在这个由两部分组成的教程系列的第一部分中,我们概述了如何在Google Cloud Storage上使用存储桶来组织文件。 我们了解了如何从Google Cloud Console管理Google Cloud Storage上的存储桶。 随后是Python脚本,其中这些操作以编程方式执行。
在这一部分中,我将演示如何管理对象,即GCS存储桶中的文件和文件夹。 本教程的结构将与上一教程的结构相似。 首先,我将演示如何使用Google Cloud Console执行与文件管理相关的基本操作。 随后是Python脚本,以编程方式执行相同的操作。
就像GCS中的存储桶命名有一些准则和约束一样,对象命名也遵循一系列准则 。 对象名称应包含有效的Unicode字符,并且不应包含回车符或换行符。 一些建议包括不要使用诸如“#”,“ [”,“]”,“ *”,“?”之类的字符。 或非法的XML控制字符,因为它们可能被错误地解释并可能导致歧义。
此外,GCS中的对象名称遵循统一的名称空间。 这实际上意味着GCS上没有目录和子目录。 例如,如果您创建一个名称为/tutsplus/tutorials/gcs.pdf
的文件,它将看起来好像gcs.pdf
位于一个名为tutorials
的目录中,该目录又是tutsplus
的子目录。 但是根据GCS,该对象只是驻留在名称为/tutsplus/tutorials/gcs.pdf
的存储桶中。
让我们看看如何使用Google Cloud Console管理对象,然后跳转到Python脚本以编程方式执行相同的操作。
使用Google Cloud Console
我将从上一个教程中剩下的地方继续。 让我们开始创建一个文件夹。

要创建一个新文件夹,请单击上方突出显示的“ 创建文件夹”按钮。 通过填写所需的名称创建一个文件夹,如下所示。 名称应遵循对象命名约定 。
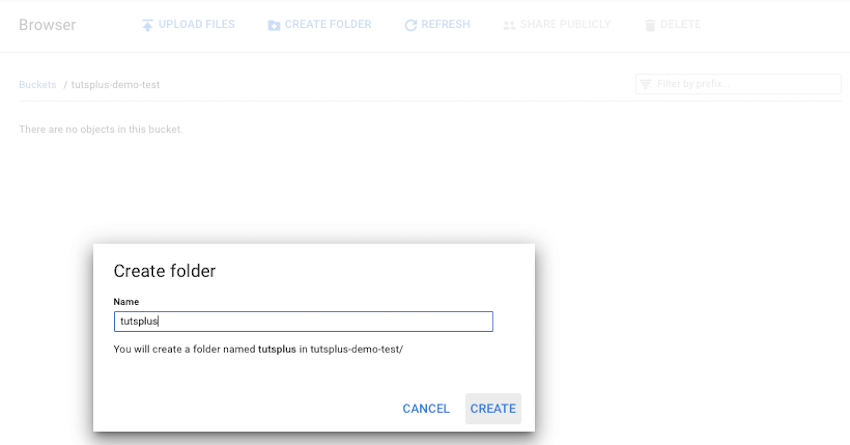
现在,我们将文件上传到新创建的文件夹中。
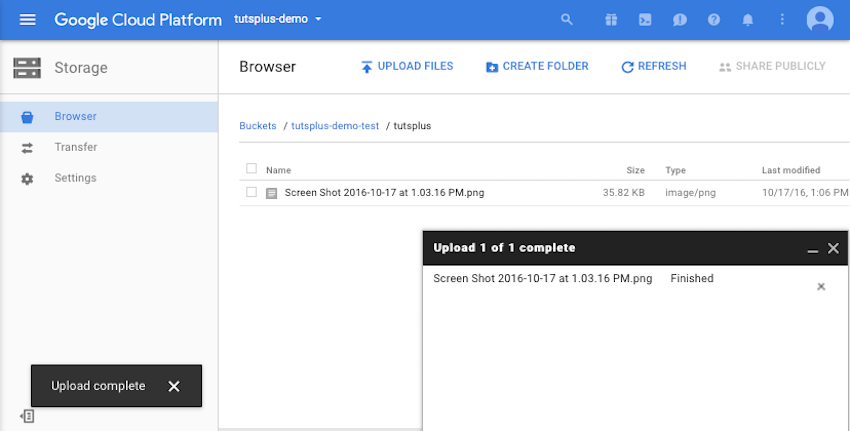
创建后,GCS浏览器将列出新创建的对象。 通过从列表中选择对象并单击删除按钮,可以删除对象。
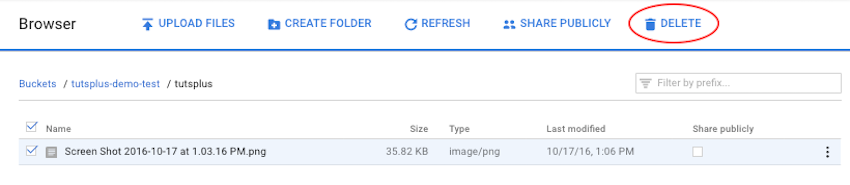
单击刷新按钮将使用对象列表的任何更改填充UI,而不会刷新整个页面。
以编程方式管理对象
在第一部分中,我们了解了如何创建Compute Engine实例。 我将在此处使用相同的内容,并在最后一部分的Python脚本的基础上进行构建。
编写Python脚本
本教程不需要遵循其他安装步骤。 有关安装或开发环境的更多详细信息,请参考第一部分。
gcs_objects.py
import sys
from pprint import pprint
from googleapiclient import discovery
from googleapiclient import http
from oauth2client.client import GoogleCredentials
def create_service():
credentials = GoogleCredentials.get_application_default()
return discovery.build('storage', 'v1', credentials=credentials)
def list_objects(bucket):
service = create_service()
