ruby 怎么抛异常
Exception Handling in Ruby involves how we handle the errors that come up in our program. By errors, we're not talking about syntax errors such as misspelling the Ruby keywords. We're referring to errors from which a program cannot recover, things that programmers won't anticipates but it can happen. Good programmers anticipate them and take actions to handle them.
Ruby中的异常处理涉及我们如何处理程序中出现的错误。 通过错误,我们不是在谈论语法错误,例如拼写Ruby关键字 。 我们指的是程序无法恢复的错误,这些错误是程序员无法预料的,但是可能会发生。 好的程序员会期望他们,并采取行动来处理它们。
Exception occurs due to things the system can't handle when the program is running.
由于程序运行时系统无法处理的事情而发生异常 。
Ruby:异常示例 (Ruby: Examples of Exception)
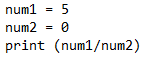
In the above program, we try to divide a number by zero. When running the program, it gives the following result :
在上面的程序中,我们尝试将数字除以零。 运行该程序时,将得到以下结果:

A ZeroDivisionError
occurs because we try to divide a number by zero and that operation is not defined in Ruby.
发生ZeroDivisionError
是因为我们尝试将数字除以零,并且该操作未在Ruby中定义。
Let's see another example:
让我们看另一个例子:

Following is the result of the above program:
以下是上述程序的结果:

When we execute this program we get an error (No such file or directory) because the program tries to open the file which is not present in the disk. Again when we were typing the program the compiler has no way to know about the file whether such file exists or not.
当我们执行该程序时,由于程序试图打开磁盘中不存在的文件,因此会出现错误(没有此类文件或目录) 。 同样,当我们键入程序时,编译器无法知道该文件是否存在。
In these two examples, the program terminates completely which is not what we expected. So, we have to handle the exceptions
and to prevent the program from crashing and exiting to the Operating system.
在这两个示例中,程序完全终止,这不是我们期望的。 因此,我们必须处理exceptions
并防止程序崩溃并退出到操作系统 。
Ruby:处理异常 (Ruby: Handling Exceptions)
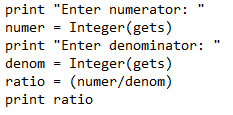
When we enter 0 for denominator we get the divide by zero.
当我们为分母输入0时 ,除以零。
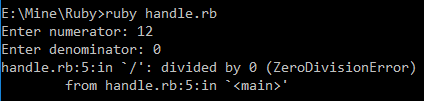
We have to isolate the code that causes the exception
. So that, if an exception is raised we can transfer the control to another part of the program that handles that exception.
我们必须隔离导致exception
的代码。 这样,如果引发异常,我们可以将控件转移到处理该异常的程序的另一部分。
Here is how we do it:
这是我们的方法:
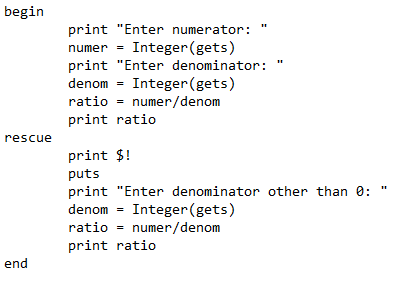
The code that causes exception are written in begin
block and the rescue
block contains code that handles the exception. When the code in the begin
block raises an exception control is automatically transferred to the rescue
block and then the code inside rescue block executes and program terminates.
导致异常的代码写在begin
块中,而rescue
块包含处理异常的代码。 当begin
块中的代码引发异常时,控制权将自动转移到rescue
块,然后执行救援块中的代码并终止程序。
The exception is stored in the global variable !(exclamation mark)
. The statement print $!
prints the exception.
异常存储在全局变量!(exclamation mark)
。 语句print $!
打印异常。
The output of the above program is :
上面程序的输出是:
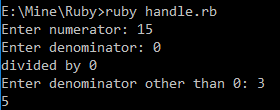
When the value 0 is entered for denominator the exception
is raised and the control is transferred to the rescue
block and the statements are executed. It prints the exception and prompts to enter another value for denominator and then performs the operation and the program terminates. Here the program does not crash and does not halts completely. But this is not a perfect solution to this problem because when the user again enters the value 0 it results in exception
again which is not handled. We must use loop to handle the exception
in a perfect manner.
为分母输入值0时,会引发exception
,并将控制权转移到rescue
块并执行语句。 它打印异常并提示输入另一个分母值,然后执行该操作,程序终止。 此处程序不会崩溃 ,也不会完全停止 。 但这不是解决此问题的理想方法,因为当用户再次输入值0时 ,会再次导致无法处理的exception
。 我们必须使用循环来完美地处理exception
。
Ruby:处理多个异常 (Ruby: Handling multiple Exceptions)
When a block of code raises multiple exception
we must handle it in a different way. For example, consider the below code
当代码块引发multiple exception
我们必须以不同的方式处理它。 例如,考虑以下代码
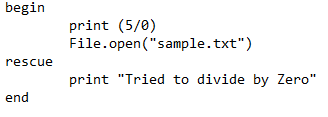
The begin
block contains statements that raises "Divide by Zero" exception as well as "File not found exception". We have handled the exception only for "Divide by zero" exception.
begin
块包含引发“除以零”异常以及“找不到文件异常”的语句。 我们仅针对“除以零”异常处理了该异常。
Now, consider this code :
现在,考虑以下代码:
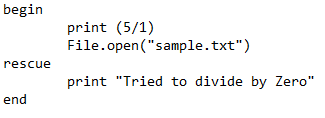
Here the "Divide by zero" exception is handled and there is no use for it. So, we have to be particular in handling the exception in case of multiple exceptions.
此处处理了“被零除”异常,没有任何用处。 因此,在有多个异常的情况下,我们必须特别处理异常 。
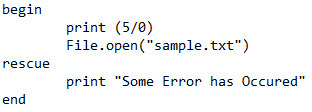
Here the exception is handled for all the types. We can handle particular exception by using the name of the exception.
在这里,所有类型的异常都得到处理。 我们可以通过使用异常的名称来处理特定的异常。
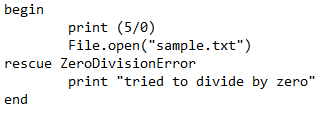
We have handled ZeroDivisonError
exception by specifying its name.
我们已经通过指定其名称来处理ZeroDivisonError
异常。

