一、前言
vector 是封装动态数组的顺序容器,连续存储数据,所以我们不仅可以通过迭代器访问存储在 vector 容器中的数据,还能用指向 vector 容器中的数据的常规指针访问数据。这意味着指向 vector 容器中的数据的指针能传递给任何期待指向数组元素的指针的函数。
vector 容器的存储是自动管理的,按需扩张收缩。vector 容器通常占用多于静态数组的空间,因为要分配更多内存以管理将来的增长。 vector 容器增长不是在原空间之后接新空间,而是找更大的内存空间,然后将已有数据拷贝到新空间,释放原空间,vector 容器所用的增长方式不是在每次插入元素时,而是在额外空闲内存耗尽时重分配。
vector 容器是类模板,可以存储内置数据类型(例如:char,int,float 等),自定义数据类型,也可以 vector 容器嵌套容器。使用时包含头文件 <vector>
template<class T, class Allocator = allocator<T>>
class vector {
// 代码块
};
vector 容器的迭代器是支持随机访问的迭代器。例如:
vector<int>::iterator it = v.begin();
cout << *(it + 2) << endl; //访问 v 中下标为 2 的元素
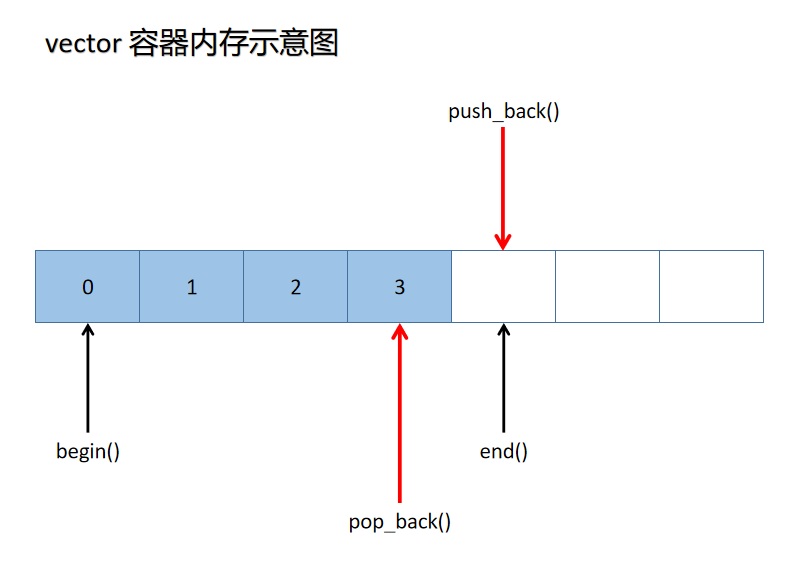
二、 vector 容器类函数
构造函数
vector();
功能:默认构造函数
形数:
返回值:
vector(const vector& right);
功能:拷贝构造函数
形参:
right :另一个 vector 容器对象的常引用
返回值:
vector(iterator first, iterator last);
功能:创建一个vector 容器对象,并将 [first, last ) 区间的元素拷贝到这个 vector 容器对象中
形参:
first :起始迭代器
lsat :末尾迭代器(注意:这个末尾迭代器的元素是 没有被拷贝到这个 vector 容器对象中的)
返回值:
vector(const size_type count, const T& val);
功能:创建一个 vector 容器对象,并将 count 个 T 类型数据拷贝到这个 vector 容器对象中
形参:
count :数量
val :T类型数据的引用(T是模板参数)
返回值:
vector_constructor.cpp
#include <iostream>
#include <vector>
using namespace std;
int main(int argc, char *argv[])
{
vector<int> v1; //调用默认构造函数
v1.push_back(1);
v1.push_back(2);
cout << "---- v1 容器 -----" << endl;
for (vector<int>::iterator it = v1.begin(); it != v1.end(); it++)
cout << *it << endl;
vector<int> v2(v1); //调用拷贝构造函数
cout << "---- v2 容器 -----" << endl;
for (vector<int>::iterator it = v2.begin(); it != v2.end(); it++)
cout << *it << endl;
vector<int> v3(v1.begin(), v1.end()); //调用 vector(iterator first, iterator last);
cout << "---- v3 容器 -----" << endl;
for (vector<int>::iterator it = v3.begin(); it != v3.end(); it++)
cout << *it << endl;
vector<int> v4(3, 100); //调用 vector(const size_type count, const int& val);
cout << "---- v4 容器 -----" << endl;
for (vector<int>::iterator it = v4.begin(); it != v4.end(); it++)
cout << *it << endl;
return 0;
}
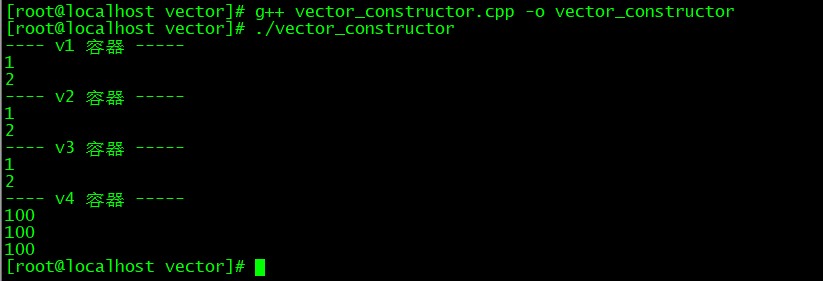
迭代器
begin(); cbegin(); //返回指向起始迭代器(cbegin(); C++11标准加入的)
