ruby 三目运算符
In this lesson, we are going to look at the different operators
that ruby contains and how to use them in the expressions
.
在本课程中,我们将研究ruby包含的不同operators
以及如何在expressions
使用它们。
Ruby:加法运算符 (Ruby: Addition Operator)
+
symbol is used. It is both binary and unary operator. As the name suggests a Binary operator
needs two operands/values on the either side of the operator to perform an operation and a unary operator needs just a single operand. Unary Plus serves no purpose, it is present just for the symmetry with unary minus.
+
符号。 它既是二进制运算符又是一元运算符。 顾名思义, Binary operator
在运算符的任一侧都需要两个操作数/值来执行运算,而一元运算符只需要一个运算数。 一元加号(Unary Plus)毫无用处,仅出于一元减号的对称性而存在。
For example : 1 + 2 //binary addition
value = +3 //unary plus
Ruby:减法运算符 (Ruby: Subtraction Operator)
-
symbol is used. You can use unary minus to reverse sign of a variable.
-
符号。 您可以使用一元减号来反转变量的符号。
For Example : 23.2 - 2.2 //binary subtraction
value = -3 //unary minus
Ruby:乘法运算符 (Ruby: Multiplication Operator)
*
symbol is used. Perfoms Multiplication on two numeric operands.
*
符号。 在两个数字操作数上执行Perfoms乘法。
For Example : 122 * 3.14
Ruby:除法运算符 (Ruby: Division Operator)
/
symbol is used. Returns result of devision of the first numeric operand by second operand.
/
符号被使用。 返回第二个操作数除以第一个数字操作数的结果。
For Example : 18 / 3
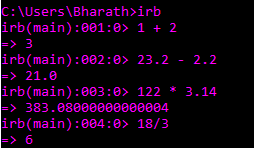
Ruby:模运算符 (Ruby: Modulo Operator)
Returns remainder after division.
返回除法后的余数。
For Example : 13 % 2 //returns 1
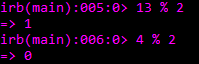
Ruby:指数运算符 (Ruby: Exponential Operator)
If you want to raise x
to the power of y
(i.e) x ^ y
.
如果要将x
提高到y
的幂(即x ^ y
。
It is done by x ** y

2 raised to the power 4 returns 16.
2提升到4的幂返回16。
Ruby:运算符优先级 (Ruby: Operator Precedence)
Operators
have some order of precedence
which determines the order in which an expression will be evaluated.
Operators
具有一些precedence
顺序,该precedence
确定了表达式的求值顺序。
Consider this example, 1 + 2 * 3
We might think that the 1 + 2 is performed and the result 3 will be multiplied by 3 and gives 9. But the multiplication, division and exponential operator have higher precedence than addition and subtraction operators. Therefore, 2 *3 is performed first and the result is added to 1 and gives 7 as an answer.
我们可能会认为执行了1 + 2并将结果3乘以3得到9 。 但是乘法 , 除法和指数运算符的优先级高于加法和减法运算符。 因此,首先执行2 * 3并将结果加到1并给出7作为答案。
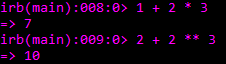
However, we can modify the order of precedence by putting a subexpression in parentheses. In the expression 1 + 2 * 3, if 1 + 2 need to be performed first, put that expression in parentheses.
但是,我们可以通过在括号中放置一个子表达式来修改优先顺序。 在表达式1 + 2 * 3中 ,如果首先需要执行1 + 2 ,则将该表达式放在括号中。
(1 + 2) * 3 this expression produces 9
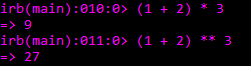
Ruby:关系运算符 (Ruby: Relational Operators)
Relational operators
are used for comparisons. They return Boolean values
. We compare two values whether they are equal, not equal, less than, greater than, less than or equal to and greater than or equal to.
Relational operators
用于比较。 它们返回Boolean values
。 我们比较两个值是否相等,不相等,小于,大于,小于或等于以及大于或等于。
==
sign is used. Used to check whether two numbers are equal or not.
==
符号。 用于检查两个数字是否相等。
1 == 1 returns true because 1 is equal to 1.
<
- Less than
<
-小于
>
- Greater than
>
-大于
<=
- Less than or equal to
<=
-小于或等于
>=
- Greater than or equal to
>=
-大于或等于
Less than operator checks whether a number is less than the another number, if yes it returns true else returns false. Less than or equal to operator checks whether a number is less than to another number and also checks whether a number is equal to another number, if any one of the condition is correct it returns true else returns false. Greater than and Greater than or equal to does the same and checks whether it is greater.
