Functions are a core part of JavaScript; they’re used everywhere. Thankfully, basic functions are also pretty straightforward.
函数是JavaScript的核心部分; 它们无处不在。 值得庆幸的是,基本功能也非常简单。
功能自动化任务 (Functions Automate Tasks)
Let’s imagine you’re coming home after a hard day’s work, and that at the end of the day, there’s nothing you like better than a glass of ice-cold lemonade. Good news: in the magical future world of home automation, you won’t have to make it yourself; your friendly household robot can prepare your drink for you. All we have to do is to write a function to make that happen.
假设您辛苦了一天的工作后回到家,到一天结束时,没有比喝一杯冰镇柠檬水更好的了。 好消息:在神奇的未来家庭自动化世界中,您将不必自己动手制作; 您友好的家用机器人可以为您准备饮料。 我们要做的就是编写一个函数来实现这一目标。
To start, let’s write it in pseudo-code: a “sketch” that won’t actually work in a browser, but will give us an idea of what we’re after:
首先,让我们用伪代码编写它:一种“草图”,它实际上不会在浏览器中起作用,但是会给我们一个大致的概念:
door.open {
makeLemonade();
}
In this scenario, the door
is an object, open
is an event, and makeLemonade
is a function. We haven’t written that function yet, so our home automation system has no idea what to do when it encounters our call to makeLemonade()
. Let’s change that by adding the following:
在这种情况下, door
是一个对象 , open
是一个事件,而makeLemonade
是一个函数。 我们尚未编写该函数,因此我们的家庭自动化系统在遇到对makeLemonade()
调用时不知道该怎么办。 让我们通过添加以下内容来更改它:
function makeLemonade() {
squeezeLemons;
addSugar;
…
}
Pretty straightforward, right? The function breaks down the task of making lemonade into individual statements in a code block between opening and closing curly braces. When we want those statements to be executed, we call on the function by name.
很简单吧? 该函数将在打开和关闭大括号之间的代码块中使柠檬水变成单个语句的任务分解。 当我们想要执行这些语句时,我们按名称调用该函数。
Let’s write some actual code now. Sadly, we don’t yet have a lemonade-making household robot; instead, we’ll write some JavaScript to change an image and some text on a web page.
现在让我们写一些实际的代码。 令人遗憾的是,我们还没有制造柠檬水的家用机器人。 相反,我们将编写一些JavaScript来更改网页上的图像和文本。
First, let’s add default text and an image to the page, just in case the JavaScript doesn’t execute for any reason, using the principles of graceful degradation:
首先,让我们在页面上添加默认文本和图像 ,以防JavaScript由于某种原因而无法执行,请使用正常降级的原则:
<section id="welcomebeverage">
<h1>You deserve a Coke</h1>
<img src="coke.jpg" alt="A glass filled with Coca-Cola
splashing with a dropped ice-cube">
</section>
We’ll declare our function in a script at the end of the page (positioning the script in this location will effectively act as a “document load / door open” event).
我们将在页面末尾的脚本中声明函数(将脚本放置在此位置将有效地充当“文档加载/开门”事件)。
var welcome = document.getElementById("welcomebeverage"),
welcomeMsg = welcome.querySelector("h1"),
welcomeImg = welcome.querySelector("img");
function makeLemonade() {
welcomeMsg.innerHTML = "You deserve a frosty lemonade.";
welcomeImg.src = "lemonade.jpg";
}
makeLemonade();
Assuming we have coke.jpg
and lemonade.jpg
beside our page, the result will look something like this:
假设我们在页面旁边有coke.jpg
和lemonade.jpg
,结果将如下所示:
注意: (Note:)
The function may be written before or after the call to it; JavaScript knows to “look ahead” in your code for functions. Traditionally, functions are written first, to make the code cleaner and easier to understand.
该函数可以在调用之前或之后编写; JavaScript知道要在代码中“向前看”功能。 传统上,函数是首先编写的,以使代码更简洁,更易于理解。
Named functions are not run unless they are explicitly called. (Anonymous functions are an exception, and can run automatically).
