循环中的continue
In any programming language including C, loops are used to execute a set of statements repeatedly until a particular condition is satisfied.
在包括C在内的任何编程语言中,循环均用于重复执行一组语句,直到满足特定条件为止。
这个怎么运作 (How it Works)
The below diagram depicts a loop execution,
下图描述了循环执行,
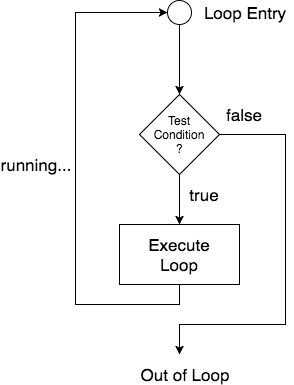
As per the above diagram, if the Test Condition is true, then the loop is executed, and if it is false then the execution breaks out of the loop. After the loop is successfully executed the execution again starts from the Loop entry and again checks for the Test condition, and this keeps on repeating.
如上图所示,如果“测试条件”为true,则执行循环,如果为“ false”,则执行跳出循环。 成功执行循环后,再次从“循环”条目开始执行,并再次检查“测试”条件,此操作不断重复。
The sequence of statements to be executed is kept inside the curly braces { }
known as the Loop body. After every execution of the loop body, condition is verified, and if it is found to be true the loop body is executed again. When the condition check returns false, the loop body is not executed, and execution breaks out of the loop.
要执行的语句序列保存在称为“ 循环”主体的花括号{ }
。 在循环体的每次执行之后,将对条件进行验证,如果发现条件为真 ,则再次执行循环体。 当条件检查返回false时 ,不执行循环主体,并且执行跳出循环。
循环类型 (Types of Loop)
There are 3 types of Loop in C language, namely:
C语言中的Loop有3种类型,分别是:
while
loopwhile
循环for
loopfor
循环do while
loopdo while
循环
while
循环 (while
loop)
while
loop can be addressed as an entry control loop. It is completed in 3 steps.
while
循环可以作为入口控制循环来解决。 分3步完成。
Variable initialization.(e.g
int x = 0;
)变量初始化(例如
int x = 0;
)condition(e.g
while(x <= 10)
)条件(例如
while(x <= 10)
)Variable increment or decrement (
x++
orx--
orx = x + 2
)可变增量或减量(
x++
或x--
或x = x + 2
)
Syntax :
句法 :
variable initialization;
while(condition)
{
statements;
variable increment or decrement;
}
示例:打印前10个自然数的程序 (Example: Program to print first 10 natural numbers)
#include<stdio.h>
void main( )
{
int x;
x = 1;
while(x <= 10)
{
printf("%d\t", x);
/* below statement means, do x = x+1, increment x by 1*/
x++;
}
}
1 2 3 4 5 6 7 8 9 10
1 2 3 4 5 6 7 8 9 10
for
循环 (for
loop)
for
loop is used to execute a set of statements repeatedly until a particular condition is satisfied. We can say it is an open ended loop.. General format is,
for
循环用于重复执行一组语句,直到满足特定条件为止。 我们可以说这是一个开放式循环。 。 通用格式是
for(initialization; condition; increment/decrement)
{
statement-block;
}
In for
loop we have exactly two semicolons, one after initialization and second after the condition. In this loop we can have more than one initialization or increment/decrement, separated using comma operator. But it can have only one condition.
在for
循环中,我们恰好有两个分号,一个在初始化后,另一个在条件后。 在此循环中,我们可以使用逗号运算符分隔多个初始化或增量/减量。 但是它只能有一个条件 。
The for
loop is executed as follows:
for
循环执行如下:
It first evaluates the initialization code.
它首先评估初始化代码。
Then it checks the condition expression.
然后,它检查条件表达式。
If it is true, it executes the for-loop body.
如果为true ,则执行for循环主体。
Then it evaluate the increment/decrement condition and again follows from step 2.
然后,它评估递增/递减条件,并再次从步骤2开始。
When the condition expression becomes false, it exits the loop.
