FileGDBAPI学习
环境:vs2010 win7_64
- 首先可以在官网上下载对应版本的源码及例子
这里贴出官网下载地址http://appsforms.esri.com/products/download/#File_Geodatabase_API_1.3(下载速度比较慢)
这里我贴出我的环境对应的(点击下载)(密码:e4wl) -
熟悉 并编译例子
下载的压缩包解压后,可以看到如下文件链接:
其中 /bin目录下主要包含我们调用API函数需要的.dll文件 /bin64 64位程序使用
/doc目录下包含完整的api介绍文档,建议详读
/include目录下包含所需的头文件,我们的项目中需要添加引用
/lib目录下主要包含需要使用的库文件
/samples目录下是C++调用API的例子
/samplesC#目录下是C#调用API的例子
OpenTK.dll和OpenTK.GLControl.dll是C#例子中要添加引用的dll(我自己下载源码编译得到的,顺便就放过来了)
用VS2010打开C++解决方案,解决方案如下图:
然后打开项目属性,确认包含目录和库目录是否正确:对应的include目录和lib目录
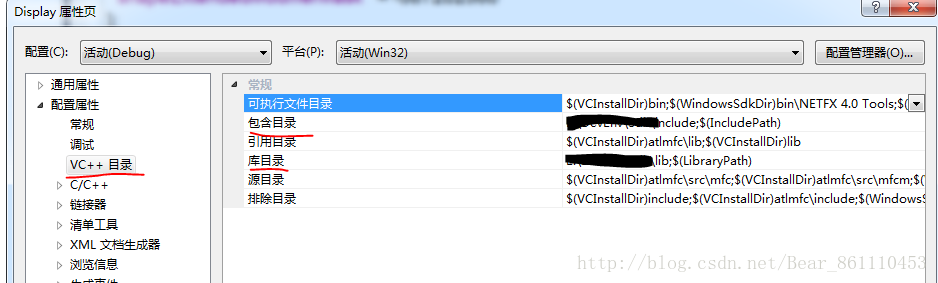
查看项目输出目录:
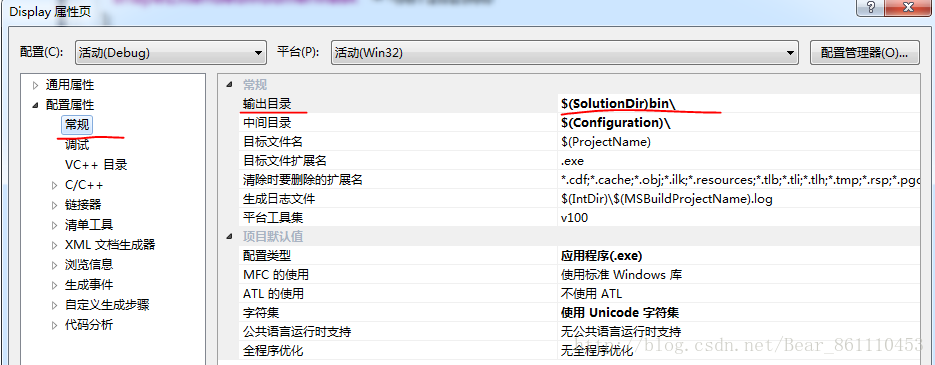
确保上面的提到的dll放入到该输出目录。
然后就可以点击生成并运行:
如果生成成功但运行时报以下错误:
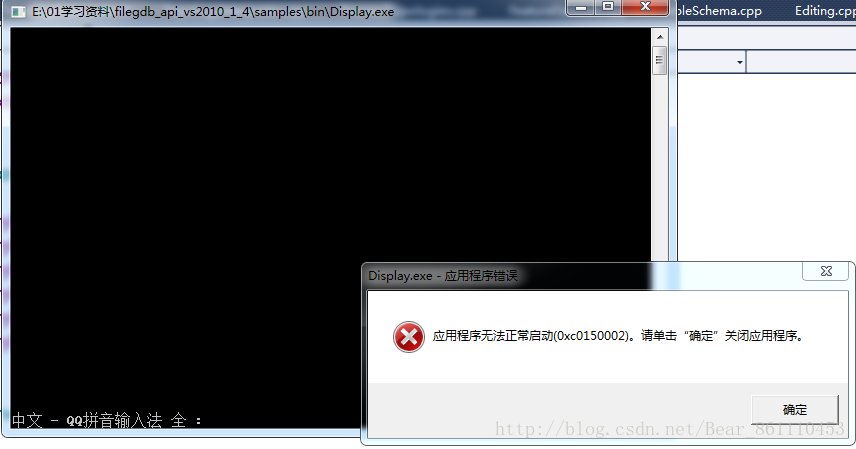
是因为缺少vs2008运行时环境(点击下载),将其放在生成目录下,或者windows下的System32中
运行成功后得到如下结果:
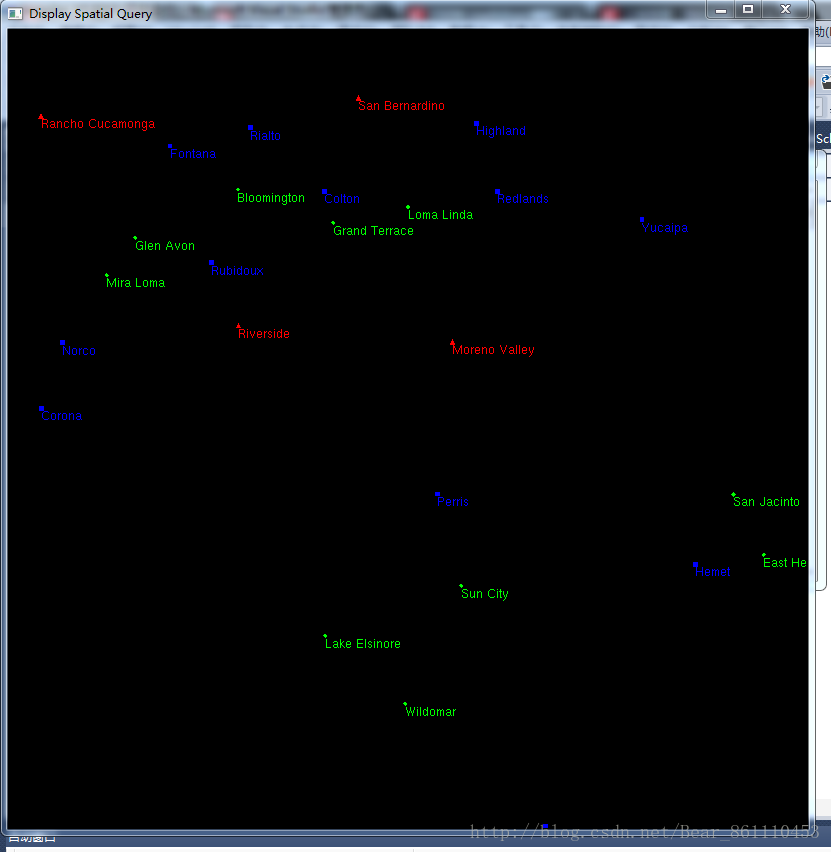
-
利用API开发拷贝功能
这里主要编写了一些简单的Copy功能,可能实现不够完善,希望能够有大神指导。
拷贝整个FileGDB数据库,分两种方式
1.通过文件夹拷贝方式,详情参见
2.通过调用FileGDBAPI所提供的API:
实现比较简单,大致想法是细化问题,先实现拷贝要素函数、利用该函数实现拷贝要素数据集函数,然后利用上述两个函数实现整个数据库的拷贝。
这里贴出源码:
#include <Windows.h>
#include <shellapi.h>
#include <atlstr.h>
#include "FileGDBCopyHelp.h"
#include "FileGDBAPI.h"
using namespace std;
using namespace FileGDBAPI;
int CopyRows(wstring sourceGdbFilePath, wstring targetGdbFIlePath, wstring tablePath)
{
fgdbError hr;
Geodatabase geodatabase;
if ((hr = OpenGeodatabase(sourceGdbFilePath, geodatabase)) != S_OK)
{
wcout << "An error occurred while opening the geodatabase." << endl; return -1;
}
Table theTable;
if ((hr = geodatabase.OpenTable(tablePath, theTable)) != S_OK)
{
wcout << "An error occurred while opening the table." << endl; return -1;
}
EnumRows avQueryResult;
if ((hr = theTable.Search(L"*", L"", false, avQueryResult)) != S_OK)
{
wcout << "An error occurred while performing attribute search." << endl; return -1;
}
Geodatabase geodatabaseT;
if ((hr = OpenGeodatabase(targetGdbFIlePath, geodatabaseT)) != S_OK)
{
wcout << "An error occurred while opening the geodatabase." << endl; return -1;
}
