方式1:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
</head>
<body>
<div id="map"></div>
<button onclick="clickStatus()">激活弹窗</button>
<script>
let isInit=false;
let addDiv = '';
let addtitle = '';
let addcontent = '';
let addClose='';
let flag=true;
let initTool=function (frameDiv) {
if (!isInit) {
return 0;
}
const rightdiv = document.createElement('DIV');
rightdiv.className = "tooltip-right";
rightdiv.style = `
position:absolute;
min-width:380px;
min-height:100px;
max-height:400px;
background:#f2f2f2;
border-radius:10px;
box-shadow: 2px 4px 5px #888888;
`;
const arrow = document.createElement('DIV');
arrow.className = "tooltip-arrow";
arrow.style = `
position:absolute;
left:-24px;
top:38px;
width:0;
height:0;
border-top: 12px solid transparent;
border-right: 12px solid #f2f2f2;
border-bottom: 12px solid transparent;
border-left: 12px solid transparent;`;
rightdiv.appendChild(arrow);
const title = document.createElement('DIV');
title.className = "tooltip-title";
title.style = `
width:100%;
height:30px;
line-height:30px;
font-size: small;
text-align: left;
border-bottom: 1px solid #777;
padding: 0 0 0 0px;
`
rightdiv.appendChild(title);
const close = document.createElement('DIV');
close.className = "tooltip-close";
close.style = `
position:absolute;
right:-5px;
top:0px;
width:30px;
height:30px;
line-height:30px;
color: #4876ff;
z-index:999;`
rightdiv.appendChild(close);
const content = document.createElement('DIV');
content.className = "tooltip-content";
content.style = `
width:100%;
box-sizing:border-box;
padding:10px 0 10px 10px;
word-break:break-all;
`
rightdiv.appendChild(content);
addtitle = title;
addcontent = content;
addClose=close;
addDiv = rightdiv;
frameDiv.appendChild(rightdiv);
isInit = true;
}
let setVisible=function (visible) {
if (!isInit) {
return 0;
}
addDiv.style.display = visible ? 'block' : 'none';
}
let showAt=function (position, message, content) {
if (!isInit) {
return 0;
}
if (position && message && content) {
setVisible(true);
addtitle.innerHTML = message;
addcontent.innerHTML = content;
addClose.innerHTML = '✕';
addDiv.style.left = position.x + 30 + "px";
addDiv.style.top = (position.y - 50) + "px";
}
}
