由于Hystrix没有更多更新,阿里巴巴技术团队建议使用Sentinel作为替代方案。 这是 Sentinel开源 系列的一部分。
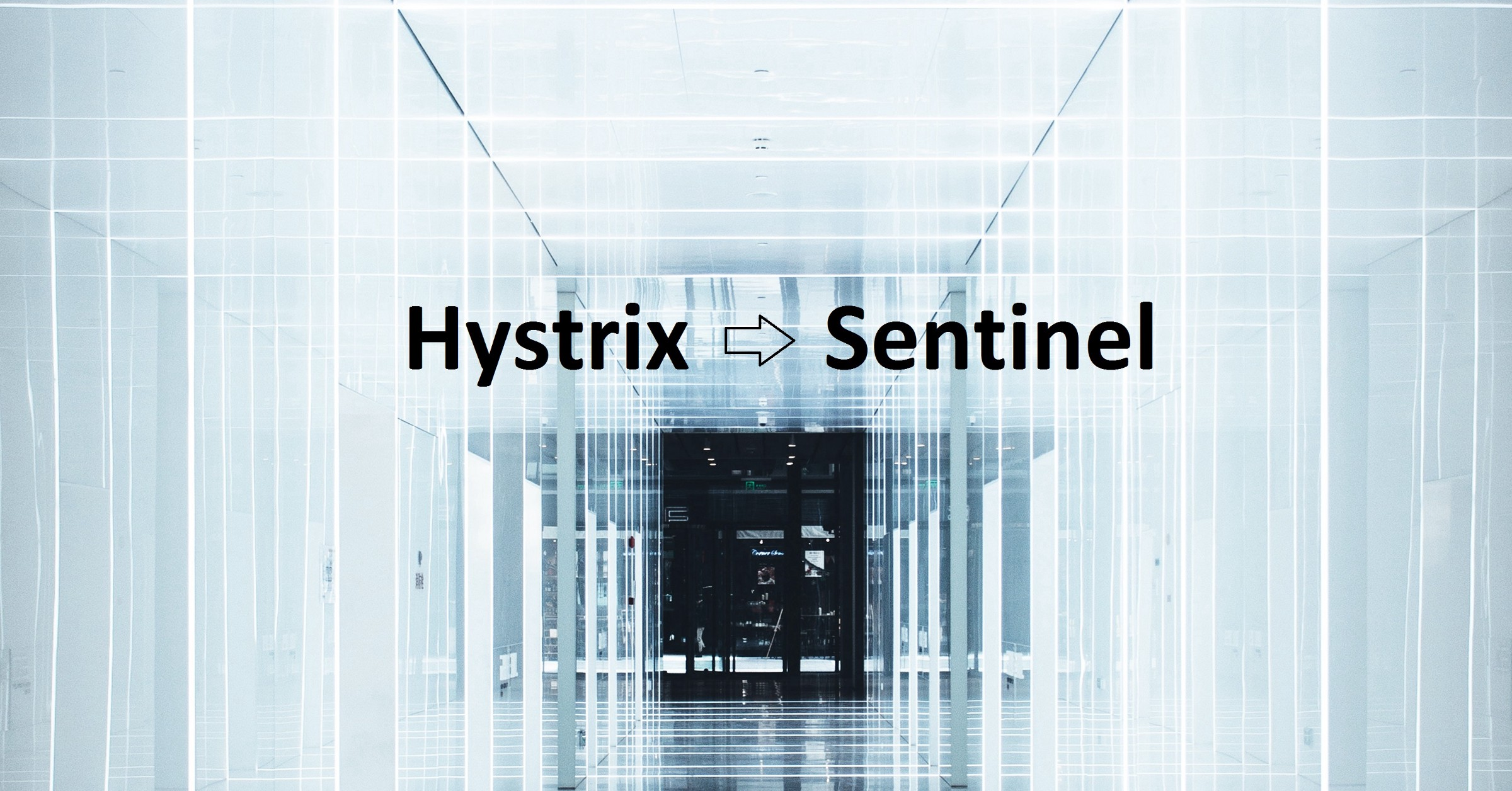
随着微服务变得越来越流行,服务之间的稳定性变得越来越重要。 流服务,容错和系统负载保护等技术已广泛用于微服务系统中,以提高系统的鲁棒性并保证业务的稳定性,并最大程度地减少由于过多的访问流量和沉重的系统负载而导致的系统中断。
Hystrix是Netflix的一个开放源代码延迟和容错库,最近在GitHub主页上宣布不再开发新功能。 建议开发人员使用其他仍处于活动状态的开源项目。那么有什么替代方案?
