一、 基础知识
1. 安装python环境
2. 概念(算法、表达式、变量、语句、函数、模块、程序、字符串)
函数:
abs(number) 返回数字的绝对值
cmath.sqrt(number) 返回平方根,或是负数的平方根
float(object) 将字符串和数字转换为浮点数
help() 提供交互式帮助
input(prompt) 获取用户输入
int(object) 将字符串和数字转换为整数
long(object) 将字符串和数字转换为厂整型数
math.ceil(number) 返回数的上入整数,返回值的类型为浮点数
math.floor(number) 返回输的下舍整数,返回值的类型为浮点数
math.sqrt(number) 返回平方根,不适用负数
pow(x, y[, z]) 返回x的y次幂(所得结果对z取模)
raw_input(prompt) 获取用户输入,结果被看做是原始字符串
repr(object) 返回值的字符串表现形式
round(number[, ndigits]) 根据给定的精度对数字进行四舍五入
str(object) 将值转换为字符串
二、 列表和元祖
1. 序列
2. 成员资格
3. 方法
函数:
cmp(x, y) 比较两个值
len(seq) 返回序列的长度
list(seq) 把序列转换成列表
max(args) 返回序列或参数集合中最大的值
min(args) 返回序列或参数集合中最小的值
reversed(seq) 对序列进行反向迭代
sorted(seq) 返回已排序的包含seq所有元素的列表
tuple(seq) 把序列转换成元祖
三、 字符串
1. 字符串格式化
2. 字符串方法
函数:
string.capwords(s[, seq]) 使用splite函数分割字符串s(以seq分隔符),使用capitalize函数分割得到的个单词的首字母大写,并且使用join 函数以seq为分隔符将各个单词连接起来
string.maketrans(from, to) 创建用于转换的转换表
四、 字典
1. 映射
2. 利用字典格式化字符串
3. 字典的方法
函数:
dict(seq) 用(键,值)对(或者映射和关键字参数)建立字典
五、 条件、循环和语句
1. 打印
2. 导入
3. 赋值
4. 块
5. 条件
6. 断言
7. 循环
8. 列表推导式
9. pass 、del、exec 、eval
函数:
chr(n) 当传入序号n时,返回n所代表的包含一个字符的字符串0<=n<=256
eval(source[, globals[, locals]]) 将字符串作为表达式计算,并且返回值
enumerate(seq) 产生用于迭代的(索引、值)对
ord(c) 返回单字符字符串的int值
range([start, ] stop[, step]) 创建整数的列表
stored(seq) 产生seq中值的反向版本,用于迭代
xrange([start, ] stop[, stop]) 创建xrange 对象用于迭代
zip(seq1, seq2, …) 创建用于并行迭代的新序列
六、 抽象(函数、作用域、递归)
1. 抽象
2. 函数定义
3. 参数
4. 作用域
5. 递归
6. 函数式编程
函数:
map(func, seq[, seq, …]) 对序列中的每个元素 应用函数
filter(func, seq) 返回其函数为真的元素的列表
reduce(fun, seq[, initial]) 等同于func(func(func(seq[0], seq[1],…)))
sum(seq) 返回seq中所有元素的和
apply(func[, args[, kwargs]]) 调用函数,可以提供参数
七、 抽象(对象、类和类型、面相对象)
1. 对象
2. 类
3. 多态
4. 封装
5. 继承
6. 接口和内省
7. 面相对象设计
函数:
callable(object) 确定对象是否可调用(比如函数或者方法)
getattr(object, name[,default]) 确定特性的值,可选择提供默认值
hasattr(object, name) 确定对象是否有给定的特性
isinstance(object, class) 确定对象是否是类的实例
issubclass(A, B) 确定A是否为B的子类
random.choice(sequence) 从非空序列中随机选择元素
setattr(object, name, value) 设定对象的给定特性为value
type(object) 返回对象的类型
八、 异常
1. 异常对象
2. 警告
3. 引发异常
4. 自定义异常类
5. 捕获异常
6. else子句
7. finally
8. 异常和函数
函数:warnings.filterwarnings(action…) 用户过滤警告
九、 方法、属性、迭代器
1. 旧式类和新类
2. 魔法方法
3. 构造方法
4. 重写
5. 序列和映射
6. 迭代器
7. 生成器
8. 八皇后问题
函数:
iter(obj) 从一个可迭代的对象得到迭代器
property(fget, fset, fdel, doc) 返回一个属性,所有的参数都是可选的
supper(class, obj) 返回一个类的超类的绑定实例
十、 标准Python库中的模块(创建、探究、使用)
模块
包
探究模块
标准库
函数:
dir(obj) 返回按字母顺序排序的属性名称列表
help(obj) 提供交互式帮助或关于特定对象的交互式帮助信息
reload(obj) 返回已经导入模块的重新载入版本,Python3.0废除
十一、 文件和流
类文件对象
打开和关闭文件
模式和文件类型
标准流
读写
读写行
迭代文件内容
函数:
file(name[, mode[, buffering]]) 打开一个文件并返回一个文件对象
