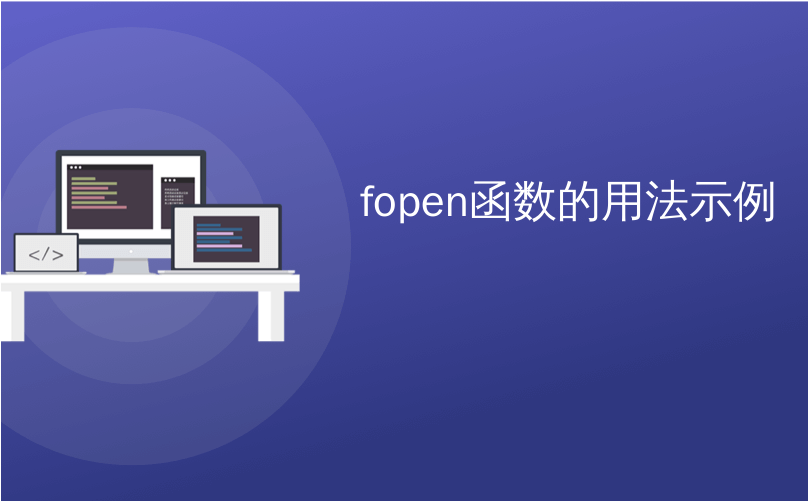
fopen函数的用法示例
In C and C++ programming languages fopen()
function is used to open files and make operations like add, update, create for data. In this tutorial we will learn the syntax, usage and errors about the fopen()
function.
在C和C ++编程语言中, fopen()
函数用于打开文件并进行诸如添加,更新和创建数据之类的操作。 在本教程中,我们将学习有关fopen()
函数的语法,用法和错误。
fopen()函数语法 (fopen() Function Syntax)
fopen()
function is provided by the standard C library. The fopen() function has the following syntax.
fopen()
函数由标准C库提供。 fopen()函数具有以下语法。
FILE *fopen(const char *FILENAME, const char *MODE)
- `FILE *` is the return type of the fopen() function which is a pointer to a FILE structure. This file pointer is used for operations to add, update, etc. FILE *是fopen()函数的返回类型,它是指向FILE结构的指针。 该文件指针用于添加,更新等操作。
- `const char *FILENAME` is simply the file name that will be opened by fopen() function. `const char * FILENAME`只是将由fopen()函数打开的文件名。
- `const char *MODE` is the file open mode that will set the behavior of the file operations like only read, write, append, etc. const char * MODE是文件打开模式,它将设置文件操作的行为,例如仅读,写,追加等。
The fopen() function will return a FILE pointer to the opened file in successful execution. If there is error the return value will be NULL and the global variable errno
will be set for the related error.
fopen()函数将在成功执行后返回指向已打开文件的FILE指针。 如果存在错误,则返回值将为NULL,并且将为相关错误设置全局变量errno
。
文件打开模式 (File Open Modes)
Before starting the examples of the fopen() function we will learn file open modes. File open modes sets and restrict file access type like only read, update, create the file if do not exists, etc. File modes are represented as characters and + sign.
在开始fopen()函数的示例之前,我们将学习文件打开模式。 文件打开模式设置和限制文件访问类型,例如仅读取,更新,创建文件(如果不存在)等。文件模式表示为字符和+号。
- `”r”` is used as the file for reading. This will open the file as read-only and the file cannot be edited in this mode. As expected the file should exist where it will not be created automatically. “ r”用作读取文件。 这将以只读方式打开文件,并且在此模式下无法编辑文件。 如预期的那样,该文件应存在于不会自动创建的位置。
- `”w”` will create an empty file for writing. If the file already exists the existing file will be deleted or erased and the new empty file will be used. Be cautious while using these options. “” w”将创建一个用于写入的空文件。 如果文件已存在,则将删除或删除现有文件,并使用新的空文件。 使用这些选项时要小心。
- `”a”` is used for appending new data into the specified file. The file will be created if it doesn’t exist. “ a”用于将新数据附加到指定文件中。 如果该文件不存在,则将创建该文件。
- `”r+”` mode will open the file to update which will provide read and write rights. But the file must already exist if not it will not be created and throw an error. “ r +”模式将打开要更新的文件,该文件将提供读写权限。 但是文件必须已经存在,否则将无法创建并抛出错误。
- `”w+”` will create an empty file for both reading and writing. “” w +”`将创建一个用于读取和写入的空文件。
- `”a+”` will open a file for reading and appending. “” a +”`将打开一个文件以供读取和附加。
打开文件进行读取(Open File For Reading)
We will start with a simple example where we will open a file named myfile.txt
which is a text file as you expect. But keep in mind that the type or content of the file is not important for fopen() function.