end(); cend(); //返回指向末尾的迭代器(cend(); C++11标准加入的)
rbegin(); crbegin(); //返回指向起始的逆向迭代器(crbegin(); C++11标准加入的)
rend(); crend(); //返回指向末尾的逆向迭代器(crend(); C++11标准加入的)
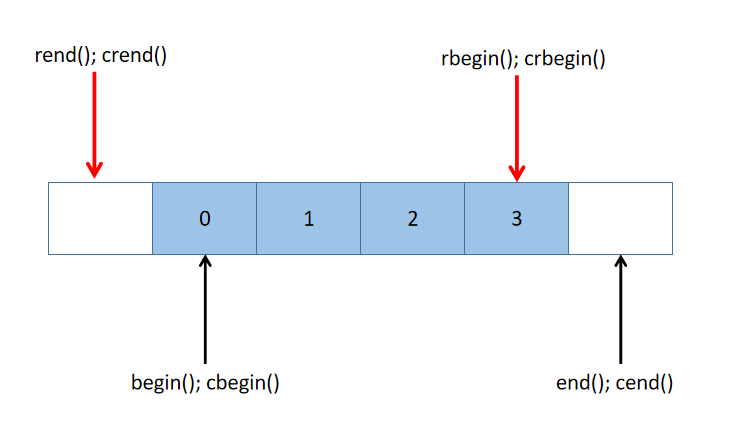
vector_iterator.cpp
#include <iostream>
#include <vector>
using namespace std;
int main(int argc, char *argv[])
{
vector<int> v;
v.push_back(0);
v.push_back(1);
v.push_back(2);
v.push_back(3);
cout << "*begin = " << *(v.begin()) << endl;
cout << "*cbegin = " << *(v.cbegin()) << endl;
cout << "*rbegin = " << *(v.rbegin()) << endl;
cout << "*crbegin = " << *(v.crbegin()) << endl;
return 0;
}
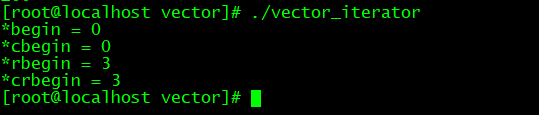
赋值操作
operator=
vector& operator=(const vector& right);
功能:将右值 right 容器对象中的所有元素赋值给这个 vector 容器对象(左值)
形参:
right :另一个 vector 容器对象的常引用
返回值:被赋值的 vector 容器对象的引用
assign
void assign(iterator first, iterator last);
功能:将 [first, last ) 区间的元素赋值给这个 vector 容器对象
形参:
first :起始迭代器
last :末尾迭代器(注意:这个末尾迭代器的元素是 没有被赋值给这个 vector 容器对象中的)
返回值:void
void assign(const size_type count, const T& val);
功能:将 count 个 T 类型数据赋值给这个 vector 容器对象
形参:
count :数量
val :T 类型数据的引用(T是模板参数)
返回值:void
vector_assign.cpp
#include <iostream>
#include <vector>
using namespace std;
int main(int argc, char *argv[])
{
vector<int> v1;
v1.push_back(1);
v1.push_back(2);
cout << "---- v1 容器 ----" << endl;
for (vector<int>::iterator it = v1.begin(); it != v1.end(); it++)
cout << *it << endl;
vector<int> v2;
v2 = v1; //调用 vector& operator=(const vector& right);
cout << "---- v2 容器 ----" << endl;
for (vector<int>::iterator it = v2.begin(); it != v2.end(); it++)
cout << *it << endl;
vector<int> v3;
v3.assign(v1.begin(), v1.end()); //调用 void assign(iterator first, iterator last);
cout << "---- v3 容器 ----" << endl;
for (vector<int>::iterator it = v3.begin(); it != v3.end(); it++)
cout << *it << endl;
vector<int> v4;
v4.assign(3, 100); //调用 void assign(const size_type count, const int& val);
for (vector<int>::iterator it = v4.begin(); it != v4.end(); it++)
cout << *it << endl;
return 0;
}
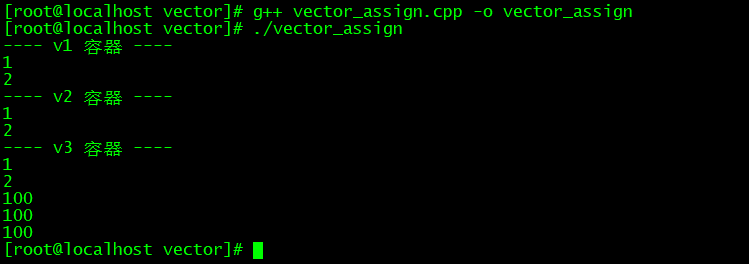
元素访问
at
T& at(const size_type pos);
