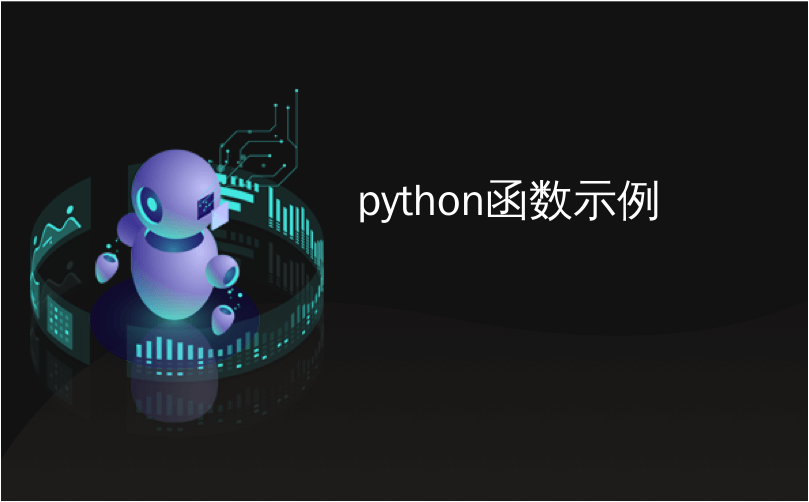
python函数示例
Python programming language provides range()
function in order to create a sequence of numbers in a different start number, increments, etc. range()
function provides lightweight creation of sequences during run-time which makes this type of execution faster.
Python编程语言提供了range()
函数,以便以不同的起始数字,增量等方式创建数字序列。range range()
函数在运行时提供轻量级的序列创建,从而使这种类型的执行速度更快。
语法和参数 (Syntax and Parameters)
range() function has the following syntax where 3 parameters can be accepted but not all of them are mandatory. Only the STOP parameter is mandatory.
range()函数具有以下语法,其中可以接受3个参数,但并非所有参数都是强制性的。 仅STOP参数是必需的。
range(START,STOP,STEP)
START
is used to specify the start number of the sequence. This number can be a positive or negative value like 4, -8, etc.START
用于指定序列的开始编号。 该数字可以是正数或负数,例如4,-8等。STOP
specifies the number we want to end the sequence. STOP is a must for range() function usage.STOP
指定我们要结束序列的数字。 STOP是range()函数用法的必需项。STEP
is used to specify the increment value. If the step is not specified the default value will be 1. We can also specify the STEP negative in order to decrement from the given START number to the STOP number.STEP
用于指定增量值。 如果未指定步长,则默认值为1。我们也可以指定STEP负数,以便从给定的START编号减少到STOP编号。
创建1到10的简单序列 (Create Simple Sequence From 1 to 10)
We will start using range()
function in order to create a simple sequence. We will just specify the START and STOP. We will start at 1 and increment to the 10 with one by one.
我们将开始使用range()
函数来创建一个简单的序列。 我们将仅指定START和STOP。 我们将从1开始并一一递增到10。
myrange=range(1,10)
print(list(myrange))

创建递增2的序列(Create Sequence Which Increments 2)
In the previous example, we will use 1 as an increment value. We can specify the increment value explicit the value we want. We will just add the increment value as the 3rd parameter to the range() function. In this example, we will start at 0 and count to 10 by incrementing 2.
在前面的示例中,我们将使用1作为增量值。 我们可以指定增量值,明确指定所需的值。 我们只将增量值作为第三个参数添加到range()函数。 在此示例中,我们将从0开始,并通过递增2计数到10。
myrange=range(0,10,2)
print(list(myrange))

从range()创建列表(Create List From range())
As stated previously range()
function is computed during executions of the code which means if we do not run range()
and set to a different type like list it will be just a text. Let’s look below code which is just printed the range(0,10)
not the sequence.
如前所述, range()
函数是在代码执行期间计算的,这意味着如果我们不运行range()
并将其设置为其他类型(如list),它将只是一个文本。 让我们看下面的代码,它只是打印range(0,10)
而不是序列。
myrange=range(1,10)
print(myrange)

So we need to create new data structures from the range()
function. range() function will literally create a list where we can use the created sequence as a list like below.
因此,我们需要从range()
函数创建新的数据结构。 range()函数会从字面上创建一个列表,在这里我们可以将创建的序列用作如下所示的列表。
myrange=range(1,10)
print(list(myrange))

