远程共享桌面
Introduction
介绍
Remote Share is a simple remote sharing tool, enabling you to see, add and remove remote or local shares.
远程共享是一个简单的远程共享工具,使您可以查看,添加和删除远程或本地共享。
The application is written in VB.NET targeting the .NET framework 2.0.
该应用程序以VB.NET为目标,.NET Framework 2.0编写。
The source code and the compiled programs have been included in the article.
本文中包含了源代码和编译的程序。
Usage
用法
Enter a computername, Share Name, Share Path and Share Description if needed
如果需要,输入计算机名,共享名,共享路径和共享描述
You can get a list of shares by pressing the Show button
您可以通过按显示按钮获取股票列表
You can Create a share if the necessary information has been filled in by pressing Create
如果已通过按创建填写了必要的信息,则可以创建共享
You can Remove a share by selecting the share and pressing Remove
您可以通过选择共享并按“删除”来删除共享。
You can Open the share by dubbleclicking on the share listed in the listbox
您可以通过双击列表框中列出的共享来打开共享
Background
背景
This tool has been made to facilitate creating shares on remote machines.
制作此工具是为了方便在远程计算机上创建共享。
Many times it has been very handy, a user wants some files on this machine.
很多时候,它非常方便,用户需要在这台计算机上添加一些文件。
You simply create a share on this machine, browse to the users desktop and place the files
您只需在此计算机上创建共享,浏览到用户桌面并将文件放置
right under their nose...
就在他们的鼻子下面...
Using the code
使用代码
The code is pretty straight forward in using, it exists in 2 versions.
该代码使用起来非常简单,它有2个版本。
A GUI version and a Console version.
GUI版本和控制台版本。
The Main functions are self-explanatory, one for create a share, one for removing a share and of course a function that gives you a list of the available shares on that machine.
主要功能是不言自明的,一个功能用于创建共享,一个功能用于删除共享,当然还有一个功能,可为您提供该计算机上可用共享的列表。
You must an administrator on the remote computer for the connection to be made through WMI.
您必须是远程计算机上的管理员,才能通过WMI建立连接。
For the moment no impersonation is implemented.
目前还没有模拟。
Screenshot
屏幕截图
GUI
图形用户界面
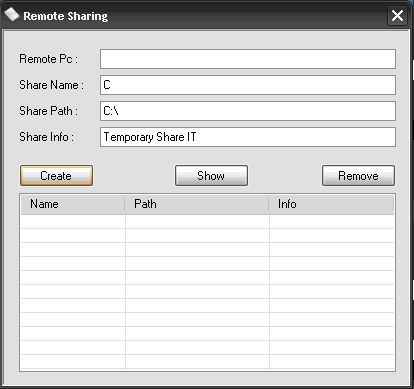
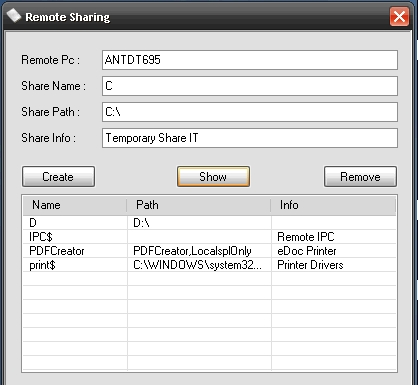
Console
安慰
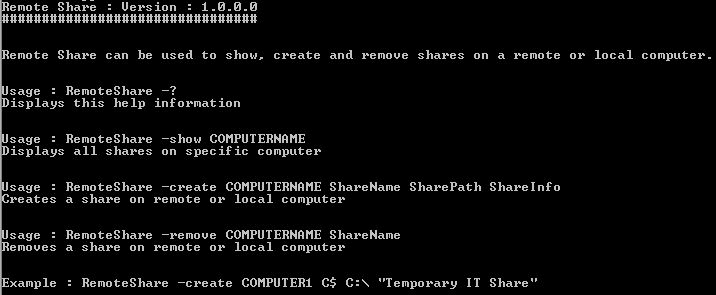
Main Functions
主要功能
All of the functions are based on WMI methods, these methods will do the dirty work for you.
所有功能均基于WMI方法,这些方法将为您完成工作。
What's WMI? Windows Management Instrumentation
什么是WMI? Windows管理规范
Using the .NET Framework namespace System.Management, you can develop applications that obtain enterprise data and automate administrative tasks using Windows Management Instrumentation (WMI). You can also develop applications that provide data to WMI classes using the WMI Provider Extensions.
