micrometer
在上一个博客中,我们使用由InfluxDB支持的Micrometer设置了React式应用程序。
在本教程中,我们将使用传统的带有JDBC的基于Servlet的阻塞Spring堆栈。 我选择的数据库是postgresql。 我将使用与先前博客文章相同的脚本。
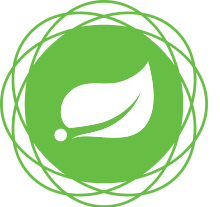
因此,我们将拥有初始化数据库的脚本
#!/bin/bash set -e psql -v ON_ERROR_STOP=1 --username "$POSTGRES_USER" --dbname "$POSTGRES_DB" <<-EOSQL
create schema spring_data_jpa_example;
create table spring_data_jpa_example.employee(
id SERIAL PRIMARY KEY ,
firstname TEXT NOT NULL ,
lastname TEXT NOT NULL ,
email TEXT not null ,
age INT NOT NULL ,
salary real ,
unique (email)
);
insert into spring_data_jpa_example.employee (firstname,lastname,email,age,salary)
values ( 'John' , 'Doe 1' , 'john1@doe.com' ,18,1234.23);
insert into spring_data_jpa_example.employee (firstname,lastname,email,age,salary)
values ( 'John' , 'Doe 2' , 'john2@doe.com' ,19,2234.23);
insert into spring_data_jpa_example.employee (firstname,lastname,email,age,salary)
values ( 'John' , 'Doe 3' , 'john3@doe.com' ,20,3234.23);
insert into spring_data_jpa_example.employee (firstname,lastname,email,age,salary)
values ( 'John' , 'Doe 4' , 'john4@doe.com' ,21,4234.23);
insert into spring_data_jpa_example.employee (firstname,lastname,email,age,salary)
values ( 'John' , 'Doe 5' , 'john5@doe.com' ,22,5234.23); EOSQL
我们将拥有一个包含InfluxDB,Postgres和Grafana的docker compose文件。
version: '3.5' services:
influxdb:
image: influxdb
restart: always
ports:
- 8086 : 8086
grafana:
image: grafana / grafana
restart: always
ports:
- 3000 : 3000
postgres:
image: postgres
restart: always
environment:
POSTGRES_USER: db - user
POSTGRES_PASSWORD: your - password
POSTGRES_DB: postgres
ports:
- 5432 : 5432
volumes:
- $PWD / init - db - script.sh: / docker - entrypoint - initdb.d / init - db - script.sh
现在是时候从我们的maven依赖关系开始构建我们的spring应用程序了。
<? xml version = "1.0" encoding = "UTF-8" ?> < project xmlns = " http://maven.apache.org/POM/4.0.0 " xmlns:xsi = " http://www.w3.org/2001/XMLSchema-instance " xsi:schemaLocation = " http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd " >
< modelVersion >4.0.0</ modelVersion >
< parent >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-starter-parent</ artifactId >
< version >2.2.4.RELEASE</ version >
</ parent >
< groupId >com.gkatzioura</ groupId >
< artifactId >EmployeeApi</ artifactId >
< version >1.0-SNAPSHOT</ version >
< build >
< defaultGoal >spring-boot:run</ defaultGoal >
< plugins >
< plugin >
< groupId >org.apache.maven.plugins</ groupId >
< artifactId >maven-compiler-plugin</ artifactId >
< configuration >
< source >8</ source >
< target >8</ target >
</ configuration >
</ plugin >
< plugin >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-maven-plugin</ artifactId >
</ plugin >
</ plugins >
</ build >
< dependencies >
< dependency >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-starter-web</ artifactId >
</ dependency >
< dependency >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-starter-data-jpa</ artifactId >
</ dependency >
< dependency >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-starter-actuator</ artifactId >
</ dependency >
< dependency >
< groupId >org.postgresql</ groupId >
< artifactId >postgresql</ artifactId >
< version >42.2.8</ version >
</ dependency >
< dependency >
< groupId >io.micrometer</ groupId >
< artifactId >micrometer-core</ artifactId >
< version >1.3.2</ version >
</ dependency >
< dependency >
< groupId >io.micrometer</ groupId >
< artifactId >micrometer-registry-influx</ artifactId >
< version >1.3.2</ version >
</ dependency >
< dependency >
