Hello World
is a popular term used in programming courses. Hello World
means a new start for a programming language or applications. It is used as a salute from the first application to its developer and the world like a newborn baby.
Hello World
是编程课程中常用的术语。 Hello World
意味着编程语言或应用程序的新起点。 从首次应用到开发人员,乃至全世界,它都像婴儿一样被用作礼炮。
世界历史你好 (Hello World History)
Before starting to define and create a hello world we need to learn the history start of the hello world. The c programming language is created in order to develop a popular operating system named Unix. Brian Kernighan the creator of the C published a reference book named C Programming Language
in order to describe and reference the C programming language in 1973. The introduction and first example was a simple C program that simply outputs the text “Hello World” to the standard output or screen. The example hello world code was like below.
在开始定义和创建问候世界之前,我们需要学习问候世界的历史起点。 创建c编程语言是为了开发名为Unix的流行操作系统。 C的创建者Brian Kernighan于1973年出版了一本名为C Programming Language
参考书,以描述和引用C编程语言。引言和第一个示例是一个简单的C程序,该程序将文本“ Hello World”简单地输出到标准输出或屏幕。 你好世界代码示例如下所示。
main( ) {
extrn a, b, c;
putchar(a); putchar(b); putchar(c); putchar(’!*n’);
} 1 ’hell’;
b ’o, w’;
c ’orld’;
This clever introduction example and text is used by other authors and programming languages later which make the “Hello World” so much popular. “Hello World” is repeated over and over again by new programming languages even in 2020 after 47 years later.
这个聪明的介绍示例和文本后来被其他作者和编程语言使用,这使得“ Hello World”如此流行。 47年后的2020年,新的编程语言不断地重复“ Hello World”。
简单的Hello World程序源代码 (Simple Hello World Program Source Code)
Below we can see a simple HelloWorld applications source code. In general, these applications will print “Hello World” to the standard output which is generally the console or command-line interface.
下面我们可以看到一个简单的HelloWorld应用程序源代码。 通常,这些应用程序会将“ Hello World”打印到标准输出,通常是控制台或命令行界面。
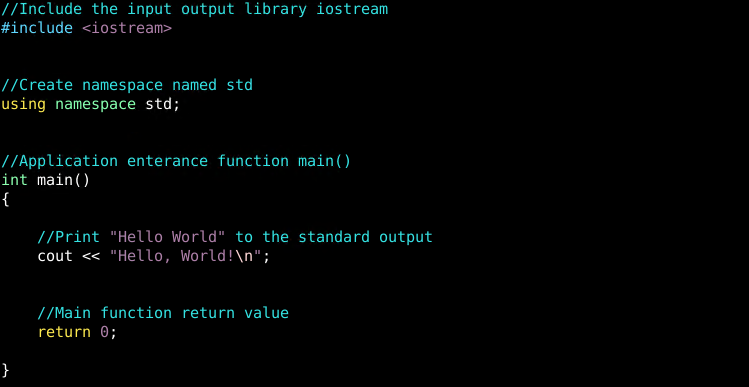
//Include the input output library iostream
#include <iostream>
//Create namespace named std
using namespace std;
//Application enterance function main()
int main()
{
//Print "Hello World" to the standard output
cout << "Hello, World!\n";
//Main function return value
return 0;
}
Let’s explain the given code step by step.
让我们逐步解释给定的代码。
//
lines are comments which are not executed. They are just comments and explanations.//
行是未执行的注释。 它们只是评论和解释。#include <iostream>
is used to import and include input and output libraries which providescout
andcin
.#include
is a C++ directive that will import or include a given library.<iostream>
is a library that provides functions to read and write from standard input and output.#include <iostream>
用于导入和包含提供cout
和cin
输入和输出库。#include
是将导入或包含给定库的C ++指令。<iostream>
是一个提供从标准输入和输出读取和写入功能的库。using namespace std;
is used to create and set namespace. A namespace is used to create a block of code that will be effective in the current source code page.using namespace std;
用于创建和设置名称空间。 名称空间用于创建将在当前源代码页面中生效的代码块。int main()
is a method definition but a special one. main() function is a special name where it is used to create a starting point for the application or executable file.{
and}
are used to specify the start and end of the main function block. All codes related to the main function will be stored inside these curly braces.int main()
是一种方法定义,但是是一个特殊的定义。 main()函数是一个特殊名称,用于为应用程序或可执行文件创建起点。{
和}
用于指定主功能块的开始和结束。 与主要功能有关的所有代码都将存储在这些花括号内。The most magical part is
cout << "Hello World!\n;"
which will print “Hello World” to the standard output and\n
will be used to set the end of the line and put cursor to the next line.最神奇的部分是
cout << "Hello World!\n;"
它将打印“ Hello World”到标准输出,并且\n
将用于设置行的结尾并将光标移至下一行。return 0;
will return the main function with value 0. Actually, this is a standard function convention that generally has no meaning with the special main function.return 0;
将返回值为0的main函数。实际上,这是标准函数约定,通常对于特殊main函数没有任何意义。
输入和输出的Hello World示例(Hello World Example with Input and Output)
The hello world example can be extended with some input from the user. We will use the cin
keyword which will read data from the standard user input and out the data to the given variable.
