tomcat 和Servlet版本的对应关系
tomcat安装
bin 专门用来执行服务
conf 专门用来存放及配置文件
lib 专门用来存放tomcat服务器jar包
logs 专门用来存放tomcat服务器输出的日记信息
temp 专门用来存放tomcat运行时产生的临时数据
webapps 专门用来存放web工程
work Tomcat工作时的目录,用来存放Tomcat运行时jsp翻译为servlet的源码,和session的钝化的目录
配置tomcat服务器
双击startup.bat
地址http://localhost:8080
idea创建动态web工程
1.导入tomcat(idea2019适配tomcat9)
2.文件→设置→构建执行部署→Applicationserver添加tomcat路劲
3.创建tomcat工程
web工程添加第三方jar包
方法于java添加jar包一样
创建idea tomcat工程
工程选javaee, webapplication一定要打勾,工程创建在src下
Servlet
什么是Servlet
1.是javaEE规范之一。就是接口
2.javaweb三大组件。Servlet程序、Filter过滤器、Listener监听器.
3.Servlet是运行在服务器上的一个java小程序,它可以接受客户端发送过来的请求,并响应数据给客户端。
Servlet的使用
1.实现Servlet接口
2.实现Servlet方法
//service方法咱们用来处理请求和响应的
@Override
public void service(ServletRequest servletRequest,
ServletResponse servletResponse) throws ServletException, IOException {
//这里面写方法测试是否被访问
}
3.到web.xml中配置servlet程序的访问地址
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<servlet>
<!-- 标签一般就是给Servlet标签其一个别名,一般是类名。-->
<servlet-name>me</servlet-name>
<!-- class是Servlet程序的全类名-->
<servlet-class>js.me</servlet-class>
</servlet>
<servlet-mapping>
<!-- 标签作用就是配置的地址给哪个Servlet使用-->
<servlet-name>me</servlet-name>
<!-- 配置访问地址-->
<!-- / 斜杆在服务器解析的时候,表示地址为http://ip:port/工程路径(tomcat就是工程路径)-->
<!-- /hello 表示地址为http://ip:port/工程路径/hello(别名)-->
<url-pattern>/hello</url-pattern>
</servlet-mapping>
</web-app>
4.运行tomcat,在浏览器路径后加入别名可以在控制台看到servlet被访问了
这里我出现了控制台输出乱码的问题解决方案
解决办法
1.打开到tomcat安装目录下的conf/logging.properties文件夹 修改logging.properties文件,
将 java.util.logging.ConsoleHandler.encoding = utf-8
更改为 java.util.logging.ConsoleHandler.encoding = GBK
运行原理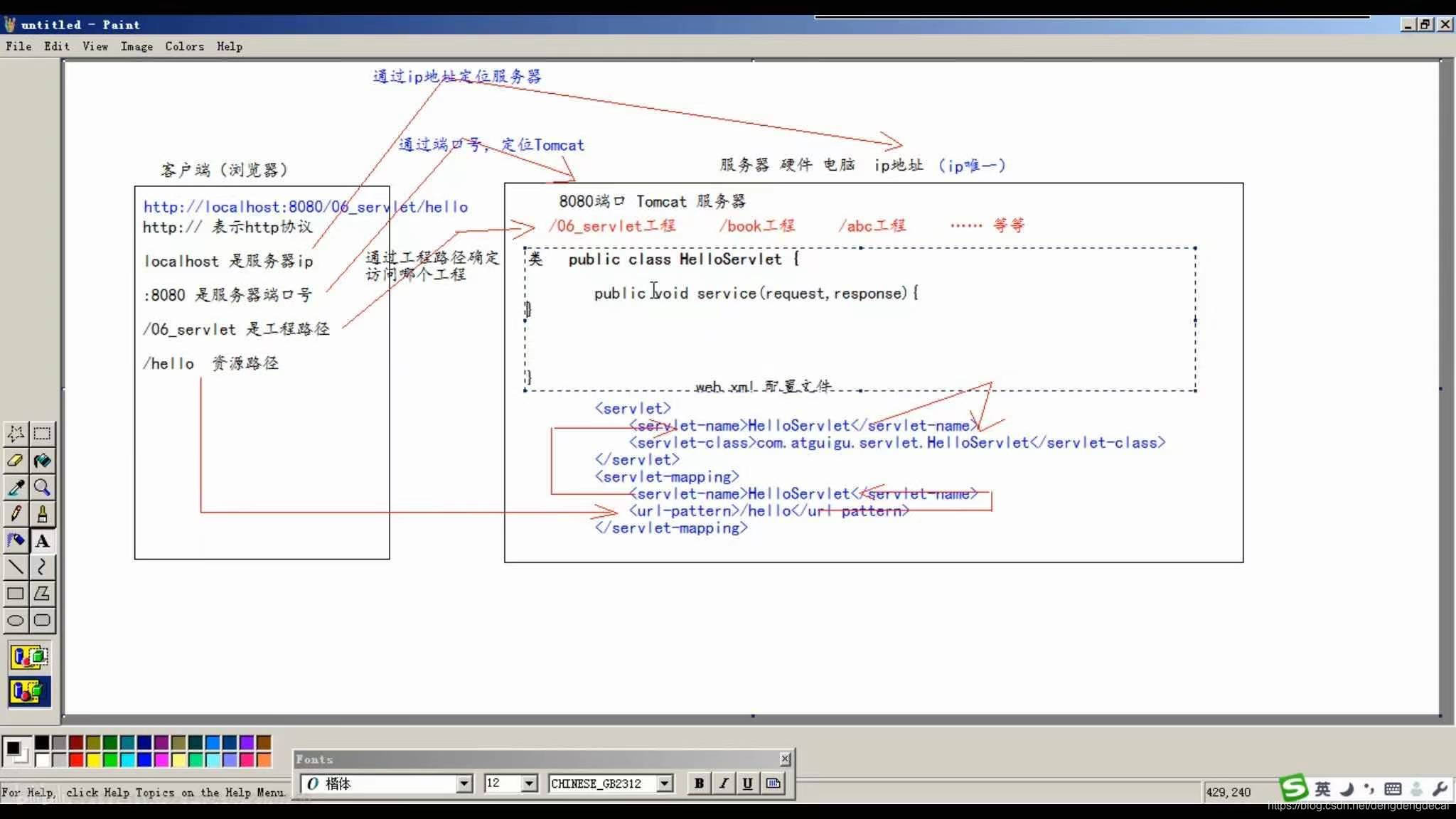
Selvet生命周期
import javax.servlet.*;
import java.io.IOException;
/**
* @Author kevin
* @Description
* @Create 2021-03-15-10:24
* @Modify
*/
public class Seltest implements Servlet {
@Override
public void init(ServletConfig servletConfig) throws ServletException {
//第一步执行初始化方法
}
@Override
public ServletConfig getServletConfig() {
return null;
}
@Override
public void service(ServletRequest servletRequest, ServletResponse servletResponse) throws ServletException, IOException {
//第二步执行服务
}
@Override
public String getServletInfo() {
return null;
}
@Override
public void destroy() {
//第三步销毁
}
}
GET和POST请求分发处理
获取get或post请求
后台
import javax.servlet.*;
import javax.servlet.http.HttpServletRequest;
import java.io.IOException;
/**
* @Author kevin
* @Description
* @Create 2021-03-15-10:24
* @Modify
*/
public class Seltest implements Servlet {
@Override
public void init(ServletConfig servletConfig) throws ServletException {
//第一步执行初始化方法
}
@Override
public ServletConfig getServletConfig() {
return null;
}
@Override
public void service(ServletRequest servletRequest, ServletResponse servletResponse) throws ServletException, IOException {
//第二步执行服务
//这里需要getmethod方法,因为父类没有这个方法,需要强转到子类
HttpServletRequest httpServletRequest =(HttpServletRequest)servletRequest;