使用.NET Framework命名空间System.Management,您可以开发应用程序,这些应用程序使用Windows Management Instrumentation(WMI)获取企业数据并自动执行管理任务。 您还可以开发使用WMI提供程序扩展向WMI类提供数据的应用程序。
For more information about WMI please visit the MSDN website
有关WMI的更多信息,请访问MSDN网站。
Create Share
建立分享
This function will allow you to create a share on a local or remote machine using WMI.
使用此功能,您可以使用WMI在本地或远程计算机上创建共享。
To execute the function below, you will have to specify the name of the share, the path, the computer name and if you like a bit of information about the share.
要执行以下功能,您将必须指定共享的名称,路径,计算机名称,以及是否需要有关共享的一些信息。
The most important method in this function is of course the WMI Create Share Method.
当然,此功能中最重要的方法是WMI创建共享方法。
WMI Create Share Function is constructed like this:
WMI创建共享功能的构造如下:
WMIObject.Create(Path,Name
WMIObject.Create(路径,名称 ,类型,最大 妈妈允许的 ,描述 上,密码 d,Win32_Se curityDesc riptor访问)
Create Method of the Win32_Share Class Win32_Share类的创建方法Public Sub CreateShare(ByVal strShareName As String, ByVal strPath As String, ByVal computername As String, ByVal shareinfo As String)
Try
Dim objSWbemServices As Object
Dim objSWbemObject As Object
Dim colSWbemObject As Object
Dim intRet As Integer
Dim blnExists As Boolean
Dim objSWbem As Object
objSWbemServices = GetObject("winmgmts://" + computername + "/root/cimv2")
colSWbemObject = objSWbemServices.InstancesOf("Win32_Share")
For Each objSWbem In colSWbemObject
If (objSWbem.name = strShareName) Then
blnExists = True
Exit For
Else
blnExists = False
End If
Next
If (blnExists = False) Then
objSWbemObject = objSWbemServices.Get("Win32_Share")
intRet = objSWbemObject.Create(strPath, strShareName, 0, 25, shareinfo)
System.Console.WriteLine("Share has been created on : " + computername)
System.Console.WriteLine("ShareName : " + ShareName + " SharePath : " + SharePath + " ShareInfo : " + shareinfo)
Else
System.Console.WriteLine("Share is already present on computer : " + computername)
System.Console.WriteLine("ShareName : " + ShareName + " SharePath : " + SharePath + " ShareInfo : " + shareinfo)
End If
Catch ex As Exception
System.Console.Write("Error occured while trying to create shares on remote pc : " + computername + vbCrLf + "Check if you have the necessary rights and/or that the pc is turned on." + vbCrLf + ex.ToString)
System.Console.WriteLine("ShareName : " + ShareName + " SharePath : " + SharePath + " ShareInfo : " + shareinfo)
End Try
End Sub
Show Shares
显示分享
This function requires the computer name and will make the connection to that machine.
此功能需要计算机名称,并将与该计算机建立连接。
By using a WMI query that gets all instance of Win32_Share will fill the ListView on the form (if you use the GUI version)
通过使用获取Win32_Share的所有实例的WMI查询,将在表单上填充ListView(如果使用GUI版本)
Public Function ShowShares(ByVal ComputerName) As Boolean
Try
Dim objSWbemServices As Object
Dim colSWbemObject As Object
objSWbemServices = GetObject("winmgmts://" + ComputerName + "/root/cimv2")
colSWbemObject = objSWbemServices.InstancesOf("Win32_Share")
' Loop through each share on the machine to see if it already exists
System.Console.WriteLine("Remote Shares on : " + ComputerName)
For Each objSWbem In colSWbemObject
System.Console.WriteLine("ShareName : " + objSWbem.name + " SharePath : " + objSWbem.path + " ShareInfo : " + objSWbem.description)
Next
Catch ex As Exception
System.Console.Write("Error occured while trying to show shares on remote pc : " + ComputerName + vbCrLf + "Check if you have the necessary rights and/or that the pc is turned on." + vbCrLf + ex.ToString)
End Try
End Function
Remove Share
删除分享
This function will allow you to remove shares from the machine either local or remote.
