
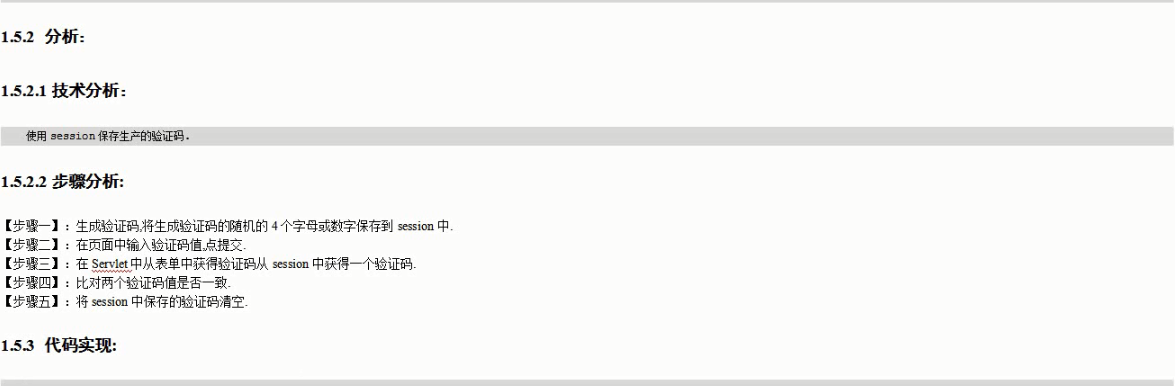
<!DOCTYPE html>
<html>
<head>
<title>MyHtml.html</title>
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="this is my page">
<meta http-equiv="content-type" content="text/html; charset=UTF-8">
<!--<link rel="stylesheet" type="text/css" href="./styles.css">-->
<script type="text/javascript">
function changeimage(){
document.getElementById("imag").src="/J2EE/checkimagservlet?time="+new Date().getTime();
//由于没有改变路径,浏览器有缓存,自动加载缓存内的值,加上时间戳后每次路径不一样
}
</script>
</head>
<body>
<form action="/J2EE/myhttpservlet" method="post">
姓名 <input name="username" type="text"> 密码 <input
name="password" type="password">
<div>
验证码<input name="code" type="text">
</div>
<div>
<img src="/J2EE/checkimagservlet" id="imag" οnclick="changeimage()">
</div>
<!-- //验证码生成的servlet -->
<input type="submit" name="提交">
</form>
</body>
</html>
package javapack;
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Random;
import javax.imageio.ImageIO;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class checkimagservlet extends HttpServlet {
/**
*
*/
private static final long serialVersionUID = 1L;
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
int width=120;
int height=30;
//步骤一 绘制一张内存中的图片
BufferedImage bi=new BufferedImage (width,height,BufferedImage.TYPE_INT_RGB);//表示一个图像,该图像具有整数像素的 8 位 RGB 颜色
//步骤二 图片绘制背景颜色 ---通过绘图对象
Graphics g=bi.getGraphics();//得到画图对象---画笔
//绘制任何图形之前,都必须指定一个颜色
g.setColor(getRandColor(200,250));
g.fillRect(0, 0, width, height);//画填充矩形,前两个参数指的是矩形左上角的坐标,后两个参数是矩形的宽和高
//步骤三 绘制边框
g.setColor(Color.WHITE);
g.drawRect(0, 0, width-1, height-1);
//步骤四 四个随机数字
Graphics2D g2=(Graphics2D) g;
//设置输出字体
g2.setFont(new Font("宋体",Font.BOLD,18));//参数为字体、风格、字号
String words="ABCDEFGHIGKLMNOPQRSTUVWXYZ1234567890";
Random random=new Random();//生成随机数
//将生成的验证码保存到session中
StringBuffer buffer=new StringBuffer();
//定义X坐标
int x=10;
for(int i=0;i<4;i++){
//随机颜色new corlor(r,g,b)
g2.setColor(new Color(20+random.nextInt(110),20+random.nextInt(110),20+random.nextInt(110)));//nextInt(n),生成一个0到n的数
//旋转-30,---30度
int jiaodu =random.nextInt(60)-30;
//换算弧度
double theta =jiaodu*Math.PI/180;
//生成一个随机数字
int index=random.nextInt(words.length());//从word中随便选取一个值
//获得字母数字
char c=words.charAt(index);
//将生成的字加入到buffer中
buffer.append(c);
//将c输出到图片
g2.rotate(theta, x, 20);
g2.drawString(String.valueOf(c), x, 20);
g2.rotate(-theta, x, 20);
x+=30;
}
request.getSession().setAttribute("code", buffer.toString());
//步骤五 绘制干扰线
g.setColor(getRandColor(160,200));
int x1;
int x2;
int y1;
int y2;
for(int i=0;i<30;i++){
x1=random.nextInt(width);
x2=random.nextInt(12);
y1=random.nextInt(height);
y2=random.nextInt(12);
g.drawLine(x1, y1, x1+x2, y1+y2);
}
//将上面图片输出到浏览器
g.dispose();//释放资源
ImageIO.write(bi,"jpg",response.getOutputStream());
}
private Color getRandColor(int fc, int bc) {
// TODO Auto-generated method stub
Random random=new Random();
if(fc>255){
fc=255;
}
if(bc>255){
bc=255;
}
int r=fc+random.nextInt(bc-fc);
int g=fc+random.nextInt(bc-fc);
int b=fc+random.nextInt(bc-fc);
return new Color(r, g, b);
}
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doGet(request, response);
}
}
package javapack;
import java.io.IOException;
import java.io.PrintWriter;
import java.sql.SQLException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class httpservlet extends HttpServlet {
@Override
public void init() throws ServletException {
// TODO Auto-generated method stub
//初始化一个变量count值为0,用来记录登录的人数
int count=0;
//将这个值存入servletcontext
this.getServletContext().setAttribute("count", count);
}
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html; charset=UTF-8");//解决向页面输出中文乱码,而且还可以写HTML
final long serialVersionUID=1L;
//校验验证码
String code1 = request.getParameter("code");
String code2 = (String) request.getSession().getAttribute("code");
request.getSession().removeAttribute("code");//清除session
if(!code1.equalsIgnoreCase(code2))//忽略大小写
{
request.setAttribute("msg", "验证码输入错误");
request.getRequestDispatcher("/html/login.jsp").forward(request,response);
return;
}
//接收表单参数
String username=request.getParameter("username");//通过input的name值获得输入的username
String password=request.getParameter("password");
//封装到实体对象
User user=new User();
user.setUsername(username);//将username与password封装到user对象中
user.setPassword(password);
//调用业务层处理数据
userservice us=new userservice();
try {
User existuser=us.login(user);//将user传入到login方法判断用户是否在数据库中存在
//根据处理结果显示信息(页面跳转)
if(existuser==null){
response.getWriter().println("<h1>登录失败</h1>");
}
else{
//记录登录成功的人数
int count=(Integer) this.getServletContext().getAttribute("count");
count++;
this.getServletContext().setAttribute("count", count);
/*response.getWriter().println("<h1>登录成功,你好,"+existuser.getUsername()+"</h1></br>");
response.getWriter().println("<h1>1s后页面跳转。。。。</h1>");
response.setHeader("Refresh","1;url=/J2EE/countservlet");*/
/*response.setStatus(302);//状态码重定向
response.setHeader("Location", "/J2EE/html/success.html");*/
response.sendRedirect( "/J2EE/countservlet");
return;
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doGet(request, response);//post方式和get方式执行同一方法
}
}