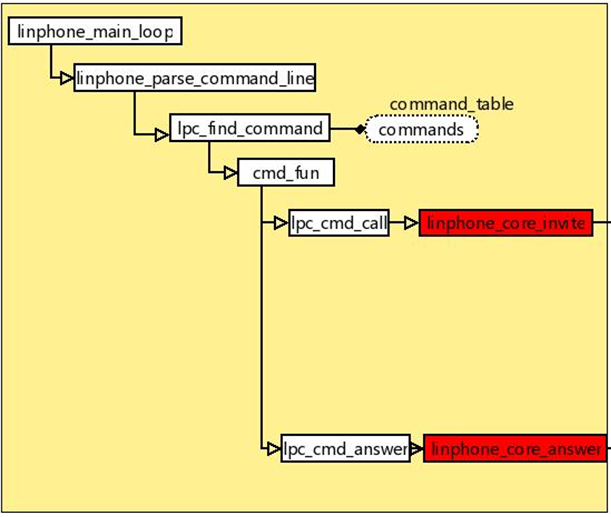
static int
linphonec_main_loop (LinphoneCore * opm)
{
char *input;
print_prompt(opm);
while (linphonec_running && (input=linphonec_readline(prompt)))
{
char *iptr; /* input and input pointer */
size_t input_len;
/* Strip blanks */
iptr=lpc_strip_blanks(input);
input_len = strlen(iptr);
/*
* Do nothing but release memory
* if only blanks are read
*/
if ( ! input_len )
{
free(input);
continue;
}
/*
* Only add to history if not already
* last item in it, and only if the command
* doesn't start with a space (to allow for
* hiding passwords)
*/
if ( iptr == input && strcmp(last_in_history, iptr) )
{
strncpy(last_in_history,iptr,sizeof(last_in_history));
last_in_history[sizeof(last_in_history)-1]='\0';
add_history(iptr);
}
linphonec_parse_command_line(linphonec, iptr);
linphonec_command_finished();
free(input);
}
return 0;
}
/***************************************************************************
*
* Public interface
*
***************************************************************************/
/*
* Main command dispatcher.
* WARNING: modifies second argument!
*
* Always return 1 currently.
*/
int
linphonec_parse_command_line(LinphoneCore *lc, char *cl)
{
char *ptr=cl;
char *args=NULL;
LPC_COMMAND *cmd;
/* Isolate first word and args */
while(*ptr && !isspace(*ptr)) ++ptr;
if (*ptr)
{
*ptr='\0';
/* set args to first nonblank */
args=ptr+1;
while(*args && isspace(*args)) ++args;
}
/* Handle DTMF */
if ( isdigit(*cl) || *cl == '#' || *cl == '*' )
{
while ( isdigit(*cl) || *cl == '#' || *cl == '*' )
{
linphone_core_send_dtmf(lc, *cl);
linphone_core_play_dtmf (lc,*cl,100);
ms_sleep(1); // be nice
++cl;
}
// discard spurious trailing chars
return 1;
}
/* Handle other kind of commands */
cmd=lpc_find_command(cl);
if ( !cmd )
{
linphonec_out("'%s': Cannot understand this.\n", cl);
return 1;
}
if ( ! cmd->func(lc, args) )
{
linphonec_out("Syntax error.\n");
linphonec_display_command_help(cmd);
}
return 1;
}