java数组
An array is a collection of similar data types. Array is a container object that hold values of homogeneous type. It is also known as static data structure because size of an array must be specified at the time of its declaration.
数组是相似数据类型的集合。 数组是一个包含齐次类型值的容器对象。 由于数组的大小必须在声明时指定,因此也称为静态数据结构。
Array starts from zero index and goes to n-1 where n is length of the array.
数组从零索引开始,到n-1 ,其中n是数组的长度 。
In Java, array is treated as an object and stores into heap memory. It allows to store primitive values or reference values.
在Java中,数组被视为对象,并存储到堆内存中 。 它允许存储原始值或参考值。
Array can be single dimensional or multidimensional in Java.
数组在Java中可以是一维或多维。
阵列的特征 (Features of Array)
It is always indexed. Index begins from 0.
它总是被索引。 索引从0开始。
It is a collection of similar data types.
它是相似数据类型的集合。
It occupies a contiguous memory location.
它占用一个连续的内存位置。
It allows to access elements randomly.
它允许随机访问元素。
一维数组 (Single Dimensional Array)
Single dimensional array use single index to store elements. You can get all the elements of array by just increment its index by one.
一维数组使用单个索引存储元素。 您可以通过将数组的索引加1来获取数组的所有元素。
数组声明 (Array Declaration)
Syntax :
句法 :
datatype[]arrayName;;
or
datatype arrayName[];
Java allows to declare array by using both declaration syntax, both are valid.
Java允许使用两种声明语法来声明数组,两者均有效。
The arrayName can be any valid array name and datatype can be any like: int, float, byte etc.
arrayName可以是任何有效的数组名称,数据类型可以是类似int,float,byte等的任何类型。
Example :
范例:
int[ ] arr;
char[ ] arr;
short[ ] arr;
long[ ] arr;
int[ ][ ] arr; // two dimensional array.
数组初始化 (Initialization of Array)
Initialization is a process of allocating memory to an array. At the time of initialization, we specify the size of array to reserve memory area.
初始化是将内存分配给数组的过程。 在初始化时,我们指定数组的大小以保留内存区域。
Initialization Syntax
初始化语法
arrayName = new datatype[size]
new
operator is used to initialize an array.
new
运算符用于初始化数组。
The arrayName is the name of array, new is a keyword used to allocate memory and size is length of array.
arrayName是数组的名称,new是用于分配内存的关键字,大小是数组的长度。
We can combine both declaration and initialization in a single statement.
我们可以将声明和初始化结合在一个语句中。
Datatype[] arrayName = new datatype[size]
Example : Create An Array
示例:创建数组
Lets create a single dimensional array.
让我们创建一个一维数组。
class Demo
{
public static void main(String[] args)
{
int[] arr = new int[5];
for(int x : arr)
{
System.out.println(x);
}
}
}
0 0 0 0 0
0 0 0 0 0
In the above example, we created an array arr of int type and can store 5 elements. We iterate the array to access its elements and it prints five times zero to the console. It prints zero because we did not set values to array, so all the elements of the array initialized to 0 by default.
在上面的示例中,我们创建了一个int类型的数组arr ,可以存储5个元素。 我们迭代数组以访问其元素,并将其以零打印五次到控制台。 因为我们没有将值设置为数组,所以它打印为零,因此默认情况下数组的所有元素都初始化为0。
设置数组元素 (Set Array Elements)
We can set array elements either at the time of initialization or by assigning direct to its index.
我们可以在初始化时设置数组元素,也可以直接为其索引分配值。
int[] arr = {10,20,30,40,50};
Here, we are assigning values at the time of array creation. It is useful when we want to store static data into the array.
在这里,我们在创建数组时分配值。 当我们要将静态数据存储到数组中时,这很有用。
or
要么
arr[1] = 105
Here, we are assigning a value to array’s 1 index. It is useful when we want to store dynamic data into the array.
在这里,我们为数组的1索引分配一个值。 当我们要将动态数据存储到数组中时,这很有用。
数组示例 (Array Example)
Here, we are assigning values to array by using both the way discussed above.
在这里,我们通过使用上述两种方式将值分配给数组。
class Demo
{
public static void main(String[] args)
{
int[] arr = {10,20,30,40,50};
for(int x : arr)
{
System.out.println(x);
}
// assigning a value
arr[1] = 105;
System.out.println("element at first index: " +arr[1]);
}
}
10 20 30 40 50 element at first index: 105
第一个索引处的10 20 30 40 50元素:105
访问数组元素 (Accessing array element)
we can access array elements by its index value. Either by using loop or direct index value. We can use loop like: for, for-each or while to traverse the array elements.
