java继承中的is-a
Inheritance is one of the key features of Object Oriented Programming. Inheritance provided mechanism that allowed a class to inherit property of another class. When a Class extends another class it inherits all non-private members including fields and methods. Inheritance in Java can be best understood in terms of Parent and Child relationship, also known as Super class(Parent) and Sub class(child) in Java language.
继承是面向对象编程的主要功能之一。 继承提供了一种机制,该机制允许一个类继承另一个类的属性 。 当一个类扩展另一个类时,它将继承所有非私有成员,包括字段和方法。 Java的继承可以通过父子关系(在Java语言中也称为父类 (父类 )和子类 ( 子类 ))得到最好的理解。
Inheritance defines is-a relationship between a Super class and its Sub class. extends
and implements
keywords are used to describe inheritance in Java.
继承定义is-超级类及其子类之间的关系。 extends
和implements
关键字用于描述Java中的继承。
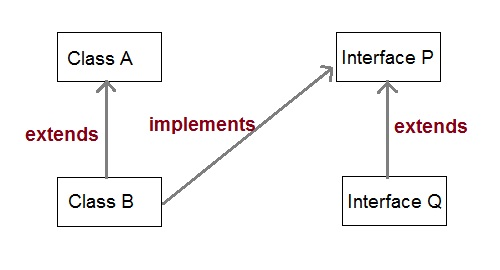
Let us see how extends keyword is used to achieve Inheritance. It shows super class and sub-class relationship.
让我们看看如何使用extends关键字实现继承。 它显示了超类和子类的关系。
class Vehicle
{
......
}
class Car extends Vehicle
{
....... //extends the property of vehicle class
}
Now based on above example. In OOPs term we can say that,
现在基于上面的示例。 用面向对象的术语,我们可以这样说:
Vehicle is super class of Car.
车辆是超一流的汽车 。
Car is sub class of Vehicle.
Car是Vehicle的子类。
Car IS-A Vehicle.
汽车IS-A车辆。
继承目的 (Purpose of Inheritance)
It promotes the code reusabilty i.e the same methods and variables which are defined in a parent/super/base class can be used in the child/sub/derived class.
它促进了代码的可重用性,即可以在子/子/派生类中使用在父/上/基类中定义的相同方法和变量。
It promotes polymorphism by allowing method overriding.
它通过允许方法重写来促进多态性。
继承的缺点 (Disadvantages of Inheritance)
Main disadvantage of using inheritance is that the two classes (parent and child class) gets tightly coupled.
使用继承的主要缺点是两个类(父类和子类) 紧密耦合 。
This means that if we change code of parent class, it will affect to all the child classes which is inheriting/deriving the parent class, and hence, it cannot be independent of each other.
这意味着,如果我们更改父类的代码,它将影响到继承/派生该父类的所有子类,因此, 它们不能彼此独立 。
继承的简单示例 (Simple example of Inheritance)
Before moving ahead let's take a quick example and try to understand the concept of Inheritance better,
在继续之前,我们先举一个简单的例子,尝试更好地理解继承的概念,
class Parent
{
public void p1()
{
System.out.println("Parent method");
}
}
public class Child extends Parent {
public void c1()
{
System.out.println("Child method");
}
public static void main(String[] args)
{
Child cobj = new Child();
cobj.c1(); //method of Child class
cobj.p1(); //method of Parent class
}
}
Child method Parent method
子方法父方法
In the code above we have a class Parent which has a method p1()
. We then create a new class Child which inherits the class Parent using the extends
keyword and defines its own method c1()
. Now by virtue of inheritance the class Child can also access the public
method p1()
of the class Parent.
在上面的代码中,我们有一个Parent类,它具有方法p1()
。 然后,我们创建一个新的Child类,该类使用extends
关键字继承了Parent类,并定义了自己的方法c1()
。 现在,通过继承,类Child也可以访问类Parent的public
方法p1()
。
继承超类的变量 (Inheriting variables of super class)
All the members of super class implicitly inherits to the child class. Member consists of instance variable and methods of the class.