功能:访问指定下标的元素,普通对象调用这个函数
形参:
pos :要返回的元素的下标
返回值:指定下标元素的引用
const T& at(const size_type pos) const;
功能:访问指定下标的元素,常对象调用这个函数
形参:
pos :要返回的元素的下标
返回值:指定下标元素的常引用
operator[]
T& operator[](const size_type pos);
功能:访问指定下标的元素,普通对象调用这个函数
形参:
pos :要返回的元素的下标
返回值:指定下标元素的引用
const T& operator[](const size_type pos) const;
功能:访问指定下标的元素,常对象调用这个函数
形参:
pos :要返回的元素的下标
返回值:指定下标元素的常引用
front
T& front();
功能:访问第一个元素,普通对象调用这个函数
形参:
返回值:第一个元素的引用
const T& front() const;
功能:访问第一个元素,常对象调用这个函数
形参:
返回值:第一个元素的常引用
back
T& back();
功能:访问最后一个元素,普通对象调用这个函数
形参:
返回值:最后一个元素的引用
const T& back() const;
功能:访问最后一个元素,常对象调用这个函数
形参:
返回值:最后一个元素的常引用
data
T* data();
功能:返回指向存储元素工作的底层数组的指针,指针满足范围 [data(), data() + size() ) 。普通对象调用这个函数
形参:
返回值:指向存储元素工作的底层数组的指针
const T* data() const;
功能:返回指向存储元素工作的底层数组的指针,指针满足范围 [data(), data() + size() ) 。常对象调用这个函数
形参:
返回值:指向存储元素工作的底层数组的常量指针
vector_visit.cpp
#include <iostream>
#include <vector>
using namespace std;
int main(int argc, char *argv[])
{
vector<int> v;
v.push_back(1);
v.push_back(2);
v.push_back(3);
const vector<int> cv(v);
cout << "---- at ----" << endl;
cout << "v[0] = " << v.at(0) << endl; //调用 int& at(const size_type pos);
//cout << "v[3] = " << v.at(3) << endl; //越界,会报 已放弃(吐核)
cout << "cv[2] = " << cv.at(2) << endl; //调用 const int& at(const size_type pos) const;
cout << endl;
cout << "---- operator[] ----" << endl;
cout << "v[0] = " << v[0] << endl; //调用 int& operator[](const size_type pos);
//cout << "v[3] = " << v[3] << endl; //越界,会报 已放弃(吐核)
cout << "cv[2] = " << cv[2] << endl; //调用 const int& operator[](const size_type pos) const;
cout << endl;
cout << "---- front ----" << endl;
cout << "v 第一个元素 = " << v.front() << endl; //调用 int& front();
cout << "cv 第一个元素 = " << v.front() << endl; //调用 const int& front() const;
cout << endl;
cout << "---- back ----" << endl;
cout << "v 最后一个元素 = " << v.back() << endl; //调用 int& back();
cout << "cv 最后一个元素 = " << v.back() << endl; //调用 const int& back() const;
cout << endl;
cout << "---- data ----" << endl;
cout << "---- v ----" << endl;
for (int *p = v.data(); p < v.data() + v.size(); p++) //调用 int* data();