# Create a request to objects.list to retrieve a list of objects.
fields_to_return = \
'nextPageToken,items(name,size,contentType,metadata(my-key))'
req = service.objects().list(bucket=bucket, fields=fields_to_return)
all_objects = []
# If you have too many items to list in one request, list_next() will
# automatically handle paging with the pageToken.
while req:
resp = req.execute()
all_objects.extend(resp.get('items', []))
req = service.objects().list_next(req, resp)
pprint(all_objects)
def create_object(bucket, filename):
service = create_service()
# This is the request body as specified:
# http://g.co/cloud/storage/docs/json_api/v1/objects/insert#request
body = {
'name': filename,
}
with open(filename, 'rb') as f:
req = service.objects().insert(
bucket=bucket, body=body,
# You can also just set media_body=filename, but for the sake of
# demonstration, pass in the more generic file handle, which could
# very well be a StringIO or similar.
media_body=http.MediaIoBaseUpload(f, 'application/octet-stream'))
resp = req.execute()
pprint(resp)
def delete_object(bucket, filename):
service = create_service()
res = service.objects().delete(bucket=bucket, object=filename).execute()
pprint(res)
def print_help():
print """Usage: python gcs_objects.py <command>
Command can be:
help: Prints this help
list: Lists all the objects in the specified bucket
create: Upload the provided file in specified bucket
delete: Delete the provided filename from bucket
"""
if __name__ == "__main__":
if len(sys.argv) < 2 or sys.argv[1] == "help" or \
sys.argv[1] not in ['list', 'create', 'delete', 'get']:
print_help()
sys.exit()
if sys.argv[1] == 'list':
if len(sys.argv) == 3:
list_objects(sys.argv[2])
sys.exit()
else:
print_help()
sys.exit()
if sys.argv[1] == 'create':
if len(sys.argv) == 4:
create_object(sys.argv[2], sys.argv[3])
sys.exit()
else:
print_help()
sys.exit()
if sys.argv[1] == 'delete':
if len(sys.argv) == 4:
delete_object(sys.argv[2], sys.argv[3])
sys.exit()
else:
print_help()
sys.exit()
上面的Python脚本演示了可以对对象执行的主要操作。 这些包括:
- 在存储桶中创建新对象
- 列出存储桶中的所有对象
- 删除特定对象
让我们看看运行脚本时上述每个操作的外观。
$ python gcs_objects.py
Usage: python gcs_objects.py <command>
Command can be:
help: Prints this help
list: Lists all the objects in the specified bucket
create: Upload the provided file in specified bucket
delete: Delete the provided filename from bucket
$ python gcs_objects.py list tutsplus-demo-test
[{u'contentType': u'application/x-www-form-urlencoded;charset=UTF-8',
u'name': u'tutsplus/',
u'size': u'0'},
{u'contentType': u'image/png',
resp = req.execute()
u'name': u'tutsplus/Screen Shot 2016-10-17 at 1.03.16 PM.png',
u'size': u'36680'}]
$ python gcs_objects.py create tutsplus-demo-test gcs_buckets.py
{u'bucket': u'tutsplus-demo-test',
u'contentType': u'application/octet-stream',
u'crc32c': u'XIEyEw==',
u'etag': u'CJCckonZ4c8CEAE=',
u'generation': u'1476702385770000',
u'id': u'tutsplus-demo-test/gcs_buckets.py/1476702385770000',
u'kind': u'storage#object',
u'md5Hash': u'+bd6Ula+mG4bRXReSnvFew==',
u'mediaLink': u'https://www.googleapis.com/download/storage/v1/b/tutsplus-demo-test/o/gcs_buckets.py?generation=147670238577000
0&alt=media',
u'metageneration': u'1',
u'name': u'gcs_buckets.py',
u'selfLink': u'https://www.googleapis.com/storage/v1/b/tutsplus-demo-test/o/gcs_buckets.py',
u'size': u'2226',
u'storageClass': u'STANDARD',
u'timeCreated': u'2016-10-17T11:06:25.753Z',
u'updated': u'2016-10-17T11:06:25.753Z'}
$ python gcs_objects.py list tutsplus-demo-test
[{u'contentType': u'application/octet-stream',
u'name': u'gcs_buckets.py',
u'size': u'2226'},
{u'contentType': u'application/x-www-form-urlencoded;charset=UTF-8',
u'name': u'tutsplus/',
u'size': u'0'},
{u'contentType': u'image/png',
u'name': u'tutsplus/Screen Shot 2016-10-17 at 1.03.16 PM.png',
u'size': u'36680'}]
$ python gcs_objects.py delete tutsplus-demo-test gcs_buckets.py
''
$ python gcs_objects.py list tutsplus-demo-test
[{u'contentType': u'application/x-www-form-urlencoded;charset=UTF-8',
u'name': u'tutsplus/',
u'size': u'0'},
{u'contentType': u'image/png',
u'name': u'tutsplus/Screen Shot 2016-10-17 at 1.03.16 PM.png',
u'size': u'36680'}]
结论
在本教程系列中,我们从鸟瞰的角度了解了Google Cloud Storage的工作原理,然后对存储桶和对象进行了深入分析。 然后,我们看到了如何通过Google Cloud Console执行与存储桶和对象相关的主要操作。
然后,我们使用Python脚本执行了相同的操作。 Google Cloud Storage可以完成更多工作,但您可以自己探索。
翻译自: https://code.tutsplus.com/tutorials/google-cloud-storage-managing-files-and-objects--cms-27460