上次我们引入Resilience4j和Sentinel: Netflix Hystrix的两个开源替代方案
本文将帮助您从Hystrix迁移到Sentinel,并帮助您快速使用Sentinel。
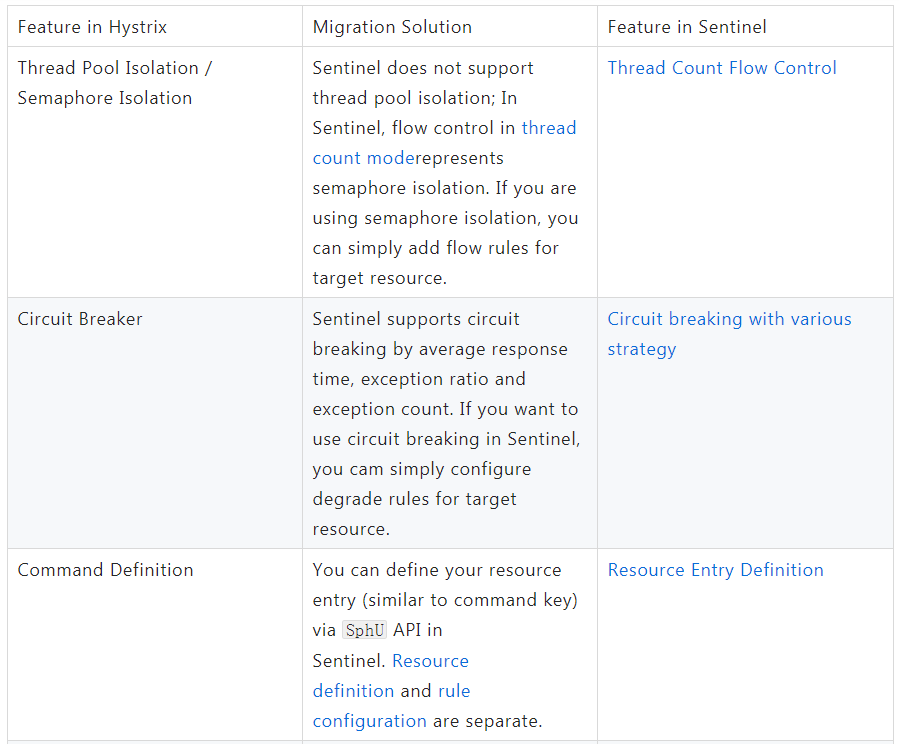
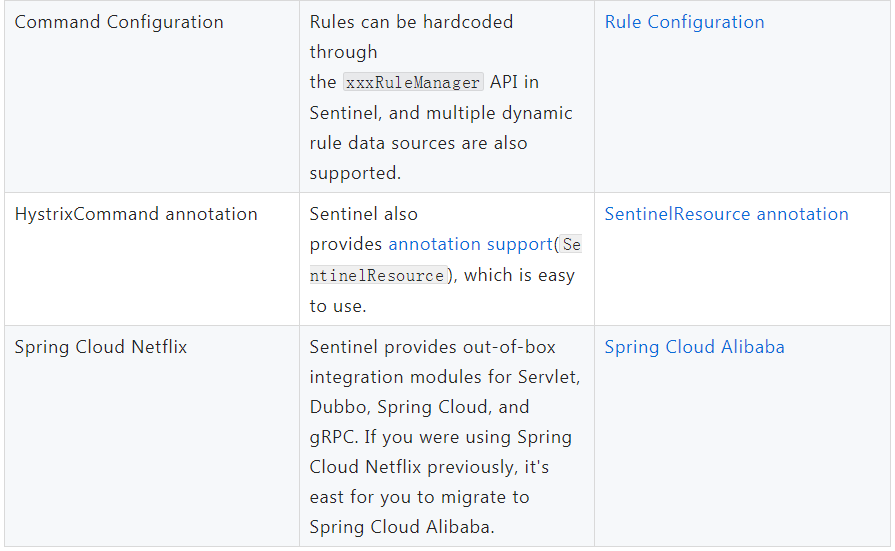
HystrixCommand
Hystrix的执行模型是通过命令模式设计的,该命令模式将业务逻辑和回退逻辑封装到单个命令对象( HystrixCommand
/ HystrixObservableCommand
)中。 一个简单的例子:
public class SomeCommand extends HystrixCommand<String> {
public SomeCommand() {
super(Setter.withGroupKey(HystrixCommandGroupKey.Factory.asKey("SomeGroup"))
// command key
.andCommandKey(HystrixCommandKey.Factory.asKey("SomeCommand"))
// command configuration
.andCommandPropertiesDefaults(HystrixCommandProperties.Setter()
.withFallbackEnabled(true)
));
}
@Override
protected String run() {
// business logic
return "Hello World!";
}
}
// The execution model of Hystrix
// sync mode:
String s = new SomeCommand().execute();
// async mode (managed by Hystrix):
Observable<String> s = new SomeCommand().observe();
Sentinel不指定执行模型,也不在乎代码的执行方式。 在Sentinel中,您应该做的只是使用Sentinel API包装代码以定义资源:
Entry entry = null;
try {
entry = SphU.entry("resourceName");
// your business logic here
return doSomeThing();
} catch (BlockException ex) {
// handle rejected
} finally {
if (entry != null) {
entry.exit();
}
}
在Hystrix中,通常必须在定义命令时配置规则。 在Sentinel中,资源定义和规则配置是分开的。 用户首先通过Sentinel API为相应的业务逻辑定义资源,然后在需要时配置规则。 有关详细信息,请参阅文档 。
线程池隔离
线程池隔离的优点是隔离相对彻底,并且可以为资源的线程池进行隔离,而不会影响其他资源。 但是缺点是线程数很大,线程上下文切换的开销非常大,尤其是对于低延迟调用。 Sentinel不提供如此繁重的隔离策略,但提供了一种相对轻量级的隔离策略-线程计数流控制作为信号隔离。
