1、前台:
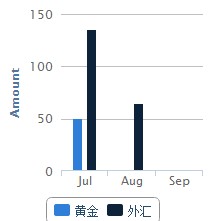
<
div
id
="container"
style
="
min-width
:
220px
;
min-height
:
300px
;
margin
:
0
auto"></
div
>
<
script
src
="script/jquery-1.7.2.min.js"
type
="text/javascript"></
script
>
<
script
src
="script/highcharts/highcharts.js"
type
="text/javascript"></
script
>
<
script
src
="script/highcharts/modules/exporting.js"
type
="text/javascript"></
script
>
<
script
type
="text/javascript">
$(
function
() {
var
analystId=$(
"#analystID"
).val();
var
month=3;
$.ajax({
url:
"/highchartsTable.ashx?id="
+ analystId +
"&month="
+month ,
type:
"POST"
,
dataType:
"json"
,
//cache: false,
success:
function
(json) {
var
aryMonths =
new
Array(
"Jan"
,
"Feb"
,
"Mar"
,
"Apr"
,
"May"
,
"Jun"
,
"Jul"
,
"Aug"
,
"Sep"
,
"Oct"
,
"Nov"
,
"Dec"
);
var
now =
new
Date();
var
nowMonth = now.getMonth();
var
lastYear=now.getFullYear()-1;
var
arrTemp=
new
Array(month);
for
(
var
i = month-1; i >=0; i--) {
if
(i < nowMonth){
arrTemp[month-i-1] = aryMonths[nowMonth-i-1];
}
else
if
(i==nowMonth){
arrTemp[0] = aryMonths[nowMonth] +
"<br/>("
+lastYear+
")"
;
}
else
if
(i > nowMonth){
arrTemp[i-nowMonth] = aryMonths[i] +
"<br/>("
+lastYear+
")"
;
}
}
$(
'#container'
).highcharts({
chart: {
type:
'column'
},
legend:{
layout:
"horizontal"
,
floating:
false
},
title: {
text:
'Analyst investment<br/> profit/loss statistics '
,
y:10
},
subtitle: {
text:
'Source: www.highcharts.com'
,
y:50
},
xAxis: {
categories:arrTemp
// [
// 'Jan',
// 'Feb',
// 'Mar',
// 'Apr',
// 'May',
// 'Jun',
// 'Jul',
// 'Aug',
// 'Sep',
// 'Oct',
// 'Nov',
// 'Dec'
// ]
},
yAxis: {
min: 0,
title: {
text:
'Amount($)'
}
},
tooltip: {
headerFormat:
'<span style="font-size:10px">{point.key}</span><br /><table>'
,
pointFormat:
'<tr><td style="color:{series.color};padding:0">{series.name}: </td>'
+
'<td style="padding:0"><b>{point.y:.1f} $</b></td></tr>'
,
footerFormat:
'</table>'
,
shared:
true
,
useHTML:
true
},
plotOptions: {
column: {
pointPadding: 0.2,
borderWidth: 0
}
},
series:json,
});
},
});
})
</
script
>
2、后台:
///
<sumMonth3y>
///
$codebehindclassname$
的摘要说明
///
</sumMonth3y>
[
WebService
(Namespace =
"http://tempuri.org/"
)]
[
WebServiceBinding
(ConformsTo =
WsiProfiles
.BasicProfile1_1)]
public
class
highchartsTable
:
IHttpHandler
,
IRequiresSessionState
{
private
static
StrategyBiz
biz =
new
StrategyBiz
();
public
void
ProcessRequest(
HttpContext
context)
{
context.Response.ContentType =
"text/plain"
;
string
responseJson =
null
;
string
analystId = context.Request.QueryString[
"id"
];
string
month = context.Request.QueryString[
"month"
];
if
(!
string
.IsNullOrEmpty(analystId) && !
