java包
Package is a collection of related classes. Java uses package to group related classes, interfaces and sub-packages.
包是相关类的集合。 Java使用包将相关的类,接口和子包分组。
We can assume package as a folder or a directory that is used to store similar files.
我们可以将package假定为用于存储类似文件的文件夹或目录。
In Java, packages are used to avoid name conflicts and to control access of class, interface and enumeration etc. Using package it becomes easier to locate the related classes and it also provides a good structure for projects with hundreds of classes and other files.
在Java中,包用于避免名称冲突并控制类,接口和枚举等的访问。使用包可以更轻松地找到相关的类,并且还为具有数百个类和其他文件的项目提供了良好的结构。
Lets understand it by a simple example, Suppose, we have some math related classes and interfaces then to collect them into a simple place, we have to create a package.
让我们通过一个简单的示例理解它,假设我们有一些与数学相关的类和接口,然后将它们收集到一个简单的地方,我们必须创建一个包。
包装类型 (Types Of Package )
Package can be built-in and user-defined, Java provides rich set of built-in packages in form of API that stores related classes and sub-packages.
包可以是内置的和用户定义的,Java以API的形式提供了丰富的内置包集,用于存储相关的类和子包。
Built-in Package: math, util, lang, i/o etc are the example of built-in packages.
内置软件包: math,util,lang,I / O等是内置软件包的示例。
User-defined-package: Java package created by user to categorize their project's classes and interface are known as user-defined packages.
用户定义的软件包:用户创建的Java软件包,用于对其项目的类和接口进行分类,称为用户定义的软件包。
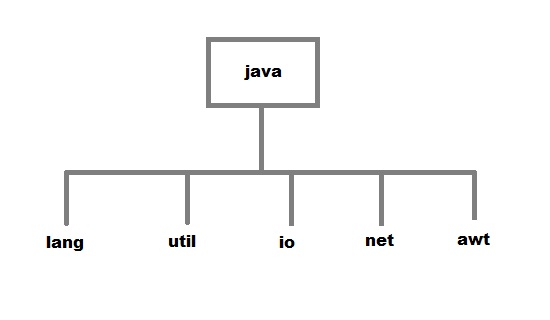
如何创建包装 (How to Create a Package)
Creating a package in java is quite easy, simply include a package command followed by name of the package as the first statement in java source file.
在Java中创建一个包非常容易,只需在Java源文件中添加一个package命令,后跟该包的名称即可。
package mypack;
public class employee
{
String empId;
String name;
}
The above statement will create a package woth name mypack in the project directory.
上面的语句将在项目目录中创建一个名为mypack的软件包。
Java uses file system directories to store packages. For example the .java
file for any class you define to be part of mypack package must be stored in a directory called mypack.
Java使用文件系统目录来存储软件包。 例如,您定义为mypack软件包一部分的任何类的.java
文件都必须存储在名为mypack的目录中 。
有关包装的其他要点: (Additional points about package:)
Package statement must be first statement in the program even before the import statement.
Package语句必须是程序中的第一个语句,甚至在import语句之前。
A package is always defined as a separate folder having the same name as the package name.
包始终被定义为一个单独的文件夹,其名称与包名称相同。
Store all the classes in that package folder.
将所有类存储在该包文件夹中。
All classes of the package which we wish to access outside the package must be declared public.
我们希望在包外部访问的所有包类必须声明为public。
All classes within the package must have the package statement as its first line.
包中的所有类都必须将package语句作为第一行。
All classes of the package must be compiled before use.
包的所有类都必须在使用前进行编译。
Java包示例 (Example of Java packages)
Now lets understand package creation by an example, here we created a leanjava package that stores the FirstProgram class file.
现在让我们通过一个例子来了解包的创建,在这里我们创建了一个leanjava包来存储FirstProgram类文件。
//save as FirstProgram.java
package learnjava;
public class FirstProgram{
public static void main(String args[]) {
System.out.println("Welcome to package example");
}
}
如何在包内编译Java程序? (How to compile Java programs inside packages?)
This is just like compiling a normal java program. If you are not using any IDE, you need to follow the steps given below to successfully compile your packages:
这就像编译普通的Java程序一样。 如果不使用任何IDE,则需要按照以下步骤成功编译软件包:
javac -d . FirstProgram.java
The -d
switch specifies the destination where to put the generated class file. You can use any directory name like d:/abc (in case of windows) etc. If you want to keep the package within the same directory, you can use .
(dot).
-d
开关指定将生成的类文件放入的位置。 您可以使用任何目录名称,例如d:/ abc (对于Windows)等。如果要将软件包保留在同一目录中,可以使用.
