float java
The Float class wraps a value of primitive type float in an object. An object of type Float contains a single field whose type is float.
Float类将原始类型float的值包装在对象中。 Float类型的对象包含一个类型为float的字段。
In addition, this class provides several methods for converting a float to a String and a String to a float, as well as other constants and methods useful when dealing with a float.
此外,此类提供了几种将float转换为String并将String转换为float的方法,以及在处理float时有用的其他常量和方法。
宣言: (Declaration:)
public final class Float extends Number implements Comparable<Float>
Below are the Methods of Float class and their example.
下面是Float方法类及其示例。
1. toString() (1. toString())
It returns a new String representing of specified float object. Syntax of the method is given below.
它返回一个新的String,它表示指定的float对象。 该方法的语法如下。
句法: (Syntax:)
public String toString(float b)
例: (Example:)
Lets take an example to get string object of a float type. We used toString()
method which is static so we can call it by using the class name.
让我们以获取浮点类型的字符串对象为例。 我们使用了toString()
方法,该方法是静态的,因此我们可以使用类名来调用它。
public class FloatDemo1
{
public static void main(String[] args)
{
float a = 72.05F;
System.out.println("toString(a) = " + Float.toString(a));
}
}
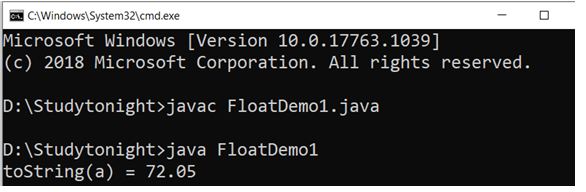
2. valueOf() (2. valueOf())
This method returns a Float instance representing the specified float value. This method should generally be used in preference to the constructor Float(float).
此方法返回一个Float实例,该实例表示指定的float值。 通常应优先于构造方法Float(float)使用此方法。
句法 (Syntax)
public static Float valueOf(float b)
例: (Example:)
In this example, we are using valueOf() method that returns instance of Float class which represents the specified float type.
在此示例中,我们使用valueOf()方法返回表示指定浮点类型的Float类的实例。
public class FloatDemo1
{
public static void main(String[] args)
{
float a = 72.05F;
String b = "63";
Float x = Float.valueOf(a);
System.out.println("valueOf(a) = " + x);
x = Float.valueOf(b);
System.out.println("ValueOf(b) = " + x);
}
}
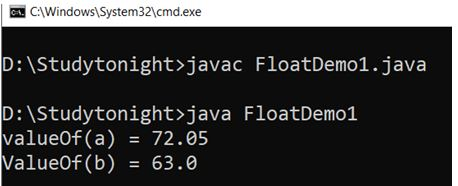
3. parseFloat() (3. parseFloat())
This method returns a float value of the specified string value. We can use it to get a float value from string type value.
此方法返回指定字符串值的浮点值。 我们可以使用它从字符串类型值中获取浮点值。
句法: (Syntax:)
public static float parseFloat(String val) throws NumberFormatException
例: (Example:)
Lets take an example in which we have a string type variable and getting its float value using the parseFloat()
method.
让我们举一个例子,其中我们有一个字符串类型的变量,并使用parseFloat()
方法获取其float值。
public class FloatDemo1
{
public static void main(String[] args)
{
float a = 72.05F;
String b = "63";
float x = Float.parseFloat(b);
System.out.println("parseFloat(b) = " + x);
}
}
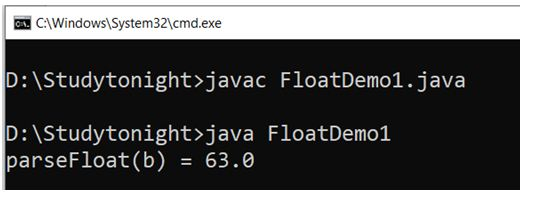
4. byteValue() (4. byteValue() )
This method is used to get a primitive type float value from Float object. It returns the numeric value represented by this object after conversion to type float.
此方法用于从Float对象获取基本类型的float值。 转换为float类型后,它将返回此对象表示的数值。
句法 (Syntax)
public byte byteValue()
5. shortValue() (5. shortValue() )
This method returns the value of this Float as a short after a widening primitive conversion.
在扩展原始图元转换之后,此方法以短暂的形式返回此Float的值。
句法 (Syntax)
public short shortValue()
6. intValue() (6. intValue() )
The intValue()
method returns the value of this Float as a primitive int type after a widening primitive conversion. Syntax of this method is given below.
