Google Cloud Storage(GCS)是Google提供的非常简单和强大的对象存储产品,是其Google Cloud Platform (GCP)的一部分 。 它为开发人员提供了高度耐用,可扩展,一致且可用的存储解决方案,并且与Google用于支持其自己的对象存储的技术相同。
您可以为使用的模型付费 ,并且GCP拥有60天的试用期,因此可以免费试用一下它是否适合您的组织需求。 GCS具有不同的服务级别(也称为存储类),可以根据需要选择它们(关于这些的详细讨论不在本教程的讨论范围内)。 GCS可以用于多种目的,例如提供静态/动态网站内容,存储特定于用户的应用程序文件,灾难恢复或使大数据对象可下载给用户。
那些从事过GCP工作的人会知道,GCP中的一切都围绕着项目展开。 每个项目可以有多个存储桶,围绕它们构建Google Cloud Storage的架构。 值区是GCS上包含已存储数据的基本容器。 这些用作组织数据的基本块,看起来像操作系统上的文件夹,但不能嵌套。
每个存储桶可以包含任意数量的对象,可以是文件夹和/或文件。 创建存储桶时,会为其分配存储类别和地理位置。 这些设置可以在创建存储桶时指定,但以后不能更改。
存储桶具有特定的命名约定 ,必须严格遵守这些约定 ,否则GCP将不允许您创建存储桶。 存储桶名称在全球范围内是唯一的,因此需要以防止冲突的方式进行选择。 但是,删除的存储桶使用的名称可以重复使用。
此外,将名称分配给存储桶后就无法更改。 如果要更改它,唯一的解决方案是使用所需名称创建一个新存储桶,将内容从前一个存储桶移动到新存储桶,然后删除前一个存储桶。
在本教程中,我将介绍如何从Google Cloud Console管理存储桶。 接下来是Python脚本,我将在此演示如何以编程方式执行相同的操作。
使用Google Cloud Console
首先,让我们看看如何使用GCP提供的Web用户界面(称为Google Cloud Console)来管理存储桶。
在您选择的Web浏览器中打开Storage Browser 。 如果您是首次用户,系统将提示您首先创建一个项目。 另外,将显示一个选项来注册免费试用。 继续免费试用注册,否则您将无法自己创建新存储桶。 默认情况下,GCP每个App Engine实例仅提供一个免费存储区。
完成所有这些正式过程后,导航至此页面应打开下面显示的页面。
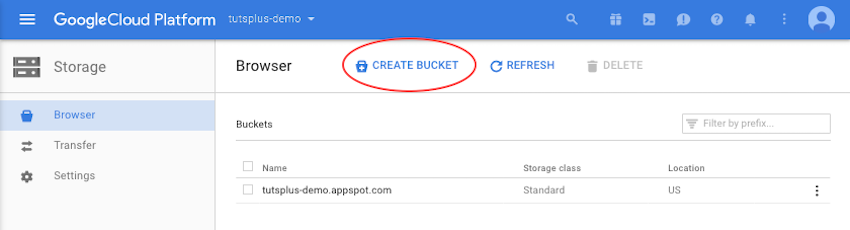
要创建新存储桶,请单击上方突出显示的“ 创建存储桶”按钮。 通过填写所需的名称来创建存储桶,如下所示。 名称应遵循存储桶命名约定 。
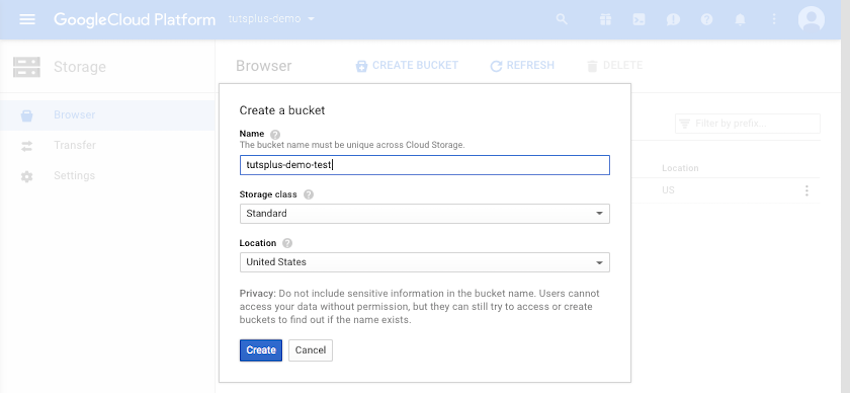
创建存储桶后,GCS浏览器将列出该存储桶。 可以通过从列表中选择存储桶并单击删除按钮来删除存储桶。
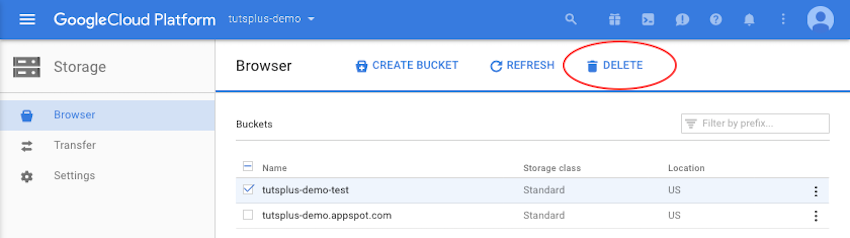
单击刷新按钮将使用对存储桶列表的任何更改填充UI,而不会刷新整个页面。
以编程方式管理存储桶
首先,让我们创建一个Google Compute Engine实例,因为它可以快速演示目标概念,而不是在本地计算机上处理额外的身份验证步骤。 要创建GCE实例,请打开链接 ,然后单击“ 创建实例”按钮,如下所示。
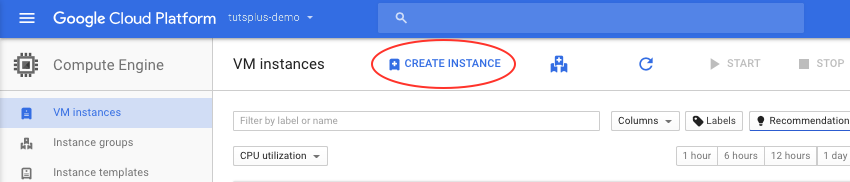
将会出现一个表格,询问相关细节,可以在您方便时填写。 创建GCE实例后,如下所示打开SSH客户端,默认情况下,它将在新的浏览器窗口中打开。

SSH客户端屏幕如下所示。 本教程中的所有其他操作将直接在SSH客户端本身上完成。

编写Python脚本
以下是为Python开发环境设置新创建的服务器所需运行的命令。
$ sudo apt-get update
$ sudo apt-get install python-dev python-setuptools
$ sudo easy_install pip
下面是编写此脚本需要安装的依赖项。
$ sudo pip install google-api-python-client
在生产系统上,建议不要使用“ sudo”安装库。 为此,请遵循Python virtualenv最佳做法。
gcs_bucket.py
import sys
from pprint import pprint