超类的所有成员都隐式继承到子类。 成员由实例变量和类的方法组成。
例 (Example)
In this example the sub-class will be accessing the variable defined in the super class.
在此示例中,子类将访问超类中定义的变量。
class Vehicle
{
// variable defined
String vehicleType;
}
public class Car extends Vehicle {
String modelType;
public void showDetail()
{
vehicleType = "Car"; //accessing Vehicle class member variable
modelType = "Sports";
System.out.println(modelType + " " + vehicleType);
}
public static void main(String[] args)
{
Car car = new Car();
car.showDetail();
}
}
Sports Car
跑车
继承类型 (Types of Inheritance)
Java mainly supports only three types of inheritance that are listed below.
Java主要仅支持下面列出的三种继承类型。
Single Inheritance
单继承
Multilevel Inheritance
多级继承
Heirarchical Inheritance
继承继承
NOTE: Multiple inheritance is not supported in java
注意: Java不支持多重继承
We can get a quick view of type of inheritance from the below image.
我们可以从下图快速了解继承的类型。
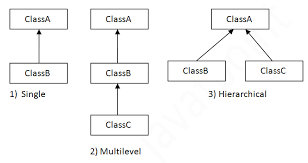
单继承 (Single Inheritance)
When a class extends to another class then it forms single inheritance. In the below example, we have two classes in which class A extends to class B that forms single inheritance.
当一个类扩展到另一个类时,它将形成单一继承。 在下面的示例中,我们有两个类,其中类A扩展到形成单个继承的类B。
class A{
int a = 10;
void show() {
System.out.println("a = "+a);
}
}
public class B extends A{
public static void main(String[] args) {
B b = new B();
b.show();
}
}
a=10
a = 10
Here, we can notice that show() method is declared in class A, but using child class Demo object, we can call it. That shows the inheritance between these two classes.
在这里,我们可以注意到show()方法是在类A中声明的,但是使用子类Demo对象,我们可以调用它。 这表明了这两个类之间的继承。
多级继承 (Multilevel Inheritance )
When a class extends to another class that also extends some other class forms a multilevel inheritance. For example a class C extends to class B that also extends to class A and all the data members an methods of class A and B are now accessible in class C.
当一个类扩展到另一个类时,该类又扩展了一些其他类,形成了多级继承。 例如,类C扩展到类B,该类也扩展到类A,并且现在可以在类C中访问类A和B的所有数据成员。
例: (Example:)
class A{
int a = 10;
void show() {
System.out.println("a = "+a);
}
}
class B extends A{
int b = 10;
void showB() {
System.out.println("b = "+b);
}
}
public class C extends B{
public static void main(String[] args) {
C c = new C();
c.show();
c.showB();
}
}
a=10 b=10
a = 10 b = 10
层次继承 (Hierarchical Inheritance)
When a class is extended by two or more classes, it forms hierarchical inheritance. For example, class B extends to class A and class C also extends to class A in that case both B and C share properties of class A.
当一个类被两个或多个类扩展时,它将形成层次继承。 例如,在这种情况下,B和C都共享A类的属性,因此B类扩展到A类,C类也扩展到A类。
class A{
int a = 10;
void show() {
System.out.println("a = "+a);
}
}
class B extends A{
int b = 10;
void showB() {
System.out.println("b = "+b);
}
}
public class C extends A{
public static void main(String[] args) {
C c = new C();
c.show();
B b = new B();
b.show();
}
}
a = 10 a = 10
a = 10 a = 10
为什么Java不支持多重继承? (Why multiple inheritance is not supported in Java?)
To remove ambiguity.
消除歧义。
To provide more maintainable and clear design.
