你处理过多线程中的异常吗?如何捕获多线程中发生的异常?捕获子线程的异常与捕获当前线程的异常一样简单吗?
除了try catch。Java中还可以通过异常处理器
UncaughtExceptionHandler
来处理那些未捕获的异常。
# 在当前线程捕获当前线程发生的异常:
/**
* @author futao
* @date 2020/6/17
*/
@Slf4j
public class ExceptionInCurThread {
public static void main(String[] args) {
try {
throw new RuntimeException("在主线程抛出异常,在主线程捕获");
} catch (RuntimeException e) {
log.error("捕获到异常", e);
}
}
}
结果:

结论:在当前线程通过
try catch
可以捕获当前线程抛出的异常。
# 可以在当前通过try catch
的方式捕获其他线程抛出的异常吗?'
/**
* @author 喜欢天文的pony站长
* Created on 2020/6/16.
*/
public class ExceptionInChildThread implements Runnable {
@Override
public void run() {
throw new RuntimeException("子线程发生了异常...");
}
/**
* 模拟子线程发生异常
*
* @throws InterruptedException
*/
private static void exceptionThread() throws InterruptedException {
new Thread(new ExceptionInChildThread()).start();
TimeUnit.MILLISECONDS.sleep(200L);
new Thread(new ExceptionInChildThread()).start();
TimeUnit.MILLISECONDS.sleep(200L);
new Thread(new ExceptionInChildThread()).start();
TimeUnit.MILLISECONDS.sleep(200L);
new Thread(new ExceptionInChildThread()).start();
TimeUnit.MILLISECONDS.sleep(200L);
}
/**
* 在主线程尝试通过try catch捕获异常
*/
private static void catchInMain() {
try {
exceptionThread();
} catch (Exception e) {
//无法捕获发生在其他线程中的异常
log.error("捕获到了异常?", e);
}
}
public static void main(String[] args) throws InterruptedException {
ExceptionInChildThread.catchInMain();
}
}
(错误的)预期:
在运行第一个线程的时候发生了异常,被catch捕获,打印
捕获到了异常?
和异常堆栈且后面的线程将不会运行。
实际运行结果:
并不符合预期。
没有被try catch捕获。
后续的线程没有因为第一个线程发生异常而跳过。
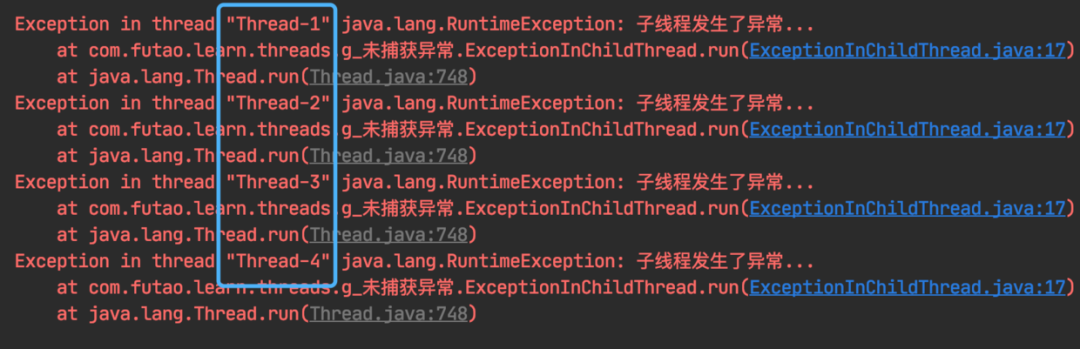
结论:
无法在一个线程中通过try catch捕获另外一个线程的异常。
# 解决方案
在每个线程内部run()方法内通过try catch捕获当前线程发生的异常。
缺点:每个线程都需要编写重复的try catch 代码
使用线程异常处理器
UncaughtExceptionHandler
给所有线程设置统一的异常处理器
给每个线程设置特定的异常处理器
给线程组设置异常处理器
给线程池设置异常处理器
因为线程池也是通过
new Thread()
的方式创建的线程,所以思想与上面两种方法一致。注意:
execute()
与submit()
方式对 异常处理的不同。
# 在线程内部
run()
通过try catch捕获异常/** * @author 喜欢天文的pony站长 * Created on 2020/6/16. */ @Slf4j public class ExceptionInChildThread implements Runnable { @Override public void run() { try { //do something else... throw new RuntimeException("子线程发生了异常..."); } catch (Exception e) { log.error("在线程内部捕获异常", e); } } /** * 模拟子线程发生异常 * * @throws InterruptedException */ private static void exceptionThread() throws InterruptedException { new Thread(new ExceptionInChildThread()).start(); TimeUnit.MILLISECONDS.sleep(200L); new Thread(new ExceptionInChildThread()).start(); TimeUnit.MILLISECONDS.sleep(200L); new Thread(new ExceptionInChildThread()).start(); TimeUnit.MILLISECONDS.sleep(200L); new Thread(new ExceptionInChildThread()).start(); TimeUnit.MILLISECONDS.sleep(200L); } /** * 在主线程尝试通过try catch捕获异常 */ private static void catchInMain() { try { exceptionThread(); } catch (Exception e) { //无法捕获发生在其他线程中的异常 log.error("捕获到了异常?", e); } } public static void main(String[] args) throws InterruptedException { ExceptionInChildThread.catchInMain(); } }
结果:
成功在子线程内部
run()
方法捕获到了异常
image.png # 使用线程异常处理器
UncaughtExceptionHandler
当一个线程由于未捕获异常而退出时,JVM会把这个事件报告给应用程序提供的UncaughtExceptionHandler异常处理器
自定义线程异常处理器