cout << *p << endl;
cout << "---- cv ----" << endl;
for (const int *p = cv.data(); p < cv.data() + cv.size(); p++) //调用 const int* data() const;
cout << *p << endl;
return 0;
}
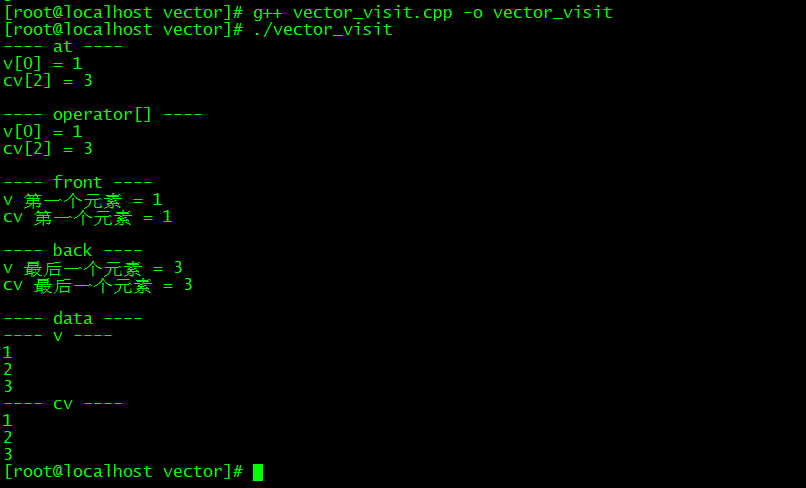
容量
empty
bool empty() const;
功能:判断容器是否为空
形参:
返回值:若容器为空返回 true,否则返回 false
size
size_type size() const;
功能:返回容器中元素个数
形参:
返回值:容器中元素个数
resize
void resize(const size_type newsize);
功能:设置容器的元素个数为 newsize 个
如果 newsize == size(),什么都不做
如果 newsize > size(), 则尾部插入 newsize - size() 个默认的元素(比如 int 元素,默认0)
如果 newsize < size(), 则留下以首元素开始的前 newsize 个元素,后面的都会被擦除掉
形参:
newsize :容器新的元素个数
返回值:
void resize(const size_type newsize, const T& val)
功能:设置容器的元素个数为 newsize 个
如果 newsize == size(),什么都不做
如果 newsize > size(), 则尾部插入 newsize - size() 个值为 val 的元素
如果 newsize < size(), 则留下以首元素开始的前 newsize 个元素,后面的都会被擦除掉
形参:容器新的元素个数
返回值:
max_size
size_type max_size() const;
功能:返回根据系统或库实现限制的容器可存储的元素最大数量
形参:
返回值:根据系统或库实现限制的容器可存储的元素最大数量
reserve
void reserve(const size_type new_capacity);
功能:增加容器的容量到 >= new_capacity;如果 new_capacity 大于当前的 capacity(),则分配新空间,并把原有的元素拷贝到新空间,释放原空间,否则该方法什么也不做
形参:
new_capacity :新容量值
返回值:
capacity
size_type capacity() const;
功能:返回当前存储空间能够容纳的元素个数
形参:
返回值:当前存储空间能够容纳的元素个数
shrink_to_fit
void shrink_to_fit(); (注意:C++11标准加入)
功能:请求移除容器中未使用的容量,请求是否能达成依赖实现
形参:
返回值:
vector_capacity.cpp
#include <iostream>
#include <vector>
using namespace std;
#define MAX 4
int main(int argc, char *argv[])
{
vector<int> v;
for (int i = 0; i < MAX; i++)
v.push_back(i);
vector<int> vm;
cout << "---- v ----" << endl;
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
cout << *it << " ";
cout << endl;
cout << "---- vm ----" << endl;
for (vector<int>::iterator it = vm.begin(); it != vm.end(); it++)
cout << *it << " ";
cout << endl;
cout << endl;
cout << "---- empty ----" << endl;
if (v.empty())
cout << "v is empty." << endl;
else
cout << "v isn't empty." << endl;
if (vm.empty())
cout << "vm is empty." << endl;
else
cout << "vm isn't empty." << endl;
cout << endl;
cout << "---- max_size ----" << endl;