String method =httpServletRequest.getMethod();
System.out.println(method);
}
@Override
public String getServletInfo() {
return null;
}
@Override
public void destroy() {
//第三步销毁
}
}
web
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<form action="http://localhost:8080/Tom_war_exploded/hello" method="post" id="123">
<button onclick="123" >请求</button>
</form>
</body>
</html>
通过继承HttpServlet实现Servlet程序
开发中很少通过使用实现类区别get和post请求,一般在实际中的方法
1.编写一个类去继承HttpServlet类
2.根据业务需要重写doGet或doPost
3.到web.xml中的配置Servlet程序的访问地址
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<form action="http://localhost:8080/Tom_war_exploded/hello" method="get" id="123">
<button onclick="123" >请求</button>
</form>
</body>
</html>
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
/**
* @Author kevin
* @Description
* @Create 2021-03-17-11:17
* @Modify
*/
public class SonOfHttpServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("Hello,doGet");
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("Hello,doPost");
}
}
IDEA创建Servlet程序
Selvet类的继承体系
ServletConfig类(配置信息类)
三大作用
1.可以获取Servlet程序的别名servlet-name的值servletConfig.getServletName()
public class Sel implements Servlet {
@Override
public void init(ServletConfig servletConfig) throws ServletException {
System.out.println("hello " + servletConfig.getServletName());
}
2.初始化参数init-param
servletConfig.getInitParameter(“kevin”)
import javax.servlet.*;
import javax.servlet.Servlet;
import java.io.IOException;
/**
* @Author kevin
* @Description
* @Create 2021-03-17-13:35
* @Modify
*/
public class Sel implements Servlet {
@Override
public void init(ServletConfig servletConfig) throws ServletException {
System.out.println("hello " + servletConfig.getServletName());
System.out.println(servletConfig.getInitParameter("kevin"));
}
@Override
public ServletConfig getServletConfig() {
return null;
}
@Override
public void service(ServletRequest servletRequest, ServletResponse servletResponse) throws ServletException, IOException {
}
@Override
public String getServletInfo() {
return null;
}
@Override
public void destroy() {
}
}
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<servlet>
<!-- 标签一般就是给Servlet标签其一个别名,一般是类名。-->
<servlet-name>Selvet</servlet-name>
<!-- class是Servlet程序的全类名-->
<servlet-class>Sel</servlet-class>
<!-- 初始化参数-->
<init-param>
<!-- 参数名-->
<param-name>kevin</param-name>
<!-- 参数值-->
<param-value>https://helloworld.com</param-value>
<!-- 参数名-->
</init-param>
<init-param>
<param-name>durant</param-name>
<!-- 参数值-->
<param-value>https://hellojava.com</param-value>
</init-param>
</servlet>
<servlet-mapping>
<!-- 标签作用就是配置的地址给哪个Servlet使用-->
<servlet-name>Selvet</servlet-name>
<!-- 配置访问地址-->
<!-- / 斜杆在服务器解析的时候,表示地址为http://ip:port/工程路径(tomcat就是工程路径)-->
<!-- /hello 表示地址为http://ip:port/工程路径/hello(别名)-->
<url-pattern>/hello</url-pattern>
</servlet-mapping>
</web-app>
3.获取ServletContext对象
1.什么是ServletContext?
是一个接口,表示Servlet上下文对象
