对比
1配置项
3.0 config文件已经被移除,但是多了.env.production和env.development文件,除了文件位置,实际配置起来和2.0没什么不同
没了config文件,跨域需要配置域名时,从config/index.js 挪到了vue.config.js中,配置方法不变
2.渲染
Vue2.x使用的Virtual Dom实现的渲染
Vue3.0不论是原生的html标签还是vue组件,他们都会通过h函数来判断,如果是原生html标签,在运行时直接通过Virtual Dom来直接渲染,同样如果是组件会直接生成组件代码
3.数据监听
Vue2.x大家都知道使用的是es5的object.defineproperties中getter和setter实现的,而vue3.0的版本,是基于Proxy进行监听的,其实基于proxy监听就是所谓的lazy by default,什么意思呢,就是只要你用到了才会监听,可以理解为‘按需监听’,官方给出的诠释是:速度加倍,同时内存占用还减半。
4.按需引入
Vue2.x中new出的实例对象,所有的东西都在这个vue对象上,这样其实无论你用到还是没用到,都会跑一变。而vue3.0中可以用ES module imports按需引入,如:keep-alive内置组件、v-model指令,等等。
5.对比与注意
-
3.0比2.0 快2倍
-
3.0去掉了filter, 么有beforeCreate created,用setup取代
-
reactivity是可以单独作为库使用的
-
单独功能可以抽离 取代了mixin 优于mixin 解决上下反复横跳
-
支持多个子节点 fragment
-
setup里没有this
-
Proxy实现响应式不需要set delete 兼容性并不好
-
响应式方面 性能得到很大提升 不用初始化的时候就递归遍历属性
-
响应式不区分数组和对象
-
3.0兼容IE12以上
-
composition api 可以和 options API 同时存在
作者:前端大镖客_
链接:https://www.jianshu.com/p/deec63c85360
来源:简书
著作权归作者所有。商业转载请联系作者获得授权,非商业转载请注明出处。
$: Object
$attrs: Proxy
$data: Proxy
$el: div.hello
$emit: ƒ ()
$forceUpdate: () => queueJob(i.update)
$nextTick: ƒ nextTick(fn)
$options: Object
$parent: Proxy
$props: Proxy
$refs: Object
$root: Proxy
$slots: Proxy
$watch
provide/inject
在这个版本上提供了依赖注入,在上级组件provide 提供注入选项,在需要注入变量的组件inject 注入。话不多说上代码。
const app = Vue.createApp({})
app.component('todo-list', {
data() {
return {
todos: ['Feed a cat', 'Buy tickets']
}
},
provide: {
user: 'John Doe'
},
template: `
<div>
{{ todos.length }}
<!-- rest of the template -->
</div>
`
})
app.component('todo-list-statistics', {
inject: ['user'],
created() {
console.log(`Injected property: ${this.user}`) // > Injected property: John Doe
}
})
Async Components
在大型应用程序中,我们可能需要将应用程序分成更小的块,并且只在需要时从服务器加载组件。为了实现这一点,Vue有一个defineAsyncComponent
const app = Vue.createApp({})
const AsyncComp = Vue.defineAsyncComponent(
() =>
new Promise((resolve, reject) => {
resolve({
template: '<div>I am async!</div>'
})
})
)
app.component('async-example', AsyncComp)
import { createApp, defineAsyncComponent } from 'vue'
createApp({
// ...
components: {
AsyncComponent: defineAsyncComponent(() =>
import('./components/AsyncComponent.vue')
)
}
})
teleport
当使用初始HTML结构内该组件,我们可以看到一个问题-模态正在呈现内的深度嵌套div和position: absolute模态的花费相对定位父div作为参考。
Teleport提供了一种干净的方法,使我们可以控制要在DOM中哪个父对象下呈现HTML,而不必求助于全局状态或将其拆分为两个部分。
app.component('modal-button', {
template: `
<button @click="modalOpen = true">
Open full screen modal! (With teleport!)
</button>
<teleport to="body">
<div v-if="modalOpen" class="modal">
<div>
I'm a teleported modal!
(My parent is "body")
<button @click="modalOpen = false">
Close
</button>
</div>
</div>
</teleport>
`,
data() {
return {
modalOpen: false
}
}
})
Reactive
Reactive 提供了一个proxy 对象
定义 Reactive state
// 1. 返回的proxy
import { reactive } from 'vue'
// reactive state
const state = reactive({
count: 0
})
// 2.返回RefImpl
import { ref } from 'vue'
const count = ref(0)
// 3.例子
<template>
<div>
<span>{{ count }}</span>
<button @click="count ++">Increment count</button>
</div>
</template>
<script>
import { ref } from 'vue'
export default {
setup() {
const count = ref(0)
return {
count
}
}
}
</script>
// 4.readonly 可以设置响应式数据只读
import { reactive, readonly } from 'vue'
const original = reactive({ count: 0 })
const copy = readonly(original)
// mutating original will trigger watchers relying on the copy
original.count++
// mutating the copy will fail and result in a warning
copy.count++ // 只读 warning: "Set operation on key 'count' failed: target is readonly."
toRef
为 reactive 对象的属性创建一个 ref
setup() {
const user = reactive({ age: 1 });
const age = toRef(user, "age");
age.value++;
console.log(user.age); // 2
user.age++;
console.log(age.value); // 3
}
toRefs - 解构响应式对象数据
把一个响应式对象转换成普通对象,该普通对象的每个 property 都是一个 ref ,和响应式对象 property 一一对应。
当想要从一个组合逻辑函数中返回响应式对象时,用 toRefs 是很有效的,该 API 让消费组件可以 解构 / 扩展(使用 … 操作符)返回的对象,并不会丢失响应性:
### setup
> 替代created
生命周期
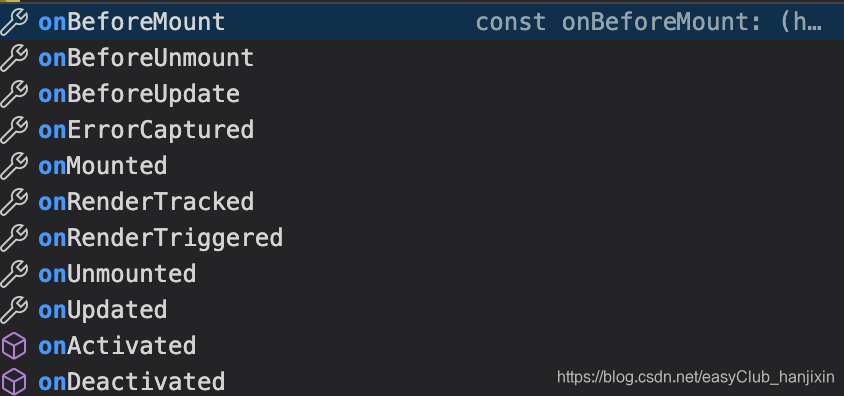
//todo
## [边缘处理](https://v3.vuejs.org/guide/component-edge-cases.html#forcing-an-update)
1.强制更新 $forceUpdate。
2.静态缓存 v-once
> 在Vue中,呈现纯HTML元素的速度非常快,但是有时您可能会拥有一个包含大量静态内容的组件。在这些情况下,可以通过将v-once指令添加到根元素来确保仅对它求值一次,然后对其进行缓存,如下所示:
```javascript
app.component('terms-of-service', {
template: `
<div v-once>
<h1>Terms of Service</h1>
... a lot of static content ...
</div>
`
})