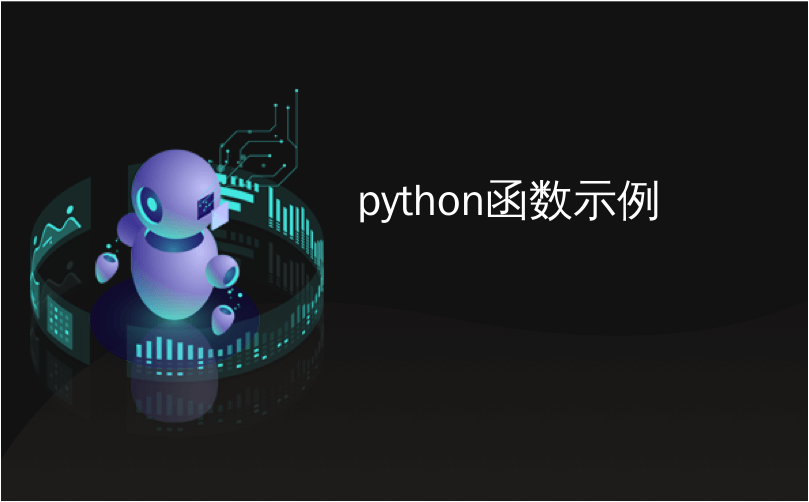
python函数示例
Python provides different useful functions in order to help developers. reduce()
function is one of them where it is used to evaluate given list items with the provided function.
Python提供了各种有用的功能来帮助开发人员。 reduce()
函数是其中之一,用于通过提供的函数评估给定的列表项。
reduce()函数语法 (reduce() Function Syntax)
reduce() function use the following syntax.
reduce()函数使用以下语法。
reduce(FUNCTION, SEQUENCE, INITIAL)
- `reduce` is the name reduce function 减少是名称减少功能
- `FUNCTION`is the function name we want to use for evaluation of the SEQUENCE items.“功能”是我们要用于评估SEQUENCE项目的功能名称。
- `SEQUENCE` is the list that contains multiple items that are processed or evaluated by the FUNCTION. SEQUENCE是包含由FUNCTION处理或评估的多个项目的列表。
- `INITIAL` can be set as the first argument to the FUNCTION but INITIAL is optional and generally not used. 可以将“ INITIAL”设置为FUNCTION的第一个参数,但是INITIAL是可选的,通常不使用。
导入functools模块/库 (Import functools Module/Library)
In order to use the reduce() function we need to provide the module which provides it. functools
module provides the reduce() function so we will import functools like below.
为了使用reduce()函数,我们需要提供提供它的模块。 functools
模块提供了reduce()函数,因此我们将如下所示导入functools。
import functools
reduce()函数示例 (reduce() Function Example)
We will use the following example to explain the reduce() function. We will first import the reduce() function from the functools module. Then create a list of which name is numbers
and contains numbers. Then we will create a function that will be used inside the reduce() with the name of my_sum()
. Then we will call the reduce() function by providing the function name my_sum() and the list named numbers.
我们将使用以下示例来说明reduce()函数。 我们将首先从functools模块导入reduce()函数。 然后创建名称为numbers
并包含数字的列表。 然后,我们将创建一个将在reduce()内部使用的函数,其名称为my_sum()
。 然后,我们将通过提供函数名称my_sum()和名为number的列表来调用reduce()函数。
from functools import reduce
numbers = [ 1 , 2 , 3 , 4 , 5 ]
def my_sum(a,b):
return a+b
result = reduce(my_sum,numbers)
print(result)
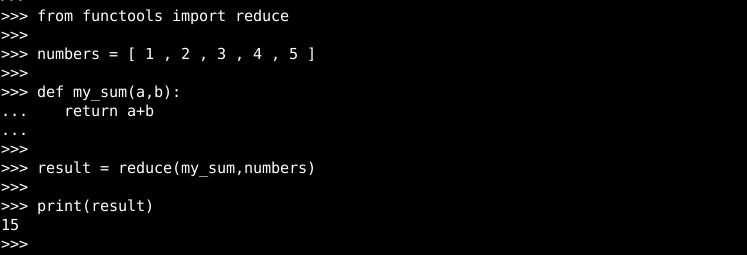
- For the first time, the first and second elements of the numbers list will be provided to the my_sum() function.首次将数字列表的第一和第二个元素提供给my_sum()函数。
- As my_sum() function sum the given arguments which are 1 and 3 the function will return 3. 当my_sum()函数将给定参数1和3相加时,该函数将返回3。
- Now the 3 and next which is third in the list number 3 will be provided to the my_sum() function which will sum them and return 6 现在,将在列表编号3中排在第三位的3和下一个提供给my_sum()函数,该函数将它们求和并返回6
- Now we have 6 and the fourth element of the numbers list which is 4 will be provided to the my_sum() function. 现在我们有了6,数字列表的第四个元素4将被提供给my_sum()函数。
- … this will continue to every item in the list are evaluated with the given function and the last value will be set to the `result` variable. …这将继续使用给定功能对列表中的每个项目进行评估,并且最后一个值将设置为`result`变量。
带初始值的reduce()示例 (reduce() Example with Initial Value)
reduce() function generally used without an initial value which is described in the syntax part. But in some cases using initial value may be useful even necessary. by using initial value the first element will be the initial value and the provided list first element will be the second parameter.