2.一个web工程,只有一个ServletContext对象实例。
3.ServletContext对象是一个域对象
什么是域对象?
域对象,是可以像map一样存取数据的对象,叫域对象
这里的域值得是存取数据的操作范围
4.四个类的作用
1.获取web.xml中配置的上下文参数context.param
2.获取当前的工程路径,格式:/工程路径
3.获取工程部署后再服务器硬盘上的绝对路径
4.像map一样存储数据
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
/**
* @Author kevin
* @Description
* @Create 2021-03-17-14:33
* @Modify
*/
@WebServlet(name = "Servlet")
public class Servlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 1.获取web.xml中配置的上下文参数context.param
// 先获取对象
ServletContext servletContext =getServletConfig().getServletContext();
String fk = servletContext.getInitParameter("kevin");
System.out.println(fk);
// 2.获取当前的工程路径,格式:/工程路径
System.out.println("当前工程路径:"+ servletContext.getContextPath());
// 3.获取工程部署后在服务器硬盘上的绝对路径
System.out.println("当前硬盘路径:"+servletContext.getRealPath("/"));
// 4.像map一样存储数据
//获取servletcontext对象
ServletContext context =getServletContext();
// 保存数据进去
context.setAttribute("key1","value1");
// 根据标签获取数据
System.out.println( context.getAttribute("key1"));
}
}
HTTP协议
什么是http协议
约定好的协议
http协议是指客户端与服务器之间通信时发送的数据需要遵守的规则
Http中发送的数据又叫报文
请求Http协议的格式
客户端发送数据叫请求。
服务器给客户端回传叫响应。
1.get请求
1、请求行
1.1请求的方式 GET
1.2请求的资源路径
1.3请求的协议和版本号
2、请求头
key:value 组成 不同的键值对,表示不同的含义。
请求方法: GET
状态码: 200 /
- 请求标头
Accept: text/html, application/xhtml+xml, image/jxr, */*
Accept-Encoding: gzip, deflate
Accept-Language: zh-CN
Connection: Keep-Alive
Cookie: JSESSIONID=17388F2D2BFC465B97ED6A5A22540190
Host: localhost:8080
User-Agent: Mozilla/5.0 (Windows NT 10.0; WOW64; Trident/7.0; rv:11.0) like Gecko
POST请求
1.请求行
2.请求头
空行
3.请求体(发送给服务器的数据 )
请求 URL: http://localhost:8080/SelvetTest_war_exploded/
请求方法: POST
状态码: 200 /
- 请求标头
Accept: text/html, application/xhtml+xml, image/jxr, */*
Accept-Encoding: gzip, deflate
Accept-Language: zh-CN
Cache-Control: no-cache
Connection: Keep-Alive
Content-Length: 0
Content-Type: application/x-www-form-urlencoded
Cookie: Idea-d8f4592a=46bab709-24fb-4a62-ac17-45f047092f7f
Host: localhost:8080
Referer: http://localhost:63342/SelvetTest/hello.html?_ijt=heus0ggaimp47mqn5i63pmfok4
User-Agent: Mozilla/5.0 (Windows NT 10.0; WOW64; Trident/7.0; rv:11.0) like Gecko
- 响应标头
Connection: keep-alive
Content-Length: 90
Content-Type: text/html; charset=UTF-8
Date: Wed, 17 Mar 2021 13:49:33 GMT
Keep-Alive: timeout=20
Set-Cookie: JSESSIONID=D4AB445C348FB28DA790E962B5FB9D93; Path=/SelvetTest_war_exploded; HttpOnly
在GET和POST中大部分请求头相同大部分请求都是get请求
HttpServletRequest类有什么用
每次只要有请求进入Tomcat服务器,Tomcat服务器就会把请求来的HTTP协议信息计息号封装到Request对象中。传递到service方法(doGet和doPost)中使用。我们可以通过HttpServletRequest对象,获取到所有请求的信息。
HttpServletRequest类的常用方法
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
/**
* @autor kevin
* @detatil
* @create 2021-03-17-22:42
*/
@WebServlet(name = "Servlet2")
public class Servlet2 extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//1.获取请求路径
System.out.println(request.getRequestURI());
//2.获取绝对路径
System.out.println(request.getRequestURL());
