PHP programming language standard library supports the cURL library named libcurl
. libcurl is a library implementation of the cURL tool which is used to communicate with servers and clients by using popular protocols like HTTP, FTP, etc. cURL can be used for HTTP POST, HTTP PUT, FTP Upload requests.
PHP编程语言标准库支持名为libcurl
的cURL库。 libcurl是cURL工具的库实现,该工具用于通过使用流行的协议(例如HTTP,FTP等)与服务器和客户端进行通信。cURL可用于HTTP POST,HTTP PUT,FTP上载请求。
PHP cURL库 (PHP cURL Library)
PHP cURL library generally does not installed by default for most of the Linux distributions. In Windows, all libraries including PHP cURL is installed with the PHP. So we do not need to install an extra library for Windows operating systems. We can install PHP cURL library for deb based distributions like Ubuntu, Debian, Mint, Kali like below.
对于大多数Linux发行版,通常默认情况下未安装PHP cURL库。 在Windows中,所有库(包括PHP cURL)都随PHP一起安装。 因此,我们不需要为Windows操作系统安装额外的库。 我们可以为基于deb的发行版(如Ubuntu,Debian,Mint,Kali)安装PHP cURL库,如下所示。
$ sudo apt install php-curl
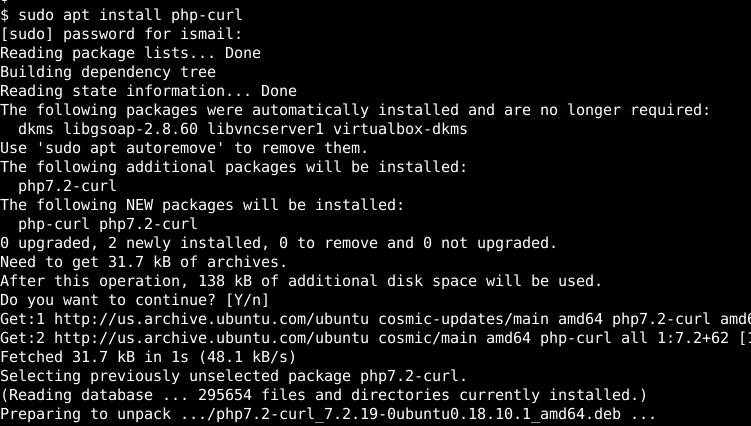
PHP cURL使用步骤(PHP cURL Usage Steps)
In order to use cURL in PHP, we have to follow the following steps that are required to initialize, configure, execute and close a cURL session. Of course, we will provide some parameters and arguments to these functions in order to set the cURL behavior.
为了在PHP中使用cURL,我们必须遵循初始化,配置,执行和关闭cURL会话所需的以下步骤。 当然,我们将为这些函数提供一些参数和参数,以设置cURL行为。
curl_init(); // initializes a cURL session
curl_setopt(); // changes the cURL session behavior with options
curl_exec(); // executes the started cURL session
curl_close(); // closes the cURL session and deletes the variable made by curl_init();
初始化cURL会话 (Initialize cURL Sessions)
The first is to use or create PHP cURL sessions is using the curl_init()
function which is used to initialize the cURL session. curl_init() function can be called without a parameter or with a URL parameter which will set the remote URL we can to use and communicate.
第一个是使用或创建PHP cURL会话,使用curl_init()
函数,该函数用于初始化cURL会话。 curl_init()函数可以不带参数或使用URL参数来调用,这将设置我们可以使用和通信的远程URL。
//Only create a cURL session and set to $ch variable
$ch = curl_init();
//Create a sessions variable named $ch
//and initialize it for the "http://www.poftut.com" URL
$ch = curl_init("http://www.poftut.com/");
设置cURL选项 (Set cURL Options)
Setting cURL options is not mandatory where if no option is set the default option configuration will be used. In order to set cURL sessions options, we will provide the cURL session variable we have already initialized and the option we want to set. There are a lot of options to set. We can set these options one by one executing the curl_setopt()
function.