cout << "v max_size = " << v.max_size() << endl;
cout << "vm max_size = " << vm.max_size() << endl;
cout << endl;
cout << "---- size ----" << endl;
cout << "v size = " << v.size() << endl;
cout << "vm size = " << vm.size() << endl;
cout << endl;
cout << "---- capacity ----" << endl;
cout << "v capacity = " << v.capacity() << endl;
cout << "vm capacity = " << vm.capacity() << endl;
v.reserve(100);
vm.reserve(100);
cout << "---- after reserve new capacity 100 ----" << endl;
cout << "v capacity = " << v.capacity() << endl;
cout << "vm capacity = " << vm.capacity() << endl;
v.reserve(50); //比当前 capacity() 小,什么都不做
vm.reserve(50); //比当前 capacity() 小,什么都不做
cout << "---- after reserve new capacity 50 ----" << endl;
cout << "v capacity = " << v.capacity() << endl;
cout << "vm capacity = " << vm.capacity() << endl;
v.shrink_to_fit();
vm.shrink_to_fit();
cout << "---- after shrink_to_fit ----" << endl;
cout << "v capacity = " << v.capacity() << endl;
cout << "vm capacity = " << vm.capacity() << endl;
v.resize(8);
cout << "----after v.resize(8) v ----" << endl;
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
cout << *it << " ";
cout << endl;
vm.resize(8, 10);
cout << "----after vm.resize(8, 10) vm ----" << endl;
for (vector<int>::iterator it = vm.begin(); it != vm.end(); it++)
cout << *it << " ";
cout << endl;
cout << "---- capacity ----" << endl;
cout << "v capacity = " << v.capacity() << endl;
cout << "vm capacity = " << vm.capacity() << endl;
v.resize(2); //从 8 个元素,resize 到 2 个,会留下从首元素开始的前 2 个元素
cout << "----after v.resize(2) v ----" << endl;
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
cout << *it << " ";
cout << endl;
vm.resize(2, 5); //从 8 个元素,resize 到 2 个,会留下从首元素开始的前 2 个元素
cout << "----after vm.resize(2, 5) vm ----" << endl;
for (vector<int>::iterator it = vm.begin(); it != vm.end(); it++)
cout << *it << " ";
cout << endl;
cout << "---- capacity ----" << endl;
cout << "v capacity = " << v.capacity() << endl;
cout << "vm capacity = " << vm.capacity() << endl;
return 0;
}
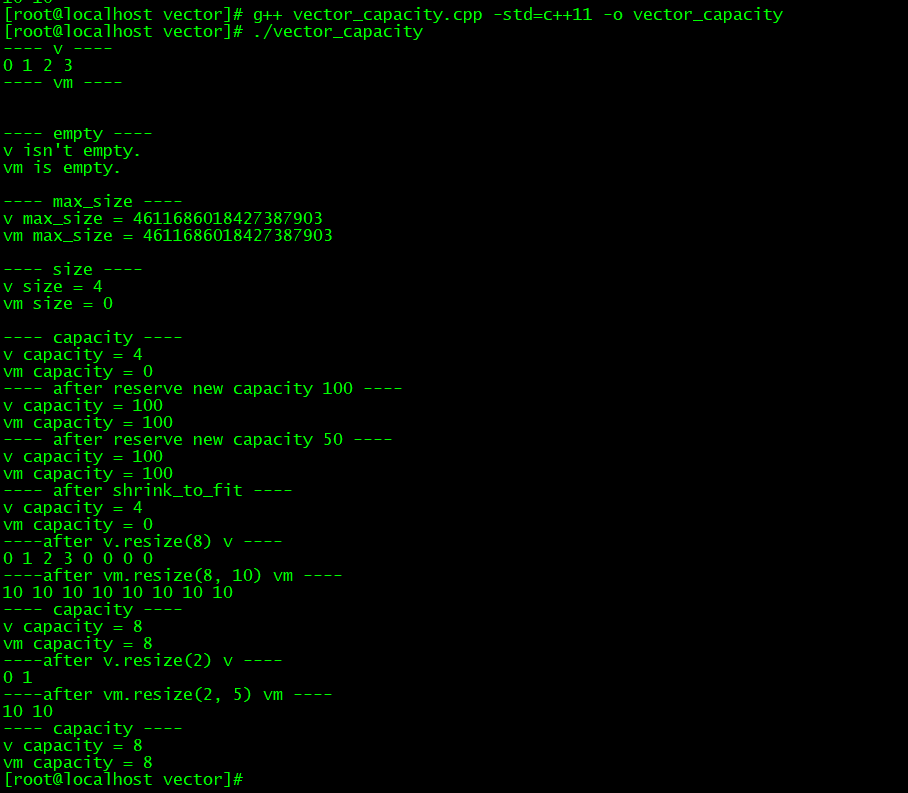
操作
