string函数 java
The methods specified below are some of the most commonly used methods of the String
class in Java. We will learn about each method with help of small code examples for better understanding.
下面指定的方法是Java中String
类最常用的方法。 我们将在小代码示例的帮助下了解每种方法,以便更好地理解。
charAt()
方法 (charAt()
method)
String charAt()
function returns the character located at the specified index.
字符串charAt()
函数返回位于指定索引处的字符。
public class Demo {
public static void main(String[] args) {
String str = "studytonight";
System.out.println(str.charAt(2));
}
}
Output: u
输出: u
NOTE: Index of a String starts from 0, hence str.charAt(2)
means third character of the String str.
注意:字符串的索引从0开始,因此str.charAt(2)
表示字符串str的第三个字符。
equalsIgnoreCase()
方法 (equalsIgnoreCase()
method)
String equalsIgnoreCase()
determines the equality of two Strings, ignoring their case (upper or lower case doesn't matter with this method).
字符串equalsIgnoreCase()
确定两个字符串的相等性,而忽略它们的大小写(此方法的大小写无关紧要)。
public class Demo {
public static void main(String[] args) {
String str = "java";
System.out.println(str.equalsIgnoreCase("JAVA"));
}
}
true
真正
indexOf()
方法 (indexOf()
method)
String indexOf()
method returns the index of first occurrence of a substring or a character. indexOf() method has four override methods:
字符串indexOf()
方法返回第一次出现的子字符串或字符的索引。 indexOf()方法具有四个覆盖方法:
int indexOf(String str)
: It returns the index within this string of the first occurrence of the specified substring.int indexOf(String str)
:它返回指定子字符串首次出现在此字符串中的索引。int indexOf(int ch, int fromIndex)
: It returns the index within this string of the first occurrence of the specified character, starting the search at the specified index.int indexOf(int ch, int fromIndex)
:它返回指定字符首次出现在此字符串中的索引,从指定索引处开始搜索。int indexOf(int ch)
: It returns the index within this string of the first occurrence of the specified character.int indexOf(int ch)
:它返回指定字符首次出现在此字符串中的索引。int indexOf(String str, int fromIndex)
: It returns the index within this string of the first occurrence of the specified substring, starting at the specified index.int indexOf(String str, int fromIndex)
:它从指定的索引开始,返回指定子字符串首次出现在此字符串中的索引。
Example:
例:
public class StudyTonight {
public static void main(String[] args) {
String str="StudyTonight";
System.out.println(str.indexOf('u')); //3rd form
System.out.println(str.indexOf('t', 3)); //2nd form
String subString="Ton";
System.out.println(str.indexOf(subString)); //1st form
System.out.println(str.indexOf(subString,7)); //4th form
}
}
2 11 5 -1
2 11 5 -1
NOTE: -1 indicates that the substring/Character is not found in the given String.
注意: -1表示在给定的字符串中找不到子字符串/字符。
length()
方法 (length()
method)
String length()
function returns the number of characters in a String.
字符串length()
函数返回字符串中的字符数。
public class Demo {
public static void main(String[] args) {
String str = "Count me";
System.out.println(str.length());
}
}
8
8
replace()
方法 (replace()
method)
String replace()
method replaces occurances of character with a specified new character.
字符串replace()
方法用指定的新字符替换出现的字符。
public class Demo {
public static void main(String[] args) {
String str = "Change me";
System.out.println(str.replace('m','M'));
}
}
;
Change Me
改变我自己
substring()
方法 (substring()
method)
String substring()
method returns a part of the string. substring()
method has two override methods.
字符串substring()
方法返回字符串的一部分。 substring()
方法有两个覆盖方法。
1. public String substring(int begin);
1. public String substring(int begin);
2. public String substring(int begin, int end);
2. public String substring(int begin,int end);
The first argument represents the starting point of the subtring. If the substring()
method is called with only one argument, the subtring returns characters from specified starting point to the end of original string.
第一个参数表示替换的起点。 如果仅使用一个参数调用substring()
方法,则subtring返回从指定起点到原始字符串结尾的字符。
If method is called with two arguments, the second argument specify the end point of substring.
如果使用两个参数调用method,则第二个参数指定子字符串的终点。
public class Demo {
public static void main(String[] args) {
String str = "0123456789";
System.out.println(str.substring(4));
System.out.println(str.substring(4,7));
}
}
456789 456
456789 456
toLowerCase()
方法 (toLowerCase()
method)
String toLowerCase()
method returns string with all uppercase characters converted to lowercase.