使用range()函数迭代或循环(Iterate or Loop with range() Function)
range() function creates sequences and these sequences generally used to loop or iterate. We can use range() function inside a loop statement like for
, while
etc to iterate over elements of the sequence. In this example, we will iterate from 1 to the 20 with range function in for a loop.
range()函数创建序列,这些序列通常用于循环或迭代。 我们可以在for
, while
等循环语句中使用range()函数来迭代序列中的元素。 在此示例中,我们将使用范围函数in从1迭代到20。
for i in range(1,20):
print(i)
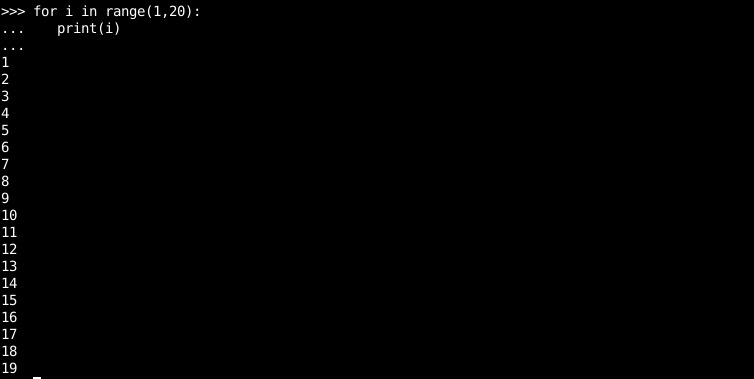
range()的默认起始编号(Default Start Number Of range() Function)
As stated in the syntax part only STOP number is must in range() function. So we do not have to provide the start number to the range() function. The default start number is 0. In this example, we will use the default start number and only specify the end number as 20.
如语法部分中所述,在range()函数中必须只有STOP号。 因此,我们不必向range()函数提供起始号。 默认起始编号为0。在本示例中,我们将使用默认起始编号,仅将终止编号指定为20。
for i in range(20):
print(i)
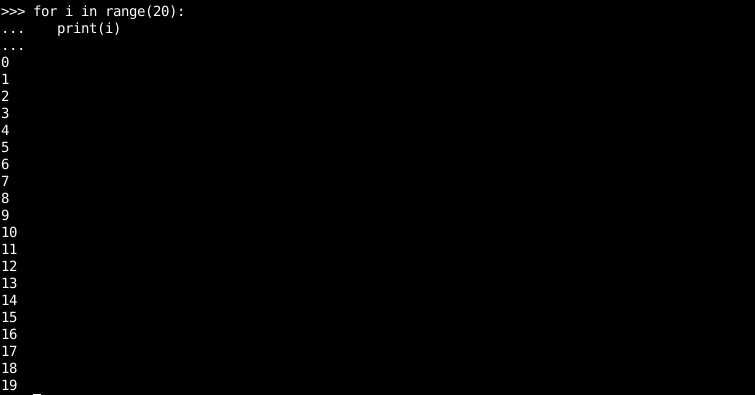
创建仅序列设置结束号(Create Sequence Only Setting End Number)
We can create a sequence with the range() function by only setting the stop or end number. In this example, we will start by default start number which 0 and increment to the 20.
我们可以通过设置range()函数来创建一个序列,只需设置终止号或终止号即可。 在此示例中,我们将默认以0开始并以20为增量开始。
for i in range(20):
print(i)
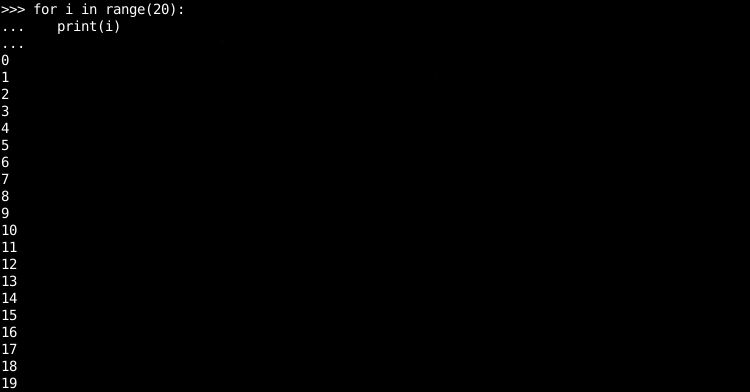
创建向后顺序或负向步骤(Create Backward Sequence or Negative Step)
Up to now, we have look how to create sequences with forwarding or positive steps. range() function also supports negative steps where the sequence will start from start number and take negative steps or decrement to the stop number. In this example, we will start from -2 and decrement to the -10 one by one. We have to also specify the decrement value which is -1
到目前为止,我们已经了解了如何使用转发步骤或积极步骤来创建序列。 range()函数还支持负数步,其中序列将从起始号开始,并采用负数步或递减到终止号。 在此示例中,我们将从-2开始,然后一个一递减到-10。 我们还必须指定减量值为-1
for i in range(-2,-10,-1):
print(i)
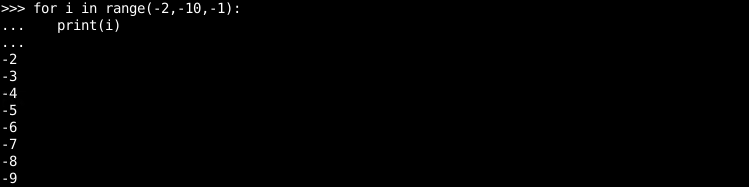
range()与xrange()(range() vs xrange())
range() is a function provided in Python3 but in Python version 2 xrange() was used popularly. xrange() function is eliminated in Python3 so we can not use it. range() function also provides very efficient executaion to the xrange() function.
range()是Python3中提供的一个函数,但在Python 2版本中,xrange()被广泛使用。 xrange()函数已在Python3中删除,因此我们无法使用它。 range()函数还为xrange()函数提供了非常有效的执行。
翻译自: https://www.poftut.com/python-range-function-tutorial-with-examples/
python函数示例