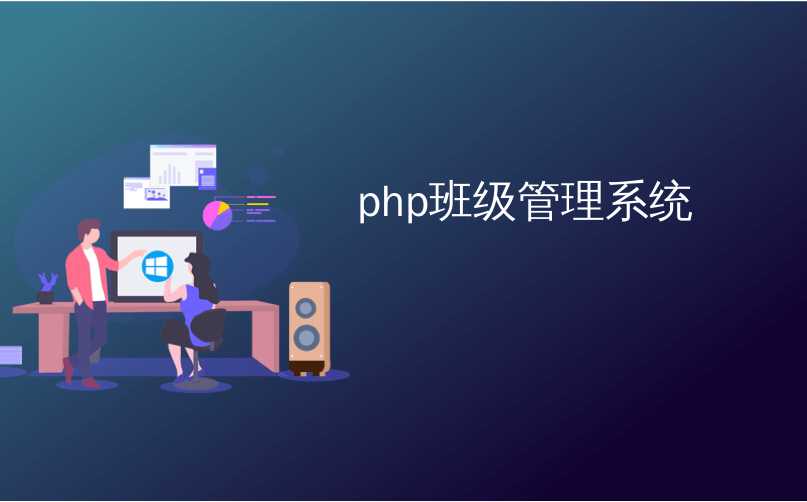
php班级管理系统
[rps-include post=6522]
[rps-include post = 6522]
Php programming language supports most of the Object Oriented Programming concepts. Object Oriented Programming provides a lot of useful features to manage big and enterprise applications.
PHP编程语言支持大多数面向对象的编程概念。 面向对象编程提供了许多有用的功能来管理大型和企业应用程序。
面向对象编程 (Object Oriented Programming)
Object Oriented Programming or OOP is a concept that provides different features to implement real world problems. Up to now we have used variables to write Php applications but as you know enterprise applications can not work with those simple types.
面向对象编程或OOP是一个概念,提供了用于实现实际问题的不同功能。 到目前为止,我们已经使用变量来编写Php应用程序,但是您知道企业应用程序无法使用这些简单类型。
For example we may need to define a student in our app. What kind of properties or actions may have a student in real life. Here an example list;
例如,我们可能需要在我们的应用中定义一个学生。 现实生活中可能会有什么样的行为或行为让学生学习。 这里是一个示例列表;
- Name 名称
- Surname姓
- Age年龄
- Class类
- Parents父母
- ……
- Select Class 选择班级
- Finish School毕业
- Add Missed Days添加错过的日子
- ……
We can resume the list but these are enough. Using only primitive data types like string, integer etc. we can not cope all of these things. Assume that we have 1000 students in school. Should we create separate variables for each students name? No. Solution is Object Oriented Programming and Class
我们可以恢复列表,但是这些就足够了。 仅使用原始数据类型(例如字符串,整数等),我们无法应对所有这些情况。 假设我们在学校有1000名学生。 我们应该为每个学生的名字创建单独的变量吗? 否。解决方案是面向对象的编程和类
定义类别(Define Class)
Class is used to specify composite types. We can use different type of variables and methods in a class. Class makes them easy to access and use. Classes can be used for other class definitions which makes complex class inheritance an easy job. We can create a class with class
keyword and curly brackets {}
which is class body. Here is the syntax
类用于指定复合类型。 我们可以在一个类中使用不同类型的变量和方法。 类使它们易于访问和使用。 类可用于其他类定义,这使复杂的类继承变得容易。 我们可以创建一个带有class
关键字和大括号{}
的类,它是类主体。 这是语法
class NAME {
BODY
}
In this example we will create a class named Student
which have some properties and methods.
在此示例中,我们将创建一个名为Student
的类,该类具有一些属性和方法。
class Student{
public $name="";
public function SayName(){
echo $this->name;
}
}
Just concentrate the class definition and how its body look. We will learn properties and methods in next steps.
只需集中讨论类定义及其主体外观即可。 我们将在下一步中学习属性和方法。
创建对象 (Create Object)
We have defined a class in previous step but how can we use this class. Classes can not be used directly. Because classes only defines the structure and in most situations do not hold data. We should initialize the class by creating object from it with new
keyword. Objects holds given data according to their class definitions.
我们在上一步中定义了一个类,但是如何使用该类。 类不能直接使用。 因为类仅定义结构,并且在大多数情况下不保存数据。 我们应该通过使用new
关键字从类创建对象来初始化类。 对象根据其类定义保存给定的数据。
In this example we will use Student
class to create an object named john
. john
will represent the methods and attributes of Student
class.
在此示例中,我们将使用Student
类创建一个名为john
的对象。 john
将代表Student
类的方法和属性。
class Student{
public $name="";
public function SayName(){
echo $this->name;
}
}
$john = new Student;
类属性 (Class Properties)
Properties are used to store some data . Another usage of properties are access to data from object directly. Properties types are primitive types like integer, string, float, boolean etc.
属性用于存储一些数据。 属性的另一种用法是直接从对象访问数据。 属性类型是基本类型,例如整数,字符串,浮点型,布尔型等。
In this example we will add Student
class surname, number and age properties.
在此示例中,我们将添加Student
班的姓氏,数字和年龄属性。
class Student{
public $name="";
public $surname="";
public $number="";
public $age=-1;
public function SayName(){
echo $this->name;
}
}
访问类内部的类属性 (Access Class Properties Inside Class)
We generally need to access class properties inside class. We can use $this
keyword in order to access. We will also use ->
as delimiter between $this
and property name. In the function named SayName
we used $this->
to access name
property of the class.
我们通常需要访问类内部的类属性。 我们可以使用$this
关键字来访问。 我们还将使用->
作为$this
和属性名称之间的分隔符。 在名为SayName
的函数中,我们使用$this->
访问该类的name
属性。
class Student{
public $name="";
public $surname="";
public $number="";
public $age=-1;
public function SayName(){
echo $this->name;
}
}
访问类属性 (Access Class Properties)
We can access class properties with the object and property name. We will use ->
to address property name.
我们可以使用对象和属性名称访问类属性。 我们将使用->
地址属性名称。
In this example we will access the $name
and $surname
properties of the $john
object.
在此示例中,我们将访问$john
对象的$name
和$surname
属性。
class Student{
public $name="";
public $surname="";
public $number="";
public $age=-1;
public function SayName(){
echo $this->name;
}
}
$john = new Student();
echo $john->name." "$john->surname;
类方法 (Class Methods)
One of the powerful feature of the class and object is methods. We can define methods in class and call them over objects. We can easily access object properties in these methods too.
方法是类和对象的强大功能之一。 我们可以在类中定义方法并通过对象调用它们。 我们也可以通过这些方法轻松访问对象属性。
In this example we will create a function named PrintStudent()
which will print name and surname student informations;
在本例中,我们将创建一个名为PrintStudent()
的函数,该函数将打印姓名和姓氏的学生信息。
class Student{
public $name="";
public $surname="";
public $number="";
public $age=-1;
public function SayName(){
echo $this->name;
}
public function PrintStudent(){
echo $this->name;
echo $this->surname;
}
}
$john = new Student();
$john->PrintStudent();
[rps-include post=6522]
[rps-include post = 6522]
php班级管理系统