在上一教程中,您了解了如何使用Angular路由在Angular Web应用程序中实现路由。
在本教程中,您将学习如何使用Angular HttpClient
与Angular应用程序中的Web API和服务进行通信。
Angular HttpClient
您使用XMLHttpRequest(XHR)
对象从浏览器进行服务器端API调用。 XHR
对象使更新网页的一部分成为可能,而无需重新加载整个页面。 网页加载后,您可以从服务器发送和接收数据。
Angular HttpClient
为XHR
对象提供了包装器,使从Angular应用程序进行API调用变得更加容易。
从官方文档中:
通过HttpClient
,@angular/common/http
在浏览器公开的XMLHttpRequest
接口的基础上,为Angular应用程序提供了用于HTTP功能的简化API。HttpClient
其他好处包括可测试性支持,对请求和响应对象的强类型输入,对请求和响应拦截器的支持以及通过基于Observable的api更好的错误处理。
入门
您将从克隆本系列第一部分的源代码开始。
git clone https://github.com/royagasthyan/AngularComponent.git
获得源代码后,导航到项目目录并安装所需的依赖项。
cd AngularComponent
npm install
安装依赖项后,通过键入以下命令来启动应用程序:
ng serve
您应该在http:// localhost:4200 /上运行该应用程序。
为了在Angular应用程序中使用HttpClient
模块,您需要首先在app.module.ts
文件app.module.ts
其导入。
在项目目录中,导航到src/app/app.module.ts
。 在app.module.ts
文件中,导入HttpClientModule
。
import {HttpClientModule} from '@angular/common/http';
在imports部分下包括HttpClientModule
。
imports: [
BrowserModule,
FormsModule,
HttpClientModule
]
这是app.module.ts
文件的外观:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
import { CalcComponent } from './calc/calc.component'
import { FormsModule } from '@angular/forms';
import { HttpClientModule } from '@angular/common/http';
@NgModule({
declarations: [
AppComponent,
CalcComponent
],
imports: [
BrowserModule,
FormsModule,
HttpClientModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
现在,您可以通过在特定的Angular组件中导入HttpClientModule
在整个Angular应用程序中使用它。
创建角度组件
让我们从创建Angular组件开始。 在src/app
文件夹中创建一个名为get-data
的文件夹。 创建一个名为get-data.component.ts
的文件,并添加以下代码:
import { Component } from '@angular/core';
@Component({
selector: 'get-data',
templateUrl: 'get-data.component.html',
styleUrls: ['get-data.component.css']
})
export class GetDataComponent {
constructor(){
}
}
创建一个名为get-data.component.html
文件,该文件将用作get-data
组件的模板文件。 为get-data.component.html
文件添加以下代码:
<h3>
Get Data Component
</h3>
将GetDataComponent
导入src/app/app.module.ts
文件。
import { GetDataComponent } from './get-data/get-data.component';
将GetDataComponent
添加到ngModule
中的app.module.ts
。
declarations: [
AppComponent,
CalcComponent,
GetDataComponent
]
这是修改后的app.module.ts
文件的外观:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
import { CalcComponent } from './calc/calc.component';
import { GetDataComponent } from './get-data/get-data.component';
import { FormsModule } from '@angular/forms';
import { HttpClientModule } from '@angular/common/http';
@NgModule({
declarations: [
AppComponent,
CalcComponent,
GetDataComponent
],
imports: [
BrowserModule,
FormsModule,
HttpClientModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
将get-data
组件选择器添加到app.component.html
文件。 外观如下:
<div style="text-align:center">
<get-data></get-data>
</div>
保存以上更改并启动服务器。 您将能够查看在加载应用程序时显示的get-data
组件。
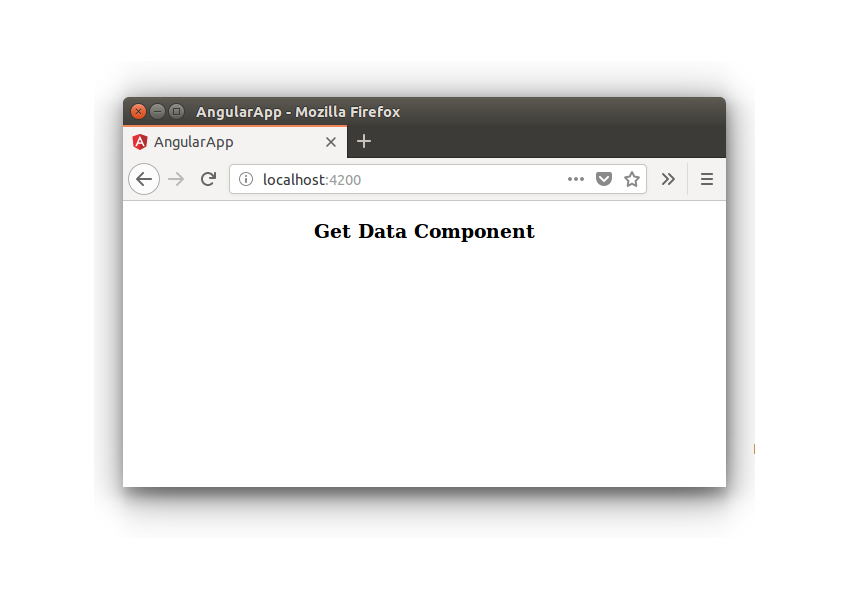
使用Angular HttpClient模块
您将使用HttpClient
模块进行API调用。 让我们创建一个单独的文件来创建用于进行API调用的Angular服务。 创建一个名为get-data.service.ts
