/javascript'>");
echo("this.styleListArray = new Array();");
if ($result) {
while($row = mysql_fetch_array($result)) {
echo("this.nameArray[" . $counter . "] = '" . $row['user_lname'] . ", " . $row['user_fname'] . "';"); // displays 'lname, fname'
$counter += 1;
}
}
echo("</script>");
And the last thing that we need is to set the onclick event for the body so that when the user clicks anywhere on the page outside of the suggestions box, the suggestions box will hide. And when the user types in the box we need to call the doSuggestionBox function - so we need to set the onkeyup event for the text box.
最后,我们需要为正文设置onclick事件,以便当用户在建议框之外的页面上单击任意位置时,建议框将隐藏。 当用户在框中输入内容时,我们需要调用doSuggestionBox函数-因此我们需要为文本框设置onkeyup事件。
<body onclick="document.getElementById('divSuggestions').style.visibility='hidden'">
onkeyup="doSuggestionBox(this.value);"
-cheers
-干杯
suggestion-box.php proposal-box.php翻译自: https://www.experts-exchange.com/articles/3569/Auto-suggestion-textbox-control-for-your-webpage.html
文本框控件