We can also have multiple rescue blocks to handle each exception.
我们还可以有多个救援块来处理每个异常 。
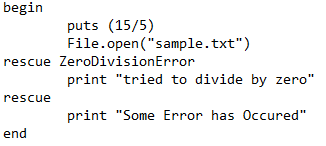
We have two rescue
blocks one to handle ZeroDivisionError
and another block for unknown exception
. There is no particular exception name for file not found exception like ZeroDivisionError
. But we can use SystemCallError
exception to handle general exception situation.
我们有两个rescue
块,一个用于处理ZeroDivisionError
,另一个用于未知exception
。 对于找不到文件的异常(例如ZeroDivisionError
),没有特定的异常名称。 但是我们可以使用SystemCallError
异常来处理一般的异常情况。
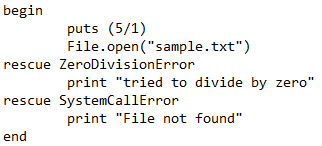
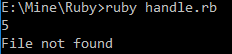
When the general exception situation happens the control transfers to the second rescue
block to handle the exception
. We can also store the exception message in the variable. Like this,
当发生一般异常情况时,控制权转移到第二个rescue
块以处理exception
。 我们还可以将异常消息存储在变量中。 像这样,
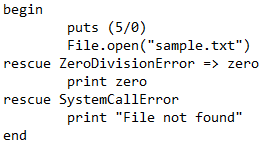
The output is :
输出为:

The actual error message for the exception ZeroDivisionError
is stored in the variable zero and we print the variable.
ZeroDivisionError
异常的实际错误消息存储在变量零中 ,我们打印该变量。
Ruby:引发异常 (Ruby: Raising exceptions)
We can create our own exceptions in Ruby to handle errors which Ruby does not recognizes. User defined exceptions are created using raise
keyword.
我们可以在Ruby中创建自己的异常来处理Ruby无法识别的错误 。 用户定义的异常是使用raise
关键字创建的。
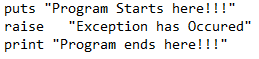
The output of the program is :
该程序的输出为:

Here, the first statement is executed and we have raised our exception. But the last statement is not printed because when the exception is raised, the program terminates completely.
在这里,第一条语句已执行,并且我们提出了exception 。 但是不会打印最后一条语句,因为当引发异常时,程序将完全终止。
翻译自: https://www.studytonight.com/ruby/exception-handling-in-ruby
ruby 怎么抛异常