除非显式调用 命名函数,否则它们不会运行。 ( 匿名函数是一个例外,可以自动运行)。
In this case the variables could be placed inside the function, as long as we were aware of the effect on scope. I’ll do that in the next version of the script.
在这种情况下,只要我们知道对scope的影响,就可以将变量放置在函数中。 我将在脚本的下一个版本中进行操作。
Both the function name and our call to it must have parentheses, even if we are not passing any arguments. (I’ll discuss arguments and parameters in a moment).
即使我们没有传递任何参数 ,函数名称和对其的调用都必须带有括号。 (我将在稍后讨论参数)。
When it has finished executing, the function returns to the point in the code from which it was called.
完成执行后,函数将返回到代码中调用它的位置 。
We should also change the image’s
alt
attribute with JavaScript to reflect the content of the new image; I’ll leave that as an exercise for the reader.我们还应该使用JavaScript更改图片的
alt
属性 ,以反映新图片的内容; 我将其留给读者练习。
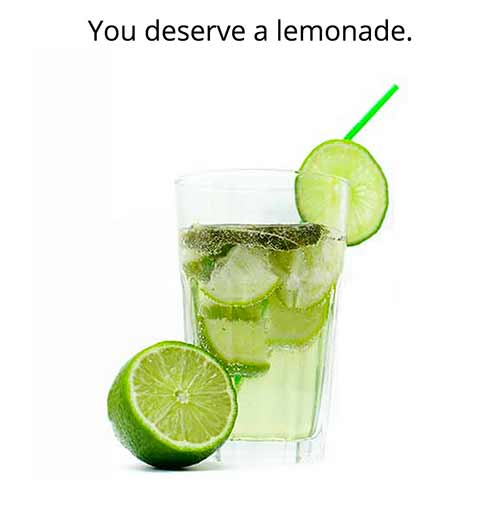
This is good, but there’s really nothing here that couldn’t be made without a function, as the code is called just once. Let’s expand on that: say you wanted the function to serve you a cappuccino in the morning and a lemonade in the afternoon/evening, with a Coke at all other times. To do this, we’ll abstract our code to include other beverages:
很好,但是这里实际上没有没有函数无法完成的事情,因为代码仅被调用一次。 让我们对其进行扩展:假设您希望该功能在早上为您提供一杯卡布奇诺咖啡,在下午/晚上为您提供柠檬水,而在其他所有时间都提供可乐。 为此,我们将抽象代码以包含其他饮料:
function makeBeverage(drink) {
var welcome = document.getElementById("welcomebeverage");
var welcomeMsg = welcome.querySelector("h1");
var welcomeImg = welcome.querySelector("img");
var beverages = ["Coke", "cappuccino", "lemonade"];
welcomeMsg.innerHTML="Hey - you deserve a "+beverages[drink];
welcomeImg.src=beverages[drink]+".jpg";
}
var currentTime = new Date();
var timePeriod = Math.round(currentTime.getHours()/12);
makeBeverage(timePeriod);
The JavaScript function has been expanded to include a parameter. This parameter is referenced inside the function as a variable, and usually contributes to changing its output. In this case, we’re sending makeBeverage
an argument: the current time on the user’s device. This value is measured in 24-hour time, divided by 12; so it’s between midnight and 6am, the value will be 0; 6am to noon, 1, and so on.
JavaScript函数已扩展为包含参数 。 该参数在函数内部作为变量引用,通常有助于更改其输出。 在这种情况下,我们makeBeverage
发送一个参数 :用户设备上的当前时间。 该值以24小时制除以12。 因此,它是在午夜至凌晨6点之间,该值为0; 上午6点至中午1点,依此类推。
Functions are sent arguments; they receive parameters.