open(name[, mode[, buffering]]) file的别名,再打开文件时,使用open而不是file
实验代码:
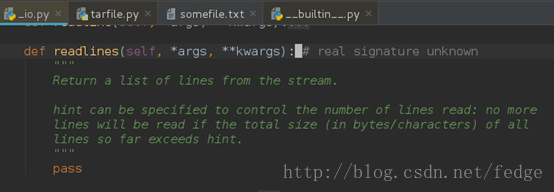
> AAAAA
> BBBBB
> CCCCC
> EOF
[fedge@localhost untitled2]$ cat somefile.txt | python test.py |sort
Traceback (most recent call last):
File "test.py", line 4, in <module>
words = test.split()
NameError: name 'test' is not defined
[fedge@localhost untitled2]$ cat somefile.txt | python test.py |sort
3
[fedge@localhost untitled2]$ cat somefile.txt | python test.py |sort
3
['AAAAA', 'BBBBB', 'CCCCC']
[fedge@localhost untitled2]$ vi somefile.txt
[fedge@localhost untitled2]$ cat somefile.txt | python test.py |sort
9
['AAAAA', '11111', '2222', 'BBBBB', '333', '54444', 'CCCCC', '666', '1111']
[fedge@localhost untitled2]$ cat somefile.txt
012a456789[fedge@localhost untitled2]$
Traceback (most recent call last):
File "<input>", line 1, in <module>
NameError: name 'w' is not defined
>>> f=open('somefile.txt','w')
>>> f.write('0123456789')
>>> f.seek(3)
>>> f.write('a')
>>> f.close()
>>> f=open('somefile.txt','r')
>>> f.readlines()
['012a456789']
>>> f.readline()
''
>>> f.read()
''
>>> f.read()
''
>>> f.read()
''
>>> f=open('somefile.txt')
>>> f.read()
'012a456789'
>>> f.close()
>>> f=open('somefile.txt','r')
>>> f.read(10)
'012a456789'
>>> f.close()
>>> f.closed
True
>>> f=open('somefile.txt')
>>> f.read(3)
'012'
>>> f.tell()
3
>>> from __future__ import with_statement
>>> with open('somefile.txt') as file:
... file.readlines()
...
>>> with open('somefile.txt') as file:
... file.read()
...
>>>
>>> with open('somefile.txt') as file:
... text=file.read()
... print text
...
012a456789
>>> file.closed
True
>>> with open(name='somefile.txt',buffer=204800) as file:
... text=file.read()
... print text
...
Traceback (most recent call last):
File "<input>", line 1, in <module>
TypeError: 'buffer' is an invalid keyword argument for this function
>>> with open(name='somefile.txt',buffering=204800) as file:
... text=file.read()
... print text
...
012a456789
>>> file.softspace
0
>>> def process(string):
... print 'Processing:',string
...
>>> f=open('somefile.txt')
>>> for char in f.read():
... process(char)
... f.close()
Processing: 0
Processing: 1
Processing: 2
Processing: a
Processing: 4
Processing: 5
Processing: 6
Processing: 7
Processing: 8
Processing: 9
>>> f=open('somefile.txt')
>>> for char in f.readlines():
... process(char)
... f.close()
Processing: 012a456789
>>> import fileinput
>>> for line in fileinput.input('somefile.txt'):
... process(line)
...
Processing: 012a456789
>>> for line in fileinput.input('somefile.txt'):
... process(line)
...
