一种不太常见的JQuery中的AJAX请求成功访问Servlet但是html页面未刷新数据的错误原因:
html页面中的AJAX请求代码如下:
<html>
<head>
<meta http-equiv="pragma" content="no-cache" />
<meta http-equiv="cache-control" content="no-cache" />
<meta http-equiv="Expires" content="0" />
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<script type="text/javascript" src="script/jquery-1.7.2.js"></script>
<script type="text/javascript">
$(function(){
// ajax请求
$("#ajaxBtn").click(function(){
// $.ajax,发起ajax请求
$.ajax({
url : "http://localhost:8080/JSON/ajaxServlet",
// 发送给服务器的数据,一般都是请求参数
data : "action=jQueryAjax",
type : "GET",
beforeSend: function() {
alert("请求前")
},
/**
* 成功的回调函数,函数必须输入一个参数,
* 这个参数是服务器返回的数据
*/
success : function (data) {
// dataType为text时需要手动将data转化成json对象
// var $jsonObj = JSON.parse(data);
// $("#msg").html("编号:" + $jsonObj.ID + ",姓名:" + $jsonObj.name);
alert("1111")
// dataType为json时会自动将返回的data转化成json对象
$("#msg").html("编号:" + data.ID + ",姓名:" + data.name);
},
dataType : "json"
// dataType : "test"
});
});
// ajax--get请求
$("#getBtn").click(function(){
// 使用方法:$.get(url,callback,function,dataType)
$.get("http://localhost:8080/JSON/ajaxServlet","action=jQueryGetAjax",
function (data) {
alert("1111");
$("#msg").html("编号:" + data.ID + ",姓名:" + data.name);
},"json");
});
// ajax--post请求
$("#postBtn").click(function(){
// 使用方法:$.post(url,callback,function,dataType)
$.post("http://localhost:8080/JSON/ajaxServlet","action=jQueryPostAjax",
function (data) {
$("#msg").html("编号:" + data.ID + ",姓名:" + data.name);
},"json");
});
// ajax--getJson请求
$("#getJSONBtn").click(function(){
// $.getJson(url,data,callback)
$.getJSON("http://localhost:8080/JSON/ajaxServlet", "action=jQueryGetJsonAjax",
function (data) {
$("#msg").html("编号:" + data.ID + ",姓名:" + data.name);
});
});
/**
* serialize方法
* serialize()可以把表单中所有表单项的内容都获取到,
* 并以 name=value&name=value 的形式进行拼接