String toLowerCase()
方法返回将所有大写字符转换为小写字母的字符串。
public class Demo {
public static void main(String[] args) {
String str = "ABCDEF";
System.out.println(str.toLowerCase());
}
}
abcdef
abcdef
toUpperCase()
方法 (toUpperCase()
method)
This method returns string with all lowercase character changed to uppercase.
此方法返回所有小写字符都变为大写的字符串。
public class Demo {
public static void main(String[] args) {
String str = "abcdef";
System.out.println(str.toUpperCase());
}
}
ABCDEF
ABCDEF
valueOf()
方法 (valueOf()
method)
String class uses overloaded version of valueOf()
method for all primitive data types and for type Object.
字符串类对所有原始数据类型和对象类型使用valueOf()
方法的重载版本。
NOTE: valueOf()
function is used to convert primitive data types into Strings.
注意: valueOf()
函数用于将原始数据类型转换为字符串。
public class Demo {
public static void main(String[] args) {
int num = 35;
String s1 = String.valueOf(num); //converting int to String
System.out.println(s1);
System.out.println("type of num is: "+s1.getClass().getName());
}
}
35 type of num is: java.lang.String
35的num类型是:java.lang.String
toString()
方法 (toString()
method)
String toString()
method returns the string representation of an object. It is declared in the Object class, hence can be overridden by any java class. (Object class is super class of all java classes).
String toString()
方法返回对象的字符串表示形式。 它在Object类中声明,因此可以被任何java类覆盖。 (对象类是所有java类的超类)。
public class Car {
public static void main(String args[])
{
Car c = new Car();
System.out.println(c);
}
public String toString()
{
return "This is my car object";
}
}
This is my car object
这是我的车对象
Whenever we will try to print any object of class Car, its toString()
function will be called.
每当我们尝试打印Car类的任何对象时,都会调用其toString()
函数。
NOTE: If we don't override the toString()
method and directly print the object, then it would print the object id that contains some hashcode.
注意:如果我们不重写toString()
方法并直接打印对象,则它将打印包含一些哈希码的对象ID。
trim()
方法 (trim()
method)
This method returns a string from which any leading and trailing whitespaces has been removed.
此方法返回一个字符串,该字符串已删除所有开头和结尾的空格。
public class Demo {
public static void main(String[] args) {
String str = " hello ";
System.out.println(str.trim());
}
}
hello
你好
contains()
方法 (contains()
Method)
String contains()
method is used to check the sequence of characters in the given string. It returns true if a sequence of string is found else it returns false.
字符串contains()
方法用于检查给定字符串中的字符序列。 如果找到字符串序列,则返回true,否则返回false。
public class Demo {
public static void main(String[] args) {
String a = "Hello welcome to studytonight.com";
boolean b = a.contains("studytonight.com");
System.out.println(b);
System.out.println(a.contains("javatpoint"));
}
}
true false
真假
endsWith()
方法 (endsWith()
Method)
String endsWith()
method is used to check whether the string ends with the given suffix or not. It returns true when suffix matches the string else it returns false.
String endsWith()
方法用于检查字符串是否以给定的后缀结尾。 后缀与字符串匹配时返回true,否则返回false。
public class Demo {
public static void main(String[] args) {
String a="Hello welcome to studytonight.com";
System.out.println(a.endsWith("m"));
System.out.println(a.endsWith("com"));
}
}
true true
真实真实
format()
方法 (format()
Method)
String format()
is a string method. It is used to the format of the given string.
