Short class is a wrapper class that is use to wrap a value of primitive short type in an object type.
短类是包装器类,用于将原始短类型的值包装为对象类型。
An object of type Short contains a single field whose type is short.
类型为Short的对象包含一个类型为short的字段。
This class provides several methods for converting a short to a String and a String to a short, as well as other constants and methods helpful while working with a short type. Declaration of short type is given below.
此类提供了几种将short转换为String和将String转换为short的方法,以及在使用short类型时有用的其他常量和方法。 简短类型的声明如下。
宣言: (Declaration:)
public final class Short extends Number implements Comparable<Short>
Here are the Methods of Short class and their example.
这是Short类的方法及其示例。
1. toString() (1. toString())
This method is used to get string representation of byte object. It returns a new String object representing the specified short. It takes String type single argument.
此方法用于获取字节对象的字符串表示形式。 它返回一个表示指定short的新String对象。 它采用String类型的单个参数。
句法: (Syntax: )
public String toString(short b)
例: (Example:)
In this example, we are using toString()
method to get string representation of a short type object.
在此示例中,我们使用toString()
方法获取简短类型对象的字符串表示形式。
public class ShortDemo1
{
public static void main(String[] args)
{
short a = 45;
String b ="25";
Short obj = new Short(a);
Short obj1 = new Short(b);
System.out.println("toString(a) = " + Short.toString(a));
}
}
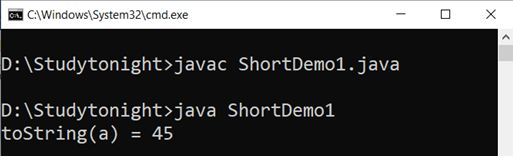
2. valueOf() (2. valueOf())
This method returns a Short instance representing the specified short value. This method should generally be used in preference to the constructor Short(short). It takes a single parameter of short type.
此方法返回表示指定short值的Short实例。 通常应优先于构造方法Short(short)使用此方法。 它采用短类型的单个参数。
句法: (Syntax:)
public static Short valueOf(short b)
例: (Example:)
In this example, we are using valueOf()
method that returns instance of Short class which represents the specified short type.
在此示例中,我们使用valueOf()
方法返回表示指定的short类型的Short类的实例。
public class ShortDemo1
{
public static void main(String[] args)
{
short a = 45;
String b ="25";
Short obj = new Short(a);
Short obj1 = new Short(b);
Short x = Short.valueOf(a);
System.out.println("valueOf(a) = " + x);
x = Short.valueOf(b);
System.out.println("ValueOf(b) = " + x);
x = Short.valueOf(b, 6);
System.out.println("ValueOf(b,6) = " + x);
}
}
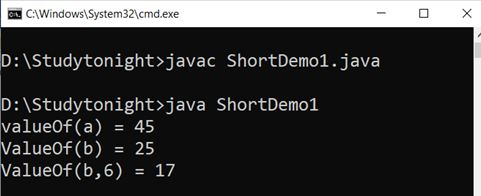
3. parseShort() (3. parseShort())
This method returns a short value of the specified string value. We can use it to get a short value from string type value. It takes two parameters: one is String type and second is int type.
此方法返回指定字符串值的短值。 我们可以使用它从字符串类型值中获取一个短值。 它有两个参数:一个是String类型,第二个是int类型。
句法: (Syntax:)
public static short parseShort(String val, int radix) throws NumberFormatException
例: (Example:)
Lets take an example in which we have a string type variable and getting its short value using the parseShort()
method.
让我们举一个例子,其中我们有一个字符串类型的变量,并使用parseShort()
方法获取它的短值。
public class ShortDemo1
{
public static void main(String[] args)
{
short a = 45;
String b ="25";
Short obj = new Short(a);
Short obj1 = new Short(b);
Short x = Short.parseShort(b);
System.out.println("parseShort(b) = " + x);
x = Short.valueOf(b, 6);
System.out.println("parseShort(b,6) = " + x);
}
}
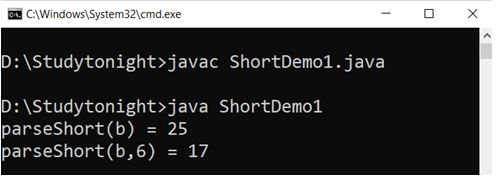
4.解码() (4. decode())
This method is used to decode a String into a Short. It accepts decimal, hexadecimal, and octal numbers. It takes a single parameter of String type.