在扩展原始转换之后, intValue()
方法以原始int类型返回此Float的值。 该方法的语法如下。
句法 (Syntax)
public int intValue()
7. longValue() (7. longValue() )
The longValue()
method returns the value of this Float type as a long type after a widening primitive conversion. Syntax of this method is given below.
longValue()
方法在扩展原始转换后,以长型返回此Float类型的值。 该方法的语法如下。
句法 (Syntax)
public long longValue()
8. doubleValue() (8. doubleValue() )
It returns the value of this Float type as a double type after a widening primitive conversion. Syntax of this method is given below.
扩展原始图元转换后,它将将此Float类型的值作为double类型返回。 该方法的语法如下。
句法 (Syntax)
public double doubleValue()
9. floatValue() (9. floatValue() )
This method is used to get value of this Float type as a float type after a widening primitive conversion. Syntax of this method is given below.
在扩展原始图元转换之后,此方法用于获取此Float类型的值作为float类型。 该方法的语法如下。
句法: (Syntax:)
public float floatValue()
例: (Example:)
Lets take an example to convert float type to int, long and float type values. In this example, we are using intValue(), floatValue(), doubleValue()
methods.
让我们以将float类型转换为int,long和float类型的值为例。 在此示例中,我们使用intValue(), floatValue(), doubleValue()
方法。
public class FloatDemo1
{
public static void main(String[] args)
{
float a = 72.05F;
Float obj = new Float(a);
System.out.println("bytevalue(obj) = " + obj.byteValue());
System.out.println("shortvalue(obj) = " + obj.shortValue());
System.out.println("intvalue(obj) = " + obj.intValue());
System.out.println("longvalue(obj) = " + obj.longValue());
System.out.println("doublevalue(obj) = " + obj.doubleValue());
System.out.println("floatvalue(obj) = " + obj.floatValue());
}
}
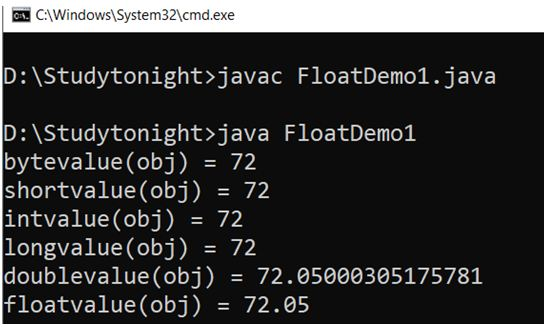
10. hashCode() (10. hashCode())
This method is used to get hash code of a float value. It returns an int value of float object.
此方法用于获取浮点值的哈希码。 它返回一个float对象的int值。
句法: (Syntax:)
public inthashCode()
例: (Example:)
public class FloatDemo1
{
public static void main(String[] args)
{
float a = 72.05F;
Float obj = new Float(a);
int x = obj.hashCode();
System.out.println("hashcode(x) = " + x);
}
}
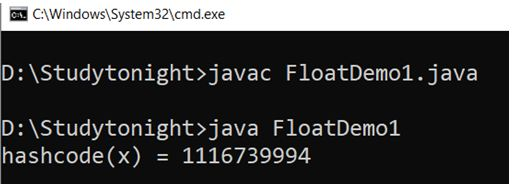
11. isNaN() (11. isNaN() )
This method returns a boolean value either true or false. It returns true, if this Float value is a Not-a-Number (NaN), false otherwise.
此方法返回布尔值true或false。 如果此Float值是一个非数字(NaN) ,则返回true,否则返回false。
句法: (Syntax:)
public booleanisNaN()
例: (Example:)
Lets take an example to check whether the given float value is NaN or not. See the below example.
让我们以一个示例检查给定的float值是否为NaN。 请参见以下示例。
public class FloatDemo1
{
public static void main(String[] args)
{
float a = 72.05F;
Float obj = new Float(a);
Float x = Float.valueOf(a);
System.out.println("isNaN(d) = " + x.isNaN());
}
}
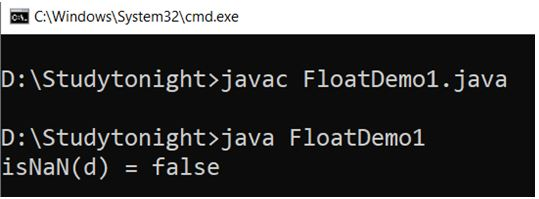
12. isInfinite() (12. isInfinite() )
This method is used to check whether the float value is infinitely large in magnitude. It returns a boolean value either true or false. Syntax of this method is given below.