reduce()函数通常不使用语法部分中描述的初始值。 但是在某些情况下,甚至有必要使用初始值也是有用的。 通过使用初始值,第一个元素将是初始值,提供的列表第一个元素将是第二个参数。
from functools import reduce
numbers = [ 1 , 2 , 3 , 4 , 5 ]
def my_sum(a,b):
return a+b
result = reduce(my_sum,numbers,7)
print(result)
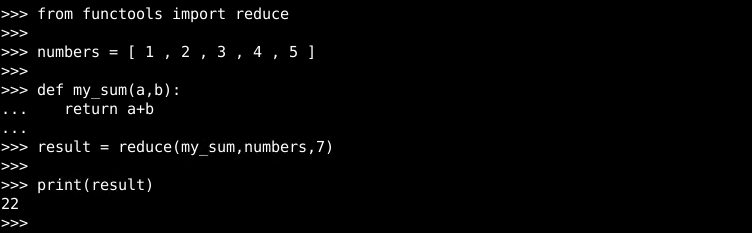
- 7 is provided as the initial value and 1 is the first element of the numbers list. 7 and 1 will be provided to the function my_sum() as parameters and the result will be 8. 提供7作为初始值,而1是数字列表的第一个元素。 将向函数my_sum()提供7和1作为参数,结果为8。
- In the second iteration, the 8 will be first and 2 will be the second argument to the function my_sum(). 在第二次迭代中,函数my_sum()的第一个参数为8,第二个为参数。
- … things will continue like normal reduce function. ……事情会像正常的reduce功能一样继续下去。
带操作符函数的reduce()函数 (reduce() Function with Operator Functions)
Python provides the operator function which is simply 4 basic calculations like add, multiply, etc. We can use these operator functions with the reduce() function which will increase the code readability. In this example, we will use the add() operator function. In order to use operator functions, we should import the operator module.
Python提供的运算符函数只是4个基本计算,如加,乘等。我们可以将这些运算符函数与reduce()函数一起使用,这将增加代码的可读性。 在此示例中,我们将使用add()运算符函数。 为了使用操作员功能,我们应该导入操作员模块。
from functools import reduce
from operator import add
numbers = [ 1 , 2 , 3 , 4 , 5 ]
result = reduce(add,numbers)
print(result)
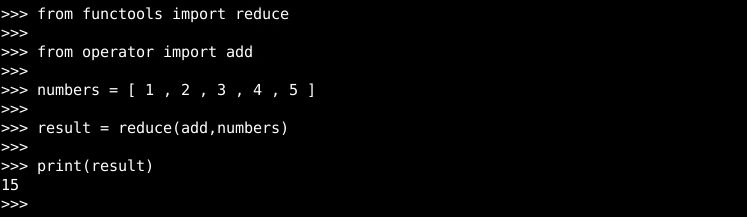
We can see that we just provided the add()
function.
我们可以看到我们只提供了add()
函数。
带Lambda函数的reduce() (reduce() with Lambda Function)
Lambda function is used to create anonymous and inline functions without defining them explicitly. We can use reduce() and lambda functions together which will be more readable then explicit function definition. In this example, we will create a lambda function which will sum given list items.
Lambda函数用于创建匿名和内联函数,而无需显式定义它们。 我们可以一起使用reduce()和lambda函数,它们将比显式函数定义更具可读性。 在此示例中,我们将创建一个lambda函数,该函数将汇总给定的列表项。
from functools import reduce
numbers = [ 1 , 2 , 3 , 4 , 5 ]
result = reduce(lambda x,y: x+y ,numbers)
print(result)
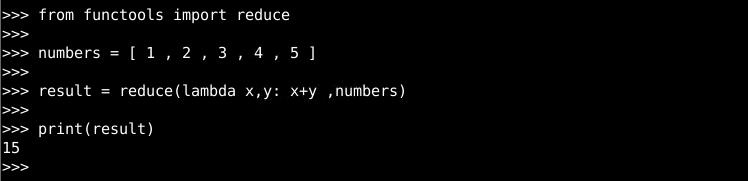
使用reduce()将列表转换为字符串 (Convert List To String with reduce())
Another use case for the reduce is providing a string list and joining all items into a single string like a sentence. In this example, we will provide the list named strlist
to the reduce() function. We will also use the lambda operation where we will join given string elements together.
reduce的另一个用例是提供一个字符串列表,并将所有项目连接到单个字符串(如句子)中。 在此示例中,我们将为strlist
()函数提供名为strlist
的列表。 我们还将使用lambda操作,将给定的字符串元素连接在一起。
from functools import reduce
from operator import add
strlist=['I ', 'love ', 'poftut.com']
result = reduce(lambda x,y: x+y ,strlist)
print(result)
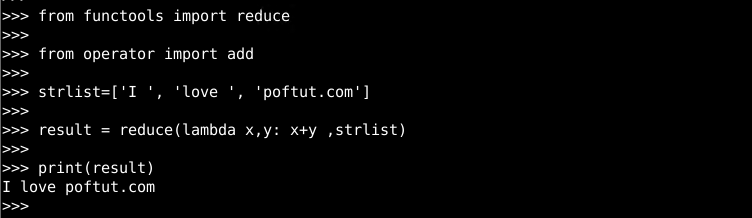
翻译自: https://www.poftut.com/python-reduce-function-tutorial-with-examples/
python函数示例