设置cURL选项不是强制性的,如果未设置任何选项,则将使用默认选项配置。 为了设置cURL会话选项,我们将提供已经初始化的cURL会话变量和我们要设置的选项。 有很多设置选项。 我们可以一次执行curl_setopt()
函数来设置这些选项。
curl_setopt(SESSION_VARIABLE, OPTION, OPTION_VALUE);
- `SESSION_VARIABLE` is the variable that is initialized as a PHP cURL session. SESSION_VARIABLE是被初始化为PHP cURL会话的变量。
- `OPTION` is the option we want the set or change. OPTION是我们想要设置或更改的选项。
- `OPTION_VALUE` is the value for the specified option. OPTION_VALUE是指定选项的值。
$ch = curl_init();
//Set URL as "http://www.poftut.com"
curl_setopt($ch, CURLOPT_URL, "http://www.poftut.com/");
//Set HTTP Header
curl_setopt($ch, CURLOPT_HEADER, Array("Content-Type: text/xml"));
//Return Page Contents
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
执行cURL请求 (Execute cURL Request)
The third step is executing the cURL request or session with the curl_exec()
function where we will provide the cURL session variable as a parameter.
第三步是使用curl_exec()
函数执行cURL请求或会话,我们将提供cURL会话变量作为参数。
$ch = curl_init();
//Set URL as "http://www.poftut.com"
curl_setopt($ch, CURLOPT_URL, "http://www.poftut.com/");
//Execute curl with given options
curl_exec($ch);
关闭cURL会话 (Close cURL Session)
The last step for a PHP cURL request is closing the session and releasing the resources. curl_close()
is used to close and release all resources like memory, network, etc.
PHP cURL请求的最后一步是关闭会话并释放资源。 curl_close()
用于关闭和释放所有资源,例如内存,网络等。
//Initialize cURL session
$ch = curl_init();
//Set URL as "http://www.poftut.com"
curl_setopt($ch, CURLOPT_URL, "http://www.poftut.com/");
//Execute curl with given options
curl_exec($ch);
//Close the cURL session
curl_close($ch);
使用cURL获取URL (Get URL with cURL)
Now we have learned basics of the PHP cURL usage. We will start examining the different types of examples. First, we will make an HTTP GET request to the google.com and print the request-response to the standard output or command line.
现在,我们学习了PHP cURL用法的基础知识。 我们将开始研究不同类型的示例。 首先,我们将向google.com发出HTTP GET请求,并将请求响应打印到标准输出或命令行。
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'http://www.google.com');
curl_exec($ch);
curl_close($ch);
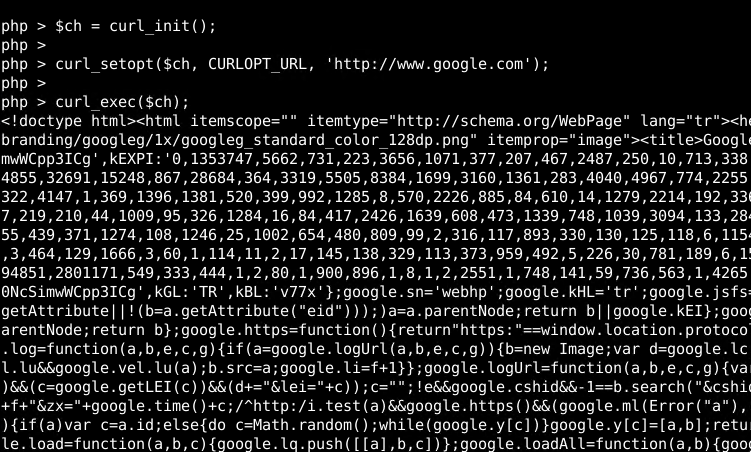
将页面内容返回到变量 (Return Page Contents To A Variable)
Alternatively, we can put the returned response from the cURL request into a variable. We will use the CURLOPT_RETURNTRANSFER
option and set this option value is 1.
或者,我们可以将cURL请求返回的响应放入变量中。 我们将使用CURLOPT_RETURNTRANSFER
选项,并将此选项的值设置为1。
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'http://www.google.com');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
$contents = curl_exec($ch);
echo $contents;
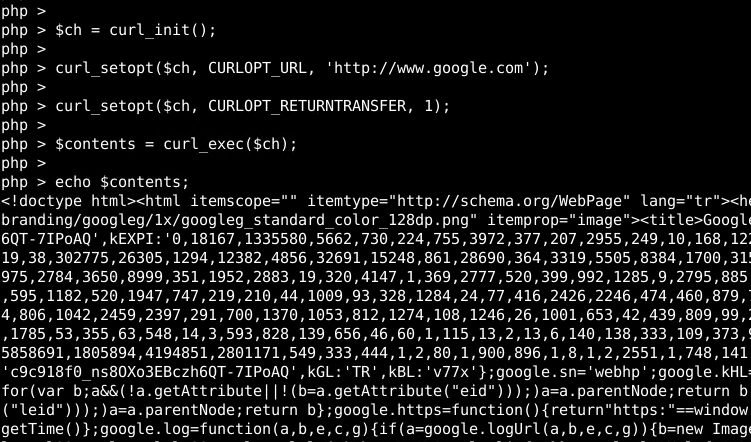
设置用户代理HTTP标头(Set User-Agent HTTP Header)
We can also set the User-Agent header of the HTTP request by using CURLOPT_HEADER
option with the "User-Agent: PofWeb"
value. In this case, the user agent or browser name expressed as PofWeb
.
