贪吃蛇游戏的组成部分
1.食物
2.蛇
3.地图
创建这些部分
1.创建地图
地图部分没有弄的很美观,比较简单,结构如下:
<html>
<style>
#map{
box-sizing: border-box;
width: 800px;
height: 600px;
background-color: black;
margin: 50px auto 0;
position: relative;
border: 1px solid red;
}
</style>
<body>
<!-- 地图-->
<div id=map></div>
</body>
</html>
2.创建食物
食物有颜色、宽度、高度。
食物的位置是随机产生的,所以会用到随机数。
我们可以通过 js实现食物的创建
((function() {
// 1.自定义食物的构造函数
function Food(width, height, color, x, y) {
this.width = width || 20;
this.height = height || 20;
this.color = color || "pink";
this.x = x || 0;
this.y = y || 0;
this.element = document.createElement("div");
}
// 2.在原型对象上初始化食物
Food.prototype.init = function(map) {
// 设置小方块的样式
var div = this.element;
div.style.width = this.width + "px";
div.style.height = this.height + "px";
div.style.backgroundColor = this.color;
div.style.position = "absolute";
// 设置随机坐标
this.x = Math.floor(Math.random() * (map.offsetWidth / this.width)) * this.width;
this.y = Math.floor(Math.random() * (map.offsetHeight / this.height)) * this.height;
// 设置小方块的left和top值
this.element.style.left = this.x + "px";
this.element.style.top = this.y + "px";
// ② 把小方块添加到map中
map.appendChild(div);
}
// 3.Food暴露给window
window.Food = Food;
})());
3.创建小蛇
(1)小蛇初始有一个脑袋和两段身体、头部和身体颜色不一样。每一截的width、height相同。小蛇会移动,还要设置方向direction 。
var elements = [];// 存放蛇的部位
function Snake(width, height, direction) {
// 小蛇每一个部分的宽高
this.width = width || 20;
this.height = height || 20;
// 方向
this.direction = direction || "right";
// 小蛇的身体
this.body = [
{x: 3, y: 1, color: "red"},// 小蛇的头
{x: 2, y: 1, color: "orange"},// 小蛇的身体
{x: 1, y: 1, color: "orange"}// 小蛇的身体
]
}
// 2.在原型对象上初始化小蛇
Snake.prototype.init = function(map) {
// 先删除再创建
remove();
// 遍历创建div
for(var i = 0; i < this.body.length; i++) {
// 每一部位的刻度
var obj = this.body[i];
// 创建div并且添加样式
var div = document.createElement("div");
div.style.width = this.width + "px";
div.style.height = this.height + "px";
div.style.backgroundColor = obj.color;
div.style.position = "absolute";
// 设置坐标
div.style.left = obj.x * this.width + "px";
div.style.top = obj.y * this.height + "px";
map.appendChild(div);
// 把蛇添加到数组里--->目的:为了删除
elements.push(div);
}
}
(2)小蛇移动的方法:
根据小蛇头部的方向移动,每次移动,给小蛇头部一个新的坐标,将小蛇头部原来的坐标给第一段身体,原来的第一段的坐标给第二段…依次下去
当吃到食物,移出食物时,给蛇的身体添加一段,并刷新食物的位置
Snake.prototype.move = function(food, map) {
// 改变小蛇身体的坐标,小蛇的头部去判断方向
for(var i = this.body.length - 1; i > 0; i--) {
// 当i = 2时,让第三块坐标x = 第二块坐标x
this.body[i].x = this.body[i - 1].x;
this.body[i].y = this.body[i - 1].y;
}
// 判断小蛇头部的坐标
switch(this.direction) {
case "right":
this.body[0].x++;
break;
case "left":
this.body[0].x--;
break;
case "top":
this.body[0].y--;
break;