使用此功能可以从本地或远程计算机上删除共享。
Obviously the function needs 2 parameters, the name of the share and the computer name.
显然,该功能需要2个参数,共享名称和计算机名称。
The WMI Query (see snippet below) will collect all the shares with the name provided.
WMI查询(请参见下面的代码段)将使用提供的名称收集所有共享。
Afterwards we'll loop through these objects and simply delete them.
之后,我们将遍历这些对象,然后将其删除。
The method that does the deletion of the share is very simple and looks like this:
删除共享的方法非常简单,如下所示:
WMIObject.Delete()
WMIObject.Delete()
Delete Method of the Win32_Share Class Win32_Share类的删除方法 Public Function RemoveShare(ByVal shareName As String, ByVal computername As String) As Boolean
Try
Dim objSWbemServices As Object
Dim objSWbemObject As Object
Dim colSWbemObject As Object
objSWbemServices = GetObject("winmgmts:{impersonationLevel=impersonate}!\\" & computername & "\root\cimv2")
colSWbemObject = objSWbemServices.ExecQuery("SELECT * FROM Win32_Share WHERE Name = '" + shareName + "'")
For Each objSWbemObject In colSWbemObject
objSWbemObject.Delete()
System.Console.WriteLine("Share has been removed on : " + computername)
System.Console.WriteLine("ShareName : " + shareName)
Next
Catch ex As Exception
System.Console.Write("Error occured while trying to remove shares on remote pc." + vbCrLf + "Check if you have the necessary rights and/or that the pc is turned on." + vbCrLf + ex.ToString)
System.Console.WriteLine("Computername : " + computername + " ShareName : " + shareName)
End Try
End Function
Source Files (VB.NET 2008)
源文件(VB.NET 2008)
Download Source Remote Share GUI 下载源远程共享GUI Download Source Remote Share Console 下载源远程共享控制台Blogs
网志
BlogSpot BlogSpot CodeProject 代码项目Public Class frmRemoteSharing
Public ComputerName As String
Public ShareName As String
Public SharePath As String
Public ShareInfo As String
Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
If Not ComputerName = "" Then
ShowShares(ComputerName)
End If
End Sub
Public Sub CreateShare(ByVal strShareName As String, ByVal strPath As String, ByVal computername As String, ByVal shareinfo As String)
Try
Dim objSWbemServices As Object
Dim objSWbemObject As Object
Dim colSWbemObject As Object
Dim intRet As Integer
Dim blnExists As Boolean
Dim objSWbem As Object
objSWbemServices = GetObject("winmgmts://" + computername + "/root/cimv2")
colSWbemObject = objSWbemServices.InstancesOf("Win32_Share")
For Each objSWbem In colSWbemObject
If (objSWbem.name = strShareName) Then
blnExists = True
Exit For
Else
blnExists = False
End If
Next
If (blnExists = False) Then
objSWbemObject = objSWbemServices.Get("Win32_Share")
intRet = objSWbemObject.Create(strPath, strShareName, 0, 25, shareinfo)
Else
MsgBox("Folder aready shared")
End If
Catch ex As Exception
MsgBox("Error occured while trying to create shares on remote pc." + vbCrLf + "Check if you have the necessary rights and/or that the pc is turned on." + vbCrLf + ex.ToString)
End Try
End Sub
'
Public Function ShowShares(ByVal ComputerName) As Boolean
Try
ListView1.Items.Clear()
Dim objSWbemServices As Object
Dim colSWbemObject As Object
objSWbemServices = GetObject("winmgmts://" + ComputerName + "/root/cimv2")
colSWbemObject = objSWbemServices.InstancesOf("Win32_Share")
' Loop through each share on the machine to see if it already exists
For Each objSWbem In colSWbemObject
Dim lvitem As New ListViewItem
With lvitem
.Text = objSWbem.name
.SubItems.Add(objSWbem.path)
.SubItems.Add(objSWbem.description)
End With
ListView1.Items.Add(lvitem)
Next
Catch ex As Exception
MsgBox("Error occured while trying to show shares on remote pc." + vbCrLf + "Check if you have the necessary rights and/or that the pc is turned on." + vbCrLf + ex.ToString)
End Try
End Function
Public Function RemoveShare(ByVal shareName As String, ByVal computername As String) As Boolean
Try
Dim objSWbemServices As Object