Processing: 012a456789
Processing: 012a456789
Processing: 012a456789
Processing: 012a456789
Processing: 012a456789
Processing: 012a456789
>>> f=open('somefile.txt')
>>> f
<open file 'somefile.txt', mode 'r' at 0x1f14780>
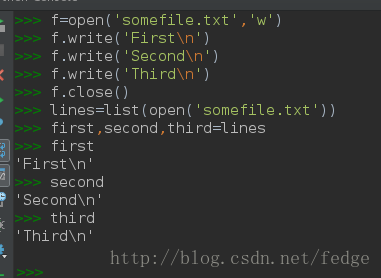
=================================================================
参考文献
《Python 基础教程(第2版.修订版)》 作者【挪】Magnus Lie Hetland
=================================================================
1. 安装python环境
2. 概念(算法、表达式、变量、语句、函数、模块、程序、字符串)
函数:
abs(number) 返回数字的绝对值
cmath.sqrt(number) 返回平方根,或是负数的平方根
float(object) 将字符串和数字转换为浮点数
help() 提供交互式帮助
input(prompt) 获取用户输入
int(object) 将字符串和数字转换为整数
long(object) 将字符串和数字转换为厂整型数
math.ceil(number) 返回数的上入整数,返回值的类型为浮点数
math.floor(number) 返回输的下舍整数,返回值的类型为浮点数
math.sqrt(number) 返回平方根,不适用负数
pow(x, y[, z]) 返回x的y次幂(所得结果对z取模)
raw_input(prompt) 获取用户输入,结果被看做是原始字符串
repr(object) 返回值的字符串表现形式
round(number[, ndigits]) 根据给定的精度对数字进行四舍五入
str(object) 将值转换为字符串
二、 列表和元祖
1. 序列
2. 成员资格
3. 方法
函数:
cmp(x, y) 比较两个值
len(seq) 返回序列的长度
list(seq) 把序列转换成列表
max(args) 返回序列或参数集合中最大的值
min(args) 返回序列或参数集合中最小的值
reversed(seq) 对序列进行反向迭代
sorted(seq) 返回已排序的包含seq所有元素的列表
tuple(seq) 把序列转换成元祖
三、 字符串
1. 字符串格式化
2. 字符串方法
函数:
string.capwords(s[, seq]) 使用splite函数分割字符串s(以seq分隔符),使用capitalize函数分割得到的个单词的首字母大写,并且使用join 函数以seq为分隔符将各个单词连接起来
string.maketrans(from, to) 创建用于转换的转换表
四、 字典
1. 映射
2. 利用字典格式化字符串
3. 字典的方法
函数:
dict(seq) 用(键,值)对(或者映射和关键字参数)建立字典
五、 条件、循环和语句
1. 打印
2. 导入
3. 赋值
4. 块
5. 条件
6. 断言
7. 循环
8. 列表推导式
9. pass 、del、exec 、eval
函数:
chr(n) 当传入序号n时,返回n所代表的包含一个字符的字符串0<=n<=256
eval(source[, globals[, locals]]) 将字符串作为表达式计算,并且返回值
enumerate(seq) 产生用于迭代的(索引、值)对
ord(c) 返回单字符字符串的int值
range([start, ] stop[, step]) 创建整数的列表
stored(seq) 产生seq中值的反向版本,用于迭代
xrange([start, ] stop[, stop]) 创建xrange 对象用于迭代
zip(seq1, seq2, …) 创建用于并行迭代的新序列
六、 抽象(函数、作用域、递归)
1. 抽象
2. 函数定义
3. 参数
4. 作用域
5. 递归
6. 函数式编程
函数:
map(func, seq[, seq, …]) 对序列中的每个元素 应用函数
filter(func, seq) 返回其函数为真的元素的列表
reduce(fun, seq[, initial]) 等同于func(func(func(seq[0], seq[1],…)))
sum(seq) 返回seq中所有元素的和
apply(func[, args[, kwargs]]) 调用函数,可以提供参数
七、 抽象(对象、类和类型、面相对象)
1. 对象
2. 类
3. 多态
4. 封装
5. 继承
6. 接口和内省
7. 面相对象设计
函数:
callable(object) 确定对象是否可调用(比如函数或者方法)
getattr(object, name[,default]) 确定特性的值,可选择提供默认值
hasattr(object, name) 确定对象是否有给定的特性
isinstance(object, class) 确定对象是否是类的实例
issubclass(A, B) 确定A是否为B的子类
random.choice(sequence) 从非空序列中随机选择元素
setattr(object, name, value) 设定对象的给定特性为value
type(object) 返回对象的类型
八、 异常
1. 异常对象
2. 警告
3. 引发异常
4. 自定义异常类
5. 捕获异常
6. else子句
7. finally
8. 异常和函数
函数:warnings.filterwarnings(action…) 用户过滤警告
九、 方法、属性、迭代器
1. 旧式类和新类
2. 魔法方法
3. 构造方法
4. 重写
5. 序列和映射
6. 迭代器
7. 生成器
8. 八皇后问题
函数:
iter(obj) 从一个可迭代的对象得到迭代器
property(fget, fset, fdel, doc) 返回一个属性,所有的参数都是可选的
supper(class, obj) 返回一个类的超类的绑定实例
十、 标准Python库中的模块(创建、探究、使用)
模块
包
探究模块
标准库
函数:
dir(obj) 返回按字母顺序排序的属性名称列表
help(obj) 提供交互式帮助或关于特定对象的交互式帮助信息
reload(obj) 返回已经导入模块的重新载入版本,Python3.0废除
十一、 文件和流
类文件对象
打开和关闭文件
模式和文件类型
标准流
读写
读写行
迭代文件内容
函数:
file(name[, mode[, buffering]]) 打开一个文件并返回一个文件对象
open(name[, mode[, buffering]]) file的别名,再打开文件时,使用open而不是file