此方法用于将String解码为Short。 它接受十进制,十六进制和八进制数字。 它采用String类型的单个参数。
public static Short decode(String s) throws NumberFormatException
例: (Example:)
We can use decode method to decode string type to a short object. See the below example.
我们可以使用解码方法将字符串类型解码为短对象。 请参见以下示例。
public class ShortDemo1
{
public static void main(String[] args)
{
String a = "25";
String b = "007";
String c = "0x0f";
Short x = Short.decode(a);
System.out.println("decod(45) = " + x);
x = Short.decode(b);
System.out.println("decode(005) = " + x);
x = Short.decode(c);
System.out.println("decode(0x0f) = " + x);
}
}
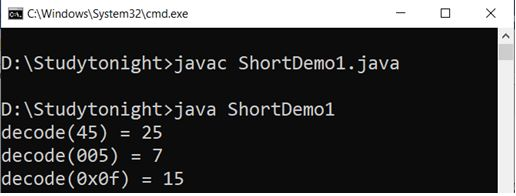
5. byteValue() (5. byteValue())
This method is used to get a primitive type byte value from Short object. It returns the numeric value represented by this object after conversion to type byte.
此方法用于从Short对象获取原始类型的字节值。 转换为字节类型后,它将返回此对象表示的数值。
句法: (Syntax:)
public byte byteValue()
6. shortValue() (6. shortValue() )
This method returns the value of this Short as a short after a widening primitive conversion.
此方法在扩展原始转换后以short的形式返回此Short的值。
句法: (Syntax:)
public short shortValue()
7. intValue() (7. intValue() )
The intValue()
method returns the value of this Short as a primitive int type after a widening primitive conversion.
在扩展原始转换后, intValue()
方法将此Short的值作为原始int类型返回。
句法: (Syntax:)
Syntax : public int intValue()
8. longValue() (8. longValue() )
The longValue()
method returns the value of this Short type as a long type after a widening primitive conversion.
longValue()
方法在扩展原语转换后,以Long类型返回此Short类型的值。
句法: (Syntax:)
public long longValue()
9. doubleValue() (9. doubleValue() )
It returns the value of this Short type as a double type after a widening primitive conversion.
扩展原始转换后,它以Double类型返回此Short类型的值。
句法: (Syntax:)
public double doubleValue()
10. floatValue() (10. floatValue() )
This method is used to get value of this Short type as a float type after a widening primitive conversion.
在扩展原始图元转换之后,此方法用于获取此Short类型的值作为浮点类型。
句法: (Syntax:)
public float floatValue()
例: (Example:)
Lets take an example to convert short type to int, long and float type values. In this example, we are using intValue(), floatValue(), doubleValue()
methods.
让我们以将short类型转换为int,long和float类型的值为例。 在此示例中,我们使用intValue(), floatValue(), doubleValue()
方法。
public class ShortDemo1
{
public static void main(String[] args)
{
short a = 75;
Short obj = new Short(a);
System.out.println("bytevalue(obj) = " + obj.byteValue());
System.out.println("shortvalue(obj) = " + obj.shortValue());
System.out.println("intvalue(obj) = " + obj.intValue());
System.out.println("longvalue(obj) = " + obj.longValue());
System.out.println("doublevalue(obj) = " + obj.doubleValue());
System.out.println("floatvalue(obj) = " + obj.floatValue());
}
}
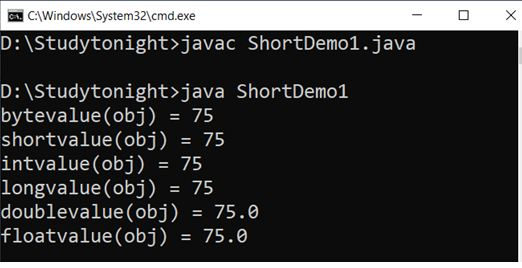
11. hashCode() (11. hashCode() )
This method is used to get hash code of a short value. It returns an int value of short object.
此方法用于获取短值的哈希码。 它返回short对象的int值。
句法: (Syntax:)
public inthashCode()
例: (Example:)
public class ShortDemo1
{
public static void main(String[] args)
{
short a = 45;
Short obj = new Short(a);
int x =obj.hashCode();
System.out.println("hashcode(obj) = " + x);
}
}
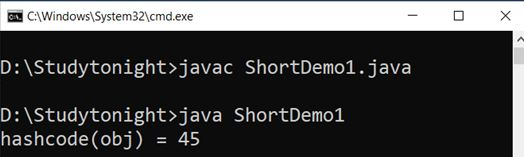
12. equals() (12. equals() )
The equals()
method compares an object to the specified object. It returns true if objects are same; false otherwise
equals()
方法将一个对象与指定对象进行比较。 如果对象相同,则返回true;否则,返回true。 否则为假
句法: (Syntax:)
public boolean equals(Object obj)
例: (Example:)
We are comparing two byte objects using the equals method that returns true if both the objects are true.