/** * 自定义线程未捕获异常处理器 * * @author futao * @date 2020/6/17 */ public class CustomThreadUncaughtExceptionHandler implements Thread.UncaughtExceptionHandler { private static final Logger LOGGER = LoggerFactory.getLogger(CustomThreadUncaughtExceptionHandler.class); @Override public void uncaughtException(Thread t, Throwable e) { LOGGER.error("捕获到线程发生的异常,线程信息:[{}]", JSON.toJSONString(t), e); } }
使用:
1. 全局:
Thread.setDefaultUncaughtExceptionHandler(new CustomThreadUncaughtExceptionHandler());
通过调用Thread的静态方法
setDefaultUncaughtExceptionHandler()
,设置Thread的静态属性defaultUncaughtExceptionHandler
.为我们自定义的异常处理器。源码:
测试:
/** * @author 喜欢天文的pony站长 * Created on 2020/6/16. */ @Slf4j public class ExceptionInChildThread implements Runnable { @Override public void run() { throw new RuntimeException("子线程发生了异常..."); } /** * 模拟子线程发生异常 * * @throws InterruptedException */ private static void exceptionThread() throws InterruptedException { new Thread(new ExceptionInChildThread()).start(); TimeUnit.MILLISECONDS.sleep(200L); new Thread(new ExceptionInChildThread()).start(); TimeUnit.MILLISECONDS.sleep(200L); new Thread(new ExceptionInChildThread()).start(); TimeUnit.MILLISECONDS.sleep(200L); new Thread(new ExceptionInChildThread()).start(); TimeUnit.MILLISECONDS.sleep(200L); } public static void main(String[] args) throws InterruptedException { //设置全局的线程异常处理器 Thread.setDefaultUncaughtExceptionHandler(new CustomThreadUncaughtExceptionHandler()); exceptionThread(); } }
结果: 成功捕获
image.png 2. 为指定线程设置特定的异常处理器
细心的同学已经发现了,在上面Thread类的截图中,还有一个实例属性
private volatile UncaughtExceptionHandler uncaughtExceptionHandler;
。通过给这个属性赋值,可以实现为每个线程对象设置不同的异常处理器。测试使用
/** * @author 喜欢天文的pony站长 * Created on 2020/6/16. */ @Slf4j public class ExceptionInChildThread implements Runnable { @Override public void run() { throw new RuntimeException("子线程发生了异常..."); } /** * 模拟子线程发生异常 * * @throws InterruptedException */ private static void exceptionThread() throws InterruptedException { Thread thread1 = new Thread(new ExceptionInChildThread()); //为指定线程设置特定的异常处理器 thread1.setUncaughtExceptionHandler(new CustomThreadUncaughtExceptionHandler()); thread1.start(); TimeUnit.MILLISECONDS.sleep(200L); new Thread(new ExceptionInChildThread()).start(); TimeUnit.MILLISECONDS.sleep(200L); new Thread(new ExceptionInChildThread()).start(); TimeUnit.MILLISECONDS.sleep(200L); new Thread(new ExceptionInChildThread()).start(); TimeUnit.MILLISECONDS.sleep(200L); } public static void main(String[] args) throws InterruptedException { exceptionThread(); } }
结果: 成功捕获线程1的异常信息
3. 线程组
/** * @author futao * @date 2020/6/20 */ @Slf4j public class ExceptionInThreadGroup implements Runnable { @Override public void run() { throw new RuntimeException("线程任务发生了异常"); } public static void main(String[] args) throws InterruptedException { ThreadGroup threadGroup = new ThreadGroup("只知道抛出异常的线程组...") { @Override public void uncaughtException(Thread t, Throwable e) { super.uncaughtException(t, e); log.error("线程组内捕获到线程[{},{}]异常", t.getId(), t.getName(), e); } }; ExceptionInThreadGroup exceptionInThreadGroup = new ExceptionInThreadGroup(); new Thread(threadGroup, exceptionInThreadGroup, "线程1").start(); TimeUnit.MILLISECONDS.sleep(300L); //优先获取绑定在thread对象上的异常处理器 Thread thread = new Thread(threadGroup, exceptionInThreadGroup, "线程2"); thread.setUncaughtExceptionHandler(new CustomThreadUncaughtExceptionHandler()); thread.start(); TimeUnit.MILLISECONDS.sleep(300L); new Thread(threadGroup, exceptionInThreadGroup, "线程3").start(); } }