当条件表达式变为false时 ,它退出循环。
示例:打印前10个自然数的程序 (Example: Program to print first 10 natural numbers)
#include<stdio.h>
void main( )
{
int x;
for(x = 1; x <= 10; x++)
{
printf("%d\t", x);
}
}
1 2 3 4 5 6 7 8 9 10
1 2 3 4 5 6 7 8 9 10
嵌套for
循环 (Nested for
loop)
We can also have nested for
loops, i.e one for
loop inside another for
loop. Basic syntax is,
我们也可以嵌套for
循环,即一个for
循环在另一个for
循环内。 基本语法是
for(initialization; condition; increment/decrement)
{
for(initialization; condition; increment/decrement)
{
statement ;
}
}
示例:程序打印一半的数字金字塔 (Example: Program to print half Pyramid of numbers)
#include<stdio.h>
void main( )
{
int i, j;
/* first for loop */
for(i = 1; i < 5; i++)
{
printf("\n");
/* second for loop inside the first */
for(j = i; j > 0; j--)
{
printf("%d", j);
}
}
}
1 21 321 4321 54321
1 21 321 4321 54321
do while
循环 (do while
loop)
In some situations it is necessary to execute body of the loop before testing the condition. Such situations can be handled with the help of do-while
loop. do
statement evaluates the body of the loop first and at the end, the condition is checked using while
statement. It means that the body of the loop will be executed at least once, even though the starting condition inside while
is initialized to be false. General syntax is,
在某些情况下,有必要在测试条件之前执行循环主体。 这种情况可以在do-while
循环的帮助下进行处理。 do
语句首先评估循环的主体,最后使用while
语句检查条件。 这意味着,即使while
里面的启动条件被初始化为false ,循环的主体也将至少执行一次。 一般语法是
do
{
.....
.....
}
while(condition)
示例:程序打印5的前10倍。 (Example: Program to print first 10 multiples of 5.)
#include<stdio.h>
void main()
{
int a, i;
a = 5;
i = 1;
do
{
printf("%d\t", a*i);
i++;
}
while(i <= 10);
}
5 10 15 20 25 30 35 40 45 50
5 10 15 20 25 30 35 40 45 50
跳出循环 (Jumping Out of Loops)
Sometimes, while executing a loop, it becomes necessary to skip a part of the loop or to leave the loop as soon as certain condition becomes true. This is known as jumping out of loop.
有时,在执行循环时,有必要跳过循环的一部分或在某些条件变为true时立即退出循环。 这称为跳出循环。
1)中断声明 (1) break statement )
When break
statement is encountered inside a loop, the loop is immediately exited and the program continues with the statement immediately following the loop.
当在循环内遇到break
语句时,循环将立即退出,程序将在循环后立即继续执行该语句。
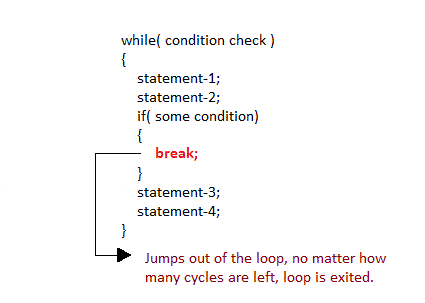
2)继续声明 (2) continue statement)
It causes the control to go directly to the test-condition and then continue the loop process. On encountering continue
, cursor leave the current cycle of loop, and starts with the next cycle.
它使控制直接进入测试条件,然后继续循环过程。 遇到continue
,光标离开当前循环循环,并从下一个循环开始。
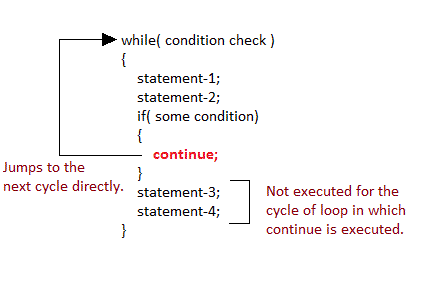
循环中的continue