In Java, if statement is used for testing the conditions. The condition matches the statement it returns true else it returns false. There are four types of If statement they are:
在Java中,if语句用于测试条件。 条件与返回true的语句匹配,否则返回false。 有四种类型的If语句:
For example, if we want to create a program to test positive integers then we have to test the integer whether it is greater that zero or not.
例如 ,如果我们要创建一个程序来测试正整数,则必须测试该整数是否大于零。
In this scenario, if statement is helpful.
在这种情况下,if语句很有帮助。
There are four types of if statement in Java:
Java中有四种类型的if语句:
if statement
如果声明
if-else statement
if-else语句
if-else-if ladder
if-else-if梯子
nested if statement
嵌套if语句
如果声明 (if Statement)
The if statement is a single conditional based statement that executes only if the provided condition is true.
if语句是一个基于条件的语句,仅在提供的条件为true时才执行。
If Statement Syntax:
如果语句语法:
if(condition)
{
//code
}
We can understand flow of if statement by using the below diagram. It shows that code written inside the if will execute only if the condition is true.
通过使用下图,我们可以了解if语句的流程。 它显示仅在条件为true时,在if内编写的代码才会执行。
If块的数据流图 (Data-flow-diagram of If Block)
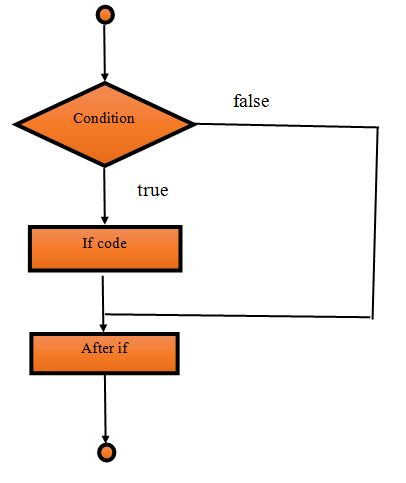
Example:
例:
In this example, we are testing students marks. If the marks are greater than 65 then student will get first division.
在此示例中,我们正在测试学生成绩。 如果分数大于65,则学生将获得一等奖。
public class IfDemo1 {
public static void main(String[] args)
{
int marks=70;
if(marks > 65)
{
System.out.print("First division");
}
}
}
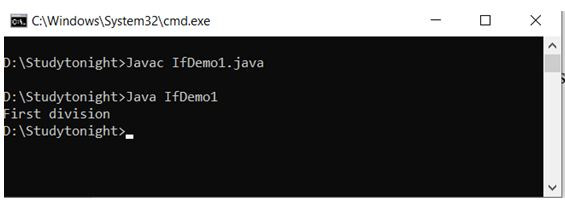
if-else语句 (if-else Statement)
The if-else statement is used for testing condition. If the condition is true, if block executes otherwise else block executes.
if-else语句用于测试条件。 如果条件为真,则执行块,否则执行块。
It is useful in the scenario when we want to perform some operation based on the false result.
当我们要基于错误的结果执行某些操作时,此方法很有用。
The else block execute only when condition is false.
else块仅在condition为false时执行。
Syntax:
句法:
if(condition)
{
//code for true
}
else
{
//code for false
}
In this block diagram, we can see that when condition is true, if block executes otherwise else block executes.
在此框图中,我们可以看到条件为真时,如果执行块,否则执行块。
If Else块的数据流图 (Data-flow-diagram of If Else Block)
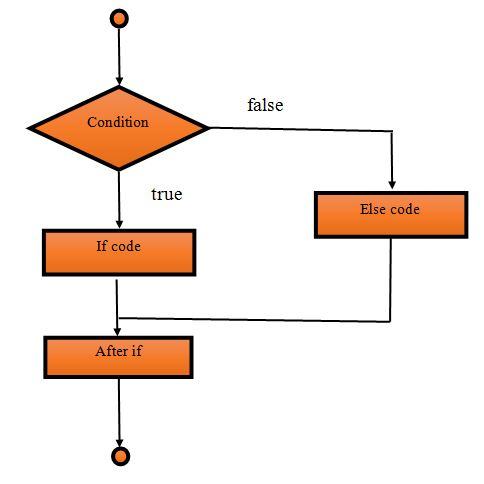
if else Example:
如果是其他示例:
In this example, we are testing student marks, if marks is greater than 65 then if block executes otherwise else block executes.
在此示例中,我们正在测试学生成绩,如果成绩大于65,则如果执行块,否则执行块。
public class IfElseDemo1 {
public static void main(String[] args)
{
int marks=50;
if(marks > 65)
{
System.out.print("First division");
}
else
{
System.out.print("Second division");
}
}
}
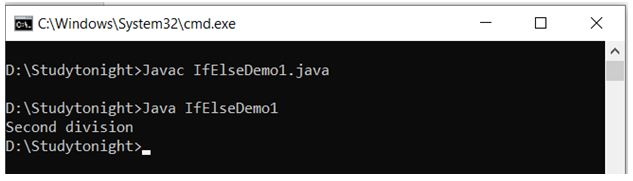
if-else-if梯形图语句 (if-else-if ladder Statement)
In Java, the if-else-if ladder statement is used for testing conditions. It is used for testing one condition from multiple statements.
