notifyicon
This tutorial demonstrates one way to create an application that runs
本教程演示了一种创建运行应用程序的方法
without any Forms but still has a GUI presence via an Icon in the System Tray.
没有任何表单,但仍通过系统任务栏中的图标具有GUI存在。
The magic lies in Inheriting from the ApplicationContext Class and passing that to Application.Run() instead of a Form instance. With this approach we automatically get a built-in message pump and do not see any ugly Console windows. Having a message pump means that an infinite loop is not necessary to keep the app "alive". It also means that we can freely create Controls and Forms at run-time and respond to their events as usual. We can even elegantly multi-thread the application by using a BackgroundWorker() Control from within the Inherited ApplicationContext Class.
魔术在于从ApplicationContext类继承并将其传递给Application.Run()而不是Form实例。 通过这种方法,我们会自动获得一个内置的消息泵,并没有看到任何丑陋的控制台窗口。 有一个消息泵意味着一个无限循环是没有必要的,以保持应用程序“活着”。 这也意味着我们可以在运行时自由创建控件和窗体,并照常响应它们的事件。 通过使用Inherited ApplicationContext类中的BackgroundWorker()控件,我们甚至可以优雅地对应用程序进行多线程处理。
Why would you want to do this? Perhaps you have a "background" operation that needs to run all the time and would like to give the user a way to interact with the application. The tray icon gives you the option to display menus or simply popup a configuration dialog if clicked. This is an easy approach compared to implementing a full blown service.
你为什么想做这个? 也许您有一个需要一直运行的“后台”操作,并且希望为用户提供一种与应用程序进行交互的方式。 托盘图标为您提供了显示菜单的选项,或者如果单击,则仅弹出一个配置对话框。 与实施全面服务相比,这是一种简单的方法。
The ApplicationContext provides complete freedom in controlling when the program is closed. You are no longer constrained to just the two shutdown mode choices of "When startup form closes" or "When last form closes". With no "startup form" you can now freely switch between forms without worrying about the app closing on you. You can display a Login form and then actually completely close it before moving on to the Main form. No more need to show the Main form with ShowDialog() or keep the Login form hidden just to keep the app alive! Shared variables in the ApplicationContext class can be used to store information that the entire program needs access to (such as privilege levels). Since the app only shuts down when you explicitly call Application.Exit() you can build a program that only closes when the conditions are right for your particular scenario.
通过ApplicationContext,可以完全自由地控制何时关闭程序。 您不再只限于“关闭启动表单时”或“关闭最后一个表单时”这两个关闭模式选项。 没有“启动表单”,您现在可以在表单之间自由切换,而不必担心应用程序会关闭。 您可以显示一个Login表单,然后在进入Main表单之前实际上完全关闭它。 不再需要使用ShowDialog()显示Main窗体或隐藏Login窗体,仅是为了保持应用程序的生命力! ApplicationContext类中的共享变量可用于存储整个程序需要访问的信息(例如特权级别)。 由于该应用程序仅在您显式调用Application.Exit()时关闭,因此您可以构建仅在条件适合您的特定方案时关闭的程序。