字符串format()
是一个字符串方法。 它用于给定字符串的格式。
Following are the format specifier and their datatype:
以下是格式说明符及其数据类型:
Format Specifier | Data Type |
---|---|
%a | floating point |
%b | Any type |
%c | character |
%d | integer |
%e | floating point |
%f | floating point |
%g | floating point |
%h | any type |
%n | none |
%o | integer |
%s | any type |
%t | Date/Time |
%x | integer |
格式说明符 | 数据类型 |
---|---|
%一个 | 浮点 |
%b | 任何类型 |
%C | 字符 |
%d | 整数 |
%e | 浮点 |
%F | 浮点 |
%G | 浮点 |
%H | 任何类型 |
%n | 没有 |
%o | 整数 |
%s | 任何类型 |
%t | 约会时间 |
%X | 整数 |
public class Demo {
public static void main(String[] args) {
String a1 = String.format("%d", 125);
String a2 = String.format("%s", "studytonight");
String a3 = String.format("%f", 125.00);
String a4 = String.format("%x", 125);
String a5 = String.format("%c", 'a');
System.out.println("Integer Value: "+a1);
System.out.println("String Value: "+a2);
System.out.println("Float Value: "+a3);
System.out.println("Hexadecimal Value: "+a4);
System.out.println("Char Value: "+a5);
}
}
Integer Value: 125 String Value: studytonight Float Value: 125.000000 Hexadecimal Value: 7d Char Value: a
整数值:125字符串值:studytonight浮点值:125.000000十六进制值:7d字符值:a
getBytes()
方法 (getBytes()
Method)
String getBytes()
method is used to get byte array of the specified string.
String getBytes()
方法用于获取指定字符串的字节数组。
public class Demo {
public static void main(String[] args) {
String a="studytonight";
byte[] b=a.getBytes();
for(int i=0;i<b.length;i++)
{
System.out.println(b[i]);
}
}
}
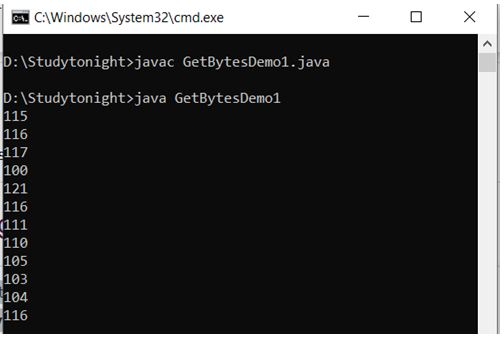
getChars()
方法 (getChars()
Method)
String getChars()
method is used to copy the content of the string into a char array.
String getChars()
方法用于将字符串的内容复制到char数组中。
public class Demo {
public static void main(String[] args) {
String a= new String("Hello Welcome to studytonight.com");
char[] ch = new char[16];
try
{
a.getChars(6, 12, ch, 0);
System.out.println(ch);
}
catch(Exception ex)
{
System.out.println(ex);
}
}
}
Welcom
威尔康
isEmpty()
方法 (isEmpty()
Method)
String isEmpty()
method is used to check whether the string is empty or not. It returns true when length string is zero else it returns false.
字符串isEmpty()
方法用于检查字符串是否为空。 当长度字符串为零时返回true,否则返回false。
public class IsEmptyDemo1
{
public static void main(String args[])
{
String a="";
String b="studytonight";
System.out.println(a.isEmpty());
System.out.println(b.isEmpty());
}
}
true false
真假
join()
方法 (join()
Method)
String join()
method is used to join strings with the given delimiter. The given delimiter is copied with each element
字符串join()
方法用于使用给定的定界符连接字符串。 给定的定界符将与每个元素一起复制
public class JoinDemo1
{
public static void main(String[] args)
{
String s = String.join("*","Welcome to studytonight.com");
System.out.println(s);
String date1 = String.join("/","23","01","2020");
System.out.println("Date: "+date1);
String time1 = String.join(":", "2","39","10");
System.out.println("Time: "+time1);
}
}
Welcome to studytonight.com Date: 23/01/2020 Time: 2:39:10
欢迎来到studytonight.com日期:2020年23月1日时间:2:39:10
startsWith()
方法 (startsWith()
Method)
String startsWith()
is a string method in java. It is used to check whether the given string starts with given prefix or not. It returns true when prefix matches the string else it returns false.