我们可以通过其索引值访问数组元素。 通过使用循环或直接索引值。 我们可以使用像这样的循环:for,for-each或while遍历数组元素。
Example to access elements
访问元素示例
class Demo
{
public static void main(String[] args)
{
int[] arr = {10,20,30,40,50};
for(int i=0;i<arr.length;i++)
{
System.out.println(arr[i]);
}
System.out.println("element at first index: " +arr[1]);
}
}
10 20 30 40 50 element at first index: 20
10 20 30 40 50第一索引元素:20
Here, we are traversing array elements using loop and accessed an element randomly.
在这里,我们使用循环遍历数组元素并随机访问一个元素。
Note:-To find the length of an array, we can use length property of array object like: array_name.length
.
注意:-要查找数组的长度,我们可以使用数组对象的length属性,例如: array_name.length
。
多维数组 (Multi-Dimensional Array)
A multi-dimensional array is very much similar to a single dimensional array. It can have multiple rows and multiple columns unlike single dimensional array, which can have only one row index.
多维数组与一维数组非常相似。 它可以具有多行和多列,而与一维数组不同,后者只能有一个行索引。
It represent data into tabular form in which data is stored into row and columns.
它以表格形式表示数据,其中数据按行和列存储。
多维数组声明 (Multi-Dimensional Array Declaration)
datatype[ ][ ] arrayName;
数组初始化 (Initialization of Array)
datatype[ ][ ] arrayName = new int[no_of_rows][no_of_columns];
The arrayName is the name of array, new is a keyword used to allocate memory and no_of_rows and no_of_columns both are used to set size of rows and columns elements.
arrayName是数组的名称, new是用于分配内存的关键字,no_of_rows和no_of_columns都用于设置行和列元素的大小 。
Like single dimensional array, we can statically initialize multi-dimensional array as well.
像一维数组一样,我们也可以静态初始化多维数组。
int[ ][ ] arr = {{1,2,3,4,5},{6,7,8,9,10},{11,12,13,14,15}};
例: (Example:)
class Demo
{
public static void main(String[] args)
{
int arr[ ][ ] = {{1,2,3,4,5},{6,7,8,9,10},{11,12,13,14,15}};
for(int i=0;i<3;i++)
{
for (int j = 0; j < 5; j++) {
System.out.print(arr[i][j]+" ");
}
System.out.println();
}
// assigning a value
System.out.println("element at first row and second column: " +arr[0][1]);
}
}
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 element at first row and second column: 2
第一行和第二列的1 2 3 4 5 6 7 8 9 10 11 12 13 14 15元素:2
锯齿状阵列 (Jagged Array)
Jagged array is an array that has different numbers of columns elements. In java, a jagged array means to have a multi-dimensional array with uneven size of columns in it.
锯齿状数组是具有不同数量的column元素的数组。 在Java中,锯齿状数组意味着要具有一个多维数组,其中包含不均匀的列大小。
锯齿阵列的初始化 (Initialization of Jagged Array)
Jagged array initialization is different little different. We have to set columns size for each row independently.
锯齿状数组的初始化有所不同。 我们必须为每行独立设置列大小。
int[ ][ ] arr = new int[3][ ];
arr[0] = new int[3];
arr[1] = new int[4];
arr[2] = new int[5];
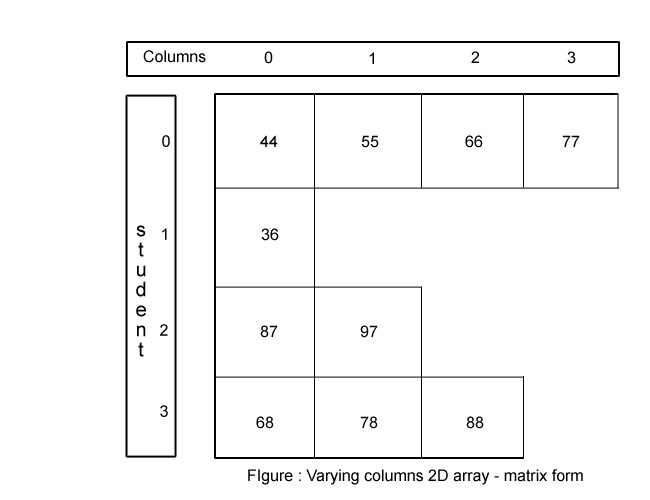
class Demo
{
public static void main(String[] args)
{
int arr[ ][ ] = {{1,2,3},{4,5},{6,7,8,9}};
for(int i=0;i<3;i++)
{
for (int j = 0; j < arr[i].length; j++) {
System.out.print(arr[i][j]+" ");
}
System.out.println();
}
}
}
1 2 3 4 5 6 7 8 9
1 2 3 4 5 6 7 8 9
Here, we can see number of rows are 3 and columns are different for each row. This type of array is called jagged array.
在这里,我们可以看到行数为3 , 每行的列数不同 。 这种数组称为锯齿数组 。
java数组