Table theTableT;
vector<FieldDef> fieldDefs;
if((hr = theTable.GetFields(fieldDefs)) != S_OK)
{
wcout << "An error occurred while getting table fields." << endl; return -1;
}
if((hr = geodatabaseT.CreateTable(tablePath, fieldDefs, L"DEFAULTS", theTableT)) != S_OK)
{
wcout << "An error occurred while creating table." << endl; return -1;
}
Row theRow;
while((hr = avQueryResult.Next(theRow)) == S_OK)
{
if ((hr = theTableT.Insert(theRow)) != S_OK)
{
wcout << "An error occurred while inserting a row." << endl; return -1;
}
else
{
wcout << "Inserted S" << endl;
}
}
if ((hr = geodatabaseT.CloseTable(theTableT)) != S_OK)
{
wcout << "An error occurred while closing Cities." << endl; return -1;
}
if ((hr = CloseGeodatabase(geodatabaseT)) != S_OK)
{
wcout << "An error occurred while closing the geodatabase." << endl; return -1;
}
if ((hr = geodatabase.CloseTable(theTable)) != S_OK)
{
wcout << "An error occurred while closing Cities." << endl; return -1;
}
if ((hr = CloseGeodatabase(geodatabase)) != S_OK)
{
wcout << "An error occurred while closing the geodatabase." << endl; return -1;
}
return 0;
}
int CopyDataSet(wstring sourceGdbFilePath, wstring targetGdbFIlePath, wstring dataSetPath, vector<wstring> tablePaths)
{
fgdbError hr;
wstring errorText;
Geodatabase geodatabase;
if ((hr = OpenGeodatabase(sourceGdbFilePath, geodatabase)) != S_OK)
{
wcout << "An error occurred while opening the geodatabase." << endl; return -1;
}
Table theTable;
if ((hr = geodatabase.OpenTable(dataSetPath + tablePaths.back(), theTable)) != S_OK)
{
wcout << "An error occurred while opening the table." << endl; return -1;
}
vector<FieldDef> fieldDefs;
if((hr = theTable.GetFields(fieldDefs)) != S_OK)
{
wcout << "An error occurred while getting the field." << endl; return -1;
}
FieldDef fieldDefGeo;
vector<FieldDef>::iterator iter = fieldDefs.begin();
vector<FieldDef>::iterator iter_end = fieldDefs.end();
for(; iter != iter_end; iter++)
{
FieldDef tempFieldDef = *iter;