Dim objSWbemObject As Object
Dim colSWbemObject As Object
objSWbemServices = GetObject("winmgmts:{impersonationLevel=impersonate}!\\" & computername & "\root\cimv2")
colSWbemObject = objSWbemServices.ExecQuery("SELECT * FROM Win32_Share WHERE Name = '" + shareName + "'")
For Each objSWbemObject In colSWbemObject
objSWbemObject.Delete()
Next
Catch ex As Exception
MsgBox("Error occured while trying to remove shares on remote pc." + vbCrLf + "Check if you have the necessary rights and/or that the pc is turned on." + vbCrLf + ex.ToString)
End Try
End Function
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
If Not ComputerName = "" And Not ShareName = "" And Not SharePath = "" Then
CreateShare(ShareName, SharePath, ComputerName, ShareInfo)
End If
ShowShares(ComputerName)
End Sub
Private Sub TextBox1_TextChanged(ByVal sender As Object, ByVal e As System.EventArgs) Handles TextBox1.TextChanged
ComputerName = TextBox1.Text
End Sub
Private Sub TextBox2_TextChanged(ByVal sender As Object, ByVal e As System.EventArgs) Handles TextBox2.TextChanged
ShareName = TextBox2.Text
End Sub
Private Sub TextBox3_TextChanged(ByVal sender As Object, ByVal e As System.EventArgs) Handles TextBox3.TextChanged
SharePath = TextBox3.Text
End Sub
Private Sub TextBox4_TextChanged(ByVal sender As Object, ByVal e As System.EventArgs) Handles TextBox4.TextChanged
ShareInfo = TextBox4.Text
End Sub
Private Sub frmRemoteSharing_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
ComputerName = TextBox1.Text
ShareName = TextBox2.Text
SharePath = TextBox3.Text
ShareInfo = TextBox4.Text
End Sub
Private Sub Button3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button3.Click
If Not ListView1.SelectedItems(0).Text = "" Then
RemoveShare(ListView1.SelectedItems(0).Text, ComputerName)
End If
ShowShares(ComputerName)
End Sub
Private Sub ListView1_DoubleClick(ByVal sender As Object, ByVal e As System.EventArgs) Handles ListView1.DoubleClick
Shell("explorer.exe \\" + ComputerName + "\" + ListView1.SelectedItems(0).Text, AppWinStyle.NormalFocus)
End Sub
End Class
Module modMain
Public ComputerName As String
Public ShareName As String
Public SharePath As String
Public ShareInfo As String
Sub main()
Dim i As Integer = 0
For Each Argument As String In My.Application.CommandLineArgs
If i = 0 Then
Select Case LCase(Argument)
Case "-?"
System.Console.WriteLine("Remote Share : Version : " + My.Application.Info.Version.ToString)
System.Console.WriteLine("################################")
System.Console.WriteLine(vbCrLf)
System.Console.WriteLine("Remote Share can be used to show, create and remove shares on a remote or local computer.")
System.Console.WriteLine(vbCrLf)
System.Console.WriteLine("Usage : RemoteShare -?")
System.Console.WriteLine("Displays this help information")
System.Console.WriteLine(vbCrLf)
System.Console.WriteLine("Usage : RemoteShare -show COMPUTERNAME")
System.Console.WriteLine("Displays all shares on specific computer")
System.Console.WriteLine(vbCrLf)
System.Console.WriteLine("Usage : RemoteShare -create COMPUTERNAME ShareName SharePath ShareInfo")
System.Console.WriteLine("Creates a share on remote or local computer")
System.Console.WriteLine(vbCrLf)
System.Console.WriteLine("Usage : RemoteShare -remove COMPUTERNAME ShareName")
System.Console.WriteLine("Removes a share on remote or local computer")
System.Console.WriteLine(vbCrLf)
System.Console.WriteLine("Example : RemoteShare -create COMPUTER1 C$ C:\ " + Chr(34) + "Temporary IT Share" + Chr(34))
Case "-show"
Dim j As Integer = 0
For Each arg As String In My.Application.CommandLineArgs
If j = 1 Then
ComputerName = arg
ShowShares(ComputerName)
Exit Sub
End If
j = j + 1
Next
Case "-create"
Dim j As Integer = 0
For Each arg As String In My.Application.CommandLineArgs
If j = 1 Then
ComputerName = arg
ElseIf j = 2 Then