此方法用于检查浮点值的大小是否无限大。 它返回布尔值true或false。 该方法的语法如下。
句法: (Syntax:)
public booleanisInfinite()
例: (Example:)
We can use this method to check the range of Float value whether it lies under infinitely or not.
我们可以使用此方法检查Float值的范围是否在无限范围内。
public class FloatDemo1
{
public static void main(String[] args)
{
float a = 72.05F;
Float obj = new Float(a);
Float x = Float.valueOf(a);
x = Float.valueOf(Float.POSITIVE_INFINITY + 1);
System.out.println("Float.isInfinite(x) = " + Float.isInfinite(x.floatValue()));
}
}
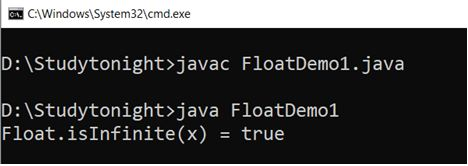
13. toHexString() (13. toHexString())
This method is used to get a hexadecimal string representation of the float argument. It takes a float type argument that would be convert into hexadecimal value. Syntax of this method is given below.
此方法用于获取float参数的十六进制字符串表示形式。 它使用一个float类型的参数,该参数将转换为十六进制值。 该方法的语法如下。
句法: (Syntax:)
public static String toHexString(float val)
例: (Example:)
public class FloatDemo1
{
public static void main(String[] args)
{
float a = 72.05F;
Float obj = new Float(a);
System.out.println("Float.toString(a) = " + Float.toHexString(a));
}
}
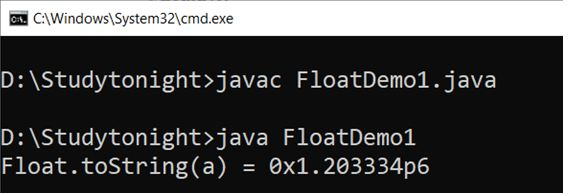
14. floatToIntBits() (14. floatToIntBits() )
This method is used to get representation of the specified floating-point value according to the IEEE 754 floating-point "single format" bit layout. It takes a floating-point argument. Syntax of this method is given below.
此方法用于根据IEEE 754浮点“单一格式”位布局来获取指定浮点值的表示形式。 它需要一个浮点参数。 该方法的语法如下。
句法: (Syntax:)
public static intfloatToIntBits(float val)
例: (Example:)
In this example, we are using floattointbits()
method that returns a bit layout of floating-point value.
在此示例中,我们使用floattointbits()
方法返回浮点值的位布局。
public class FloatDemo1
{
public static void main(String[] args)
{
float a = 72.05F;
Float obj = new Float(a);
int x = Float.floatToIntBits(a);
System.out.println("Float.floatToLongBits(a) = " + x);
}
}
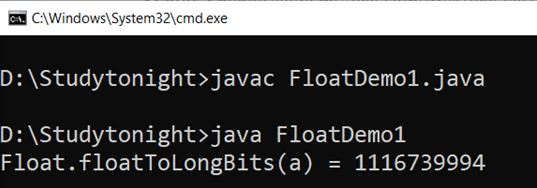
15. floatToRawIntBits() (15. floatToRawIntBits())
This method returns a representation of the specified floating-point value according to the IEEE 754 floating-point "single format" bit layout, preserving Not-a-Number (NaN) values. Syntax of this method is given below.
此方法根据IEEE 754浮点“单一格式”位布局返回指定的浮点值的表示形式,并保留非数字(NaN)值。 该方法的语法如下。
句法: (Syntax:)
public static intfloatToRawIntBits(float val)
例: (Example:)
public class FloatDemo1
{
public static void main(String[] args)
{
float a = 72.05F;
Float obj = new Float(a);
int x = Float.floatToRawIntBits(a);
System.out.println("Float.floatToRawIntBits(a) = " + x);
}
}
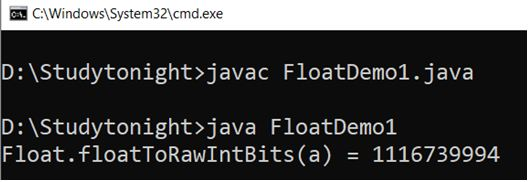
16. IntBitsToFloat() (16. IntBitsToFloat())
This method is used to get the float floating-point value with the same bit pattern. The argument is considered to be a representation of a floating-point value according to the IEEE 754 floating-point "single format" bit layout. Syntax of this method is given below.
此方法用于获取具有相同位模式的浮点浮点值。 根据IEEE 754浮点“单一格式”位布局,该参数被认为是浮点值的表示。 该方法的语法如下。
句法: (Syntax:)
public static float IntBitsToFloat(long b)
例: (Example:)
Lets take an example to understand the intbitstofloat()
method that returns floating-point value.