< groupId >org.projectlombok</ groupId >
< artifactId >lombok</ artifactId >
< version >1.18.12</ version >
< scope >provided</ scope >
</ dependency >
</ dependencies > </ project >
由于这是JDBC支持的依赖关系,因此我们将创建实体和存储库。
package com.gkatzioura.employee.model; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.Table; import lombok.Data; @Data @Entity @Table (name = "employee" , schema= "spring_data_jpa_example" ) public class Employee {
@Id
@Column (name = "id" )
@GeneratedValue (strategy = GenerationType.IDENTITY)
private Long id;
@Column (name = "firstname" )
private String firstName;
@Column (name = "lastname" )
private String lastname;
@Column (name = "email" )
private String email;
@Column (name = "age" )
private Integer age;
@Column (name = "salary" )
private Integer salary; }
然后让我们添加存储库
package com.gkatzioura.employee.repository; import com.gkatzioura.employee.model.Employee; import org.springframework.data.jpa.repository.JpaRepository; public interface EmployeeRepository extends JpaRepository<Employee,Long> { }
和控制器
package com.gkatzioura.employee.controller; import java.util.List; import com.gkatzioura.employee.model.Employee; import com.gkatzioura.employee.repository.EmployeeRepository; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; @RestController public class EmployeeController {
private final EmployeeRepository employeeRepository;
public EmployeeController(EmployeeRepository employeeRepository) {
this .employeeRepository = employeeRepository;
}
@RequestMapping ( "/employee" )
public List<Employee> getEmployees() {
return employeeRepository.findAll();
} }
最后但并非最不重要的Application类
package com.gkatzioura.employee; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class Application {
public static void main(String[] args) {
SpringApplication.run(Application. class , args);
} }
以及配置
spring:
datasource:
platform: postgres
driverClassName: org.postgresql.Driver
username: db - user
password: your - password
url: jdbc:postgresql: / / 127.0 . 0.1 : 5432 / postgres management:
metrics:
export:
influx:
enabled: true
db: employeeapi
uri: http: / / 127.0 . 0.1 : 8086
endpoints:
web:
expose: "*"
试试看
curl http: //localhost :8080 /employee
经过一些请求后,我们可以找到保留的条目。
docker exec -it influxdb- local influx > SHOW DATABASES; name: databases name ---- _internal employeeapi > use employeeapi Using database employeeapi > SHOW MEASUREMENTS name: measurements name ---- hikaricp_connections hikaricp_connections_acquire hikaricp_connections_active hikaricp_connections_creation hikaricp_connections_idle hikaricp_connections_max hikaricp_connections_min hikaricp_connections_pending hikaricp_connections_timeout hikaricp_connections_usage http_server_requests jdbc_connections_active jdbc_connections_idle jdbc_connections_max jdbc_connections_min jvm_buffer_count jvm_buffer_memory_used jvm_buffer_total_capacity jvm_classes_loaded jvm_classes_unloaded jvm_gc_live_data_size jvm_gc_max_data_size jvm_gc_memory_allocated jvm_gc_memory_promoted jvm_gc_pause jvm_memory_committed jvm_memory_max jvm_memory_used jvm_threads_daemon jvm_threads_live jvm_threads_peak jvm_threads_states logback_events process_cpu_usage process_files_max process_files_open process_start_time process_uptime system_cpu_count system_cpu_usage system_load_average_1m tomcat_sessions_active_current tomcat_sessions_active_max tomcat_sessions_alive_max tomcat_sessions_created tomcat_sessions_expired tomcat_sessions_rejected
如您所见,指标与上一个示例有些不同。 我们有jdbc连接度量标准tomcat度量标准和与我们的应用程序相关的所有度量标准。 您可以在github上找到源代码。
micrometer