我们正在使用equals方法比较两个字节的对象,如果两个对象都为true,则返回true。
public class ShortDemo1
{
public static void main(String[] args)
{
short a = 45;
String b ="25";
Short obj = new Short(a);
Short obj1 = new Short(b);
boolean z = obj.equals(obj1);
System.out.println("obj.equals(obj1) = " + z);
}
}
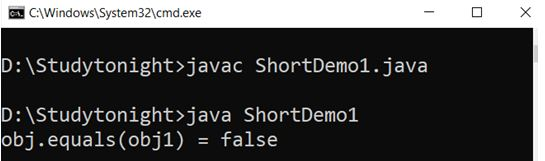
13. compareTo() (13. compareTo() )
This method is used to compare two short objects numerically. It returns 0, if the both short objects are equal. It returns less the 0, if one short object is less than argument object. It returns greater than 0, if one short object is numerically greater than the argument short object.
此方法用于数值比较两个短对象。 如果两个短对象相等,则返回0。 如果一个短对象小于参数对象,则返回的值小于0。 如果一个short对象在数量上大于参数short对象,则返回大于0的值。
句法: (Syntax:)
public intcompareTo(Short b)
例: (Example:)
In this example, we are comparing two short objects using compareTo method that compares two short objects numerically and returns a numeric value.
在此示例中,我们使用compareTo方法比较两个短对象,该方法对两个短对象进行数字比较并返回一个数值。
public class ShortDemo1
{
public static void main(String[] args)
{
short a = 45;
String b ="25";
Short obj = new Short(a);
Short obj1 = new Short(b);
int z = obj.compareTo(obj1);
System.out.println("obj.compareTo(obj1) = " + z);
}
}
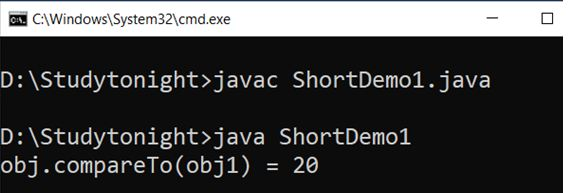
14. compare() (14. compare() )
It is used to compare two byte values numerically. The value returned is identical to what would be returned by.
它用于数字比较两个字节值。 返回的值与将返回的值相同。
句法: (Syntax:)
public static int compare(short x,short y)
例: (Example:)
We can use compare method to compare two short values. It returns 0 if both are equal else returns either negative or positive numerical value.
我们可以使用比较方法比较两个短值。 如果两者相等,则返回0,否则返回正数或负数。
public class ShortDemo1
{
public static void main(String[] args)
{
short a = 45;
String b ="25";
Short obj = new Short(a);
Short obj1 = new Short(b);
int z = Short.compare(obj, obj1);
System.out.println("compare(obj, obj1) = " + z);
}
}
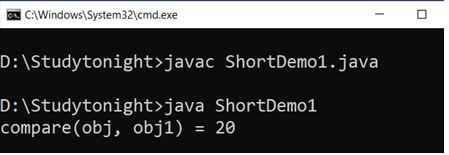
15. reverseBytes() (15. reverseBytes() )
This method is used to get value obtained by reversing the order of the bytes in the two's complement representation of the specified short value.
此方法用于获取通过反转指定的short值的二进制补码表示形式的字节顺序而获得的值。
句法: (Syntax:)
public static short reverseBytes(short val)
例: (Example:)
We are getting a value of a short after reversing its bytes. The returned value is different from the argument value.
反转字节后,我们得到一个short值。 返回的值与参数值不同。
public class ShortDemo1
{
public static void main(String[] args)
{
short a = 25;
System.out.println("Short.reverseBytes(a) = " + Short.reverseBytes(a));
}
}
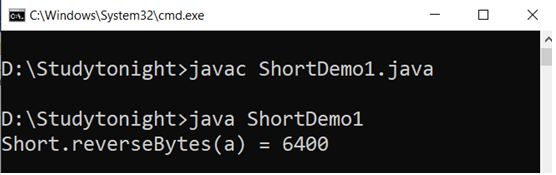