string
.IsNullOrEmpty(month))
{
responseJson = InvestTableJson(
Convert
.ToInt32(analystId),
Convert
.ToInt32(month));
}
context.Response.Write(responseJson);
}
public
bool
IsReusable
{
get
{
return
false
;
}
}
///
<sumMonth3y>
///
判断输入是否为整数类型
///
</sumMonth3y>
///
<param name="s">
待检查数据
</param>
///
<returns></returns>
public
static
bool
IsInt(
string
s)
{
if
(s ==
null
)
{
return
false
;
}
else
{
try
{
int
i =
int
.Parse(s);
return
true
;
}
catch
{
return
false
;
}
}
}
///
<sumMonth3y>
///
InvestTable
获取数据
///
</sumMonth3y>
public
string
InvestTableJson(
int
analystID,
int
months)
{
string
json =
null
;
try
{
int
nowMonth =
DateTime
.Now.Month;
AnalystInvestBiz
biz =
new
AnalystInvestBiz
();
IList
<
StrategyInvest
>[] invesListTemp =
new
List
<
StrategyInvest
>[months];
int
[] amountTemp =
new
int
[months];
IList
<
StrategyInvest
> invesList = biz.GetAllInvestByAnalystID(analystID, months);
//
近
months
个月的数据
IList
<
StrategyInvest
> typeList = biz.GetAllInvestTypeByAnalystID(analystID);
//
找到该分析师下的所有显示投资商品
List
<
InvestTable
> investData =
new
List
<
InvestTable
>();
if
(typeList.Count > 0)
{
for
(
int
j = 0; j < typeList.Count; j++)
{
InvestTable
itab =
new
InvestTable
();
yearData
yearDataTemp =
new
yearData
();
for
(
int
i = months; i > 0; i--)
{
invesListTemp[i - 1] = invesList.Where(c => c.InvestTime >=
DateTime
.Now.AddMonths(-i-1) && c.InvestTime <
DateTime
.Now.AddMonths(-i)).ToList();
amountTemp[i-1] = invesListTemp[i - 1].Count == 0 ? 0 : (invesListTemp[i - 1].Where(c => c.InvestProduct == typeList[j].InvestProduct).ToList().Count != 0 ? invesListTemp[i - 1].Where(c => c.InvestProduct == typeList[j].InvestProduct).First().AmountProfit : 0);
int
m = invesListTemp[i - 1].Count == 0 ? 0 : (invesListTemp[i - 1].Where(c => c.InvestProduct == typeList[j].InvestProduct).ToList().Count != 0 ? invesListTemp[i - 1].Where(c => c.InvestProduct == typeList[j].InvestProduct).First().InvestTime.Month : 0);
if
(m ==
DateTime
.Now.AddMonths(-months).Month)
{
yearDataTemp.Month1 = amountTemp[i - 1].ToString();
}
else
if
(m ==
DateTime
.Now.AddMonths(-(months-1)).Month)
{
yearDataTemp.Month2 = amountTemp[i - 1].ToString();
}
else
if
(m ==
DateTime
.Now.AddMonths(-(months - 2)).Month)
{
yearDataTemp.Month3 = amountTemp[i - 1].ToString();
}
//else if (m == DateTime.Now.AddMonths(-(months - 3)).Month)
//{
// yearDataTemp.Month4 = amountTemp[i - 1].ToString();
//}
//else if (m == DateTime.Now.AddMonths(-(months - 4)).Month)
//{
// yearDataTemp.Month5 = amountTemp[i - 1].ToString();
//}
//else if (m == DateTime.Now.AddMonths(-(months - 5)).Month)
//{
// yearDataTemp.Month6 = amountTemp[i - 1].ToString();
//}
//else if (m == DateTime.Now.AddMonths(-(months - 6)).Month)
//{
// yearDataTemp.Month7 = amountTemp[i - 1].ToString();
//}
//else if (m == DateTime.Now.AddMonths(-(months - 7)).Month)
//{
// yearDataTemp.Month8 = amountTemp[i - 1].ToString();
//}
//else if (m == DateTime.Now.AddMonths(-(months - 8)).Month)
//{
// yearDataTemp.Month9 = amountTemp[i - 1].ToString();
//}
//else if (m == DateTime.Now.AddMonths(-(months - 9)).Month)
//{
// yearDataTemp.Month910 = amountTemp[i - 1].ToString();
//}
//else if (m == DateTime.Now.AddMonths(-(months - 10)).Month)
//{
// yearDataTemp.Month911 = amountTemp[i - 1].ToString();
//}
//else if (m == DateTime.Now.AddMonths(-(months - 11)).Month)
//{
// yearDataTemp.Month912 = amountTemp[i - 1].ToString();
//}
}
itab.yearInvest = yearDataTemp;
itab.InvestProduct = typeList[j].InvestProduct;
int
dataCount = investData.Count;
investData.Insert(dataCount, itab);
}
}
json = JSON<
InvestTable
>(investData);
}
catch
(
ArgumentNullException
e)
{
throw
new
Exception
(e.Message);
}
return
json;
}
public
class
InvestTable
{
///
<sumMonth3y>
///
投资商品
///
</sumMonth3y>
public
string
InvestProduct {
get
;
set
; }
///
<sumMonth3y>
///
一年的数据
///
</sumMonth3y>
public
yearData
yearInvest {
get
;
set
; }
}
public
class
yearData
{
public
string
Month1 {
get
;
set
; }
public
string
Month2 {
get
;
set
; }
public
string
Month3 {
get
;
set
; }
//public string Month4 { get; set; }
//public string Month5 { get; set; }
//public string Month6 { get; set; }
//public string Month7 { get; set; }
//public string Month8 { get; set; }
//public string Month9 { get; set; }
//public string Month910 { get; set; }
//public string Month911 { get; set; }
//public string Month912 { get; set; }
}
///
<sumMonth3y>
///
转换
List
<T>
的数据为
JSON
格式
///
</sumMonth3y>
///
<typeparam name="T">
类
</typeparam>
///
<param name="vals">
列表值
</param>
///
<returns>
JSON
格式数据
</returns>
public
static
string
JSON<T>(
List
<T> vals)
{
string
ResStr =
string
.Empty;
StringBuilder
st =
new
StringBuilder
();
try
{
DataContractJsonSerializer
s =
new
DataContractJsonSerializer
(
typeof
(T));
foreach
(T city
in
vals)
{
using
(
MemoryStream
ms =
new
MemoryStream
())
{
s.WriteObject(ms, city);
st.Append(System.Text.