FieldType fieldType;
if((hr = tempFieldDef.GetType(fieldType)) != S_OK)
{
wcout << "An error occurred while getting the fieldtype." << endl; return -1;
}
if(fieldType == fieldTypeGeometry)
{
fieldDefGeo = tempFieldDef;
break;
}
}
GeometryDef geometryDef;
if((hr = fieldDefGeo.GetGeometryDef(geometryDef)) != S_OK)
{
wcout << "An error occurred while getting the geometryDef." << endl; return -1;
}
SpatialReference spatialReference;
if((hr = geometryDef.GetSpatialReference(spatialReference)) != S_OK)
{
wcout << "An error occurred while getting the spatialReference." << endl; return -1;
}
Geodatabase geodatabaseT;
if ((hr = OpenGeodatabase(targetGdbFIlePath, geodatabaseT)) != S_OK)
{
wcout << "An error occurred while opening the geodatabase." << endl; return -1;
}
if ((hr = geodatabaseT.CreateFeatureDataset(dataSetPath, spatialReference)) != S_OK)
{
wcout << "An error occurred while creating the feature dataset." << endl; return -1;
}
vector<wstring>::iterator tableIter = tablePaths.begin();
vector<wstring>::iterator tableIter_end = tablePaths.end();
for(; tableIter != tableIter_end; tableIter++)
{
Table theTable;
wstring tempPath = *tableIter;
if ((hr = geodatabase.OpenTable(dataSetPath + tempPath, theTable)) != S_OK)
{
wcout << "An error occurred while opening the table." << endl; return -1;
}
EnumRows avQueryResult;
if ((hr = theTable.Search(L"*", L"", false, avQueryResult)) != S_OK)
{
wcout << "An error occurred while performing attribute search." << endl; return -1;
}
vector<FieldDef> fieldDefs;
if((hr = theTable.GetFields(fieldDefs)) != S_OK)
{
wcout << "An error occurred while getting the field." << endl; return -1;
}
Table theTableT;
if((hr = geodatabaseT.CreateTable(dataSetPath + tempPath, fieldDefs, L"DEFAULTS", theTableT)) != S_OK)
{
wcout << "An error occurred while creating the table." << endl; return -1;
}
Row theRow;
while((hr = avQueryResult.Next(theRow)) == S_OK)
{
if ((hr = theTableT.Insert(theRow)) != S_OK)
{