Note that this technique can also be used without any GUI elements at all! The app will happily run without any interface and will still have a message pump making it easier to work with than a Console app.
请注意,这种技术也可以完全不用任何GUI元素使用! 该应用程序将愉快地运行而无需任何界面,并且仍将具有消息泵,这使得它比控制台应用程序更易于使用。
Let's get started:
让我们开始吧:
1.项目类型 (1. Project Type)
从标准WinForms应用程序开始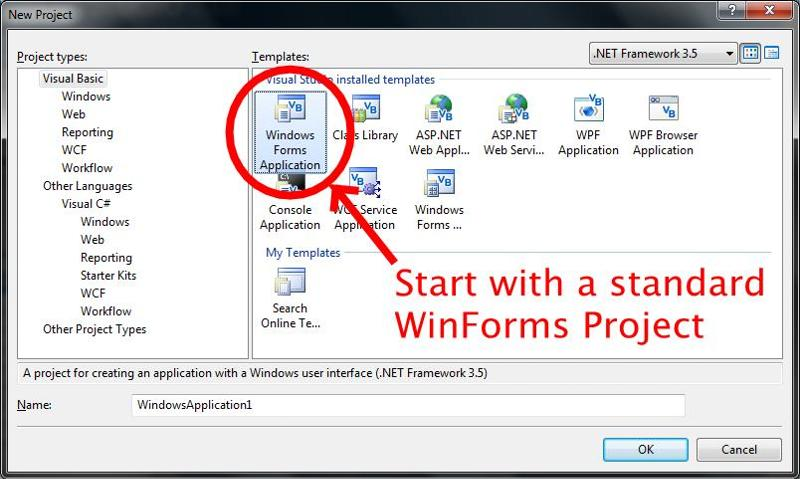
2.项目属性 (2. Project Properties)
单击项目->属性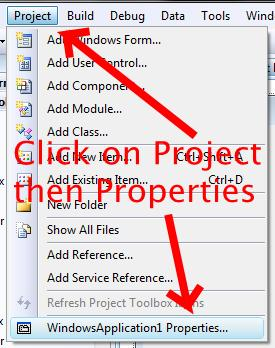
3.更改入口点 (3. Change the Entry Point)
禁用应用程序框架并设置“启动对象”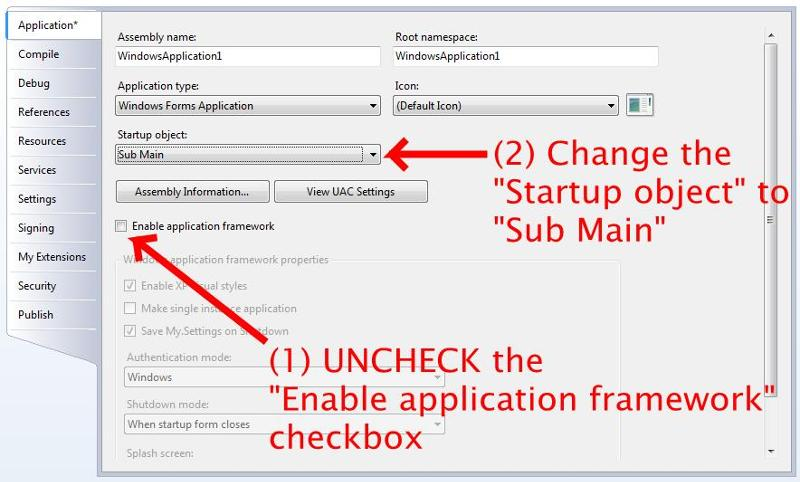
4.添加任务栏图标 (4. Add Tray Icon)
切换到“资源”选项卡并添加您的图标文件(.ico)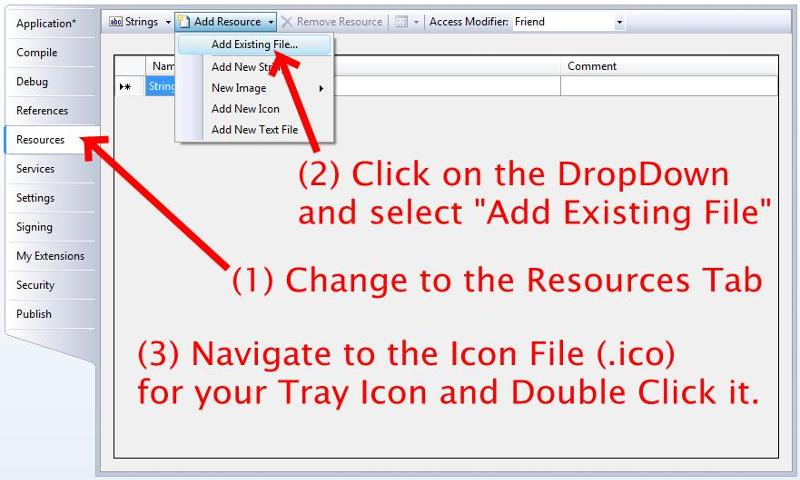
5.嵌入图标 (5. Embed the Icon)
将图标的持久模式更改为嵌入式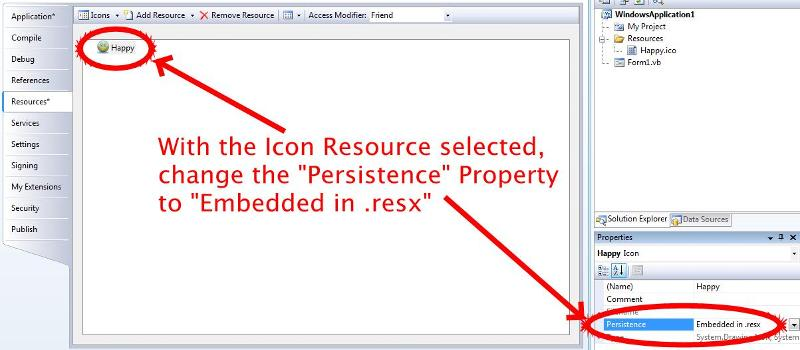
6.添加模块 (6. Add Module)
Add a Module to the Project. Leave the default name, Module1
7.([b]可选[/ b])删除Form1 (7. ([b]Optional[/b]) Remove Form1)
如果不需要,请删除默认表单(Forms can still be used in the application if you desire!)
8.添加代码 (8. Add Code)
用下面的清单替换模块中的代码。Important! Change "My.Resources.Happy" in line #17 so that it correctly reflects the name of your Embedded Icon
重要! 更改“My.Resources.Happy”的行#17,使其正确反映你的嵌入式图标的名称
Module Module1
Public Sub Main()
Application.Run(New MyContext)
End Sub
End Module
Public Class MyContext
Inherits ApplicationContext
Private WithEvents TrayIcon As New NotifyIcon
Private WithEvents CMS As New ContextMenuStrip
Private WithEvents ExitMenu As New ToolStripMenuItem("Exit")
Public Sub New()
TrayIcon.Icon = My.Resources.Happy
TrayIcon.Text = "ApplicationContext with a NotifyIcon" ' <-- Tooltip on the NotifyIcon
TrayIcon.Visible = True
CMS.Items.Add(ExitMenu)
TrayIcon.ContextMenuStrip = CMS
' You can add a BackgroundWorker control to the class and start a lengthy process from here...
End Sub
Private Sub CMS_Opening(ByVal sender As Object, ByVal e As System.ComponentModel.CancelEventArgs) Handles CMS.Opening
' From this event you can control which menu items appear (visibility or disabled) or
' even cancel the event and prevent the context menu from appearing at all
End Sub
Private Sub ExitMenu_Click(ByVal sender As Object, ByVal e As System.EventArgs) Handles ExitMenu.Click
Application.Exit()
End Sub
Private Sub MyContext_ThreadExit(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.ThreadExit
TrayIcon.Visible = False
End Sub
End Class
9.测试一下! (9. Test it out!)
运行项目Hopefully you'll get an Icon in the System Tray that has an "Exit" option when you Right Click it
希望您在系统任务栏中获得一个图标,当您右键单击该图标时,它会带有“退出”选项
In the images below, the Happy face is the icon being displayed by the application:
notifyicon