push_back
void push_back(const T& val);
功能:添加数据到容器末尾
形参:
val :T 类型数据的常引用(T 是模板参数)
返回值:
pop_back
void pop_back();
功能:删除容器的末元素
形参:
返回值:
clear
void clear();
功能:清空容器所有元素。调用这个函数后 size() 返回 0
形参:
返回值:
erase
iterator erase(iterator pos); //C++11标准前
iterator erase(const_iterator pos); //C++11 标准起
功能:删除位于 pos 位置的元素
形参:
pos :指向要删除元素的迭代器
返回值:后随被删除元素的迭代器
1. 如果 pos 指向末元素,则返回 end() 迭代器
2. 如果删除不存在的元素,会报 段错误
iterator erase(iterator first, iterator last); //C++11 标准前
iterator erase( const_iterator first, const_iterator last ); //C++11 标准起
功能:删除位于 [first, last ) 区间的元素
形参:
first :要删除元素区间的起始迭代器
last :要删除元素区间的末尾迭代器
返回值:后随最后被删除元素的迭代器
1. 如果 last == end(),则返回更新后的 end() 迭代器
2. 如果 [first, last ) 为空范围,则返回 last 迭代器
iterator insert(iterator pos, const T& val);
功能:在 pos 位置前插入元素
void insert(iterator pos, size_type count, const T& val);
功能:在 pos 位置前插入 count 个 val
void insert(iterator pos, InputIt first, InputIt last);
功能:在 pos 位置 插入 [first, last ) 区间的所有元素
void swap(vector& right);
功能:将容器的内容与 right 的交换
vector_operate.cpp
#include <iostream>
#include <vector>
using namespace std;
#define MAX 4
int main(int argc, char *argv[])
{
vector<int> v;
cout << "---- push_back v ----" << endl;
for (int i = 0; i < MAX; i++)
{
v.push_back(i+1); //调用 void push_back(const T& val);
cout << v[i] << " ";
}
cout << endl;
cout << "---- after v.pop_back() v ----" << endl;
v.pop_back(); //调用 void pop_back();
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
cout << *it << " ";
cout << endl;
cout << "---- erase ----" << endl;
vector<int>::iterator eraseIt = v.begin() + 1;
v.erase(eraseIt); //调用 iterator erase(iterator pos);
cout << "---- after erase(v.begin() + 1) v ----" << endl;
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
cout << *it << " ";
cout << endl;
//v.erase(v.end()); 删除不存在的元素,报段错误
cout << "---- after erase(v.begin(), v.end()) v ---- " << endl;
v.erase(v.begin(), v.end()); //调用 iterator erase(iterator first, iterator last);
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
cout << *it << " ";
cout << endl;
cout << "---- after insert(v.begin(), 1) v ----" << endl;