结果:
4. 线程池
/** * @author futao * @date 2020/6/17 */ public class CatchThreadPoolException { public static void main(String[] args) { ThreadPoolExecutor threadPoolExecutor = new ThreadPoolExecutor( 2, 4, 1L, TimeUnit.MINUTES, new LinkedBlockingDeque<>(1024), new ThreadFactory() { @Override public Thread newThread(Runnable r) { Thread thread = new Thread(r); //设置线程异常处理器 thread.setUncaughtExceptionHandler(new CustomThreadUncaughtExceptionHandler()); return thread; } } ); threadPoolExecutor.execute(new Runnable() { @Override public void run() { throw new RuntimeException("execute()发生异常"); } } ); threadPoolExecutor.submit(new Runnable() { @Override public void run() { throw new RuntimeException("submit.run()发生异常"); } }); threadPoolExecutor.submit(new Callable<String>() { @Override public String call() throws Exception { throw new RuntimeException("submit.call()发生异常"); } }); threadPoolExecutor.shutdown(); } }
结果: 并不符合预期,预期应该捕获三个异常
只捕获到了通过execute()提交的任务的异常
没有捕获到通过submit()提交的任务的异常
通过
afterExecute()
捕获submit()
任务的异常通过
submit()
方法的源码可以发现,submit()
是将runnable()
封装成了RunnableFuture<Void>
,并最终调用execute(ftask);
执行。
/** * @author futao * @date 2020/6/17 */ @Slf4j public class CatchThreadPoolException { public static void main(String[] args) throws InterruptedException, ExecutionException { ThreadPoolExecutor threadPoolExecutor = new ThreadPoolExecutor( 2, 4, 1L, TimeUnit.MINUTES, new LinkedBlockingDeque<>(1024), new ThreadFactory() { @Override public Thread newThread(Runnable r) { Thread thread = new Thread(r); //设置线程异常处理器 thread.setUncaughtExceptionHandler(new CustomThreadUncaughtExceptionHandler()); return thread; } } ) { /** * 捕获{@code FutureTask<?>}抛出的异常 * * @param r * @param t */ @Override protected void afterExecute(Runnable r, Throwable t) { super.afterExecute(r, t); if (r instanceof FutureTask<?>) { try { //get()的时候会将异常内的异常抛出 ((FutureTask<?>) r).get(); } catch (InterruptedException e) { e.printStackTrace(); Thread.currentThread().interrupt(); } catch (ExecutionException e) { log.error("捕获到线程的异常返回值", e); } } //Throwable t永远为null,拿不到异常信息 //log.error("afterExecute中捕获到异常,", t); } }; threadPoolExecutor.execute(new Runnable() { @Override public void run() { throw new RuntimeException("execute()发生异常"); } } ); TimeUnit.MILLISECONDS.sleep(200L); threadPoolExecutor.submit(new Runnable() { @Override public void run() { throw new RuntimeException("submit.run()发生异常"); } }); TimeUnit.MILLISECONDS.sleep(200L); threadPoolExecutor.submit(new Callable<String>() { @Override public String call() throws Exception { throw new RuntimeException("submit.call()发生异常"); } }).get(); //get()的时候会将异常抛出 threadPoolExecutor.shutdown(); } }
欢迎在评论区留下你看文章时的思考,及时说出,有助于加深记忆和理解,还能和像你一样也喜欢这个话题的读者相遇~
# 本文源代码
https://github.com/FutaoSmile/learn-thread/tree/master/src/main/java/com/futao/learn/threads/捕获线程异常
# 系列文章