小于运算符检查一个数字是否小于另一个数字,如果是,则返回true,否则返回false 。 小于或等于运算符检查一个数字是否小于另一个数字,还检查一个数字是否等于另一个数字,如果任一条件正确,则返回true,否则返回false。 大于和大于或等于进行相同操作,并检查它是否更大 。
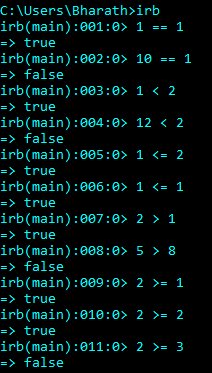
Another way to compare two values is using General comparison operator. It returns 0(zero), -1 or +1 depending on the operands. If both the values are equal it returns zero, if the first operand is less than the second operand it returns -1 and +1 if the first operand is greater than the second.
比较两个值的另一种方法是使用常规比较运算符 。 根据操作数返回0(零) ,- 1或+1 。 如果两个值相等,则返回零 ;如果第一个操作数小于第二个操作数,则返回-1 ;如果第一个操作数大于第二个操作数,则返回+1 。
The General Comparison operator is <=>(< = >).
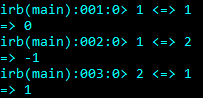
Relational operators
can be used with strings also.
Relational operators
也可以与字符串一起使用。
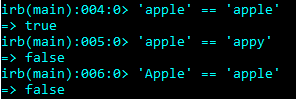
If it is same it returns true. You could see that it returns false for the operation 'Apple' == 'apple' This is because Ruby is case-sensitive and one of the word has Uppercase A
while the other word has lowercase a
. We can also use General comparison operator with strings.
如果相同,则返回true。 您可能会看到它对操作'Apple'=='apple'返回false,这是因为Ruby区分大小写,并且其中一个单词具有Uppercase A
lowercase a
Uppercase A
而另一个单词具有lowercase a
。 我们还可以对字符串使用General比较运算符 。
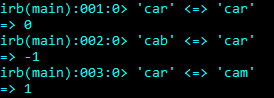
When comparing 'car' and 'car' it returned 0 since both are equal. When comparing 'cab' with 'car' it returned -1 because the 3rd letter of the word 'b' in 'cab' is less than 'r' in 'car'.
比较“ car”和“ car”时 ,由于两者相等,因此返回0 。 当将'cab'与'car'比较时,它返回-1,因为'cab'中单词'b'的第三个字母小于'car'中的 'r ' 。
Ruby:逻辑运算符 (Ruby: Logical Operators)
Logical operators
allow you to combine two or more relational expressions and returns Boolean
value.
Logical operators
允许您组合两个或多个关系表达式并返回Boolean
值。
AND operator:
AND运算子:
It returns true when all of the expressions are true and returns false if even one of the expression evaluates to false. You could use this operator using and
or &&
.
当所有表达式都为true时,它返回true;即使其中一个表达式的计算结果为false,它也返回false。 您可以通过and
或&&
使用此运算符。
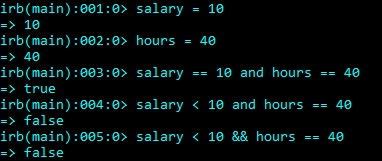
It returned true for the expression salary == 10 && hours == 40 because we've initialized the value of the variables salary and hours as 10 and 40. It will return false for all other cases.
对于表达式salary == 10 && hours == 40,它返回true,因为我们已经将变量salary和hours的值初始化为10和40。对于所有其他情况,它将返回false。
OR operator:
或运算符:
OR
operator returns true when any one condition/expression is true and returns false only when all of them are false. You can use this operator using or
(or) ||
当任一条件/表达式为true时, OR
运算符将返回true;仅当所有条件/表达式均为false时,才返回false。 您可以使用or
(或) ||
使用此运算符

You can see that even when only one expression is true OR operator returned true.
您可以看到,即使只有一个表达式为true 或OR运算符也返回true。
NOT operator:
非运算符:
NOT
operator negates a relational expression. You can use not
(or) !
for this operator.
NOT
运算符会否定关系表达式。 您可以not
使用(或) !
对于这个操作员。
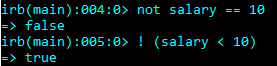
In the first expression it returned false because the expression salary == 10 returns true and the not operator negates true and returns false. Likewise, the expression salary < 10 returns false and not operator negates and returns true.
在第一个表达式中它返回false,因为表达式salary == 10返回true,而not运算符取反true并返回false。 同样,表达式salary <10返回false,而不是运算符取反,然后返回true。
ruby 三目运算符