ShareName = arg
ElseIf j = 3 Then
SharePath = arg
ElseIf j = 4 Then
ShareInfo = arg
End If
j = j + 1
Next
CreateShare(ShareName, SharePath, ComputerName, ShareInfo)
Exit Sub
Case "-remove"
Dim j As Integer = 0
For Each arg As String In My.Application.CommandLineArgs
If j = 1 Then
ComputerName = arg
ElseIf j = 2 Then
ShareName = arg
End If
j = j + 1
Next
RemoveShare(ShareName, ComputerName)
Case Else
System.Console.WriteLine("Unknown argument supplied")
Exit Sub
End Select
End If
i = i + 1
Exit Sub
Next
End Sub
Public Sub CreateShare(ByVal strShareName As String, ByVal strPath As String, ByVal computername As String, ByVal shareinfo As String)
Try
Dim objSWbemServices As Object
Dim objSWbemObject As Object
Dim colSWbemObject As Object
Dim intRet As Integer
Dim blnExists As Boolean
Dim objSWbem As Object
objSWbemServices = GetObject("winmgmts://" + computername + "/root/cimv2")
colSWbemObject = objSWbemServices.InstancesOf("Win32_Share")
For Each objSWbem In colSWbemObject
If (objSWbem.name = strShareName) Then
blnExists = True
Exit For
Else
blnExists = False
End If
Next
If (blnExists = False) Then
objSWbemObject = objSWbemServices.Get("Win32_Share")
intRet = objSWbemObject.Create(strPath, strShareName, 0, 25, shareinfo)
System.Console.WriteLine("Share has been created on : " + computername)
System.Console.WriteLine("ShareName : " + ShareName + " SharePath : " + SharePath + " ShareInfo : " + shareinfo)
Else
System.Console.WriteLine("Share is already present on computer : " + computername)
System.Console.WriteLine("ShareName : " + ShareName + " SharePath : " + SharePath + " ShareInfo : " + shareinfo)
End If
Catch ex As Exception
System.Console.Write("Error occured while trying to create shares on remote pc : " + computername + vbCrLf + "Check if you have the necessary rights and/or that the pc is turned on." + vbCrLf + ex.ToString)
System.Console.WriteLine("ShareName : " + ShareName + " SharePath : " + SharePath + " ShareInfo : " + shareinfo)
End Try
End Sub
'
Public Function ShowShares(ByVal ComputerName) As Boolean
Try
Dim objSWbemServices As Object
Dim colSWbemObject As Object
objSWbemServices = GetObject("winmgmts://" + ComputerName + "/root/cimv2")
colSWbemObject = objSWbemServices.InstancesOf("Win32_Share")
' Loop through each share on the machine to see if it already exists
System.Console.WriteLine("Remote Shares on : " + ComputerName)
For Each objSWbem In colSWbemObject
System.Console.WriteLine("ShareName : " + objSWbem.name + " SharePath : " + objSWbem.path + " ShareInfo : " + objSWbem.description)
Next
Catch ex As Exception
System.Console.Write("Error occured while trying to show shares on remote pc : " + ComputerName + vbCrLf + "Check if you have the necessary rights and/or that the pc is turned on." + vbCrLf + ex.ToString)
End Try
End Function
Public Function RemoveShare(ByVal shareName As String, ByVal computername As String) As Boolean
Try
Dim objSWbemServices As Object
Dim objSWbemObject As Object
Dim colSWbemObject As Object
objSWbemServices = GetObject("winmgmts:{impersonationLevel=impersonate}!\\" & computername & "\root\cimv2")
colSWbemObject = objSWbemServices.ExecQuery("SELECT * FROM Win32_Share WHERE Name = '" + shareName + "'")
For Each objSWbemObject In colSWbemObject
objSWbemObject.Delete()
System.Console.WriteLine("Share has been removed on : " + computername)
System.Console.WriteLine("ShareName : " + shareName)
Next
Catch ex As Exception
System.Console.Write("Error occured while trying to remove shares on remote pc." + vbCrLf + "Check if you have the necessary rights and/or that the pc is turned on." + vbCrLf + ex.ToString)
System.Console.WriteLine("Computername : " + computername + " ShareName : " + shareName)
End Try
End Function
End Module
翻译自: https://www.experts-exchange.com/articles/3272/Remote-Share.html
远程共享桌面