case "bottom":
this.body[0].y++;
break;
}
// 判断小蛇是否吃到食物--->即判断头部坐标和食物的坐标一致
// 头部的坐标
var headX = this.body[0].x * this.width;
var headY = this.body[0].y * this.height;
// 食物的坐标
var foodX = food.x;
var foodY = food.y;
if(headX == foodX && headY == foodY) {
// 追加一个蛇的身体到body最后
var last = this.body[this.body.length - 1];// 复制小蛇的尾巴
// 添加到数组
this.body.push({
x: last.x,
y: last.y,
color: last.color
})
// 食物吃完了要刷新位置
food.init(map);
}
}
每一次移动后都要清除,之前的轨迹,不然就会蛇就会从出生点的位置,一直延长,就像下图
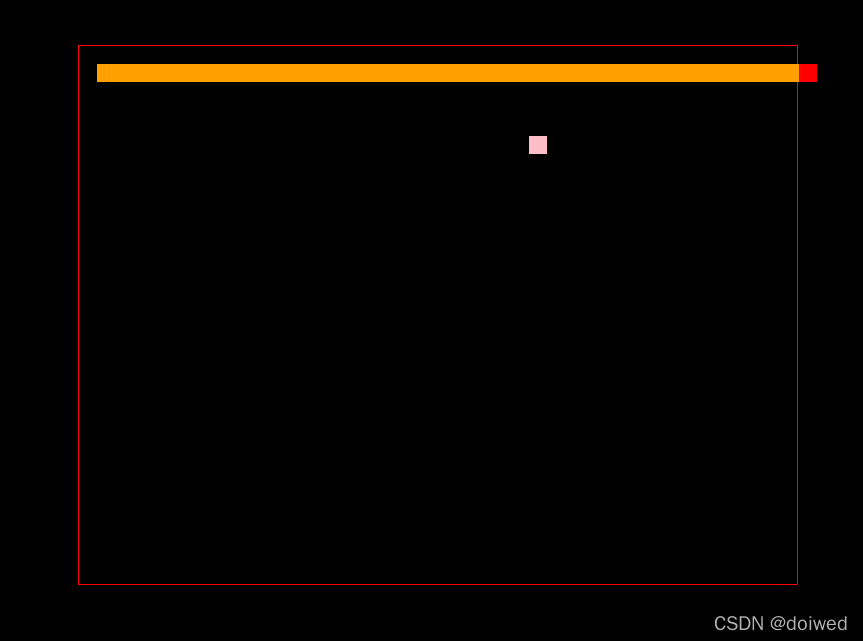
而正常的蛇,是先清除原来位置的身体,再在新的位置创建蛇,这里就会添加一个新的删除蛇的操作
function remove() {
// 先删尾巴
for(var i = elements.length - 1; i >= 0; i--) {
// 数组中的每一个部位
var ele = elements[i];
// 在map中删除div
ele.parentNode.removeChild(ele);
// 删数组的div
elements.splice(i, 1);
}
}
最后将小蛇暴漏给window
window.Snake = Snake;
4创建游戏条件
1.小蛇碰到墙壁,就会game over。
2.小蛇不能碰到自己的身体,碰到就会game over
3.设置按键,点击按键小蛇就会移动
4.当小蛇往左时,按往相反的方向失灵
这里我们创建一个Game对象,把之前创建好的小蛇和食物引入进来。
// 四、游戏对象
((function() {
var that;
// 1.自定义游戏的构造函数
function Game(map) {
this.food = new Food();// 食物对象
this.snake = new Snake();// 小蛇对象
this.map = map;
that = this;
}
// 2.初始化游戏
Game.prototype.init = function() {
this.food.init(this.map);
this.snake.init(this.map);
// 调用
this.runSnake(this.map);
// 调用按键方法
this.bindKey();
}
// 3.添加方法使小蛇自动跑起来
Game.prototype.runSnake = function(map) {
var timeId = setInterval(function() {
that.snake.move(that.food, that.map);
that.snake.init(map);
// 判断横纵坐标的最大最小值
var maxX = map.offsetWidth / that.snake.width;
var maxY = map.offsetHeight / that.snake.height;
// 蛇头的坐标
var headX = that.snake.body[0].x;
var headY = that.snake.body[0].y;
// 不能碰墙
if(headX < 0 || headX >= maxX || headY < 0 || headY >= maxY) {
// 清除定时器
clearInterval(timeId);
alert("你可真是菜死了!");
}
// 不能吃自己的身体
for(var i = 1; i < that.snake.body.length; i++){
if(headX == that.snake.body[i].x && headY == that.snake.body[i].y) {
// 清除定时器
clearInterval(timeId);
alert("吃自己可还行?你可真是菜死了!");
}
}
}, 200)
}
// 4.设置用户的按键,来改变蛇移动的方向
Game.prototype.bindKey = function() {
document.addEventListener("keydown", function(e) {
// console.log(e.keyCode);
switch(e.keyCode) {
case 37:
that.snake.direction = that.snake.direction == "right" ? "right" : "left";
break;
case 38:
that.snake.direction = that.snake.direction == "bottom" ? "bottom" : "top";
break;
case 39:
that.snake.direction = that.snake.direction == "left" ? "left" : "right";
break;
case 40:
that.snake.direction = that.snake.direction == "top" ? "top" : "bottom";
break;
}
})
}
window.Game = Game;
})());
var map = document.getElementById("map");
var game = new Game(map);
game.init();
做的有些简陋
链接:https://pan.baidu.com/s/1V__LA1YA-mBZ-23zOhEOkg
提取码:2han