canvas静态仪表盘
My recent explorations in canvas animation, starfields and randomness came together in an experiment recreating television static… and as it turns out, they’re all related to each other. Like the starfield article, this will be an exploration of code with an improving, ongoing example.
我最近在画布动画 , 星空和随机性方面的探索结合在一起进行了重新创建电视静态影像的实验……事实证明,它们彼此相关。 像《星空》中的文章一样,这将是一个代码的探索,上面有一个不断完善的示例。
静态静态 (Static static)
The first task is to create static, as shown in the example above. For an image, that’s fairly straightforward: take a <canvas>
element of known width
and height
and fill it with small drawn rectangles in various shades of grey.
如上例所示,第一个任务是创建静态对象。 对于图像,这非常简单:采用已知width
和height
的<canvas>
元素,并用各种灰色阴影绘制的小矩形填充它。
Our canvas
element:
我们的canvas
元素:
<canvas id="static" width="750" height="500"></canvas>
The initial variables in the script, added to the bottom of the page:
脚本中的初始变量,添加到页面底部:
var canvas = document.getElementById("static"),
context = canvas.getContext("2d"),
tvHeight = canvas.offsetHeight,
tvWidth = canvas.offsetWidth,
smallestPixel = 4;
Using this information, we can create the function to draw the static:
使用此信息,我们可以创建绘制静态函数:
function drawStatic() {
for (var v=0; v < tvHeight; v += smallestPixel){
for (var h=0; h < tvWidth; h += smallestPixel){
lum = Math.floor( Math.random() * 50 );
context.fillStyle = "hsl(0, 0%," + lum + "%)";
context.fillRect(h,v, smallestPixel, smallestPixel);
}
}
}
The function contains two nested for
loops. The first works vertically, increasing by the distance determined by smallestPixel
. Within the loop is another working horizontally, filling each row with a square filled with an HSL grey. When a row completes, the next horizontal row starts.
该函数包含两个嵌套的for
循环 。 第一个作品垂直,由距离增加所确定smallestPixel
。 在循环中,另一个是水平工作的,在每行中填充一个用HSL灰色填充的正方形。 当一行完成时,下一个水平行开始。
To make the static pixels darker, reduce the lum
value. To make them finer, reduce the smallestPixel
value. You could make the static elements rectangular by providing different variables for “pixel” height and width, or by multiplying smallestPixel
to produce a different width.
要使静态像素更暗,请降低lum
值。 为了使其更精细 ,请减小smallestPixel
值。 您可以通过为“像素”的高度和宽度提供不同的变量,或通过将smallestPixel
乘以产生不同的宽度,来使静态元素变为矩形。
使画布充满屏幕 (Making The Canvas Fill The Screen)
To make the <canvas>
fill the page we turn to an old and trusted technique in CSS:
为了使<canvas>
填充页面,我们转向CSS中的一种古老且值得信赖的技术:
body, html {
margin: 0; height:100%;
}
#static {
position:absolute;
width:100%;
height:100%;
}
优化绘图时间 (Optimising The Draw Time)
If the <canvas>
is 800 pixels across and 400 high, and each “pixel” is 4 × 4, that makes for 200 “pixels” per row, for a total of 20,000 drawn squares. JavaScript can achieve this, but it may struggle to draw updates to the rectangles 30 times a second; if we want to animate the effect, we may need to consider reducing the number of elements.
如果<canvas>
的<canvas>
为800像素,高度为400,并且每个“像素”为4×4,则每行200个“像素”,总共20,000个绘制的正方形。 JavaScript可以实现这一点,但是它可能很难每秒更新30次矩形。 如果要动画效果,则可能需要考虑减少元素数量。
To do that, we could reduce the height
and width
of the <canvas>
element and / or increase the “pixel” size. Alternatively, we could draw larger pixel shapes, and fewer of them. We could create the default “color” of the TV screen by flood-filling the background with CSS:
为了做到这一点 ,我们可以减少height
及width
的的<canvas>
元件和/或增加“像素”的尺寸。 或者,我们可以绘制较大的像素形状,而绘制较少的像素形状。 我们可以通过用CSS填充背景来创建电视屏幕的默认“颜色”:
#static { background: #333; }
Then, randomly filling the space with rectangles. The rectangles will be a random height and width, between certain limits. That height and width will also determine the position of the rectangle: it will be positioned from 0 to the width or height of the canvas, less the dimension of the pixel. We’ll create 1200 rectangles using a new function. First, I’ll use a function from my random recipes collection:
然后,用矩形随机填充空间。 矩形将是一定高度之间的随机高度和宽度。 该高度和宽度也将确定矩形的位置:它将从0到画布的宽度或高度(减去像素的尺寸)定位。 我们将使用新功能创建1200个矩形。 首先,我将使用随机食谱集合中的一个函数:
function getRandomInRange(min, max) {
return Math.floor(Math.random() * (max - min + 1)) + min;
}
The rest of the code starts the same as the previous example:
其余代码与前面的示例相同:
var canvas = document.getElementById("static"),
context = canvas.getContext("2d"),
tvHeight = canvas.offsetHeight,
tvWidth = canvas.offsetWidth,
staticWidth = 0,
staticHeight = 0,
smallestWidth = 8,
largestWidth = 32,
smallestHeight = 4,
largestHeight = 8,
maxPixels = 1200,
horizontalPosition = 0,
verticalPosition = 0;
The drawStatic
function changes too:
drawStatic
函数也会更改:
function drawStatic() {
for (var i=0; i < maxPixels; i++){
lum = getRandomInRange(40,80);
context.fillStyle = "hsla(0, 0%," + lum + "%, 0.8)";
staticWidth = getRandomInRange(smallestWidth, largestWidth);
staticHeight = getRandomInRange(smallestHeight, largestHeight);
horizontalPosition = getRandomInRange(0, tvWidth - staticWidth);
verticalPosition = getRandomInRange(0, tvHeight - staticHeight);
context.fillRect(horizontalPosition,verticalPosition, staticWidth, staticHeight);
}
}
The result is “rougher”, and perhaps more suited for animation:
结果是“更粗糙”,也许更适合动画:
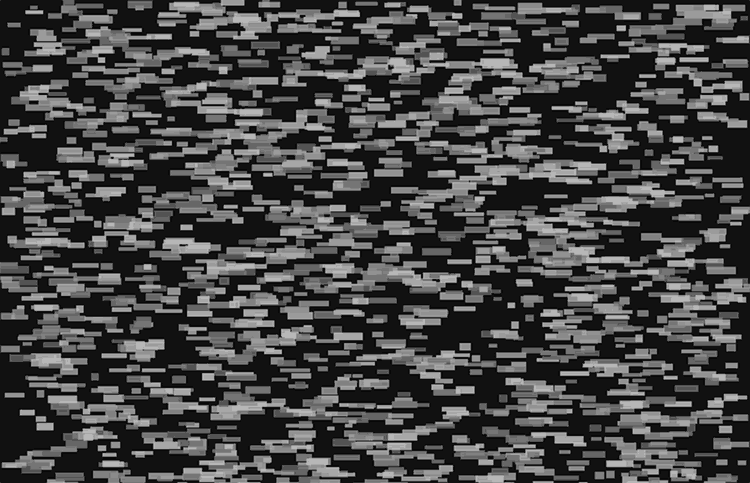
I’ll be looking at this animation in the next article.
我将在下一篇文章中观看此动画。
翻译自: https://thenewcode.com/1101/Generating-Static-in-HTML5-Canvas
canvas静态仪表盘