*/
$("#submit").click(function(){
// $("#form01").serialize()把表单的参数序列化
$.getJSON("http://localhost:8080/JSON/ajaxServlet",
"action=jQuerySerialize" + "&" + // 这个&号不能少!!!
$("#form01").serialize(), // 加上序列化后的请求参数
function (data) {
$("#msg").html("编号:" + data.ID + ",姓名:" + data.name);
});
});
});
</script>
</head>
<body>
<div>
<button id="ajaxBtn">$.ajax请求</button>
<button id="getBtn">$.get请求</button>
<button id="postBtn">$.post请求</button>
<button id="getJSONBtn">$.getJSON请求</button>
</div>
<div id="msg"></div>
<br/><br/>
<form id="form01" >
用户名:<input name="username" type="text" /><br/>
密码:<input name="password" type="password" /><br/>
下拉单选:<select name="single">
<option value="Single">Single</option>
<option value="Single2">Single2</option>
</select><br/>
下拉多选:
<select name="multiple" multiple="multiple">
<option selected="selected" value="Multiple">Multiple</option>
<option value="Multiple2">Multiple2</option>
<option selected="selected" value="Multiple3">Multiple3</option>
</select><br/>
复选:
<input type="checkbox" name="check" value="check1"/> check1
<input type="checkbox" name="check" value="check2" checked="checked"/> check2<br/>
单选:
<input type="radio" name="radio" value="radio1" checked="checked"/> radio1
<input type="radio" name="radio" value="radio2"/> radio2<br/>
</form>
<button id="submit">提交--serialize()</button>
</body>
</html>
Servlet代码:
public class AjaxServlet extends BaseServlet {
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
super.doPost(request, response);
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
super.doGet(request, response);
}
protected void javaScriptAjax(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("Ajax请求到达。");
// 对Ajax请求的响应
Gson gson = new Gson();
// 转成JSON格式字符串,将数据转给客户端
String json = gson.toJson(new Person(23, "James"));
