前言
在日常开发中,我们常常需要将一个对象映射到另一个对象,这个过程中可能需要编写大量的重复性代码,如果每次都手动编写,不仅会影响开发效率,而且当项目越来越复杂、庞大的时候还容易出现错误。为了解决这个问题,对象映射库就随之而出了,这些库可以自动完成对象之间的映射,从而减少大量的开发工作量,提高开发工作效率。今天我们来讲讲在ASP.NET Core Web中使用AutoMapper快速进行对象映射。
使用对象映射库有哪些好处?
减少开发工作量,提高开发效率。
减少开发过程中的错误和bug。
简化代码结构,提高代码可读性和可维护性。
AutoMapper对象映射库介绍
AutoMapper是一个简单易用的.NET对象映射库,用于快速、方便地进行对象之间的转换和映射,极大的简化了开发人员在处理对象映射时的工作量。
GitHub开源地址:https://github.com/AutoMapper/AutoMapper
在线文档地址:https://docs.automapper.org/en/stable/Getting-started.html
安装AutoMapper NuGet包
在ASP.NET Core Web API项目中搜索:AutoMapper
NuGet包安装。
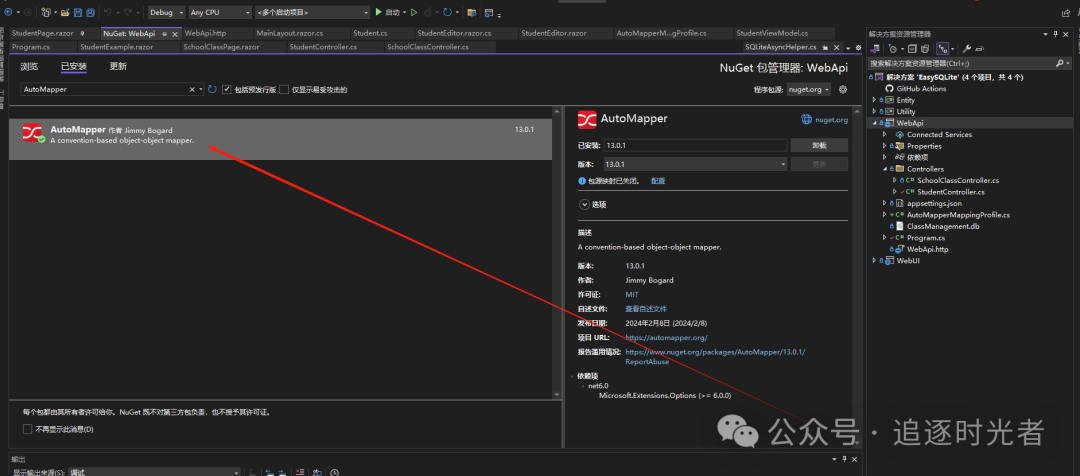
创建源对象和目标对象
接下来我们分别定义一个源对象(Student
)和一个目标对象(StudentViewModel
)。
Student(源对象)
public class Student
{
/// <summary>
/// 学生ID [主键,自动递增]
/// </summary>
[PrimaryKey, AutoIncrement]
[Display(Name = "学生ID")]
public int StudentID { get; set; }
/// <summary>
/// 班级ID
/// </summary>
[Display(Name = "班级ID")]
public int ClassID { get; set; }
/// <summary>
/// 学生姓名
/// </summary>
[Display(Name = "学生姓名")]
public string Name { get; set; }
/// <summary>
/// 学生年龄
/// </summary>
[Display(Name = "学生年龄")]
public int Age { get; set; }
/// <summary>
/// 学生性别
/// </summary>
[Display(Name = "学生性别")]
public string Gender { get; set; }
}
StudentViewModel(目标对象)
public class StudentViewModel
{
/// <summary>
/// 学生ID [主键,自动递增]
/// </summary>
[PrimaryKey, AutoIncrement]
[Display(Name = "学生ID")]
public int StudentID { get; set; }
/// <summary>
/// 班级ID
/// </summary>
[Display(Name = "班级ID")]
public int ClassID { get; set; }
/// <summary>
/// 学生姓名
/// </summary>
[Display(Name = "学生姓名")]
public string Name { get; set; }
/// <summary>
/// 学生年龄
/// </summary>
[Display(Name = "学生年龄")]
public int Age { get; set; }
/// <summary>
/// 学生性别
/// </summary>
[Display(Name = "学生性别")]
public string Gender { get; set; }
/// <summary>
/// 班级名称
/// </summary>
[Display(Name = "班级名称")]
public string ClassName { get; set; }
}
配置AutoMapper映射规则
我们可以定义一个AutoMapperMappingProfile
的映射配置文件,并在其中定义源类型和目标类型之间的映射关系。
using AutoMapper;
using Entity;
using Entity.ViewModel;
namespace WebApi
{
/// <summary>
/// AutoMapper映射配置文件
/// </summary>
public class AutoMapperMappingProfile : Profile
{
/// <summary>
/// 添加映射规则
/// </summary>
public AutoMapperMappingProfile()
{
CreateMap<Student, StudentViewModel>();
}
}
}
Program中注册AutoMapper服务
使用AddAutoMapper()方法可以将AutoMapper所需的服务添加到该集合中,以便在应用程序的其他部分中使用。该方法需要传入一个Assembly数组,以告诉AutoMapper要扫描哪些程序集来查找映射配置(在当前作用域的所有程序集里面扫描AutoMapper的配置文件)。
public static void Main(string[] args)
{
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddControllers();
//添加 AutoMapper 的配置
//使用AddAutoMapper()方法可以将AutoMapper所需的服务添加到该集合中,以便在应用程序的其他部分中使用。
//该方法需要传入一个Assembly数组,以告诉AutoMapper要扫描哪些程序集来查找映射配置(在当前作用域的所有程序集里面扫描AutoMapper的配置文件)。
builder.Services.AddAutoMapper(AppDomain.CurrentDomain.GetAssemblies());
}
进行对象映射操作
依赖注入获取IMapper接口的实例
/// <summary>
/// 学生管理
/// </summary>
[ApiController]
[Route("api/[controller]/[action]")]
public class StudentController : ControllerBase
{
private readonly IMapper _mapper;
/// <summary>
/// 依赖注入
/// </summary>
/// <param name="mapper">mapper</param>
public StudentController(IMapper mapper)
{
_mapper = mapper;
}
}
进行对象映射操作
接下来我们使用使用IMapper接口的Map方法来进行对象映射操作。
var studentsListDto = _mapper.Map<List<StudentViewModel>>(students);
映射结果输出
完整示例源代码
https://github.com/YSGStudyHards/EasySQLite
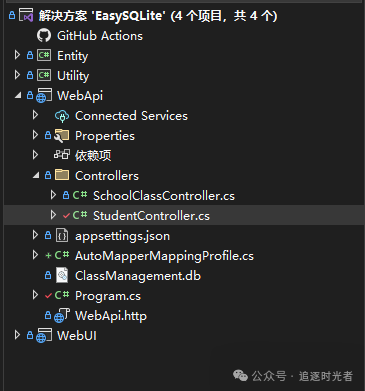