java数据类型字节
The Byte class is a wrapper class which is used to wraps a value of primitive type byte in an object. An object of type Byte contains a single field whose type is byte.
Byte类是包装器类,用于将原始类型byte的值包装在对象中。 字节类型的对象包含一个类型为字节的字段。
This class is located into java.lang package and provides several methods for converting a byte to a String and vice versa. The declaration of this class is given below.
此类位于java.lang包中,并提供了几种将字节转换为String的方法,反之亦然。 此类的声明在下面给出。
宣言: (Declaration:)
public final class Byte extends Number implements Comparable<Byte>
Now lets see its methods and their usage with the help of examples.
现在,借助示例来了解其方法及其用法。
1. toString() (1. toString() )
句法: (Syntax:)
public String toString(byte b)
例: (Example:)
Lets take an example to get string object of a byte type. We used toString()
method which is static so we can call it by using the class name.
让我们以获取字节类型的字符串对象为例。 我们使用了toString()
方法,该方法是静态的,因此我们可以使用类名来调用它。
public class ByteDemo1
{
public static void main(String[] args)
{
byte a = 65;
System.out.println("toString(a) = " + Byte.toString(a));
}
}
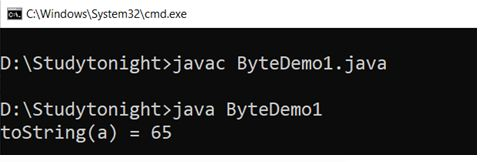
2. valueOf() (2. valueOf() )
This method returns a Byte instance representing the specified byte value. This method should generally be used in preference to the constructor Byte(byte).
此方法返回一个Byte实例,该实例表示指定的字节值。 通常应优先于构造方法Byte(byte)使用此方法。
句法: (Syntax:)
public static Byte valueOf(byte b)
例: (Example:)
In this example, we are using valueOf()
method that returns instance of Byte class which represents the specified byte type.
在此示例中,我们使用valueOf()
方法返回表示指定字节类型的Byte类的实例。
public class ByteDemo1
{
public static void main(String[] args)
{
byte a = 65;
String b = "25";
Byte x = Byte.valueOf(a);
System.out.println("ValueOf(a) = " + x);
x = Byte.valueOf(b, 6);
System.out.println("ValueOf(a,6) = " + x);
}
}
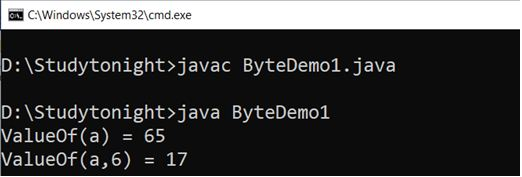
3. parseByte() (3. parseByte())
This method returns a byte value of the specified string val. We can use it to get a byte value from string type value.
此方法返回指定字符串val的字节值。 我们可以使用它从字符串类型值中获取字节值。
public static byte parseByte(String val, int radix) throws NumberFormatException
例: (Example:)
Lets take an example in which we have a string type variable and getting its byte value using the parseByte() method.
让我们举一个例子,其中我们有一个字符串类型的变量,并使用parseByte()方法获取其字节值。
public class ByteDemo1
{
public static void main(String[] args)
{
byte a = 65;
String b = "25";
byte x = Byte.parseByte(b);
System.out.println("parseByte(b) = " + x);
x = Byte.parseByte(b, 6);
System.out.println("parseByte(b,6) = " + x);
}
}
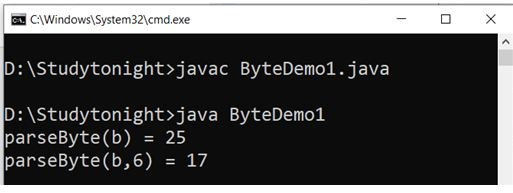
4.解码() (4. decode() )
This method is used to decode a String into a Byte. It accepts decimal, hexadecimal, and octal numbers.
此方法用于将字符串解码为字节。 它接受十进制,十六进制和八进制数字。
句法: (Syntax:)
public static Byte decode(String s) throws NumberFormatException
例: (Example:)
We can use decode method to decode string type to a byte object. See the below example.