我们还可以通过将CURLOPT_HEADER
选项与"User-Agent: PofWeb"
值一起使用来CURLOPT_HEADER
HTTP请求的User-Agent标头。 在这种情况下,用户代理或浏览器名称表示为PofWeb
。
$ch = curl_init();
//Set URL as "http://www.poftut.com"
curl_setopt($ch, CURLOPT_URL, "http://www.poftut.com/");
//Set HTTP Header
curl_setopt($ch, CURLOPT_HEADER, Array("User-Agent: PofWeb"));
//Return Page Contents
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
//Close cURL session
curl_close($ch);
跟随重定向 (Follow Redirects)
Redirects are used to redirect the user to from requested URL into another URL. Redirects can be useful if the current URL does not provide historical resources. We can also make the PHP cURL to follow redirects by using CURLOPT_FOLLOWLOCATION
options with the true
.
重定向用于将用户从请求的URL重定向到另一个URL。 如果当前URL不提供历史资源,则重定向可能很有用。 通过使用带有true
CURLOPT_FOLLOWLOCATION
选项,我们还可以使PHP cURL遵循重定向。
// Follow redirects, if any
curl_setopt($curl, CURLOPT_FOLLOWLOCATION, true);
如果响应为400、404,则cURL失败 (Fail cURL If Response Is 400, 404)
The HTTP protocol provides return or HTTP status codes in order to represent the request status. Codes like 4XX, 400, 404 are used to express different error about the processing of the request. If the current PHP cURL request is returned an error status code we can make the cURL fail and return some error code. We will use <span class="pln">CURLOPT_FAILONERROR
option.
HTTP协议提供返回或HTTP状态代码,以表示请求状态。 诸如4XX,400、404之类的代码用于表示有关请求处理的不同错误。 如果当前PHP cURL请求返回了错误状态代码,我们可以使cURL失败并返回一些错误代码。 我们将使用<span class="pln">CURLOPT_FAILONERROR
选项。
// Fail the cURL request if response code = 400 (like 404 errors)
curl_setopt($curl, CURLOPT_FAILONERROR, true);
等待指定的连接时间 (Wait For Specified Time For Connect)
While connecting to the remote server there may be some delay which can be related to internet speed server speed etc. We can set some timeout in order to prevent waiting indefinitely. We will use CURLOPT_TIMEOUT
option and provide the seconds for timeout which is 10 in this example. After 10 seconds the connection request will be stopped.
连接到远程服务器时,可能会有一些延迟,这可能与Internet速度服务器的速度等有关。我们可以设置一些超时以防止无限期地等待。 我们将使用CURLOPT_TIMEOUT
选项,并提供超时秒数,在本示例中为10。 10秒钟后,连接请求将停止。
// Execute the cURL request for a maximum of 50 seconds
curl_setopt($curl, CURLOPT_TIMEOUT, 10);
跳过SSL证书检查 (Skip SSL Certificate Check)
Today’s HTTP connections are very secure with the usage of the TCL/SSL certificates. TLS/SSL certificates are used to encrypt the connection and approved from certificate authorities for the usage of the different parties. The default behavior of PHP cURL is stopping request if the remote TLS/SSL certificate is not valid. We can prevent this and continue even the remote HTTP server TLS/SSL certificates are not valid. We will just set CURLOPT_SSL_VERIFYHOST
and CURLOPT_SSL_VERIFYPEER
options to false
.
通过使用TCL / SSL证书,当今的HTTP连接非常安全。 TLS / SSL证书用于加密连接,并获得证书颁发机构的批准以供不同方使用。 如果远程TLS / SSL证书无效,PHP cURL的默认行为是停止请求。 我们可以防止这种情况,即使远程HTTP服务器TLS / SSL证书无效也可以继续。 我们只是将CURLOPT_SSL_VERIFYHOST
和CURLOPT_SSL_VERIFYPEER
选项设置为false
。
// Do not check the SSL certificates
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, false);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false);
翻译自: https://www.poftut.com/php-curl-tutorial-with-examples/