hello world示例可以通过用户的一些输入进行扩展。 我们将使用cin
关键字,它将从标准用户输入中读取数据并将数据输出到给定的变量中。
//Include the input output library iostream
#include <iostream>
//Create namespace named std
using namespace std;
//Application enterance function main()
int main()
{
//Print "Hello World" to the standard output
cout << "Hello, World!\n";
//Create a string variable name
string name;
//Read from standard input and put data into name variable
cin >> name;
//Print "Hello" with the name variable
cout << "Hello " << name <<"\n";
//Main function return value
return 0;
}
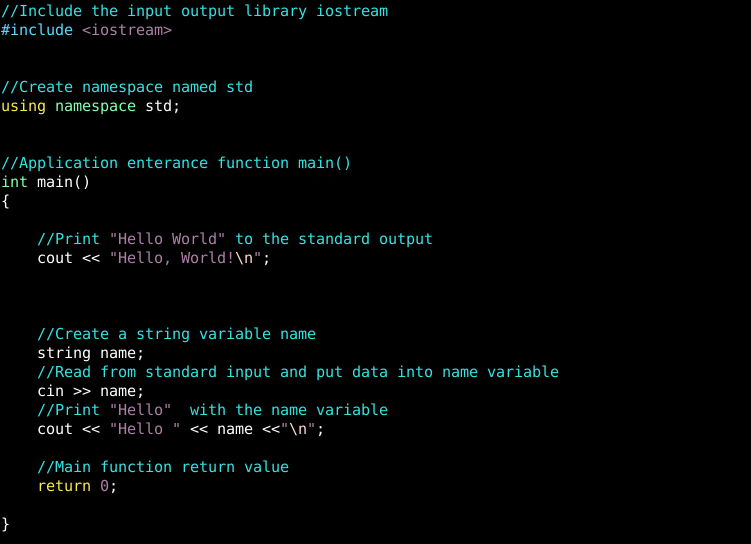
We will just talk about the differences from the previous example code.
我们将只讨论与先前示例代码的区别。
string name;
is used to create a variable as namedname
. We will store the user to input into the variable name.string name;
用于创建名为name
的变量。 我们将存储要输入的用户变量名。cin >> name;
will read from the standard input which is generally command-line interface and put the data into the variable namedname
.cin >> name;
将从标准输入(通常是命令行界面)中读取数据,并将数据放入名为name
的变量中。cout << "Hello" <<name << "\n";
will print theHello
to the standard output with thename
variable data."\n"
is used for the end of the line which will put cursor to the next line.cout << "Hello" <<name << "\n";
将使用name
变量数据将Hello
打印到标准输出。"\n"
用于该行的末尾,它将光标移至下一行。
将Hello World程序编译为可执行文件并运行 (Compile Hello World Program Into Executable and Run)
Just creating the source code will not create an application or executable file. We have to compile the given source code. There are different ways like using IDE or command-line tools. For the Linux system, we will use the g++
compiler. We will also provide the -o HelloWorld
option to set created executable name and the source code file HelloWorld.cpp
to the g++ compiler. The cpp
extension is used for C++ source files. It is not mandatory but useful for others to understand the file type.
仅创建源代码将不会创建应用程序或可执行文件。 我们必须编译给定的源代码。 有多种方法,例如使用IDE或命令行工具。 对于Linux系统,我们将使用g++
编译器。 我们还将提供-o HelloWorld
选项,以将创建的可执行文件名和源代码文件HelloWorld.cpp
设置为g ++编译器。 cpp
扩展名用于C ++源文件。 它不是强制性的,但对其他人了解文件类型很有用。
$ g++ -o HelloWorld HelloWorld.cpp
$ file HelloWorld
$ ls -lh HelloWorld
$ ./HelloWorld
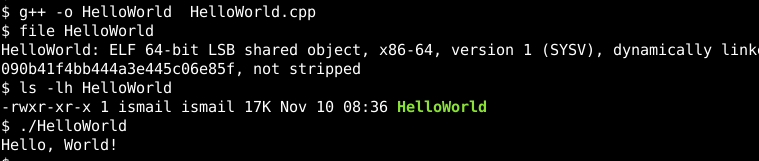