在Java中,if-else-if阶梯语句用于测试条件。 它用于测试来自多个语句的一个条件。
When we have multiple conditions to execute then it is recommend to use if-else-if ladder.
当我们有多个条件要执行时,建议使用if-else-if阶梯。
Syntax:
句法:
if(condition1)
{
//code for if condition1 is true
}
else if(condition2)
{
//code for if condition2 is true
}
else if(condition3)
{
//code for if condition3 is true
}
...
else
{
//code for all the false conditions
}
It contains multiple conditions and execute if any condition is true otherwise executes else block.
它包含多个条件,如果任何条件为真,则执行,否则执行else块。
If Else If块的数据流图 (Data-flow-diagram of If Else If Block)
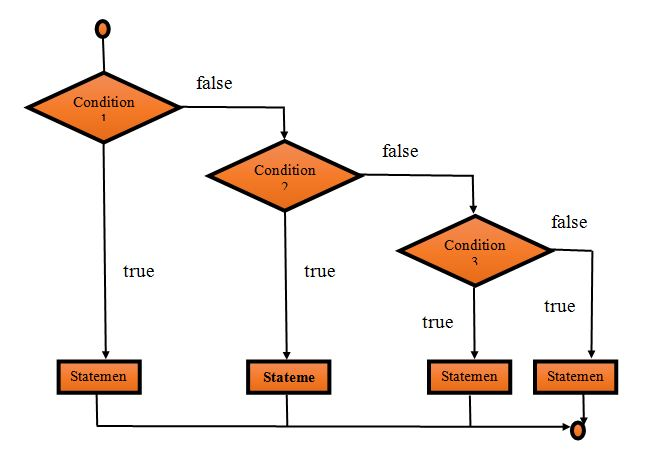
Example:
例:
Here, we are testing student marks and displaying result based on the obtained marks. If marks are greater than 50 student gets his grades.
在这里,我们正在测试学生成绩并根据获得的成绩显示结果。 如果分数大于50,则学生获得成绩。
public class IfElseIfDemo1 {
public static void main(String[] args) {
int marks=75;
if(marks<50){
System.out.println("fail");
}
else if(marks>=50 && marks<60){
System.out.println("D grade");
}
else if(marks>=60 && marks<70){
System.out.println("C grade");
}
else if(marks>=70 && marks<80){
System.out.println("B grade");
}
else if(marks>=80 && marks<90){
System.out.println("A grade");
}else if(marks>=90 && marks<100){
System.out.println("A+ grade");
}else{
System.out.println("Invalid!");
}
}
}
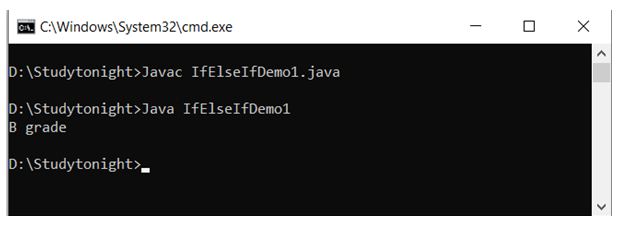
嵌套if语句 (Nested if statement)
In Java, the Nested if statement is a if inside another if. In this, one if block is created inside another if block when the outer block is true then only the inner block is executed.
在Java中,嵌套if语句是另一个if内部的if。 在这种情况下,如果外部块为true,则在另一个if块内部创建一个if块,然后仅执行内部块。
Syntax:
句法:
if(condition)
{
//statement
if(condition)
{
//statement
}
}
嵌套If块的数据流图 (Data-flow-diagram of Nested If Block)
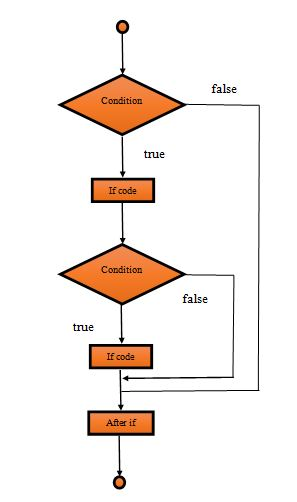
Example:
例:
public class NestedIfDemo1 {
public static void main(String[] args)
{
int age=25;
int weight=70;
if(age>=18)
{
if(weight>50)
{
System.out.println("You are eligible");
}
}
}
}
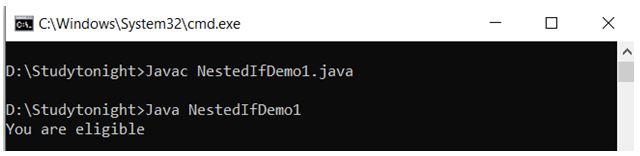
翻译自: https://www.studytonight.com/java/conditional-statement.php