Encoding
.UTF8.GetString(ms.ToArray()) +
","
);
}
}
ResStr = st.ToString();
if
(ResStr !=
""
)
{
ResStr = ResStr.Substring(0, ResStr.Length - 1);
ResStr = ResStr.Replace(
"\""
,
""
).Replace(
":{"
,
":["
).Replace(
"}}"
,
"]}"
).Replace(
"InvestProduct"
,
"\"name\""
).Replace(
"yearInvest"
,
"\"data\""
).Replace(
"\"name\":"
,
"\"name\":\""
).Replace(
",\"data\""
,
"\",\"data\""
)
.Replace(
"Month1:"
,
""
).Replace(
"Month2:"
,
""
).Replace(
"Month3:"
,
""
).Replace(
"Month4:"
,
""
).Replace(
"Month5:"
,
""
).Replace(
"Month6:"
,
""
).Replace(
"Month7:"
,
""
).Replace(
"Month8:"
,
""
).Replace(
"Month9:"
,
""
).Replace(
"Month910:"
,
""
).Replace(
"Month911:"
,
""
).Replace(
"Month912:"
,
""
).Replace(
"null"
,
"0"
);
ResStr =
"["
+ ResStr +
"]"
;
}
}
catch
(
Exception
ex)
{
throw
ex;
}
return
ResStr;
}
}
3、数据处理层
public
const
String
CSP_STRATEGY_INVEST_ALL_BY_ANALYSTID =
"SELECT [ID],[AnalystID],[InvestProduct],[InvestType],[AmountProfit],[InvestTime],[IsDisplay],[AddTime],[Status] FROM M_T_STRATEGY_INVEST where Status > 0 and AnalystID=@AnalystID and (datediff(month,InvestTime,getdate())>=0 and datediff(month,InvestTime,getdate())<=@month) order by id desc"
;
//
通过分析师
ID
查找其几个月所有投资记录
public
IList
<
StrategyInvest
> GetAllInvestByAnalystID(
int
analystID,
int
month)
{
List
<
StrategyInvest
> list =
new
List
<
StrategyInvest
>();
IDataReader
reader =
null
;
IDataParameter
[] parameters =
{
Helper.GetParameter(
"@AnalystID"
,
DbType
.Int32, analystID) ,
Helper.GetParameter(
"@month"
,
DbType
.Int32, month)
};
try
{
reader = Helper.ExecuteReader(
ConnectionString,
CommandType
.Text,
CSP_STRATEGY_INVEST_ALL_BY_ANALYSTID,
parameters);
while
(reader.Read())
{
StrategyInvest
si =
new
StrategyInvest
();
si.ID =
Int32
.Parse(reader[
"ID"
].ToString());
si.AnalystID =
Int32
.Parse(reader[
"AnalystID"
].ToString());
si.InvestProduct = reader[
"InvestProduct"
].ToString();
si.InvestType = reader[
"InvestType"
].ToString();
si.AmountProfit =
Convert
.ToInt32(reader[
"AmountProfit"
]);
si.InvestTime =
Convert
.ToDateTime(reader[
"InvestTime"
].ToString());
si.IsDisplay =
Convert
.ToBoolean(reader[
"IsDisplay"
]);
si.Status =
Convert
.ToInt32(reader[
"Status"
]);
si.AddTime =
Convert
.ToDateTime(reader[
"AddTime"
].ToString());
list.Add(si);
}
return
list;
}
catch
(
Exception
e)
{
Logger
.Error(
"executing csp_strategy_invest_all_by_analystid failed"
, e);
throw
new
DataAccessException
(
"csp_strategy_invest_all_by_analystid failure"
, e);
}
finally
{
if
(reader !=
null
&& !reader.IsClosed)
{
reader.Close();
}
}
}
4、效果图