实验代码:
[fedge@localhost untitled2]$ ls
python2test.py python2test.pyc test2.py test.py venv
> AAAAA
> BBBBB
> CCCCC
> EOF
[fedge@localhost untitled2]$ cat somefile.txt | python test.py |sort
Traceback (most recent call last):
File "test.py", line 4, in <module>
words = test.split()
NameError: name 'test' is not defined
[fedge@localhost untitled2]$ cat somefile.txt | python test.py |sort
3
[fedge@localhost untitled2]$ cat somefile.txt | python test.py |sort
3
['AAAAA', 'BBBBB', 'CCCCC']
[fedge@localhost untitled2]$ vi somefile.txt
[fedge@localhost untitled2]$ cat somefile.txt | python test.py |sort
9
['AAAAA', '11111', '2222', 'BBBBB', '333', '54444', 'CCCCC', '666', '1111']
[fedge@localhost untitled2]$ cat somefile.txt
012a456789[fedge@localhost untitled2]$
[fedge@localhost untitled2]$
Traceback (most recent call last):
File "<input>", line 1, in <module>
NameError: name 'w' is not defined
>>> f=open('somefile.txt','w')
>>> f.write('0123456789')
>>> f.seek(3)
>>> f.write('a')
>>> f.close()
>>> f=open('somefile.txt','r')
>>> f.readlines()
['012a456789']
>>> f.readline()
''
>>> f.read()
''
>>> f.read()
''
>>> f.read()
''
>>> f=open('somefile.txt')
>>> f.read()
'012a456789'
>>> f.close()
>>> f=open('somefile.txt','r')
>>> f.read(10)
'012a456789'
>>> f.close()
>>> f.closed
True
>>> f=open('somefile.txt')
>>> f.read(3)
'012'
>>> f.tell()
3
>>> from __future__ import with_statement
>>> with open('somefile.txt') as file:
... file.readlines()
...
>>> with open('somefile.txt') as file:
... file.read()
...
>>>
>>> with open('somefile.txt') as file:
... text=file.read()
... print text
...
012a456789
>>> file.closed
True
>>> with open(name='somefile.txt',buffer=204800) as file:
... text=file.read()
... print text
...
Traceback (most recent call last):
File "<input>", line 1, in <module>
TypeError: 'buffer' is an invalid keyword argument for this function
>>> with open(name='somefile.txt',buffering=204800) as file:
... text=file.read()
... print text
...
012a456789
>>> file.softspace
0
>>> def process(string):
... print 'Processing:',string
...
>>> f=open('somefile.txt')
>>> for char in f.read():
... process(char)
... f.close()
Processing: 0
Processing: 1
Processing: 2
Processing: a
Processing: 4
Processing: 5
Processing: 6
Processing: 7
Processing: 8
Processing: 9
>>> f=open('somefile.txt')
>>> for char in f.readlines():
... process(char)
... f.close()
Processing: 012a456789
>>> import fileinput
>>> for line in fileinput.input('somefile.txt'):
... process(line)
...
Processing: 012a456789
>>> for line in fileinput.input('somefile.txt'):
... process(line)
...
Processing: 012a456789
Processing: 012a456789
Processing: 012a456789
Processing: 012a456789
Processing: 012a456789
Processing: 012a456789
>>> f=open('somefile.txt')
>>> f
<open file 'somefile.txt', mode 'r' at 0x1f14780>
=================================================================
参考文献
《Python 基础教程(第2版.修订版)》 作者【挪】Magnus Lie Hetland
=================================================================