我们可以使用解码方法将字符串类型解码为字节对象。 请参见以下示例。
public class ByteDemo1
{
public static void main(String[] args)
{
String a = "25";
String b = "006";
String c = "0x0f";
Byte x = Byte.decode(a);
System.out.println("decode(25) = " + x);
x = Byte.decode(b);
System.out.println("decode(006) = " + x);
x= Byte.decode(c);
System.out.println("decode(0x0f) = " + x);
}
}
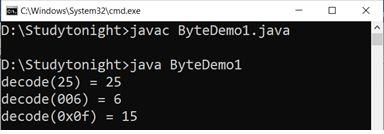
5. byteValue() (5. byteValue() )
This method is used to get a primitive type byte value from Byte object. It returns the numeric value represented by this object after conversion to type byte.
此方法用于从Byte对象获取原始类型的字节值。 转换为字节类型后,它将返回此对象表示的数值。
句法 (Syntax)
public byte byteValue()
6. shortValue() (6. shortValue() )
This method returns the value of this Byte as a short after a widening primitive conversion.
在扩展原始转换之后,此方法以短促形式返回此Byte的值。
句法 (Syntax)
public short shortValue()
7. intValue() (7. intValue() )
The intValue()
method returns the value of this Byte as a primitive int type after a widening primitive conversion.
在扩展原始转换后, intValue()
方法以原始int类型返回此Byte的值。
句法 (Syntax)
public int intValue()
8. longValue() (8. longValue() )
The longValue()
method returns the value of this Byte type as a long type after a widening primitive conversion.
longValue()
方法在扩展原语转换后,以长类型返回此Byte类型的值。
句法 (Syntax)
public long longValue()
9. doubleValue() (9. doubleValue() )
It returns the value of this Byte type as a double type after a widening primitive conversion.
在扩展原语转换之后,它以双精度类型返回此Byte类型的值。
句法 (Syntax)
public double doubleValue()
10. floatValue() (10. floatValue() )
This method is used to get value of this Byte type as a float type after a widening primitive conversion.
在扩展原始转换之后,此方法用于获取此Byte类型的值作为浮点类型。
句法: (Syntax:)
public float floatValue()
例: (Example:)
Lets take an example to convert byte type to int, long and float type values. In this example, we are using intValue(), floatValue(), doubleValue()
methods.
让我们举一个将字节类型转换为int,long和float类型值的示例。 在此示例中,我们使用intValue(), floatValue(), doubleValue()
方法。
public class ByteDemo1
{
public static void main(String[] args)
{
Byte a = 45;
Byte obj = new Byte(a);
System.out.println("bytevalue(obj) = " + obj.byteValue());
System.out.println("shortvalue(obj) = " + obj.shortValue());
System.out.println("intvalue(obj) = " + obj.intValue());
System.out.println("longvalue(obj) = " + obj.longValue());
System.out.println("doublevalue(obj) = " + obj.doubleValue());
System.out.println("floatvalue(obj) = " + obj.floatValue());
}
}
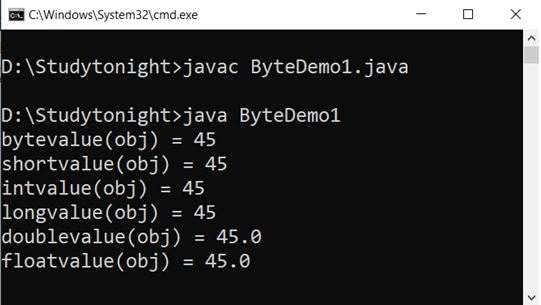
11. hashCode() (11. hashCode() )
This method is used to get hash code of a byte value. It returns an int value of byte object.