字符串startsWith()
是Java中的字符串方法。 它用于检查给定的字符串是否以给定的前缀开头。 当prefix与字符串匹配时返回true,否则返回false。
public class Demo {
public static void main(String[] args) {
String str = "studytonight";
System.out.println(str.startsWith("s"));
System.out.println(str.startsWith("t"));
System.out.println(str.startsWith("study",1));
}
}
true false false
真假假
字符串方法列表 (String Methods List)
Method | Description |
---|---|
char charAt(int index) | It returns char value for the particular index |
int length() | It returns string length |
static String format(String format, Object... args) | It returns a formatted string. |
static String format(Locale l, String format, Object... args) | It returns formatted string with given locale. |
String substring(int beginIndex) | It returns substring for given begin index. |
String substring(int beginIndex, int endIndex) | It returns substring for given begin index and end index. |
boolean contains(CharSequence s) | It returns true or false after matching the sequence of char value. |
static String join(CharSequence delimiter, CharSequence... elements) | It returns a joined string. |
static String join(CharSequence delimiter, Iterable<? extends CharSequence> elements) | It returns a joined string. |
boolean equals(Object another) | It checks the equality of string with the given object. |
boolean isEmpty() | It checks if string is empty. |
String concat(String str) | It concatenates the specified string. |
String replace(char old, char new) | It replaces all occurrences of the specified char value. |
String replace(CharSequence old, CharSequence new) | It replaces all occurrences of the specified CharSequence. |
static String equalsIgnoreCase(String another) | It compares another string. It doesn't check case. |
String[] split(String regex) | It returns a split string matching regex. |
String[] split(String regex, int limit) | It returns a split string matching regex and limit. |
String intern() | It returns an interned string. |
int indexOf(int ch) | It returns the specified char value index. |
int indexOf(int ch, int fromIndex) | It returns the specified char value index starting with given index. |
int indexOf(String substring) | It returns the specified substring index. |
int indexOf(String substring, int fromIndex) | It returns the specified substring index starting with given index. |
String toLowerCase() | It returns a string in lowercase. |
String toLowerCase(Locale l) | It returns a string in lowercase using specified locale. |
String toUpperCase() | It returns a string in uppercase. |
String toUpperCase(Locale l) | It returns a string in uppercase using specified locale. |
String trim() | It removes beginning and ending spaces of this string. |
static String valueOf(int value) | It converts given type into string. It is an overloaded method. |
方法 | 描述 |
---|---|
char charAt(int index) | 它返回特定索引的char值 |
int length() | 返回字符串长度 |
静态字符串格式(字符串格式,对象...参数) | 它返回一个格式化的字符串。 |
静态字符串格式(语言环境l,字符串格式,对象...参数) | 它以给定的语言环境返回格式化的字符串。 |
字符串子字符串(int beginIndex) | 它返回给定开始索引的子字符串。 |
字符串子字符串(int beginIndex,int endIndex) | 它返回给定的开始索引和结束索引的子字符串。 |
布尔contains(CharSequence s) | 匹配char值的序列后,它返回true或false。 |
静态字符串连接(CharSequence分隔符,CharSequence ...元素) | 它返回一个连接的字符串。 |
静态字符串连接(CharSequence分隔符,Iterable <?扩展了CharSequence>元素) | 它返回一个连接的字符串。 |
boolean equals(Object another) | 它检查字符串与给定对象的相等性。 |
布尔isEmpty() | 它检查字符串是否为空。 |
字符串concat(String str) | 它连接指定的字符串。 |
字符串替换(旧字符,新字符) | 它替换所有出现的指定char值。 |
字符串替换(旧的CharSequence,新的CharSequence) | 它替换所有出现的指定CharSequence。 |
静态字符串equalsIgnoreCase(另一个字符串) | 它比较另一个字符串。 它不检查大小写。 |
字符串[]分割(字符串正则表达式) | 它返回一个与正则表达式匹配的拆分字符串。 |
String []分割(String regex,int限制) | 它返回匹配正则表达式和限制的拆分字符串。 |
字符串intern() | 它返回一个中断的字符串。 |
int indexOf(int ch) | 它返回指定的char值索引。 |
int indexOf(int ch,int fromIndex) | 它从给定索引开始返回指定的char值索引。 |
int indexOf(字符串子字符串) | 它返回指定的子字符串索引。 |
int indexOf(字符串子串,int fromIndex) | 它返回以给定索引开头的指定子字符串索引。 |
字符串toLowerCase() | 它返回一个小写的字符串。 |
字符串toLowerCase(Locale l) | 它使用指定的语言环境以小写形式返回字符串。 |
字符串toUpperCase() | 它以大写形式返回字符串。 |
字符串toUpperCase(Locale l) | 它使用指定的语言环境以大写形式返回字符串。 |
字符串trim() | 它将删除此字符串的开头和结尾空格。 |
静态字符串valueOf(int value) | 它将给定类型转换为字符串。 这是一种重载方法。 |
翻译自: https://www.studytonight.com/java/string-class-functions.php
string函数 java