from googleapiclient import discovery
from googleapiclient import http
from oauth2client.client import GoogleCredentials
def create_service():
credentials = GoogleCredentials.get_application_default()
return discovery.build('storage', 'v1', credentials=credentials)
def list_buckets(project):
service = create_service()
res = service.buckets().list(project=project).execute()
pprint(res)
def create_bucket(project, bucket_name):
service = create_service()
res = service.buckets().insert(
project=project, body={
"name": bucket_name
}
).execute()
pprint(res)
def delete_bucket(bucket_name):
service = create_service()
res = service.buckets().delete(bucket=bucket_name).execute()
pprint(res)
def get_bucket(bucket_name):
service = create_service()
res = service.buckets().get(bucket=bucket_name).execute()
pprint(res)
def print_help():
print """Usage: python gcs_bucket.py <command>
Command can be:
help: Prints this help
list: Lists all the buckets in specified project
create: Create the provided bucket name in specified project
delete: Delete the provided bucket name
get: Get details of the provided bucket name
"""
if __name__ == "__main__":
if len(sys.argv) < 2 or sys.argv[1] == "help" or \
sys.argv[1] not in ['list', 'create', 'delete', 'get']:
print_help()
sys.exit()
if sys.argv[1] == 'list':
if len(sys.argv) == 3:
list_buckets(sys.argv[2])
sys.exit()
else:
print_help()
sys.exit()
if sys.argv[1] == 'create':
if len(sys.argv) == 4:
create_bucket(sys.argv[2], sys.argv[3])
sys.exit()
else:
print_help()
sys.exit()
if sys.argv[1] == 'delete':
if len(sys.argv) == 3:
delete_bucket(sys.argv[2])
sys.exit()
else:
print_help()
sys.exit()
if sys.argv[1] == 'get':
if len(sys.argv) == 3:
get_bucket(sys.argv[2])
sys.exit()
else:
print_help()
sys.exit()
上面的Python脚本演示了可以在存储桶上执行的主要操作。 这些包括:
- 在项目中创建新存储桶
- 列出项目中的所有存储桶
- 获取特定存储桶的详细信息
- 删除特定的存储桶
让我们看看运行脚本时这些操作的样子。
$ python gcs_bucket.py