此方法用于获取字节值的哈希码。 它返回字节对象的int值。
句法: (Syntax:)
public inthashCode()
例: (Example:)
public class ByteDemo1
{
public static void main(String[] args)
{
Byte a = 45;
Byte obj = new Byte(a);
int x =obj.hashCode();
System.out.println("hashcode(obj) = " + x);
}
}
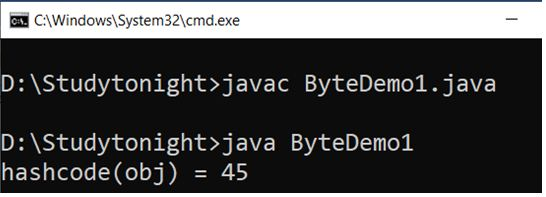
12. equals() (12. equals())
The equals()
method compares an object to the specified object. It returns true if objects are same; false otherwise.
equals()
方法将一个对象与指定对象进行比较。 如果对象相同,则返回true;否则,返回true。 否则为假。
句法: (Syntax:)
public boolean equals(Object obj)
例: (Example:)
We are comparing two byte objects using the equals method that returns true if both the objects are true.
我们正在使用equals方法比较两个字节的对象,如果两个对象都为true,则返回true。
public class ByteDemo1
{
public static void main(String[] args)
{
Byte a = 45;
String b ="25";
Byte obj = new Byte(a);
Byte obj1 = new Byte(b);
boolean z = obj.equals(obj1);
System.out.println("obj.equals(obj1) = " + z);
}
}
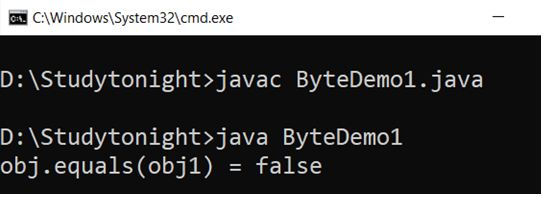
13. compareTo() (13. compareTo() )
This method is used to compare two byte objects numerically. It returns 0, if the both byte objects are equal. It returns less the 0, if one byte object is less than argument object. It returns greater than 0, if one byte object is numerically greater that the argument byte object.
此方法用于数字比较两个字节对象。 如果两个字节对象相等,则返回0。 如果一个字节对象小于参数对象,则返回的值小于0。 如果一个字节对象在数值上大于参数字节对象,则返回大于0。
句法: (Syntax:)
public int compareTo(Byte anotherByte)
public int compareTo(Byte anotherByte)
例: (Example:)
In this example, we are comparing two bytes objects using compareTo()
method that compares two byte objects numerically and returns a numeric value.
在此示例中,我们使用compareTo()
方法比较两个字节对象,该方法对两个字节对象进行数字比较并返回一个数值。
public class ByteDemo1
{
public static void main(String[] args)
{
Byte a = 45;
String b ="25";
Byte obj = new Byte(a);
Byte obj1 = new Byte(b);
int z = obj.compareTo(obj1);
System.out.println("obj.compareTo(obj1) = " + z);
}
}
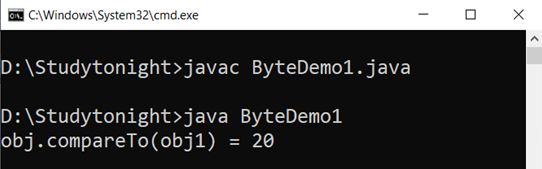
14. compare() (14. compare() )
It is used to compare two byte values numerically. The value returned is identical to what would be returned by.
它用于数字比较两个字节值。 返回的值与将返回的值相同。
句法: (Syntax:)
public static intcompare(byte x,byte y)
例: (Example:)
We can use compare method to compare two byte values. It returns 0 if both are equal else returns either negative or positive numerical value.
我们可以使用compare方法比较两个字节的值。 如果两者相等,则返回0,否则返回正数或负数。
public class ByteDemo1
{
public static void main(String[] args)
{
Byte a = 45;
String b ="25";
Byte obj = new Byte(a);
Byte obj1 = new Byte(b);
int z = Byte.compare(obj, obj1);
System.out.println("compare(obj, obj1) = " + z);
}
}
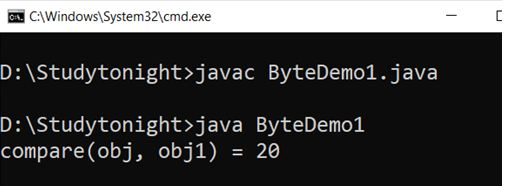
java数据类型字节