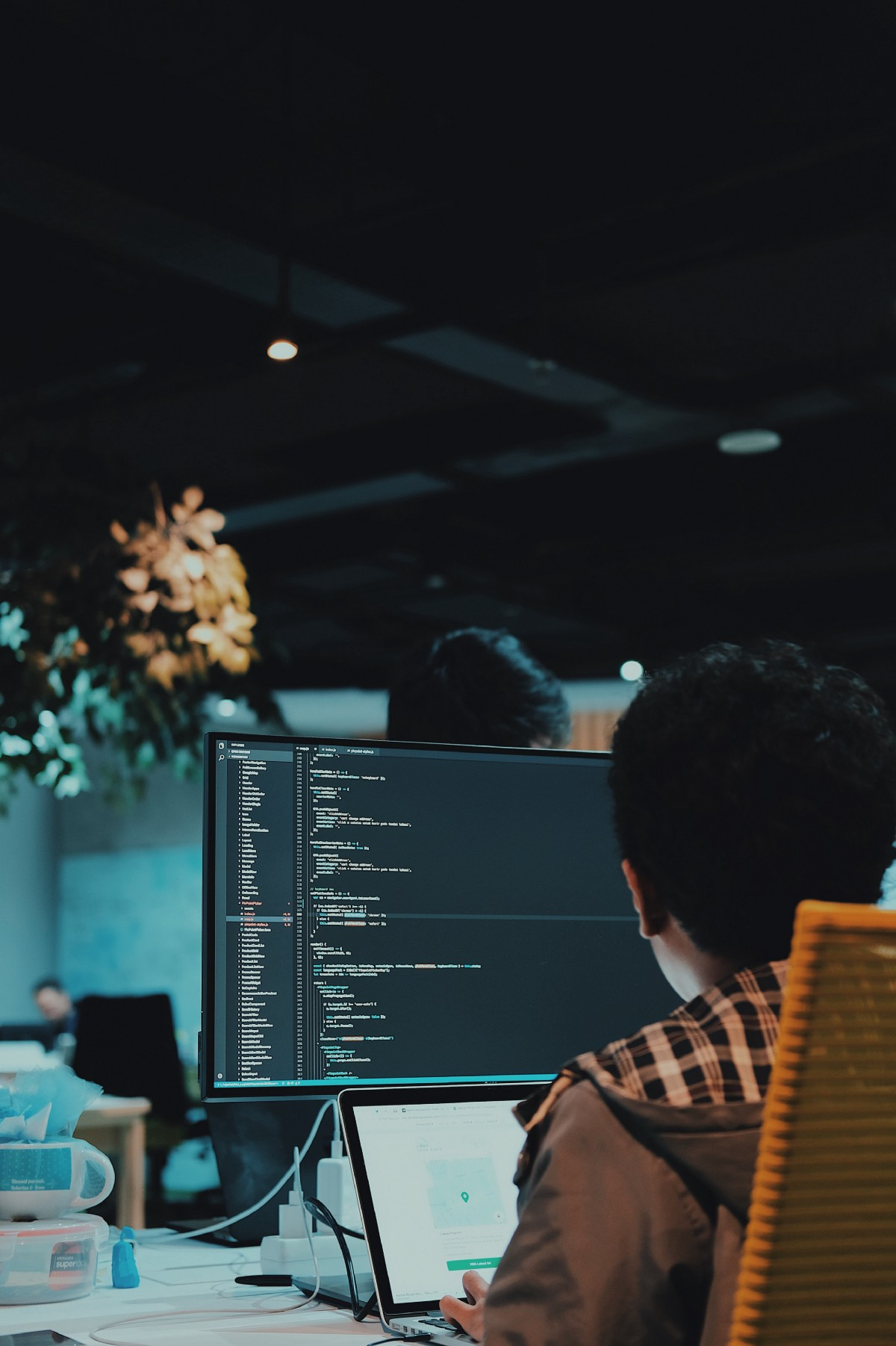
信号量隔离
Hystrix的信号量隔离在Command定义中配置,例如:
public class CommandUsingSemaphoreIsolation extends HystrixCommand<String> {
private final int id;
public CommandUsingSemaphoreIsolation(int id) {
super(Setter.withGroupKey(HystrixCommandGroupKey.Factory.asKey("SomeGroup"))
.andCommandPropertiesDefaults(HystrixCommandProperties.Setter()
.withExecutionIsolationStrategy(ExecutionIsolationStrategy.SEMAPHORE)
.withExecutionIsolationSemaphoreMaxConcurrentRequests(8)));
this.id = id;
}
@Override
protected String run() {
return "result_" + id;
}
}
在Sentinel中,信号隔离是作为流控制模式(线程计数模式)提供的,因此您只需要为资源配置流规则:
FlowRule rule = new FlowRule("doSomething") // resource name
.setGrade(RuleConstant.FLOW_GRADE_THREAD) // thread count mode
.setCount(8); // max concurrency
FlowRuleManager.loadRules(Collections.singletonList(rule)); // load the rules
如果您使用的是Sentinel仪表板,则还可以轻松地在仪表板中配置规则。
断路
Hystrix断路器支持错误百分比模式。 相关属性:
-
circuitBreaker.errorThresholdPercentage
:阈值 -
circuitBreaker.sleepWindowInMilliseconds
:断路器打开时的睡眠窗口
例如:
public class FooServiceCommand extends HystrixCommand<String> {
protected FooServiceCommand(HystrixCommandGroupKey group) {
super(Setter.withGroupKey(HystrixCommandGroupKey.Factory.asKey("OtherGroup"))
// command key
.andCommandKey(HystrixCommandKey.Factory.asKey("fooService"))