wcout << "An error occurred while insertting a row." << endl; return -1;
}
else
{
wcout << "Inserted S" << endl;
}
}
}
if ((hr = CloseGeodatabase(geodatabase)) != S_OK)
{
wcout << "An error occurred while closing the geodatabase." << endl; return -1;
}
return 0;
}
int CopyGDBFileByWinAPI(CString sourceGdbFilePath, CString targetGdbFIlePath)
{
SHFILEOPSTRUCT fop;
ZeroMemory(&fop, sizeof(fop));
fop.wFunc = FO_COPY;
sourceGdbFilePath += '\0';
targetGdbFIlePath += '\0';
fop.pFrom = sourceGdbFilePath;
fop.pTo = targetGdbFIlePath;
SHFileOperation(&fop);
return 0;
}
int CopyGDBFileByFileGDBAPI(wstring sourceGdbFilePath, wstring targetGdbFIlePath)
{
return 0;
}
int _tmain(int argc, _TCHAR* argv[])
{
int hr = 0;
FileGDBCopyHelp *fgdbh = new FileGDBCopyHelp();
hr = fgdbh->CopyGDBFileByFileGDBAPI(L"E:\\02testData\\FeatureDatasetDemo.gdb", L"E:\\a2.gdb");
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 84
- 85
- 86
- 87
- 88
- 89
- 90
- 91
- 92
- 93
- 94
- 95
- 96
- 97
- 98
- 99
- 100
- 101
- 102
- 103
- 104
- 105
- 106
- 107
- 108
- 109
- 110
- 111
- 112
- 113
- 114
- 115
- 116
- 117
- 118
- 119
- 120
- 121
- 122
- 123
- 124
- 125
- 126
- 127
- 128
- 129
- 130
- 131
- 132
- 133
- 134
- 135
- 136
- 137
- 138
- 139
- 140
- 141
- 142
- 143
- 144
- 145
- 146
- 147
- 148
- 149
- 150
- 151
- 152
- 153
- 154
- 155
- 156
- 157
- 158
- 159
- 160
- 161
- 162
- 163
- 164
- 165
- 166
- 167
- 168
- 169
- 170
- 171
- 172
- 173
- 174
- 175
- 176
- 177
- 178
- 179
- 180
- 181
- 182
- 183
- 184
- 185
- 186
- 187
- 188
- 189
- 190
- 191
- 192
- 193
- 194
- 195
- 196
- 197
- 198
- 199
- 200
- 201
- 202
- 203
- 204
- 205
- 206
- 207
- 208
- 209
- 210
- 211
- 212
- 213
- 214
- 215
- 216
- 217
- 218
- 219
- 220
- 221
- 222
- 223
- 224
- 225
- 226
- 227
- 228
- 229
- 230
这里记得将FileGDBAPID.dll(Debug版)放到工程输出目录下,并且在引用目录中加入需要加入的.h文件,以及添加工程库目录并在输入中加入FileGDBAPID.lib(Debug版)。
4. 封装成类库项目 并编写demo调用
*
这里要用到C++类库项目开发的一些知识,详情见我的另一篇博客:
*
这里我贴出源码:
- 利用托管C++封装 并编写C#代码调用