//3.获取IP地址
System.out.println(request.getRemoteHost());
//4.获取请求头
System.out.println(request.getHeader("User-Agent"));
//获取请求方式
System.out.println(request.getMethod());
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
}
}
请求转发示例web转selvet2转selvet3处理
WEB
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<form action="http://localhost:8080/Tom_war_exploded/servlet3" method="post">
用户名:<input type="text" name="username"><br>
密码:<input type="password" name="password"><br>
兴趣爱好:
<input type="checkbox" name="hobby" value="C++">C++
<input type="checkbox" name="hobby" value="java">java
<input type="checkbox" name="hobby" value="js">javaScript<br>
<input type="submit">
</form>
</body>
</html>
XML
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<servlet>
<!-- 标签一般就是给Servlet标签其一个别名,一般是类名。-->
<servlet-name>Servlet3</servlet-name>
<!-- class是Servlet程序的全类名-->
<servlet-class>Servlet3</servlet-class>
</servlet>
<servlet-mapping>
<!-- 标签作用就是配置的地址给哪个Servlet使用-->
<servlet-name>Servlet3</servlet-name>
<!-- 配置访问地址-->
<!-- / 斜杆在服务器解析的时候,表示地址为http://ip:port/工程路径(tomcat就是工程路径)-->
<!-- /hello 表示地址为http://ip:port/工程路径/hello(别名)-->
<url-pattern>/servlet3</url-pattern>
</servlet-mapping>
<servlet>
<!-- 标签一般就是给Servlet标签其一个别名,一般是类名。-->
<servlet-name>Servlet2</servlet-name>
<!-- class是Servlet程序的全类名-->
<servlet-class>Servlet2</servlet-class>
</servlet>
<servlet-mapping>
<!-- 标签作用就是配置的地址给哪个Servlet使用-->
<servlet-name>Servlet2</servlet-name>
<!-- 配置访问地址-->
<!-- / 斜杆在服务器解析的时候,表示地址为http://ip:port/工程路径(tomcat就是工程路径)-->
<!-- /hello 表示地址为http://ip:port/工程路径/hello(别名)-->
<url-pattern>/servlet2</url-pattern>
</servlet-mapping>
</web-app>
selvet2
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.util.Arrays;
/**
* @Author kevin
* @Description
* @Create 2021-03-18-8:45
* @Modify
*/
@WebServlet(name = "Servlet3")
public class Servlet3 extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//post请求传输中文可能会乱码所以在请求前可以设置utf-8,必须在获取请求参数之前调用才管用
request.setCharacterEncoding("UTF-8");
String s = request.getParameter("username");
System.out.println("获取到的名字"+s);
//域数据给材料盖一个章,并传递到Servlet2去查看
request.setAttribute("key","柜台一的章");
//请求转发必须要以/打头,斜杠是地址为:http://ip:port/工程名/,映射到IDEA代码的web目录
RequestDispatcher requestDispatcher =request.getRequestDispatcher("/servlet2");
requestDispatcher.forward(request,response);
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("get请求被访问了");
}
}
Selvet3
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
/**
* @Author kevin
* @Description
* @Create 2021-03-17-15:31
* @Modify
*/
@WebServlet(name = "Servlet2")
public class Servlet2 extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String k =request.getParameter("username");
System.out.println("我得到的值是"+k);
Object ky =request.getAttribute("key");
System.out.println("柜台一是否有章"+ky);
System.out.println("Servlet2处理自己的业务");
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
}
}
请求转发特点
1.浏览器地址栏没有变化
