平时项目中用到的,记录下来(持续更新)。
1.在导航栏右边添加多个UIBarButtonItem
UIBarButtonItem *searchScheduleBtn = [[UIBarButtonItem alloc] initWithImage:[UIImage imageNamed:@"search_small"] style:UIBarButtonItemStylePlain target:self action:@selector(clickSearchSchedule)];
UIBarButtonItem *searchPersonBtn = [[UIBarButtonItem alloc] initWithImage:[UIImage imageNamed:@"search_small"] style:UIBarButtonItemStylePlain target:self action:@selector(clickSearchPerson)];
NSArray *btnArr = @[searchScheduleBtn,searchPersonBtn];
self.navigationItem.rightBarButtonItems = btnArr;
2.字符串分割
NSString *str = @"hello world !";
NSArry *arr = [str componentsSeparatedByString:@" "];
上面代码以空格作为分割依据,得到一个的count为3的arr,arr[0]-arr[2]分别为hello、world、!
3.数组合并成一个字符串:
NSArray *pathArray = [NSArray arrayWithObjects:@"hello",@"world", @"!", nil];
NSString *str = [pathArray componentsJoinedByString:@" "];
上面使用空格来连接(即componentsJoinedByString的参数),合并后str的内容为hello world !
4.拨打电话的三种方法:
(1)NSURL *url = [NSURL URLWithString:@"tel://10086"];
[[UIApplication sharedApplication] openURL:url];
(2)//拨打前弹框提醒、通话结束后回到应用,但是是私有api
NSURL *url = [NSURL @"telprompt://10086"];
[[UIApplication sharedApplication] openURL:url];
(3)通过UIWebView
[WebView loadRequest:[NSURLRequest requestWithURL:[NSURL URLWithString:@"tel://10086"]]];
5.使得NavigationBar上按钮的图片颜色为原图颜色
添加到NavigationBar上的按钮的图片系统默认会显示为蓝色,如果要显示图片本身的颜色,只需要在选中图片,修改图中Render As的值为Original Image即可
6.给任意UIView设置圆角效果:
self.selectBtn1.layer.cornerRadius = 2.f;
self.selectBtn1.clipsToBounds = YES;
以上代码给selectBtn1按钮设置圆角大小为2.0的圆角效果,更多效果:http://blog.csdn.net/dolacmeng/article/details/45459145
7.在NSArry/NSMutableArry中存放int、bool等基本数据类型,只需包装为NSNumber
NSArray *arr1 = @[@"string",@YES,@100];//方法1
NSArray *arr2 = @[[NSNumber numberWithInt:100],[NSNumber numberWithBool:NO]];//方法2
8.导航栏添加自定义试图
UIBarButtonItem *item = [[UIBarButtonItem alloc] initWithCustomView:customView;//其中customView为用户自定义的View,可以是UIButton、UILabel、UISwitch等等或者它们的组合
self.navigationItem.leftBarButtonItems = item;
9.给任意UIView子类实例添加点击事件
UITapGestureRecognizer *tap = [[UITapGestureRecognizer alloc]init];
[tap addTarget:self action:@selector(clickMyView)];
[myView addGestureRecognizer:tap];
10.给UILabel添加下划线(UIButton同理,获取button.titleLabel即可)
UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(0, 0, 200, 30)];
NSMutableAttributedString *content = [[NSMutableAttributedString alloc] initWithString:@"这是加了下划线的文字"];
NSRange contentRange = {0, [content length]};
[content addAttribute:NSUnderlineStyleAttributeName value:[NSNumber numberWithInteger:NSUnderlineStyleSingle] range:contentRange];
label.attributedText = content;
[self.view addSubview:label];
11.从NSString得到对应的Class
NSString *classStr = @"MyClass";
Class c = NSClassFromString(classStr);
12.加速CocoaPods install速度
如果使用CocoaPods来添加第三方类库,执行pod install或pod update都卡在了Analyzing dependencies不动,可以分别使用以下命令如下:
pod install --verbose --no-repo-update
pod update --verbose --no-repo-update
原因在于当执行以上加了参数的命令后,可以省略升级CocoaPods的spec仓库这一步,然后速度就会提升不少。
13.查看APPStore平均审核时间(打开速度比较慢,推荐使用vpn)
14.命令行启动Mac自带Apache服务器
sudo apachectl -k start
15.监听UITextfield编辑事件(开始、结束、字符改变)
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(textFieldTextDidChangeOneCI:) name:UITextFieldTextDidChangeNotification object:myTextfield];
以上代码接收UITextField实例myTextfield的字符改变事件,当文字改变时调用textFieldTextDidChangeObeCI:方法。最后一个参数为nil时,代表接受所有UITextField的事件,倒数第二个参数是事件名称,如UITextFieldTextDidBeginEditingNotification、UITextFieldTextDidEndEditingNotification、UITextFieldTextDidChangeNotification等
16.隐藏键盘
(1) [[UIApplication sharedApplication] sendAction:@selector(resignFirstResponder) to:nil from:nil forEvent:nil];
(2)[self.view endEditing:YES];
17.复制文字到系统粘贴板
UIPasteboard *pasteboard = [UIPasteboard generalPasteboard];
pasteboard.string = @"这里是要复制的文字";
18.去除UITableView多余的分割线
<pre name="code" class="objc"> self.tblExpandable.tableFooterView = [[UIView alloc] initWithFrame:CGRectZero];
19. 使用KVC将json转模型时,如果json存在模型没有的字段,会crash,报setValue:forUndefinedKey错误,只需在模型.m文件中重写对应的方法即可
- (void)setValue:(id)value forUndefinedKey:(NSString *)key {
NSLog(@"key = %@, value = %@", key, value);
}
20.Github .md使用网络图片:
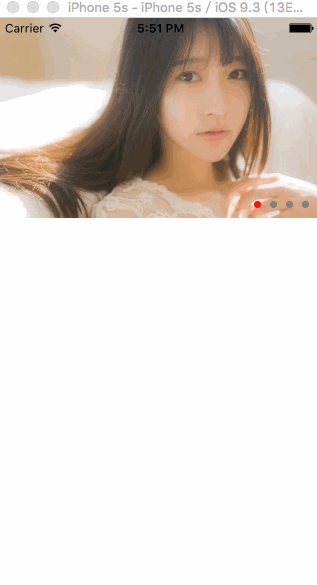
21.设置textfiled的placeholder字体颜色
//方法1
UIColor *color = [UIColor whiteColor];
_userName.attributedPlaceholder = [[NSAttributedString alloc] initWithString:@"用户名" attributes:@{NSForegroundColorAttributeName: color}];
//方法2 (kvc)
[_userName setValue:[UIColor whiteColor] forKeyPath:@"_placeholderLabel.textColor"];
22通过runtime获取某个类的所有属性(如UIGestureRecognizer)
UInt32 count;
Ivar *ivars = class_copyIvarList([UIGestureRecognizer class], &count);
for (int i=0; i<count; i++) {
NSString *name = [[NSString alloc] initWithCString:ivar_getName(ivars[i]) encoding:NSUTF8StringEncoding];
NSLog(@"%@",name);
}
23.上传git时,分割大文件、合并文件
To split file named <inputfilename>:
split -b50m inputfilename
To put the files back together, in the terminal simply type:
cat x* > outputfilename