文件。 将以下代码添加到get-data.service.ts
文件:
import { Injectable } from '@angular/core';
@Injectable()
export class GetDataService {
constructor() {
}
}
将HttpClient
模块导入上述GetDataService
。
import { HttpClient } from '@angular/common/http';
在GetDataService
构造函数中初始化HttpClient
。
constructor(private http: HttpClient) {
}
创建一个名为call_api
的方法以在GetDataService
文件中进行GET API调用。 您将使用单词同义词查找器API进行API调用。 这是call_api
方法的外观:
call_api(term) {
return this.http.get(this.url + term);
}
这是get-data.service.ts
文件的外观:
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Injectable()
export class GetDataService {
url: string
constructor(private http: HttpClient) {
this.url = 'https://api.datamuse.com/words?ml='
}
call_api(term) {
return this.http.get(this.url + term);
}
}
如上面的GetDataService
类所示, call_api
方法返回一个可观察对象,您可以从GetDataComponent
订阅该GetDataComponent
。
要从GetDataComponent
的构造函数进行订阅,您需要在GetDataComponent
导入GetDataService
。
import { GetDataService } from './get-data.service';
将GetDataService
定义为GetDataComponent
的提供程序。
@Component({
selector: 'get-data',
templateUrl: 'get-data.component.html',
styleUrls: ['get-data.component.css'],
providers:[ GetDataService ]
})
让我们订阅GetDataComponent
的构造方法。
constructor(private dataService: GetDataService){
this.dataService.call_api('hello').subscribe(response => {
console.log('Response is ', response);
})
}
这是get-data.component.ts
文件的外观:
import { Component } from '@angular/core';
import { GetDataService } from './get-data.service';
@Component({
selector: 'get-data',
templateUrl: 'get-data.component.html',
styleUrls: ['get-data.component.css'],
providers:[ GetDataService ]
})
export class GetDataComponent {
constructor(private dataService: GetDataService){
this.dataService.call_api('hello').subscribe(response => {
console.log('Response is ', response);
})
}
}
保存以上更改,然后重新启动服务器。 您将能够在浏览器控制台中看到记录的结果。
在上面的代码中,您看到了如何使用Angular HttpClient
发出GET API请求。
要发出POST请求,您需要使用http.post
方法。
this.http.post(url, {key : value});
如上面的代码所示,您需要提供API发布URL和要发布的数据作为第二个参数。
进行API调用时处理错误
每当您进行API调用时,遇到错误的可能性均等。 为了在进行API调用时处理错误,您需要将一个错误处理程序与response
处理程序一起添加到subscribe
方法中。
这是从GetDataComponent
修改后的代码的样子:
constructor(private dataService: GetDataService){
this.dataService.call_api('hello').subscribe(response => {
console.log('Response is ', response);
}, err => {
console.log('Something went wrong ', err);
})
}
修改get-data.service.ts
文件中的url
变量以创建不存在的URL,这将在进行API调用时导致错误。
this.url = 'https://ai.datamuse.com/words?ml='
保存以上更改,然后重新启动服务器。 检查浏览器控制台,您将调用错误处理程序方法并记录错误。
错误对象包含有关错误的所有详细信息,例如错误代码,错误消息等。它可以更深入地了解API调用出了什么问题。 这是浏览器控制台日志的外观:
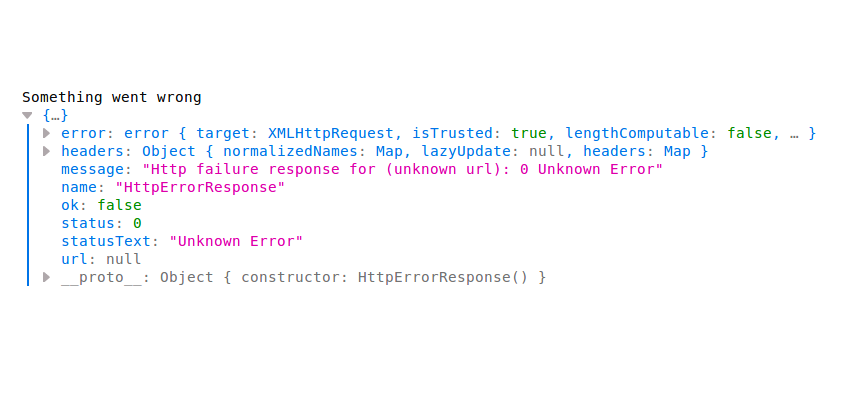
从上面的控制台日志中可以看到,所有错误详细信息都可以从错误对象中获取。
包起来
在本教程中,您了解了如何利用Angular HttpClientModule
进行API调用。 您学习了如何导入HttpClientModule
以向服务器API发出GET和POST请求。 您了解了进行API调用时如何处理错误。
您的经验如何尝试学习如何使用Angular HttpClientModule
进行API调用? 请在下面的评论中告诉我们您的想法。
该教程的源代码可在GitHub上获得 。
翻译自: https://code.tutsplus.com/tutorials/beginners-guide-to-angular-4-http--cms-29677