2.他们是一次请求
3.他们共享Request域中的数据
4.可以转发到WEB-INF目录下
5.是否可以访问工程以外的资源
base标签的作用
便于移植
跳转回的页面
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<base href="http://localhost:63342/Tom/web/a/b/c/base.html">
</head>
<body>
跳转到了
<a href="../../../test.html">放回首页</a>
</body>
</html>
这是向下跳转的页面,为了对比也加入了转发的方法
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<a href="a/b/c/base.html">往下跳转</a>
<a href="http://localhost:8080/Tom_war_exploded/servletbase">selvet跳转</a>
</body>
</html>
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
/**
* @Author kevin
* @Description
* @Create 2021-03-18-16:11
* @Modify
*/
@WebServlet(name = "Servletbase")
public class Servletbase extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//获取跳转的地址.前进
request.getRequestDispatcher("/a/b/c/base.html").forward(request,response);
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.getRequestDispatcher("/a/b/c/base.html").forward(request,response);
}
}
HttpServletResponse类
HttpServletResponse类的作用
表示要响应的信息,在使用的额使用Tomcat会自动创建一个对象供Servlet使用
两个输出流的说明
字节流 getOutputStream(); 常用于下载(传递二进制数据)
字符流 getWriter(); 常用于回传字符串(常用)
两个流同时只能使用一个
如何往客户端回传字符串数据
解决响应乱码问题
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
/**
* @autor kevin
* @detatil
* @create 2021-03-17-22:42
*/
@WebServlet(name = "Servlet2")
public class Servlet2 extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//方法一
response.setCharacterEncoding("UTF-8");
response.getWriter().println("我给你回应了");
//通过响应头,设置浏览器也设置utf-8字符集
response.setHeader("Content-Type","text/html;charset=UTF-8");
//方法二
//这个方法一定要在获取流对象之前使用
response.setContentType("text/html;charset=UTF-8");
//查看当前流对象的编码格式
System.out.println(response.getCharacterEncoding());
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
}
}
请求重定向
问题
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<servlet>
<!-- 标签一般就是给Servlet标签其一个别名,一般是类名。-->
<servlet-name>Servlet</servlet-name>
<!-- class是Servlet程序的全类名-->
<servlet-class>javaUtil.Servlet</servlet-class>
</servlet>
<servlet-mapping>
<!-- 标签作用就是配置的地址给哪个Servlet使用-->
<servlet-name>Servlet</servlet-name>
<!-- 配置访问地址-->
<!-- / 斜杆在服务器解析的时候,表示地址为http://ip:port/工程路径(tomcat就是工程路径)-->
<!-- /hello 表示地址为http://ip:port/工程路径/hello(别名)-->
<url-pattern>/welcome</url-pattern>
</servlet-mapping>
</web-app>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<link rel="stylesheet" type="text/css" href="CS.css">
</head>
<body style="background-image: url(../lib/background.jpg);
background-repeat: no-repeat;
background-size: 100% 100%;
background-attachment: fixed;">
<div>
<form method="get" action = "http://localhost:8080/WEB_war_exploded/welcome">
账户:<input value="" id="account" class="verify" name="account">
<br>
密码:<input value="" id="password" class="verify" name="password">
<br>
<input type="submit" value="提交">
</form>
</div>
</body>
</html>
package javaUtil;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
/**
* @autor kevin