v.insert(v.begin(), 1); // iterator insert(iterator pos, const T& val);
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
cout << *it << " ";
cout << endl;
cout << "---- after insert(v.end(), 2) v ----" << endl;
v.insert(v.end(), 2); // iterator insert(iterator pos, const T& val);
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
cout << *it << " ";
cout << endl;
cout << "---- after insert(v.end(), 5, 100) v ----" << endl;
v.insert(v.end(), 5, 100); // void insert(iterator pos, size_type count, const T& val);
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
cout << *it << " ";
cout << endl;
cout << "---- after insert(v.end(), v.begin(), v.end()) v ----" << endl;
v.insert(v.end(), v.begin(), v.end()); // void insert(iterator pos, InputIt first, InputIt last);
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
cout << *it << " ";
cout << endl;
cout << "---- swap ----" << endl;
vector<int> v1;
for (int i = 0; i < MAX; i++)
v1.push_back(i+1);
cout << "---- before swap v1----" << endl;
for (vector<int>::iterator it = v1.begin(); it != v1.end(); it++)
cout << *it << " ";
cout << endl;
v.swap(v1); //
cout << "---- after swap v----" << endl;
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
cout << *it << " ";
cout << endl;
cout << "---- after swap v1----" << endl;
for (vector<int>::iterator it = v1.begin(); it != v1.end(); it++)
cout << *it << " ";
cout << endl;
v.clear(); // void clear();
cout << "---- after clear() v----" << endl;
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
cout << *it << " ";
cout << endl;
return 0;
}
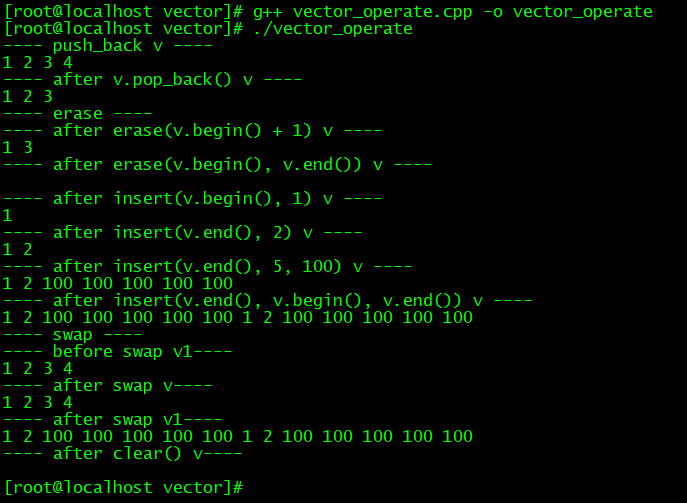
三、vector 容器存储自定义数据
假如,我们有一个自定义的 People 类,这个类中有两个公共数据,分别是名字和年龄,有多个人的数据,需要存储在 vector 容器中,示例如下:
vector_udt.cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class People
{
public:
People(const string name, const int age):m_name(name), m_age(age)
{
}
string m_name;
int m_age;
};
int main(int argc, char *argv[])
{
People p1("a", 10);
People p2("b", 20);
People p3("c", 30);
People p4("d", 40);
People p5("e", 50);