let map=document.getElementById('map')
let content=
"<div><h5>管径:<span>" +
'diameter' +"</span></h5></div>"+
"<div><h5>起点号:<span>" +
'startPoint'+"</span></h5></div>"+
"<div><h5>起点埋深:<span>" +
'startDeep'+"m</span></h5></div>" +
"<div><h5>起点管线高程:<span>" +
'starHeight'+"m</span></h5></div>"+
"<div><h5>终点号:<span>" +
'endPoint'+"</span></h5></div>"+
"<div><h5>终点埋深:<span>" +
'endDeep'+"m</span></h5></div>" +
"<div><h5>终点管线高程:<span>" +
'endHeight'+"m</span></h5></div>";
let click=function(ev){
console.log(ev);
let position ={
x:ev.clientX + document.body.scrollLeft - document.body.clientLeft,
y:ev.clientY + document.body.scrollTop - document.body.clientTop
}
showAt(position, '设施概况', content);
}
function clickStatus(){
isInit = !isInit;
console.log(isInit);
if(isInit){
initTool(map)
document.addEventListener('click',click)
addClose.addEventListener('click', function () {
isInit = !isInit;
document.removeEventListener('click', click);
addDiv.style.display = 'none';
})
}else{
document.removeEventListener('click', click);
}
}
</script>
</body>
</body>
</html>
方式2
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
.ol-popup-facility {
position: absolute;
background-color: rgba( 255,255,255,.8 );
-webkit-filter: drop-shadow(0 1px 4px rgba(0, 0, 0, 0.2));
filter: drop-shadow(0 1px 4px rgba(0, 0, 0, 0.2));
padding: 15px;
border-radius: 10px;
border: 1px solid #cccccc;
bottom: 12px;
left: -50px;
width: 420px;
height: 300px;
color: #7E8A9E;
}
.ol-popup-facility .popup-title{
color: #7E8A9E;
display: inline-block;
font:15px arial,sans-serif;
font-weight: bolder;
height: 26px;
font-size: 16px;
letter-spacing: 1px;
line-height: 26px;
}
.ol-popup-facility .popup-close{
position:absolute;
right:0px;
top:10px;
width:30px;
height:30px;
line-height:30px;
color: #4876ff;
z-index:200;
}
.ol-popup-facility:after, .ol-popup-facility:before {
top: 100%;
border: solid transparent;
content: " ";
height: 0;
width: 0;
position: absolute;
pointer-events: none;
}
.ol-popup-facility:after {
border-top-color: white;
border-width: 10px;
left: 48px;
margin-left: -10px;
}
.ol-popup-facility:before {
border-top-color: #cccccc;
border-width: 11px;
left: 48px;
margin-left: -11px;
}
</style>
</head>
<body>
<div id="map">
<div id="popup" class="ol-popup-facility">
<div id="popup-close" class="popup-close">✕</div>
<div id="popup-title" class="popup-title"></div>
<div id="popup-content" class="popup-content"></div>
</div>
</div>
<button onclick="clickStatus()">激活弹窗</button>
<script>
let map=document.getElementById('map')
let isInit=false;
let addDiv = document.getElementById('popup')
let addtitle = document.getElementById('popup-title')
let addcontent = document.getElementById('popup-content')
let addClose=document.getElementById('popup-close')
addDiv.style.display = 'none';
let setVisible=function (visible) {
if (!isInit) {
return 0;
}
addDiv.style.display = visible ? 'block' : 'none';
}
let showAt=function (position, message, content) {
if (!isInit) {
return 0;
}
if (position && message && content) {
setVisible(true);
addtitle.innerHTML = message;
addcontent.innerHTML = content;
addClose.innerHTML = '✕';
addDiv.style.left = position.x + 30 + "px";
addDiv.style.top = (position.y - 50) + "px";
}
}
let content=
"<div><h5>管径:<span>" +
'diameter' +"</span></h5></div>"+
"<div><h5>起点号:<span>" +
'startPoint'+"</span></h5></div>"+
"<div><h5>起点埋深:<span>" +
'startDeep'+"m</span></h5></div>" +
"<div><h5>起点管线高程:<span>" +
'starHeight'+"m</span></h5></div>"+
"<div><h5>终点号:<span>" +
'endPoint'+"</span></h5></div>"+
"<div><h5>终点埋深:<span>" +
'endDeep'+"m</span></h5></div>" +
"<div><h5>终点管线高程:<span>" +
'endHeight'+"m</span></h5></div>";
let click=function(ev){
console.log(ev);
let position ={
x:ev.clientX + document.body.scrollLeft - document.body.clientLeft,
y:ev.clientY + document.body.scrollTop - document.body.clientTop
}
showAt(position, '设施概况', content);
}
function clickStatus(){
isInit = !isInit;
console.log(isInit);
if(isInit){
document.addEventListener('click',click)
addClose.addEventListener('click', function () {
isInit = !isInit;
document.removeEventListener('click', click);
addDiv.style.display = 'none';
})
}else{
addDiv.style.display = 'none';
document.removeEventListener('click', click);
}
}
</script>
</body>
</body>
</html>
方式1效果
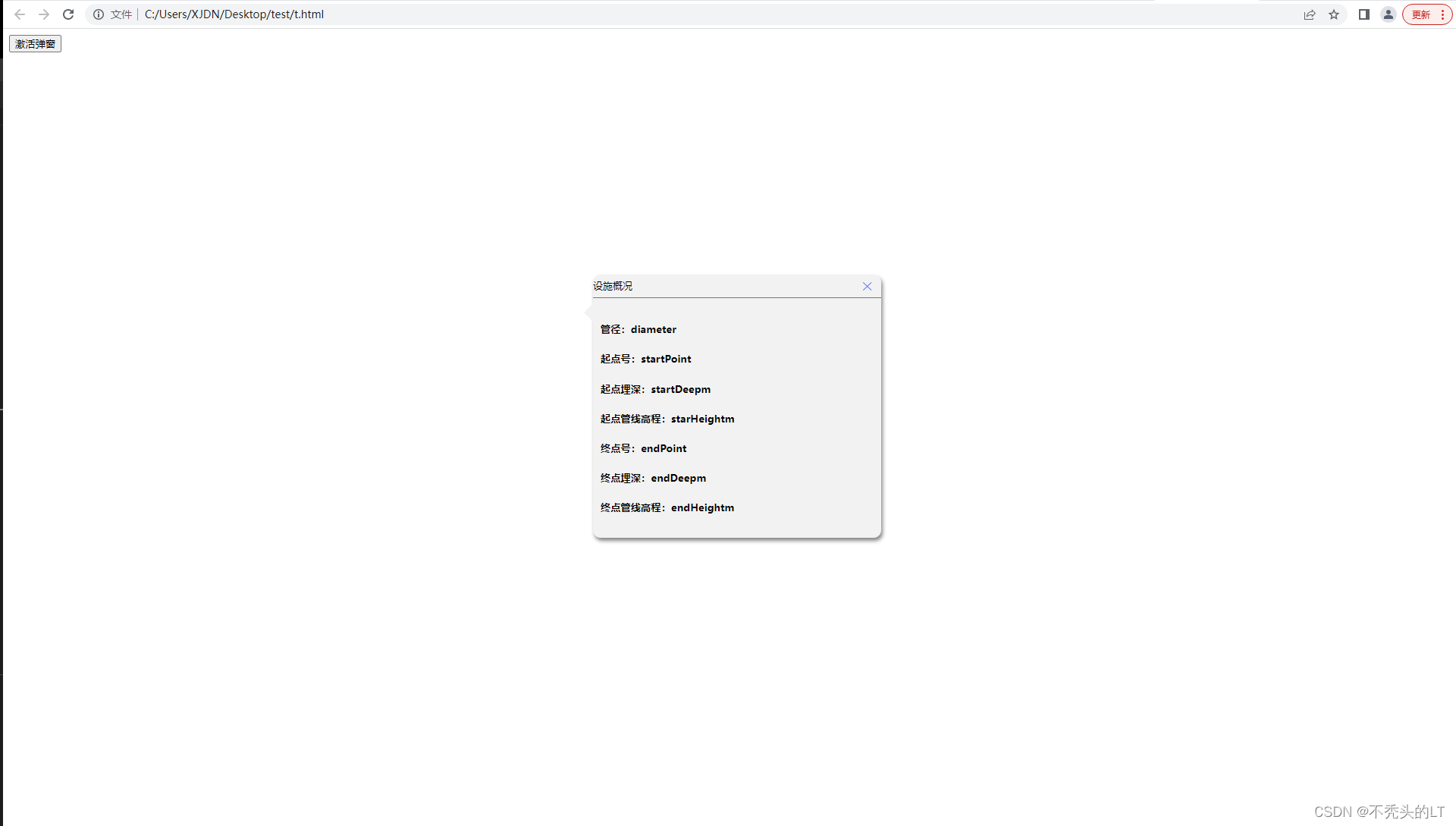
方式2效果
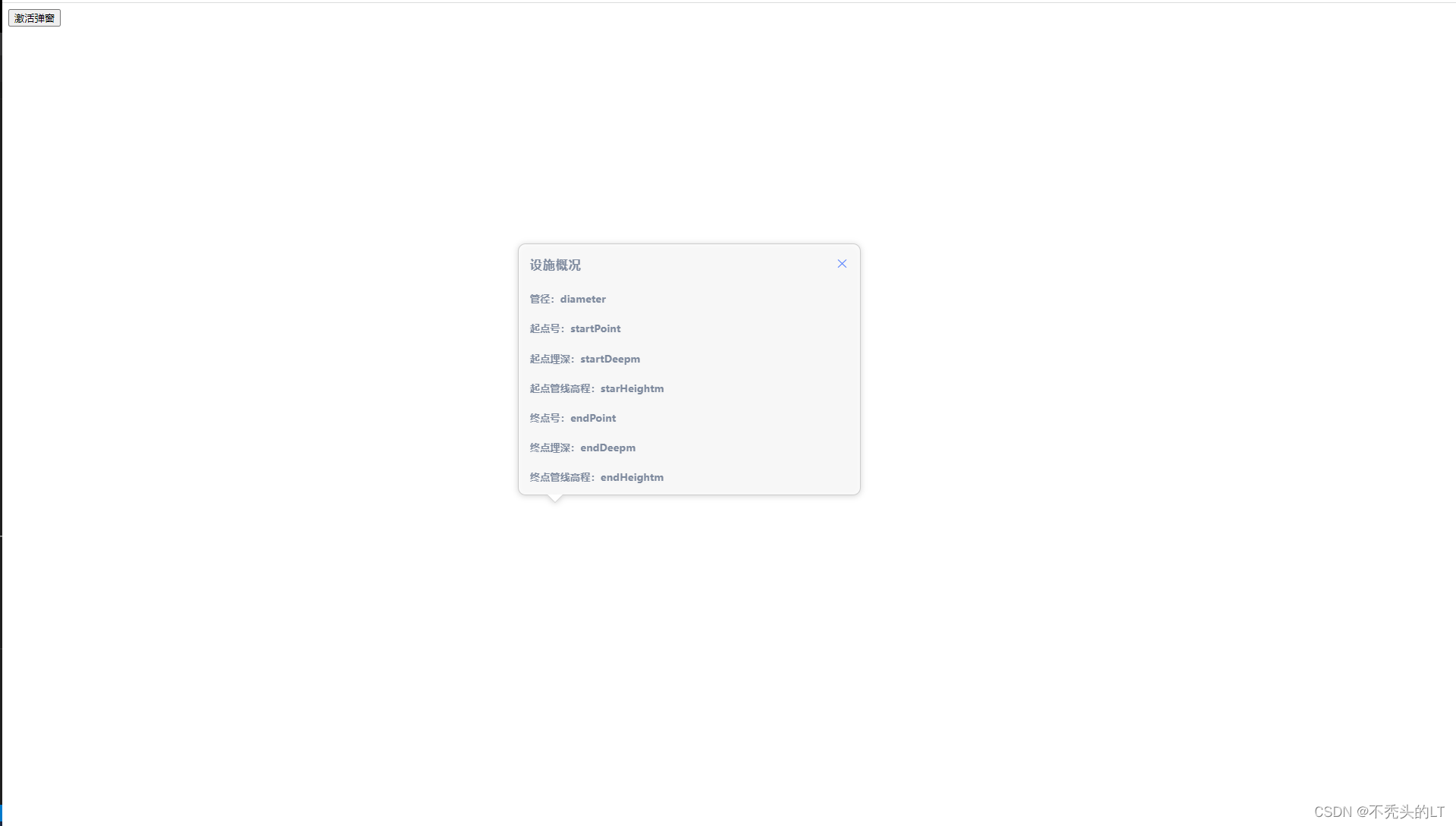