我们将从一个简单的示例开始,我们将打开一个名为myfile.txt
的文件,该文件是您所期望的文本文件。 但是请记住,文件的类型或内容对于fopen()函数并不重要。
#include <stdio.h>
int main () {
//File pointer for "myfile.txt"
FILE *fp;
int c;
//Open the file and set to pointer fp
fp = fopen("myfile.txt","r");
//Read file character by character and
//put to the standard ouput
while(1) {
c = fgetc(fp);
if( feof(fp) ) {
break ;
}
printf("%c", c);
}
//Close the file pointer
fclose(fp);
return(0);
}
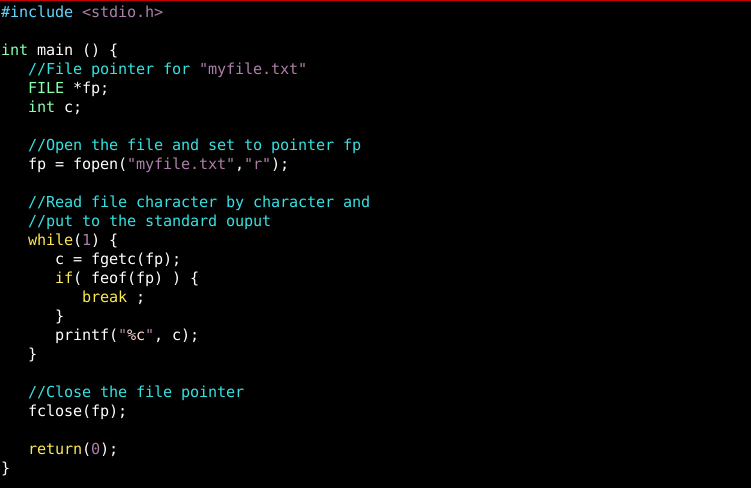
We will compile the source code file read_myfile.c
with the following gcc
command.
我们将使用以下gcc
命令编译源代码文件read_myfile.c
。
$ gcc read_myfile.c -o read_myfile
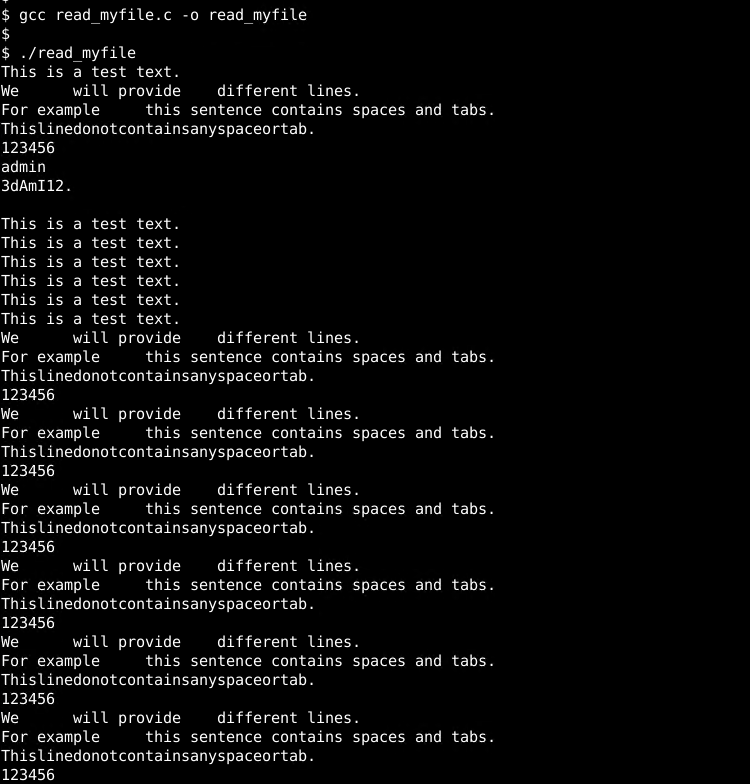
打开文件进行写入 (Open File For Writing)
We can also use "a"
for writing or appending into the existing file. In this example, we will add the following line file named myfile.txt
.
我们还可以使用"a"
将其写入或追加到现有文件中。 在此示例中,我们将添加以下名为myfile.txt
行文件。
I am new line
#include <stdio.h>
int main () {
//File pointer for "myfile.txt"
FILE *fp;
int c;
//Open the file and set to pointer fp
fp = fopen("myfile.txt","a");
//Add or append new line to myfile.txt
// by using fp pointer
fputs("I am a new line",fp);
//Close the file pointer
fclose(fp);
return(0);
}
关闭档案 (Close File)
As fopen() function is used to open a file after the operations are completed we have to close the file. fclose()
function is used to close the given file by using this file pointer and release resources. In previous examples as the last action, we closed the files like below.
由于fopen()函数用于在操作完成后打开文件,因此我们必须关闭文件。 fclose()
函数用于通过使用此文件指针关闭给定文件并释放资源。 在前面的示例中,作为最后一个操作,我们关闭了如下文件。
fclose(fp);
fopen()错误 (fopen() Errors)
While opening files with fopen() function we may get different types of errors. Here we will provide some of the most possible ones.
使用fopen()函数打开文件时,我们可能会遇到不同类型的错误。 在这里,我们将提供一些最可能的方法。
- `EACCES` is related where there is no permission for the specified operation. 如果没有指定操作的许可,则“ EACCES”相关。
- `EINVAL` will raise when the value of the mode argument is not valid. 当mode参数的值无效时,ʻEINVAL`将升高。
- `EINTR` will raise when a signal was caught during fopen(). 在fopen()期间捕获到信号时,ʻEINTR`将升高。
翻译自: https://www.poftut.com/fopen-function-usage-in-c-and-cpp-with-examples/
fopen函数的用法示例