response.getWriter().write(json);
}
protected void jQueryAjax(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("jQuery方法调用了");
// 对Ajax请求的响应
Gson gson = new Gson();
// 转成JSON格式字符串,将数据转给客户端
String json = gson.toJson(new Person(7, "Durant"));
response.getWriter().write(json);
// System.out.println(1111);
}
protected void jQueryGetAjax(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("jQueryGet方法调用了");
// 对Ajax请求的响应
Gson gson = new Gson();
// 转成JSON格式字符串,将数据转给客户端
String json = gson.toJson(new Person(30, "Curry"));
response.getWriter().write(json);
}
protected void jQueryPostAjax(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("jQueryPost方法调用了");
// 对Ajax请求的响应
Gson gson = new Gson();
// 转成JSON格式字符串,将数据转给客户端
String json = gson.toJson(new Person(13, "Harden"));
response.getWriter().write(json);
}
protected void jQueryGetJsonAjax(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("jQueryGetJsonAjax方法调用了");
// 对Ajax请求的响应
Gson gson = new Gson();
// 转成JSON格式字符串,将数据转给客户端
String json = gson.toJson(new Person(23, "Davis"));
response.getWriter().write(json);
}
protected void jQuerySerialize(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("jQuerySerialize方法调用了");
String username = request.getParameter("username");
// 对Ajax请求的响应
Gson gson = new Gson();
// 转成JSON格式字符串,将数据转给客户端
String json = gson.toJson(new Person(23, username));
response.getWriter().write(json);
}
}
页面应实现的功能是:按下按钮后,
中应出现Servlet中传回来的JSON字符串中的数据,成功启动JSON项目的Tomcat服务器后:

默认访问index.jsp页面:

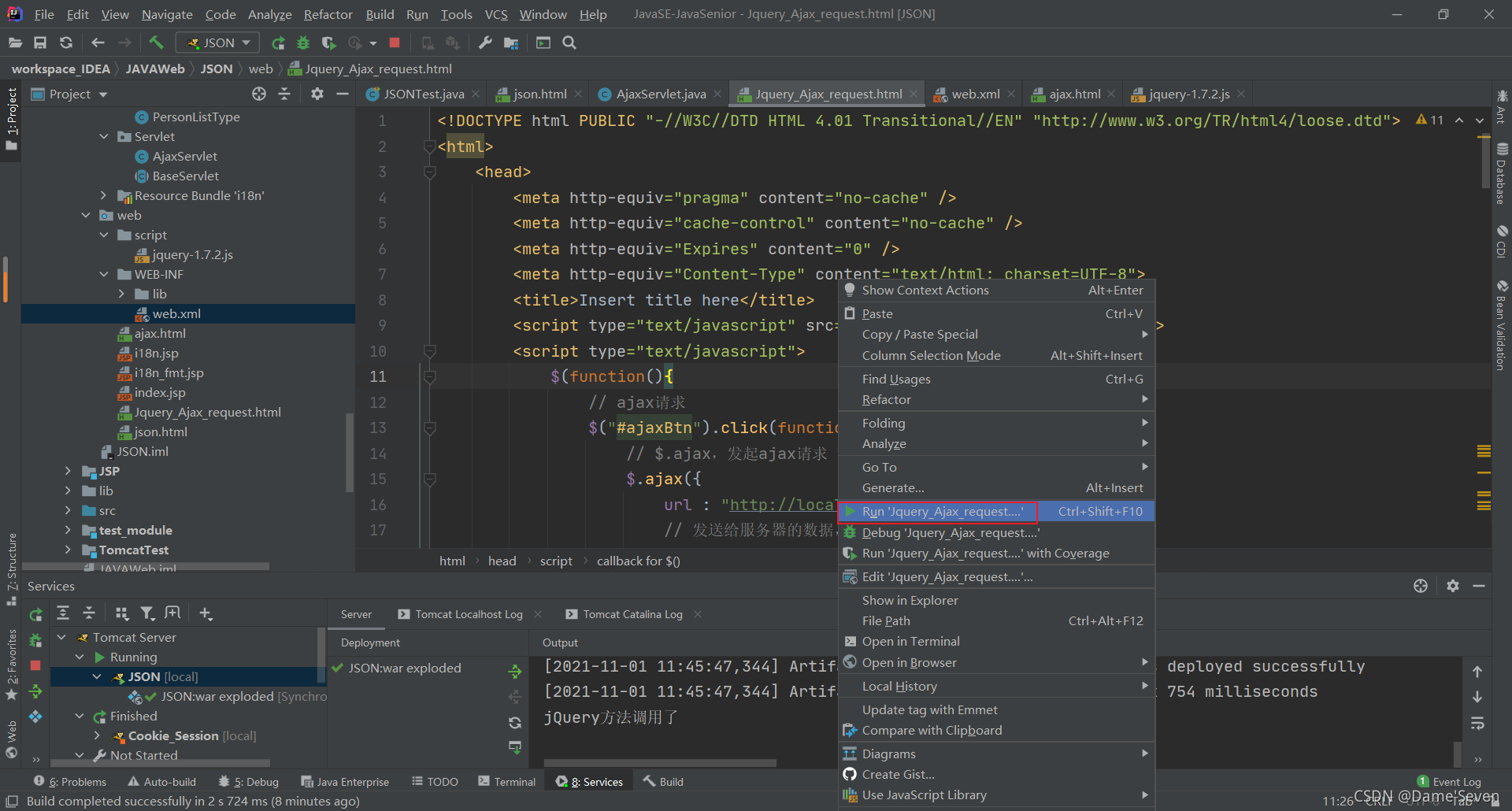
运行html页面后:
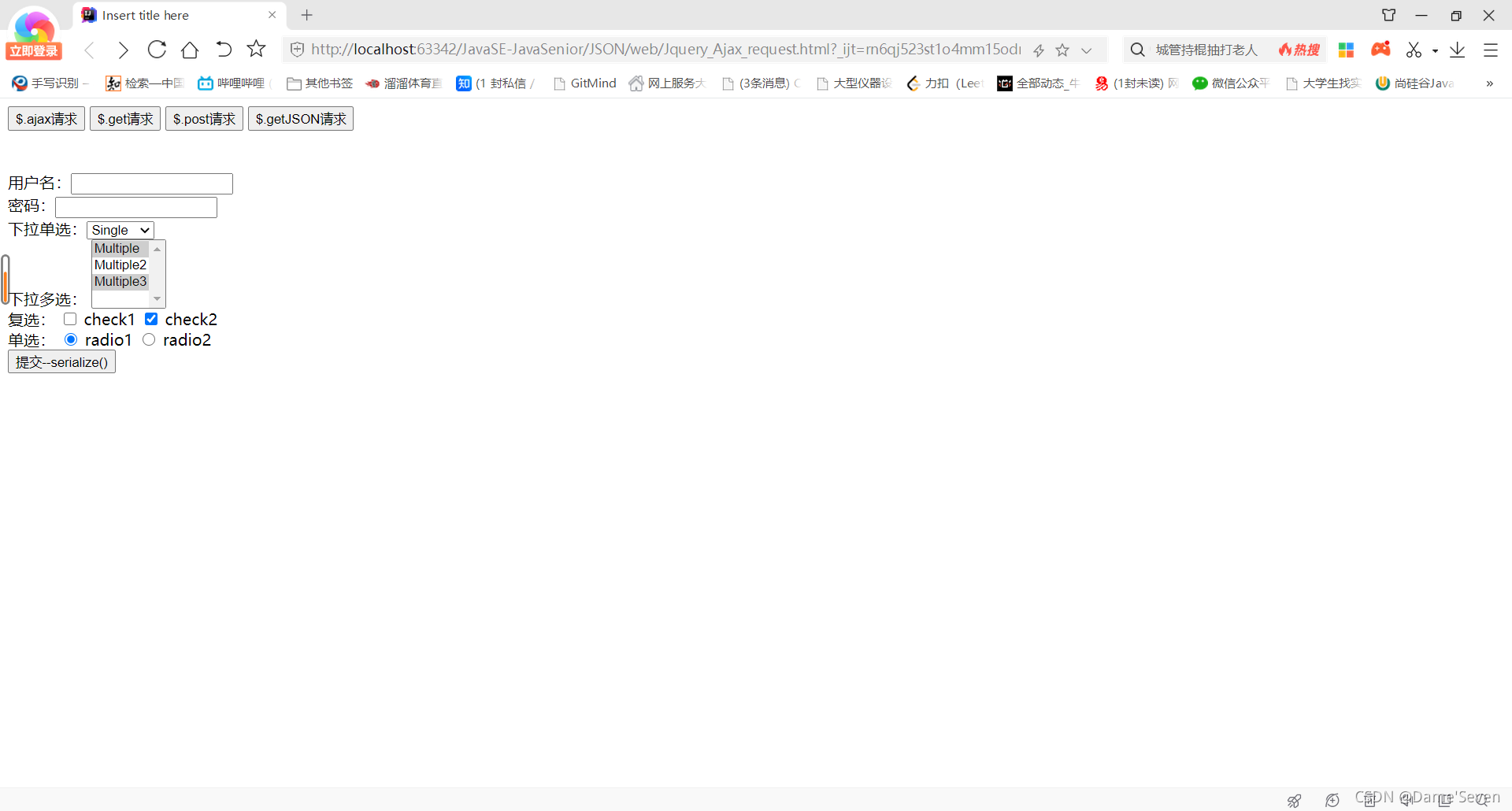
此时,点击每个按钮都会显示Servlet程序成功访问,但是页面上的内容并不会刷新,
找到错误原因:
html页面不能按之前的方式打开,因为之前方式打开的是通过360极速浏览器打开的,而我的JSON工程是部署在Micosoft Edge浏览器上打开的(和自己配置工程是的设置有关),所以Servlet返回的页面是在Micosoft Edge浏览器上的,360极速浏览器上无法成功访问!
正确的访问html页面的方式:
在启动完JSON的Tomcat服务器之后,在浏览器的地址栏输入html地址访问:
再次点击按钮后,页面数据成功刷新: