1. ZooKeeper是什么
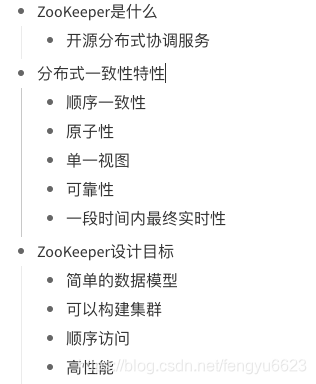
2. 基础操作
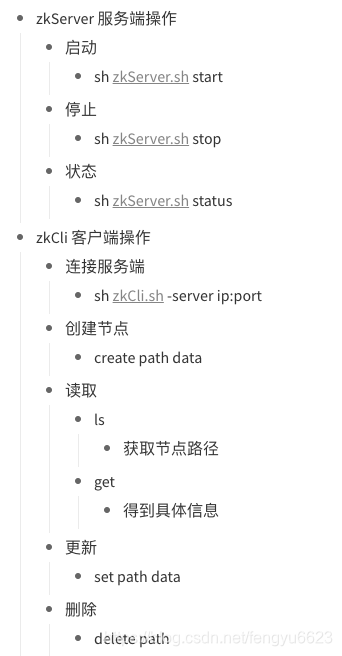
3. 原生Api支持
1、创建session
public class CreateSessionUsage implements Watcher {
private static CountDownLatch connectedSemaphore = new CountDownLatch(1);
public static void main(String[] args) throws Exception {
ZooKeeper zooKeeper = new ZooKeeper("127.0.0.1:2181", 5000, new CreateSessionUsage());
//zookeeper 运行状态
System.out.println(zooKeeper.getState());
connectedSemaphore.await();
System.out.println("ZooKeeper session established");
}
@Override
public void process(WatchedEvent event) {
System.out.println("Receive watched event: " + event);
if(Event.KeeperState.SyncConnected == event.getState()){
connectedSemaphore.countDown();
}
}
}
2、创建复用session
public class CreateSessionWithSidUsage implements Watcher {
private static CountDownLatch connectedSemaphore = new CountDownLatch(1);
public static void main(String[] args) throws Exception {
ZooKeeper zooKeeper = new ZooKeeper("127.0.0.1:2181", 5000, new CreateSessionWithSidUsage());
connectedSemaphore.await();
long sessionId = zooKeeper.getSessionId();
byte[] passwd = zooKeeper.getSessionPasswd();
//错误session id 和 passwd
zooKeeper = new ZooKeeper("127.0.0.1:2181", 5000, new CreateSessionWithSidUsage(), 1l, "test".getBytes());
//正确的session id 和 passwd
zooKeeper = new ZooKeeper("127.0.0.1:2181", 5000, new CreateSessionWithSidUsage(), sessionId, passwd);
Thread.sleep(Integer.MAX_VALUE);
}
@Override
public void process(WatchedEvent event) {
System.out.println("Receive watched event: " + event);
if (Event.KeeperState.SyncConnected == event.getState()) {
connectedSemaphore.countDown();
}
}
}
3、创建节点
3.1同步创建临时节点和临时顺序节点
public class CreateSyncNodeUsage implements Watcher {
private static CountDownLatch connectedSemaphore = new CountDownLatch(1);
public static void main(String[] args) throws Exception {
ZooKeeper zooKeeper = new ZooKeeper("127.0.0.1:2181", 5000,new CreateSyncNodeUsage());
connectedSemaphore.await();
//同步创建临时节点
String path1 = zooKeeper.create("/zk-test-node-", "".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL);
System.out.println("Success create znode: "+ path1);
//同步创建临时顺序节点
String path2 = zooKeeper.create("/zk-test-node-", "".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL_SEQUENTIAL);
System.out.println("Success create znode: "+ path2);
}
@Override
public void process(WatchedEvent event) {
if (Event.KeeperState.SyncConnected == event.getState()) {
connectedSemaphore.countDown();
}
}
}
3.2异步创建临时节点和临时顺序节点
public class CreateASyncNodeUsage implements Watcher {
private static CountDownLatch connectedSemaphore = new CountDownLatch(1);
public static void main(String[] args) throws Exception {
ZooKeeper zooKeeper = new ZooKeeper("127.0.0.1:2181", 5000, new CreateASyncNodeUsage());
connectedSemaphore.await();
//异步临时节点
zooKeeper.create("/zk-test-node-", "".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL, new IStringCallback(), "I am context");
//异步临时节点 此时已创建过 返回-110错误
zooKeeper.create("/zk-test-node-", "".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL, new IStringCallback(), "I am context");
//异步创建临时顺序节点
zooKeeper.create("/zk-test-node-", "".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL_SEQUENTIAL, new IStringCallback(), "I am context");
Thread.sleep(Integer.MAX_VALUE);
}
@Override
public void process(WatchedEvent event) {
if (Event.KeeperState.SyncConnected == event.getState()) {
connectedSemaphore.countDown();
}
}
}
class IStringCallback implements AsyncCallback.StringCallback {
@Override
public void processResult(int rc, String path, Object ctx, String name) {
System.out.println("Create path result: [" + rc + "," + path + "," + ctx + ",real path name: " + name);
}
}
4、删除节点
public void delete(String path, int version)
public void delete(String path, int version, VoidCallback cb, Object ctx)
5、读取数据
5.1同步获取子节点
public class GetChildrenUsage implements Watcher {
private static CountDownLatch connectedSemaphore = new CountDownLatch(1);
private static ZooKeeper zooKeeper = null;
public static void main(String[] args) throws Exception {
String path = "/zk-book";
zooKeeper = new ZooKeeper("127.0.0.1:2181", 5000, new GetChildrenUsage());
connectedSemaphore.await();
zooKeeper.create(path, "".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.PERSISTENT);
zooKeeper.create(path + "/c1", "".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL);
List<String> childrenList = zooKeeper.getChildren(path, true);
System.out.println(childrenList);
zooKeeper.create(path + "/c2", "".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL);
Thread.sleep(Integer.MAX_VALUE);
}
@Override
public void process(WatchedEvent event) {
if(Event.KeeperState.SyncConnected == event.getState()){
if(Event.EventType.None == event.getType() && null == event.getPath()){
connectedSemaphore.countDown();
}else if(event.getType() == Event.EventType.NodeChildrenChanged){
try {
System.out.println("ReGet Child:"+zooKeeper.getChildren(event.getPath(),true));
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
}
5.2异步获取子节点
public class AsynGetChildrenUsage implements Watcher {
private static CountDownLatch connectedSemaphore = new CountDownLatch(1);
private static ZooKeeper zooKeeper = null;
public static void main(String[] args) throws Exception {
String path = "/zk-book";
zooKeeper = new ZooKeeper("127.0.0.1:2181", 5000, new AsynGetChildrenUsage());
connectedSemaphore.await();
zooKeeper.create(path, "".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.PERSISTENT);
zooKeeper.create(path + "/c1", "".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL);
zooKeeper.getChildren(path, true,new IChildren2Callback(),null);
zooKeeper.create(path + "/c2", "".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL);
Thread.sleep(Integer.MAX_VALUE);
}
@Override
public void process(WatchedEvent event) {
if (Event.KeeperState.SyncConnected == event.getState()) {
if (Event.EventType.None == event.getType() && null == event.getPath()) {
connectedSemaphore.countDown();
} else if (event.getType() == Event.EventType.NodeChildrenChanged) {
try {
System.out.println("ReGet Child:" + zooKeeper.getChildren(event.getPath(), true));
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
}
class IChildren2Callback implements AsyncCallback.Children2Callback {
@Override
public void processResult(int rc, String path, Object ctx, List<String> list, Stat stat) {
System.out.println("Create path result: [" + rc + "," + path + "," + ctx + ",children list: " + list + "stat " + stat);
}
}
5.3同步获取数据
public class GetDataUsage implements Watcher {
private static CountDownLatch connectedSemaphore = new CountDownLatch(1);
private static ZooKeeper zooKeeper = null;
private static Stat stat = new Stat();
public static void main(String[] args) throws Exception {
String path = "/zk-book";
zooKeeper = new ZooKeeper("127.0.0.1:2181", 5000, new GetDataUsage());
connectedSemaphore.await();
zooKeeper.create(path, "123".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL);
System.out.println(new String(zooKeeper.getData(path, true, stat)));
System.out.println(stat.getCzxid() + "," + stat.getMzxid() + "," + stat.getVersion());
zooKeeper.setData(path, "123".getBytes(), -1);
Thread.sleep(Integer.MAX_VALUE);
}
@Override
public void process(WatchedEvent event) {
if (Event.KeeperState.SyncConnected == event.getState()) {
if (Event.EventType.None == event.getType() && null == event.getPath()) {
connectedSemaphore.countDown();
} else if (event.getType() == Event.EventType.NodeDataChanged) {
try {
System.out.println(new String(zooKeeper.getData(event.getPath(), true, stat)));
System.out.println(stat.getCzxid() + "," + stat.getMzxid() + "," + stat.getVersion());
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
}
5.4异步获取数据
public class AsynGetDataUsage implements Watcher {
private static CountDownLatch connectedSemaphore = new CountDownLatch(1);
private static ZooKeeper zooKeeper = null;
public static void main(String[] args) throws Exception {
String path = "/zk-book";
zooKeeper = new ZooKeeper("127.0.0.1:2181", 5000, new AsynGetDataUsage());
connectedSemaphore.await();
zooKeeper.create(path, "123".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL);
zooKeeper.getData(path, true, new IDataCallback(), null);
zooKeeper.setData(path, "123".getBytes(), -1);
Thread.sleep(Integer.MAX_VALUE);
}
@Override
public void process(WatchedEvent event) {
if (Event.KeeperState.SyncConnected == event.getState()) {
if (Event.EventType.None == event.getType() && null == event.getPath()) {
connectedSemaphore.countDown();
} else if (event.getType() == Event.EventType.NodeDataChanged) {
try {
zooKeeper.getData(event.getPath(), true, new IDataCallback(), null);
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
}
class IDataCallback implements AsyncCallback.DataCallback {
@Override
public void processResult(int rc, String path, Object ctx, byte[] data, Stat stat) {
System.out.println(rc + "," + path + "," + new String(data));
System.out.println(stat.getCzxid() + "," + stat.getMzxid() + "," + stat.getVersion());
}
}
6、更新数据
6.1同步更新数据
public class SetDataUsage implements Watcher {
private static CountDownLatch connectedSemaphore = new CountDownLatch(1);
private static ZooKeeper zooKeeper = null;
public static void main(String[] args) throws Exception {
String path = "/zk-book";
zooKeeper = new ZooKeeper("127.0.0.1:2181", 5000, new SetDataUsage());
connectedSemaphore.await();
zooKeeper.create(path, "123".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL);
zooKeeper.getData(path,true,null);
//zooKeeper中version 基于0开始 这里给-1就是一个标志 表示最新版本
Stat stat = zooKeeper.setData(path, "456".getBytes(), -1);
System.out.println(stat.getCzxid()+","+stat.getMzxid()+","+stat.getVersion());
Stat stat2 = zooKeeper.setData(path, "456".getBytes(), stat.getVersion());
System.out.println(stat2.getCzxid()+","+stat2.getMzxid()+","+stat2.getVersion());
//此处更新失败 使用了失效的版本
zooKeeper.setData(path,"456".getBytes(),stat.getVersion());
Thread.sleep(Integer.MAX_VALUE);
}
@Override
public void process(WatchedEvent event) {
if(Event.KeeperState.SyncConnected == event.getState()){
if(Event.EventType.None == event.getType() && null == event.getPath()){
connectedSemaphore.countDown();
}
}
}
}
6.2异步更新数据
public class AsynSetDataUsage implements Watcher {
private static CountDownLatch connectedSemaphore = new CountDownLatch(1);
private static ZooKeeper zooKeeper = null;
public static void main(String[] args) throws Exception {
String path = "/zk-book";
zooKeeper = new ZooKeeper("127.0.0.1:2181", 5000, new AsynSetDataUsage());
connectedSemaphore.await();
zooKeeper.create(path, "123".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL);
//此处更新失败 使用了失效的版本
zooKeeper.setData(path,"456".getBytes(),-1,new IStatCallback(),null);
Thread.sleep(Integer.MAX_VALUE);
}
@Override
public void process(WatchedEvent event) {
if(Event.KeeperState.SyncConnected == event.getState()){
if(Event.EventType.None == event.getType() && null == event.getPath()){
connectedSemaphore.countDown();
}
}
}
}
class IStatCallback implements AsyncCallback.StatCallback{
@Override
public void processResult(int rc, String path, Object ctx, Stat stat) {
if(rc == 0){
System.out.println("SUCCESS");
}
}
}
7、检测节点是否存在
public class ExistNodeUsage implements Watcher {
private static CountDownLatch connectedSemaphore = new CountDownLatch(1);
private static ZooKeeper zooKeeper = null;
public static void main(String[] args) throws Exception {
String path = "/zk-book";
zooKeeper = new ZooKeeper("127.0.0.1:2181", 5000, new ExistNodeUsage());
connectedSemaphore.await();
//true 为复用默认Watcher
zooKeeper.exists(path, true);
zooKeeper.create(path, "".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.PERSISTENT);
zooKeeper.setData(path, "123".getBytes(), -1);
zooKeeper.create(path + "/c1", "".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.PERSISTENT);
zooKeeper.delete(path + "/c1", -1);
zooKeeper.delete(path, -1);
Thread.sleep(Integer.MAX_VALUE);
}
@Override
public void process(WatchedEvent event) {
try {
if (Event.KeeperState.SyncConnected == event.getState()) {
if (Event.EventType.None == event.getType() && null == event.getPath()) {
connectedSemaphore.countDown();
} else if (Event.EventType.NodeCreated == event.getType()) {
System.out.println("Node(" + event.getPath() + ")Created");
zooKeeper.exists(event.getPath(), true);
} else if (Event.EventType.NodeDeleted == event.getType()) {
System.out.println("Node(" + event.getPath() + ")Deleted");
zooKeeper.exists(event.getPath(), true);
} else if (Event.EventType.NodeDataChanged == event.getType()) {
System.out.println("Node(" + event.getPath() + ")DataChanged");
zooKeeper.exists(event.getPath(), true);
}
}
} catch (Exception e) {
}
}
}