(点)。
如何运行Java打包程序? (How to run Java package program?)
To run the compiled class that we compiled using above command, we need to specify package name too. Use the below command to run the class file.
要运行使用上述命令编译的已编译类,我们还需要指定包名称。 使用以下命令运行类文件。
java learnjava.FirstProgram
Welcome to package example
欢迎来到包装示例
After running the program, we will get “Welcome to package example” message to the console. You can tally that with print statement used in the program.
运行该程序后,我们将向控制台显示“ Welcome to Package example”消息。 您可以使用程序中使用的print语句来计算。
如何导入Java包 (How to import Java Package)
To import java package into a class, we need to use java import keyword which is used to access package and its classes into the java program.
要将java包导入到一个类中,我们需要使用java import关键字,该关键字用于将包及其类访问到java程序中。
Use import to access built-in and user-defined packages into your java source file so that your class can refer to a class that is in another package by directly using its name.
使用import可以将内置和用户定义的包访问到Java源文件中,以便您的类可以通过直接使用其名称来引用另一个包中的类。
There are 3 different ways to refer to any class that is present in a different package:
有3种不同的方式可以引用存在于不同程序包中的任何类:
without import the package
不导入包
import package with specified class
导入指定类的包
import package with all classes
导入所有类的包
Lets understand each one with the help of example.
让我们借助示例来了解每个人。
访问没有导入关键字的软件包 (Accessing package without import keyword)
If you use fully qualified name to import any class into your program, then only that particular class of the package will be accessible in your program, other classes in the same package will not be accessible. For this approach, there is no need to use the import
statement. But you will have to use the fully qualified name every time you are accessing the class or the interface. This is generally used when two packages have classes with same names. For example: java.util
and java.sql
packages contain Date class
.
如果您使用完全限定的名称将任何类导入程序,则只能在程序中访问该包的特定类,而不能访问同一包中的其他类。 对于这种方法,不需要使用import
语句。 但是,每次访问类或接口时,都必须使用标准名称。 通常在两个包具有相同名称的类时使用。 例如: java.util
和java.sql
包包含Date class
。
例 (Example )
In this example, we are creating a class A in package pack and in another class B, we are accessing it while creating object of class A.
在此示例中,我们在包包中创建一个类A,而在另一个类B中,则在创建类A的对象时对其进行访问。
//save by A.java
package pack;
public class A {
public void msg() {
System.out.println("Hello");
}
}
//save by B.java
package mypack;
class B {
public static void main(String args[]) {
pack.A obj = new pack.A(); //using fully qualified name
obj.msg();
}
}
Hello
你好
导入特定类别 (Import the Specific Class)
Package can have many classes but sometimes we want to access only specific class in our program in that case, Java allows us to specify class name along with package name. If we use import packagename.classname
statement then only the class with name classname in the package will be available for use.
包可以有许多类,但是在这种情况下,有时我们只想访问程序中的特定类,Java允许我们指定类名和包名。 如果我们使用import packagename.classname
语句,则只有包中名称为classname的类可以使用。
例: (Example:)
In this example, we created a class Demo stored into pack package and in another class Test, we are accessing Demo class by importing package name with class name.
在此示例中,我们创建了一个存储在pack包中的Demo类,在另一个Test类中,我们通过导入带有类名的包名来访问Demo类。
//save by Demo.java
package pack;
public class Demo {
public void msg() {
System.out.println("Hello");
}
}
//save by Test.java
package mypack;
import pack.Demo;
class Test {
public static void main(String args[]) {
Demo obj = new Demo();
obj.msg();
}
}
Hello
你好
导入包的所有类 (Import all classes of the package)
If we use packagename.* statement, then all the classes and interfaces of this package will be accessible but the classes and interface inside the subpackages will not be available for use.
如果我们使用packagename。*语句 ,那么将可以访问此包的所有类和接口,但是子包中的类和接口将不可用。
The import
keyword is used to make the classes of another package accessible to the current package.
import
关键字用于使当前程序包可以访问另一个程序包的类。
范例: (Example :)
In this example, we created a class First in learnjava package that access it in another class Second by using import keyword.
在本示例中,我们在learnjava包中创建了一个类First,该类通过使用import关键字在另一个类Second中对其进行访问。
//save by First.java
package learnjava;
public class First{
public void msg() {
System.out.println("Hello");
}
}
//save by Second.java
package Java;
import learnjava.*;
class Second {
public static void main(String args[]) {
First obj = new First();
obj.msg();
}
}
Hello
你好
java包