.andCommandPropertiesDefaults(HystrixCommandProperties.Setter()
.withExecutionTimeoutInMilliseconds(500)
.withCircuitBreakerRequestVolumeThreshold(5)
.withCircuitBreakerErrorThresholdPercentage(50)
.withCircuitBreakerSleepWindowInMilliseconds(10000)
));
}
@Override
protected String run() throws Exception {
return "some_result";
}
}
在Sentinel中,您只需要为要自动降级的资源配置断路规则。 例如,与上面的Hystrix示例相对应的规则:
DegradeRule rule = new DegradeRule("fooService")
.setGrade(RuleConstant.DEGRADE_GRADE_EXCEPTION_RATIO) // exception ratio mode
.setCount(0.5) // ratio threshold (0.5 -> 50%)
.setTimeWindow(10); // sleep window (10s)
// load the rules
DegradeRuleManager.loadRules(Collections.singletonList(rule));
如果使用的是Sentinel仪表板 ,则还可以在仪表板中轻松配置断路规则。
除了例外率模式,Sentinel还支持基于平均响应时间和微小例外的自动断路。
注释支持
Hystrix提供注释支持以封装命令并对其进行配置。 这是Hystrix注释的示例:
// original method
@HystrixCommand(fallbackMethod = "fallbackForGetUser")
User getUserById(String id) {
throw new RuntimeException("getUserById command failed");
}
// fallback method
User fallbackForGetUser(String id) {
return new User("admin");
}
Hystrix规则配置与命令执行捆绑在一起。 我们可以在@HystrixCommand annotation
的commandProperties
属性中配置命令规则,例如:
@HystrixCommand(commandProperties = {
@HystrixProperty(name = "circuitBreaker.errorThresholdPercentage", value = "50")
})
public User getUserById(String id) {
return userResource.getUserById(id);
}
使用Sentinel注释类似于Hystrix,如下所示:
- 添加注释支持依赖项:
sentinel-annotation-aspectj
并将方面注册为Spring bean(如果您使用的是Spring Cloud Alibaba,则该bean将自动注入); - 将
@SentinelResource
批注添加到需要流控制和断路的方法中。 您可以在注释中设置fallback
或blockHandler
函数; - 配置规则
有关详细信息,请参阅注释支持文档 。 Sentinel注释的示例:
// original method
@SentinelResource(fallback = "fallbackForGetUser")
User getUserById(String id) {
throw new RuntimeException("getUserById command failed");
}
// fallback method (only invoked when the original resource triggers circuit breaking); If we need to handle for flow control / system protection, we can set `blockHandler` method
User fallbackForGetUser(String id) {
return new User("admin");
}
然后配置规则:
- 通过API(例如
DegradeRuleManager.loadRules(rules)
方法)
DegradeRule rule = new DegradeRule("getUserById")
.setGrade(RuleConstant.DEGRADE_GRADE_EXCEPTION_RATIO) // exception ratio mode
.setCount(0.5) // ratio threshold (0.5 -> 50%)
.setTimeWindow(10); // sleep window (10s)
// load the rules
DegradeRuleManager.loadRules(Collections.singletonList(rule));
整合方式
Sentinel具有与Web Servlet,Dubbo,Spring Cloud和gRPC集成的模块。 用户可以通过引入适配器依赖性来快速使用Sentinel,并进行简单的配置。 如果您以前使用过Spring Cloud Netflix,则可以考虑迁移到Spring Cloud Alibaba 。
动态配置
Sentinel为动态规则管理提供了动态规则数据源支持。 Sentinel提供的ReadableDataSource
和WritableDataSource
接口易于使用。
Sentinel动态规则数据源提供了扩展模块,可与流行的配置中心和远程存储集成。 当前,它支持许多动态规则源,例如Nacos,ZooKeeper,Apollo和Redis,它们可以涵盖许多生产场景。
(赵一豪赵奕豪原创)
阿里巴巴科技
关于阿里巴巴最新技术的第一手和深入信息→Facebook: “阿里巴巴技术” 。 Twitter: “阿里巴巴技术” 。