Usage: python gcs_bucket.py <command>
Command can be:
help: Prints this help
list: Lists all the buckets in specified project
create: Create the provided bucket name in specified project
delete: Delete the provided bucket name
get: Get details of the provided bucket name
$ python gcs_bucket.py list tutsplus-demo
{u'items': [{u'etag': u'CAE=',
u'id': u'tutsplus-demo.appspot.com',
u'kind': u'storage#bucket',
u'location': u'US',
u'metageneration': u'1',
u'name': u'tutsplus-demo.appspot.com',
u'projectNumber': u'1234567890',
u'selfLink': u'https://www.googleapis.com/storage/v1/b/tutsplus-demo.appspot.com',
u'storageClass': u'STANDARD',
u'timeCreated': u'2016-10-05T15:30:52.237Z',
u'updated': u'2016-10-05T15:30:52.237Z'}],
u'kind': u'storage#buckets'}
$ python gcs_bucket.py create tutsplus-demo tutsplus-demo-test
{u'etag': u'CAE=',
u'id': u'tutsplus-demo-test',
u'kind': u'storage#bucket',
u'location': u'US',
u'metageneration': u'1',
u'name': u'tutsplus-demo-test',
u'projectNumber': u'1234567890',
u'selfLink': u'https://www.googleapis.com/storage/v1/b/tutsplus-demo-test',
u'storageClass': u'STANDARD',
u'timeCreated': u'2016-10-07T05:55:29.638Z',
u'updated': u'2016-10-07T05:55:29.638Z'}
$ python gcs_bucket.py get tutsplus-demo-test
{u'etag': u'CAE=',
u'id': u'tutsplus-demo-test',
u'kind': u'storage#bucket',
u'location': u'US',
u'metageneration': u'1',
u'name': u'tutsplus-demo-test',
u'projectNumber': u'1234567890',
u'selfLink': u'https://www.googleapis.com/storage/v1/b/tutsplus-demo-test',
u'storageClass': u'STANDARD',
u'timeCreated': u'2016-10-07T05:55:29.638Z',
u'updated': u'2016-10-07T05:55:29.638Z'}
$ python gcs_bucket.py delete tutsplus-demo-test
''
结论
在本教程中,您了解了如何在Google Cloud Storage上管理存储桶。 同时还附带了有关创建Google Compute Engine实例并通过SSH客户端使用它的小介绍。
在下一个教程中,我将介绍如何管理对象,即存储桶中的文件夹和文件。
翻译自: https://code.tutsplus.com/tutorials/google-cloud-storage-managing-buckets--cms-24238