函数是发送参数; 他们接收参数。
Adding more than one argument in a function call, or more than one parameter in a function declaration, means they must be separated by commas. For example, if we wanted to control how many drinks were made by our function, we could call it with:
在函数调用中添加多个参数或在函数声明中添加多个参数,意味着它们必须用逗号分隔。 例如,如果我们想控制通过我们的功能制作的饮料数量,则可以使用以下命令进行调用:
makeBeverage(timePeriod, amount);
The function matches the arguments used in the call with its parameters, which may take the same names, or use different ones:
该函数将调用中使用的参数与其参数进行匹配,这些参数可以采用相同的名称,也可以使用不同的名称:
function makeBeverage(drink, amount) {
}
In this case, the argument timePeriod
will match the parameter drink
used in the function, and amount
will match amount
. Arguments and parameters are always matched in order, on a “first come, first served” basis.
在这种情况下,参数timePeriod
将匹配参数drink
在函数中使用,和amount
将匹配amount
。 参数和参数始终按“先到先得”的顺序进行匹配。
Because parameters remain as local variables inside the function scope, any changes to amount
inside the function will not affect the variable amount
outside it.
因为参数保持为函数范围内的局部变量,任何变化amount
的函数内不会影响变量amount
外面 。
If a function is sent more arguments than it has parameters, it ignores any excess arguments; if it is sent fewer arguments than it has parameters, the parameters that have not gained values are set to undefined.
如果向函数发送的参数多于其具有参数的参数,则它将忽略任何多余的参数; 如果向其发送的参数少于其具有参数的参数,则未获得值的参数将设置为undefined 。
Our function receives this information - the 0, 1 or 2 value - which is referenced as a variable within the scope of the function, using the parameter name between the parentheses. In this case, the value of 0, 1 or 2 is placed inside a parameter called drink
, which is used to look inside a beverages
array. The appropriate “slot” in the array, based on this value, is then used to complete the welcoming message and image via concatenation.
我们的函数使用括号之间的参数名称接收该信息-0、1或2值-在函数范围内作为变量引用。 在这种情况下,将0、1或2的值放置在称为drink
的参数内,该参数用于查看beverages
数组的内部。 然后,基于此值,在数组中使用适当的“插槽”,通过串联来完成欢迎消息和图像。
As a result, the offered beverage will differ between three options, depending on the time of day. But this function still runs only once. To change that, we’ll use setInterval
to call the function every hour.
结果,根据一天中的时间,所提供的饮料将在三个选项之间有所不同。 但是此功能仍然只运行一次。 要更改此设置,我们将使用setInterval
每小时调用一次该函数。
函数要做的事情不只一次 (Functions Do Anything You Want Done More Than Once)
We’ll add one line to the end of the script:
我们将在脚本末尾添加一行:
setInterval( function() { makeBeverage(timePeriod); }, 1000*60*60 );
We can call a function as many times as we want, and from anywhere in a script. This line uses an anonymous function to set a callback to makeBeverage
every hour. The makeBeverage()
line immediately before it remains in place, as we want the function to run immediately when the page is loaded, and again every hour after that.
我们可以在脚本中的任意位置调用任意次数的函数。 该行使用匿名函数将每小时的回调设置为makeBeverage
。 makeBeverage()
行紧接在行之前,因为我们希望函数在页面加载后立即运行,此后每小时运行一次。
Time in JavaScript is measured in milliseconds (thousandths of a second), making our delay of 1000 × 60 × 60 equal to one hour.
JavaScript中的时间以毫秒(千分之一秒)为单位,这使我们的1000×60×60延迟等于一小时。
奖金回合 (Bonus Round)
Once you understand functions, by association you also understand methods, which are simply functions that are part of an object.
结论 (Conclusion)
There’s much more to functions, including functions returning values, function expressions, and immediately invoked functions. I’ll cover those in future articles; for now, experiment with the example on CodePen, with the knowledge that you’ve just learned of the most powerful features of JavaScript and most other coding languages.
函数还有更多功能,包括返回值的函数,函数表达式和立即调用的函数。 我将在以后的文章中介绍这些内容。 现在,使用您刚刚了解JavaScript和大多数其他编码语言的最强大功能的知识,在CodePen上试用该示例。
翻译自: https://thenewcode.com/1033/Introduction-to-JavaScript-Functions