让我们以一个示例来了解返回浮点值的intbitstofloat()
方法。
public class FloatDemo1
{
public static void main(String[] args)
{
int a = 72;
float y = Float.intBitsToFloat(a);
System.out.println("Float.intBitsToFloat(a) = " + y);
}
}
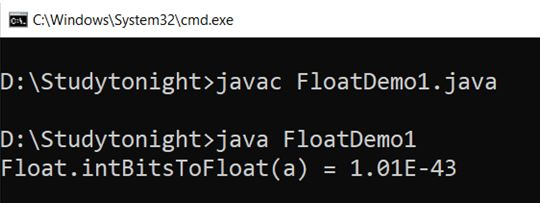
17. equals() (17. equals())
The equals()
method compares an object to the specified object. It returns true if objects are same; false otherwise. Syntax of this method is given below.
equals()
方法将一个对象与指定对象进行比较。 如果对象相同,则返回true;否则,返回true。 否则为假。 该方法的语法如下。
句法: (Syntax:)
public boolean equals(Object obj)
例: (Example:)
We are comparing two float objects using the equals method that returns true if both the objects are true.
我们正在使用equals方法比较两个float对象,如果两个对象都为true,则返回true。
public class FloatDemo1
{
public static void main(String[] args)
{
float a = 25.34F;
String b = "26";
Float obj = new Float(a);
Float obj1 = new Float(b);
boolean x = obj.equals(obj1);
System.out.println("obj.equals(obj1) = " + x);
}
}
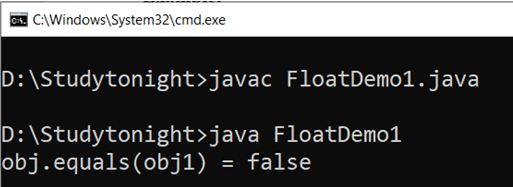
18. compareTo() (18. compareTo())
This method is used to compare two float objects numerically. It returns 0, if the both float objects are equal. It returns less the 0, if one float object is less than argument object. It returns greater than 0, if one float object is numerically greater than the argument float object. Syntax of this method is given below.
此方法用于数值比较两个浮点对象。 如果两个float对象相等,则返回0。 如果一个浮点对象小于参数对象,则返回的值小于0。 如果一个float对象在数值上大于参数float对象,则返回大于0。 该方法的语法如下。
句法: (Syntax:)
public intcompareTo(Float b)
例: (Example:)
In this example, we are comparing two float objects using compareTo()
method that compares two float objects numerically and returns a numeric value.
在此示例中,我们使用compareTo()
方法比较两个浮点对象,该方法对两个浮点对象进行数字比较并返回一个数值。
public class FloatDemo1
{
public static void main(String[] args)
{
float a = 25.34F;
String b = "26";
Float obj = new Float(a);
Float obj1 = new Float(b);
int x = obj.compareTo(obj1);
System.out.println("obj.compareTo(obj1) = " + x);
}
}
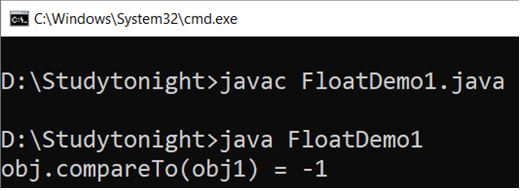
19. compare() (19. compare())
It is used to compare two float values numerically. The value returned is identical to what would be returned by. Syntax of this method is given below.
用于数值比较两个浮点值。 返回的值与将返回的值相同。 该方法的语法如下。
句法: (Syntax:)
public static int compare(float x,float y)
例: (Example:)
We can use compare method to compare two float values. It returns 0 if both are equal else returns either negative or positive numerical value.
我们可以使用compare方法比较两个float值。 如果两者相等,则返回0,否则返回正数或负数。
public class FloatDemo1
{
public static void main(String[] args)
{
float a = 25.34F;
String b = "26";
Float obj = new Float(a);
Float obj1 = new Float(b);
int x = Float.compare(obj, obj1);
System.out.println("compare(obj, obj1) = " + x);
}
}
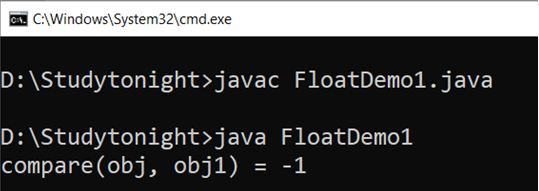
float java