java中访问修饰符
Access modifiers are keywords in Java that are used to set accessibility. An access modifier restricts the access of a class, constructor, data member and method in another class.
访问修饰符是Java中用于设置可访问性的关键字。 访问修饰符限制对另一个类中的类,构造函数,数据成员和方法的访问。
Java language has four access modifier to control access level for classes and its members.
Java语言具有四个访问修饰符,用于控制类及其成员的访问级别。
Default: Default has scope only inside the same package
默认值:默认值仅在同一包内具有作用域
Public: Public has scope that is visible everywhere
公众:公众的视野无处不在
Protected: Protected has scope within the package and all sub classes
受保护的:受保护的在包和所有子类中具有作用域
Private: Private has scope only within the classes
私有:私有仅在课程内具有作用域
Java also supports many non-access modifiers, such as static, abstract, synchronized, native, volatile, transient etc. We will cover these in our other tutorial.
Java还支持许多非访问修饰符,例如静态,抽象,同步,本机,易失,瞬态等。我们将在其他教程中介绍这些。
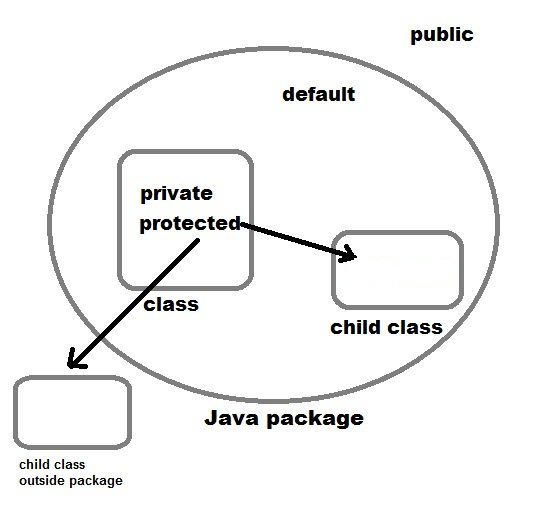
默认访问修饰符 (Default Access Modifier)
If we don’t specify any access modifier then it is treated as default modifier. It is used to set accessibility within the package. It means we can not access its method or class fro outside the package. It is also known as package accessibility modifier.
如果我们未指定任何访问修饰符,则将其视为默认修饰符。 它用于设置包内的可访问性。 这意味着我们无法在包外部访问其方法或类。 也称为包可访问性修饰符。
例: (Example:)
In this example, we created a Demo class inside the package1 and another class Test by which we are accessing show() method of Demo class. We did not mentioned access modifier for the show() method that’s why it is not accessible and reports an error during compile time.
在此示例中,我们在package1和另一个Test 类中创建了一个Demo类 ,通过该类我们可以访问Demo类的show()方法。 我们没有提到show()方法的访问修饰符,这就是为什么它不可访问并在编译时报告错误的原因。
Demo.java
演示程序
package package1;
public class Demo {
int a = 10;
// default access modifier
void show() {
System.out.println(a);
}
}
Test.java
Test.java
import package1.Demo;
public class Test {
public static void main(String[] args) {
Demo demo = new Demo();
demo.show(); // compile error
}
}
The method show() from the type Demo is not visible
演示类型中的show()方法不可见
公共访问修饰符 (Public Access Modifier)
public access modifier is used to set public accessibility to a variable, method or a class. Any variable or method which is declared as public can be accessible from anywhere in the application.
public access修饰符用于将公共可访问性设置为变量,方法或类。 任何声明为公共的变量或方法都可以从应用程序中的任何位置访问。
例: (Example:)
Here, we have two class Demo and Test located in two different package. Now we want to access show method of Demo class from Test class. The method has public accessibility so it works fine. See the below example.
在这里,我们有两个类Demo和Test放在两个不同的包中。 现在我们要从Test类访问Demo类的show方法。 该方法具有公共可访问性,因此效果很好。 请参见以下示例。
Demo.java
演示程序
package package1;
public class Demo {
int a = 10;
// public access modifier
public void show() {
System.out.println(a);
}
}
Test.java
Test.java
package package2;
import package1.Demo;
public class Test {
public static void main(String[] args) {
Demo demo = new Demo();
demo.show();
}
}
10
10
受保护的访问修饰符 (Protected Access Modifier)
Protected modifier protects the variable, method from accessible from outside the class. It is accessible within class, and in the child class (inheritance) whether child is located in the same package or some other package.
