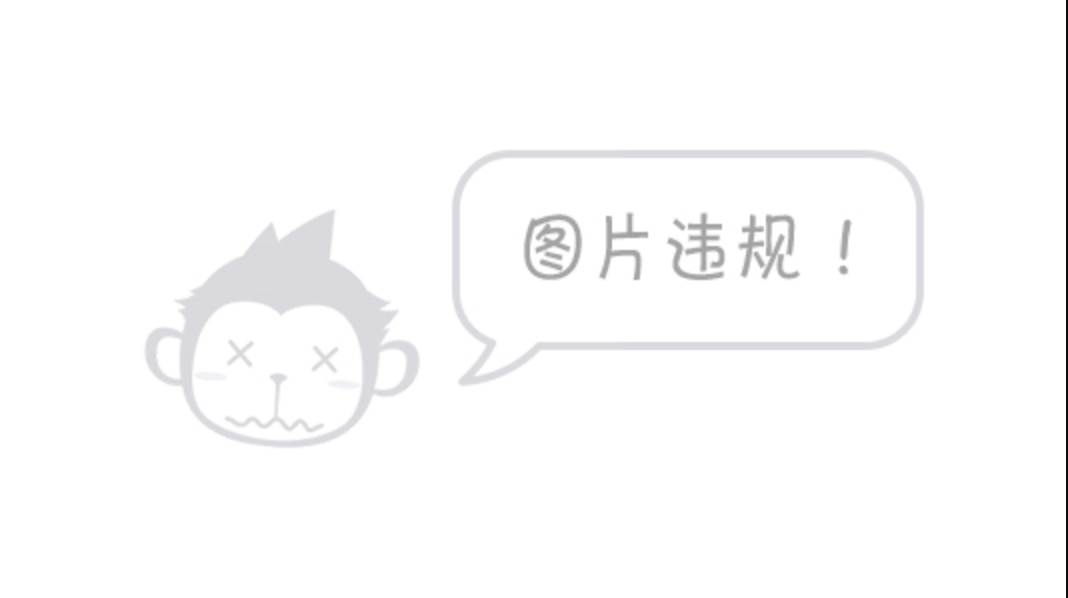
trait说明
- 为enum实现Display接口后,可以通过to_string获取到String类型的enum值
- 为String实现From<Platform>,可以在调用into函数将enum转为String
- 同理,为enum实现From<String>,也可以将String转为enum
使用例子
use core::fmt::{Display, Formatter, Result};
#[derive(Debug)]
enum Platform {
Linux,
MacOS,
Windows,
Unknown,
}
impl Display for Platform {
fn fmt(&self, f: &mut Formatter) -> Result {
match self {
Platform::Linux => write!(f, "Linux"),
Platform::MacOS => write!(f, "MacOS"),
Platform::Windows => write!(f, "Windows"),
Platform::Unknown => write!(f, "unknown"),
}
}
}
impl From<Platform> for String {
fn from(platform: Platform) -> Self {
match platform{
Platform::Linux => "Linux".into(),
Platform::MacOS => "MacOS".into(),
Platform::Windows => "Windows".into(),
Platform::Unknown => "unknown".into(),
}
}
}
impl From<String> for Platform{
fn from(platform: String) -> Self {
match platform {
platform if platform == "Linux" => Platform::Linux,
platform if platform == "MacOS" => Platform::MacOS,
platform if platform == "Windows" => Platform::Windows,
platform if platform == "unknown" => Platform::Unknown,
_ => Platform::Unknown,
}
}
}
fn main(){
let platform: String = Platform::MacOS.to_string();
println!("{}", platform);
let platform: String = Platform::Linux.into();
println!("{}", platform);
let platform: Platform = "Windows".to_string().into();
println!("{}", platform);
}