vector<People> v;
v.push_back(p1);
v.push_back(p2);
v.push_back(p3);
v.push_back(p4);
v.push_back(p5);
for (vector<People>::iterator it = v.begin(); it != v.end(); it++)
cout << "name = " << it->m_name << ", age = " << it->m_age << endl;
return 0;
}
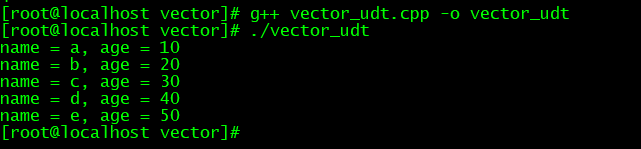
四、vector 容器嵌套容器
vector 容器嵌套 vector 容器,其实就是二维数组
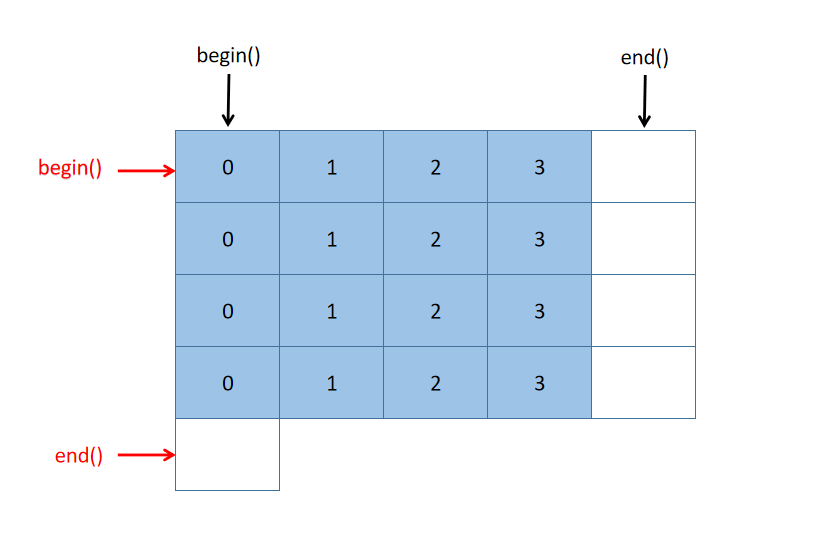
vector_dim.cpp
#include <iostream>
#include <vector>
using namespace std;
int main(int argc, char *argv[])
{
vector<vector<int> > vv;
vector<int> v1;
vector<int> v2;
vector<int> v3;
vector<int> v4;
for (int i = 0; i < 4; i++)
{
v1.push_back(i+1);
v2.push_back(i+2);
v3.push_back(i+3);
v4.push_back(i+4);
}
vv.push_back(v1);
vv.push_back(v2);
vv.push_back(v3);
vv.push_back(v4);
for (vector<vector<int> >::iterator itvv = vv.begin(); itvv != vv.end(); itvv++)
{
for (vector<int>::iterator it = itvv->begin(); it != itvv->end(); it++)
cout << *it << " ";
cout << endl;
}
return 0;
}
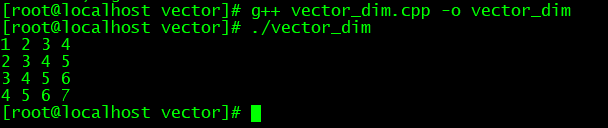
五、循环访问 vector 容器的三种方式(while,for,for_each)
while
依据迭代器
vector<T>::iterator itBegin = v.begin();
vector<T>::iterator itEnd = v.end();
while(itBegin != itEnd)
{
//代码块
itBegin++;
}
for
依据迭代器
for (vector<T>::iterator it = v.begin(); it != v.end(); it++)
{
//代码块
}
for_each
依据头文件 <algorithm> 中提供的 for_each 函数
void func_callback(const T &val) //回调函数
{
//代码块
}
for_each(v.begin(), v.end(), my_print);
vector_loop.cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
void my_print(const int &val)
{
cout << val << endl;
}
int main(int argc, char *argv[])
{
vector<int> v;
for (int i = 0; i < 5; i++)
v.push_back(i);
cout << "---while print----" << endl;
vector<int>::iterator itBegin = v.begin();
vector<int>::iterator itEnd = v.end();
while(itBegin != itEnd)
{
cout << *itBegin << endl;
itBegin++;
}
cout << "----for print----" << endl;
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
{
cout << *it << endl;
}
cout << "----for_each----" << endl;
for_each(v.begin(), v.end(), my_print);
return 0;
}
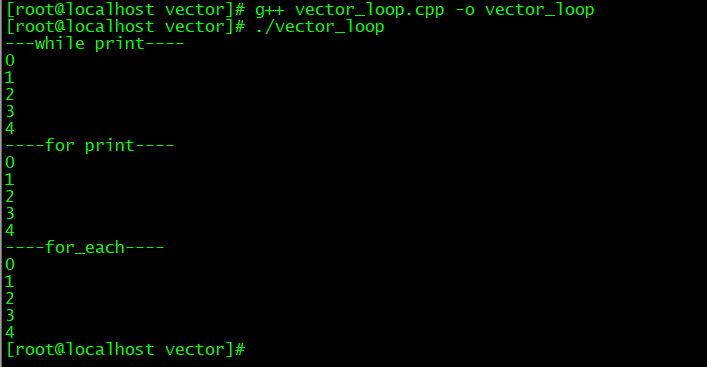