受保护的修饰符可防止从类外部访问变量方法。 它可以在类内以及在子类(继承)中访问,无论child 位于 同一包中还是其他某个包中 。
例: (Example:)
In this example, Test class is extended by Demo and called a protected method show() which is accessible now due to inheritance.
在此示例中,Test类由Demo扩展,并称为受保护的方法show(),由于继承现在可以访问该方法。
Demo.java
演示程序
package package1;
public class Demo {
int a = 10;
// public access modifier
protected void show() {
System.out.println(a);
}
}
Test.java
Test.java
package package2;
import package1.Demo;
public class Test extends Demo{
public static void main(String[] args) {
Test test = new Test();
test.show();
}
}
10
10
专用访问修饰符 (Private Access Modifier)
Private modifier is most restricted modifier which allows accessibility within same class only. We can set this modifier to any variable, method or even constructor as well.
私有修饰符是最受限制的修饰符,仅允许在同一类中进行访问。 我们可以将此修饰符设置为任何变量,方法甚至构造函数。
例: (Example:)
In this example, we set private modifier to show() method and try to access that method from outside the class. Java does not allow to access it from outside the class.
在此示例中,我们将private修饰符设置为show()方法,并尝试从类外部访问该方法。 Java不允许从类外部访问它。
Demo.java
演示程序
class Demo {
int a = 10;
private void show() {
System.out.println(a);
}
}
Test.java
Test.java
public class Test {
public static void main(String[] args) {
Demo demo = new Demo();
demo.show(); // compile error
}
}
The method show() from the type Demo is not visible
演示类型中的show()方法不可见
非访问修饰符 (Non-access Modifier)
Along with access modifiers, Java provides non-access modifiers as well. These modifier are used to set special properties to the variable or method.
Java与访问修饰符一起,还提供了非访问修饰符。 这些修饰符用于为变量或方法设置特殊属性 。
Non-access modifiers do not change the accessibility of variable or method, but they provide special properties to them. Java provides following non-access modifiers.
非访问修饰符不会更改变量或方法的可访问性,但是它们为它们提供了特殊的属性。 Java提供了以下非访问修饰符。
Final
最后
Static
静态的
Transient
短暂的
Synchronized
已同步
Volatile
易挥发的
最终修饰符 (Final Modifier)
Final modifier can be used with variable, method and class. if variable is declared final then we cannot change its value. If method is declared final then it can not be overridden and if a class is declared final then we can not inherit it.
Final修饰符可以与变量,方法和类一起使用。 如果将变量声明为final,则无法更改其值。 如果将方法声明为final , 则不能覆盖该方法 ;如果将一个类声明为final,则不能继承它 。
静态修饰符 (Static modifier )
static modifier is used to make field static. We can use it to declare static variable, method, class etc. static can be use to declare class level variable. If a method is declared static then we don’t need to have object to access that. We can use static to create nested class.
static修饰符用于使字段静态。 我们可以使用它来声明静态变量,方法,类等。static可以用来声明类级别的变量。 如果一个方法被声明为静态的,那么我们就不需要对象来访问它。 我们可以使用static创建嵌套类。
瞬态修饰符 (Transient modifier)
When an instance variable is declared as transient, then its value doesn't persist when an object is serialized
当实例变量被声明为瞬时变量时,则对象被序列化时其值不会持久
同步修饰符 (Synchronized modifier)
When a method is synchronized it can be accessed by only one thread at a time. We will discuss it in detail in Thread.
方法同步后,一次只能由一个线程访问。 我们将在Thread中对其进行详细讨论。
挥发性改性剂 (Volatile modifier)
Volatile modifier tells to the compiler that the volatile variable can be changed unexpectedly by other parts of a program. Volatile variables are used in case of multi-threading program. volatile keyword cannot be used with a method or a class. It can be only used with a variable.
易失性修饰符告诉编译器,易失性变量可能会被程序的其他部分意外更改。 在多线程程序中使用易失变量。 volatile关键字不能与方法或类一起使用。 它只能与变量一起使用。
java中访问修饰符