提供更易于维护和清晰的设计。
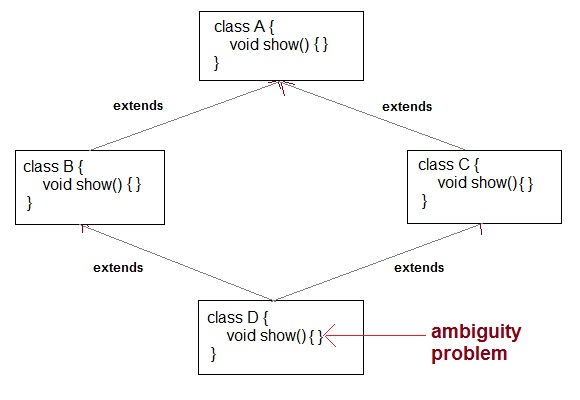
super
关键字 (super
keyword)
In Java, super
keyword is used to refer to immediate parent class of a child class. In other words super keyword is used by a subclass whenever it need to refer to its immediate super class.
在Java中, super
关键字用于引用子类的直接父类。 换句话说,每当子类需要引用其直接父类时,就会使用super关键字。
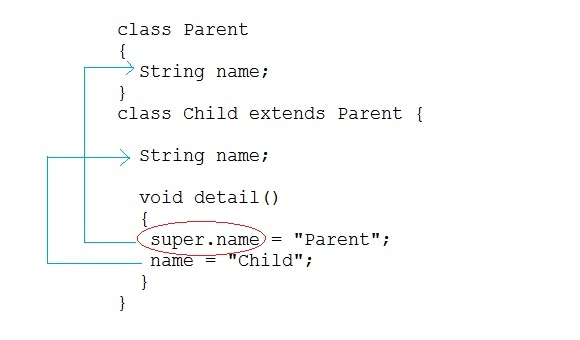
子类使用super
关键字引用父类属性的示例 (Example of Child class referring Parent class property using super
keyword)
In this examle we will only focus on accessing the parent class property or variables.
在此示例中,我们将仅着重于访问父类的属性或变量。
class Parent
{
String name;
}
public class Child extends Parent {
String name;
public void details()
{
super.name = "Parent"; //refers to parent class member
name = "Child";
System.out.println(super.name+" and "+name);
}
public static void main(String[] args)
{
Child cobj = new Child();
cobj.details();
}
}
Parent and Child
父母与子女
子类使用super
关键字引用父类方法的示例 (Example of Child class refering Parent class methods using super
keyword)
In this examle we will only focus on accessing the parent class methods.
在此示例中,我们将仅着重于访问父类方法。
classParent
{
String name;
public void details()
{
name = "Parent";
System.out.println(name);
}
}
public class Child extends Parent {
String name;
public void details()
{
super.details(); //calling Parent class details() method
name = "Child";
System.out.println(name);
}
public static void main(String[] args)
{
Child cobj = new Child();
cobj.details();
}
}
Parent Child
亲子
子类使用super
关键字调用父类constructor
示例 (Example of Child class calling Parent class constructor
using super
keyword)
In this examle we will focus on accessing the parent class constructor.
在本示例中,我们将重点介绍访问父类的构造函数。
classParent
{
String name;
public Parent(String n)
{
name = n;
}
}
public class Child extends Parent {
String name;
public Child(String n1, String n2)
{
super(n1); //passing argument to parent class constructor
this.name = n2;
}
public void details()
{
System.out.println(super.name+" and "+name);
}
public static void main(String[] args)
{
Child cobj = new Child("Parent","Child");
cobj.details();
}
}
Parent and Child
父母与子女
Note: When calling the parent class constructor from the child class using super keyword, super keyword should always be the first line in the method/constructor of the child class.
注意:当使用super关键字从子类中调用父类的构造函数时,super关键字应始终是子类的方法/构造函数的第一行。
问:可以在构造函数中同时使用this()
和super()
吗? (Q. Can you use both this()
and super()
in a Constructor?)
NO, because both super() and this() must be first statement inside a constructor. Hence we cannot use them together.
否,因为super()和this()都必须是构造函数中的第一条语句。 因此,我们不能一起使用它们。
翻译自: https://www.studytonight.com/java/inheritance-in-java.php
java继承中的is-a