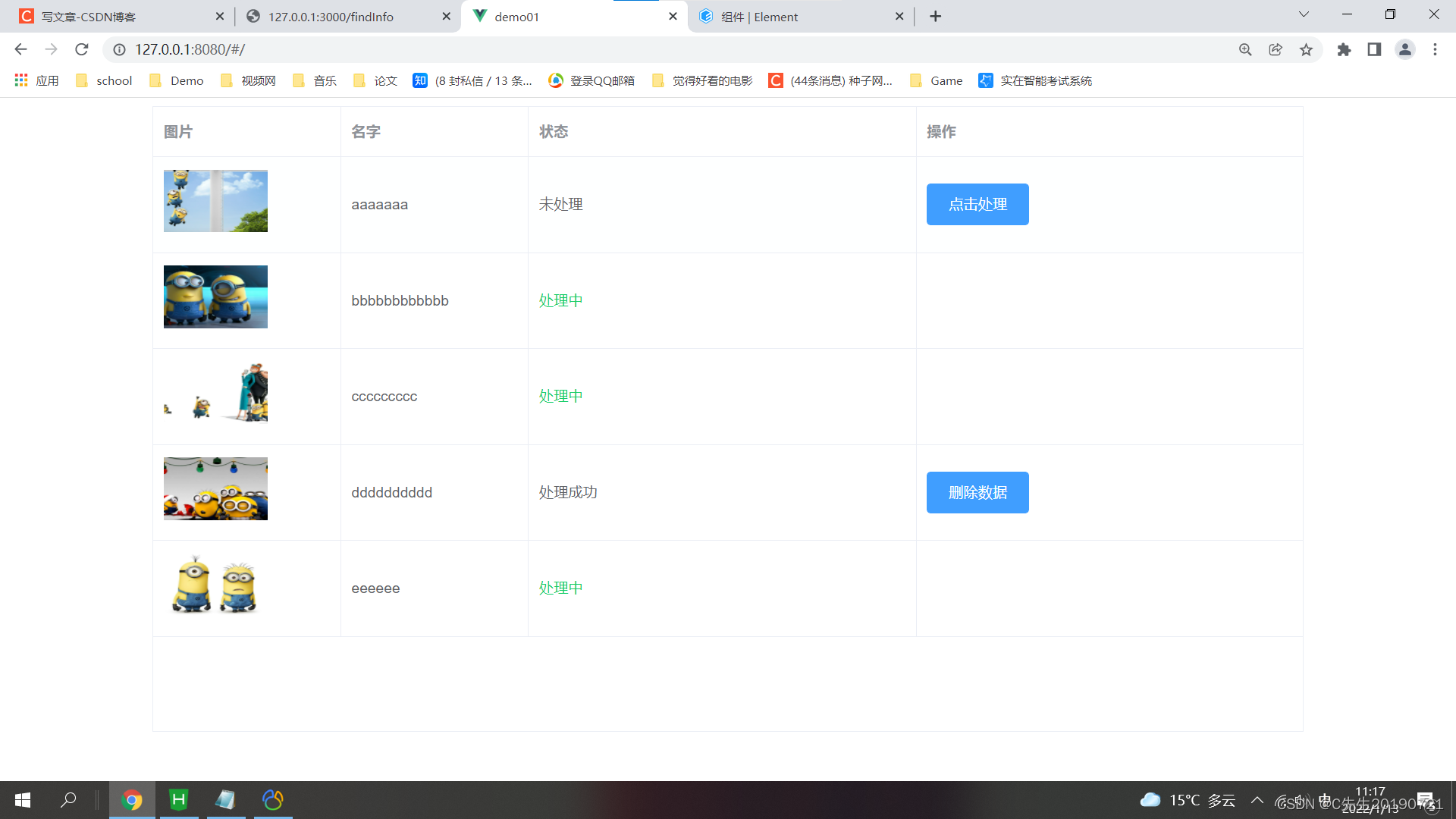
<template>
<div id="app">
<el-table
:data="tableData"
border
style="width: 80%;height: 600px;margin: 0 auto;">
<el-table-column
label="图片"
width="180">
<template slot-scope="scope">
<img :src="scope.row.pic" style="width: 100px;height: 60px;">
</template>
</el-table-column>
<el-table-column
prop="name"
label="名字"
width="180">
</el-table-column>
<el-table-column
label="状态">
<template slot-scope="scope">
<p v-if="scope.row.status === '0'">未处理</p>
<p v-if="scope.row.status === '1'" style="color: #13CE66;">处理中</p>
<p v-if="scope.row.status === '2'">处理成功</p>
</template>
</el-table-column>
<el-table-column
label="操作">
<template slot-scope="scope">
<el-button type="primary" v-if="scope.row.status === '0'" @click="handle(scope.row.id)">点击处理</el-button>
<el-button type="primary" v-if="scope.row.status === '2'">删除数据</el-button>
</template>
</el-table-column>
</el-table>
</div>
</template>
<script>
export default {
name: 'App',
data () {
return {
tableData: []
}
},
methods: {
getData: function () {
let apiUrl = 'http://127.0.0.1:3000/findInfo'
// 视频预览
this.$axios({
url: apiUrl,
method: 'GET',
params: {
// id:1
}
}).then(res => {
console.info(res.data)
this.tableData = res.data
}).catch(e => {
console.log(e)
})
},
handle: function (id) {
let apiUrl = 'http://127.0.0.1:3000/setInfo'
// 视频预览
this.$axios({
url: apiUrl,
method: 'post',
params: {
// id:1
},
data: {
infoId: id
}
}).then(res => {
console.info(res)
this.getData()
}).catch(e => {
console.log(e)
})
}
},
created () {
this.getData()
}
}
</script>
<style>
#app {
font-family: 'Avenir', Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin: 0 auto;
}
</style>
handle: function (id, row) {
let apiUrl = 'http://127.0.0.1:3000/setInfo'
// 视频预览
this.$axios({
url: apiUrl,
method: 'post',
data: {
infoId: id
}
}).then(res => {
console.info(res)
row.status = '1'
}).catch(e => {
console.log(e)
})
}