* @detatil
* @create 2021-04-10-21:20
*/
@WebServlet(name = "Servlet")
public class Servlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("我爱李炎燕");
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String name = request.getParameter("account");
String password = request.getParameter("password");
System.out.println(name);
System.out.println(password);
String sql = "INSERT INTO USER(Account,Password)VALUES(?,?);";
JDBCutil.Insert(sql,name,password);
}
}
package javaUtil;
import com.alibaba.druid.pool.DruidDataSourceFactory;
import org.apache.commons.dbutils.QueryRunner;
import javax.sql.DataSource;
import java.io.InputStream;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.Properties;
/**
* @autor kevin
* @detatil
* @create 2021-04-10-22:31
*/
public class JDBCutil {
static Connection connection =null;
static {
Properties properties =new Properties();
InputStream resourceAsStream = JDBCutil.class.getClassLoader().getResourceAsStream("javaUtil\\JDBC.property");
try {
properties.load(resourceAsStream);
DataSource dataSource = DruidDataSourceFactory.createDataSource(properties);
connection = dataSource.getConnection();
} catch (Exception e) {
e.printStackTrace();
}
}
public static void Insert(String sql,Object ...arg){
QueryRunner queryRunner = new QueryRunner();
System.out.println("正在修改");
try {
queryRunner.update(connection,sql,arg);
} catch (SQLException e) {
e.printStackTrace();
}
try {
connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
System.out.println("修改成功");
}
}
解决
原来我把前端也放到src下了,偶然一次处理同样问题,用同样方法解决了,唯一的区别就是这次我把前端放到web目录中了
第一次
第二次
我修改了第一次的web目录,报了另外一个错误,说明之前问题解决。
现在的错误是
java.lang.NoClassDefFoundError: org/apache/commons/dbutils/ResultSetHandler
最近这次也出现类似java.lang.NoClassDefFound错误
解决办法
重启服务器就OK 了。
AJAX请求
概念
全局刷新:使用form,href等发起的请求是全局刷新(web跳转,转发)
缺点:数据量大,等待时间长,占用带宽,浏览器需要创新渲染页面
过程
Form表单发起请求,服务器使用servlet,jsp接收请求
局部刷新:Ajax请求,页面局部刷新
优点:一个页面可以做多个局部刷新
用户体验好,现在基本都尽量用局部刷新
过程
异步对象
Ajax的异步请求对象(XMLHttpRequest)
步骤
第一步当然需要XMLHttpRequest对象
Var xmlhttprequest = New XMLHttpRequest;
第二步声明请求方式,请求地址,是否异步
Var addr =”servlet地址=信息名”+信息的值”
xmlHttpRequest.open(method:GET,url”addr”,true)
第三步发布请求
xmlHttpRequest.send()
XMLHttpRequest的属性方法
Readystate属性:请求的状态
0:表示创建异步对象,new XMLHttpRequst();
1:表示初始异步对象的请求参数。执行open方法
2:使用send()方法发送请求。
3:使用一部对象从服务器段接收数据
4:一部对象接收了数据,并在一部对象内部处理完成后。
Status属性:网络状态,和Http的状态码对应
200:请求成功
404:服务器资源没有找到
500:服务器内部代码有误
ResponseText属性:表示服务器端返回的数据
例如:var data = xmlhttprequest.responseTest;
全局刷新实例(这里有值得一提的点是,setattrabute方法,在java中设置的是属性和属性值。在js中设置的是标签的属性和属性值如 setattrabute(id,1))
//给处理的值赋予属性
request.setAttribute("info",ret);
//转发数据给别的地址
request.getRequestDispatcher("/Result.jsp").forward(request,response);
转发给对象调用值
接收对象
<%--
Created by IntelliJ IDEA.
User: 信息中心
Date: 2021/4/24
Time: 21:53
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
我收到值: ${info}
</body>
</html>
局部刷新就不写了
同步和异步的区别
同步只创建一个servlet对象接收发送请求,一个一个来。
异步创建多个对象处理请求。