-
路由跳转
-
核心代码
import { useRouter,useRoute } from 'vue-router'; const $router = useRouter() const $route = useRoute() const toAbout = () => { console.log($router) $router.push('/about/888') }
-
全部代码
-
常量路由
export const constantRoute = [ { path: '/about/:id', component:()=> import('../vue-base/about1.vue') }, { path: '/', component:()=> import('../vue-base/home.vue') }, { path: '/about', component:()=> import('../vue-base/about.vue') }, { path: '/:pathMatch(.*)*', name: 'NotFound', component: ()=>import('../vue-base/404.vue') }, ]
-
home.vue
<template> <div> <h1>我是home</h1> <router-link to="/about/123">Go to about1</router-link> <br> <button @click="toAbout">点我前往About页面</button> </div> </template> <script setup lang="ts"> import { useRouter } from 'vue-router'; let $router = useRouter() const toAbout = () => { console.log($router) $router.push('/about') } </script> <style scoped></style>
-
about.vue
<template> <div> <h1>about</h1> <router-link to="/home">Go to Home</router-link> <br> <button @click="toAbout">点我前往About1页面</button> </div> </template> <script setup lang="ts"> import { useRouter,useRoute } from 'vue-router'; const $router = useRouter() const $route = useRoute() console.log($route) const toAbout = () => { console.log($router) $router.push('/about/888') } </script> <style scoped></style>
-
about1.vue
<template> <div> home1 <br> <button @click="toHome">点我也可以前往Home页面</button> <h1>{{ params}}</h1> </div> </template> <script setup lang="ts"> import { useRouter,useRoute } from 'vue-router'; import { ref,computed } from 'vue'; let params = ref() const $router = useRouter() const $route = useRoute() console.log($route) params.value = computed(()=> Number($route.params.id) ) const toHome = () => { console.log($router) $router.push('/') } </script> <style scoped></style>
-
效果
-
home
-
about
-
about1
-
404
-
-
-
-
带参数的动态路由匹配
- 核心代码
export const constantRoute = [ { path: '/about/:id', component:()=> import('../vue-base/about1.vue') }, ]
-
匹配途径
- 核心代码
-
404路由
- 核心代码
export const constantRoute = [ { path: '/:pathMatch(.*)*', name: 'NotFound', component: ()=>import('../vue-base/404.vue') }, ]
- 核心代码
-
嵌套路由
- 核心代码
export const constantRoute = [ { path: '/about/:id', component:()=> import('../vue-base/about1.vue'), redirect:'/about/:id/AboutChildren1', children:[ { path:'AboutChildren1', component:()=>import('../vue-base/aboutChildren1.vue') }, { path:'AboutChildren2', component:()=>import('../vue-base/aboutChildren2.vue') } ] } ]
- about1.vue
<template> <div> about1 <br> <button @click="toHome">点我也可以前往Home页面</button> <h1>{{ params}}</h1> <button @click="toAboutChildren">点我可以前往AboutChildren页面</button> <router-view></router-view> </div> </template> <script setup lang="ts"> import { useRouter,useRoute } from 'vue-router'; import { ref,computed } from 'vue'; let params = ref() const $router = useRouter() const $route = useRoute() console.log($route) params.value = computed(()=> Number($route.params.id) ) const toHome = () => { console.log($router) $router.push('/') } const toAboutChildren = ()=>{ $router.push('/about/8888/AboutChildren1') } </script> <style scoped></style>
- aboutChildren1.vue
<template> <div> <h1>我是AboutChildren1</h1> <br> <router-link to="/"></router-link> </div> </template> <script setup lang="ts"> </script> <style scoped></style>
- aboutChildren2.vue
<template> <div> <h1>我是AboutChildren2</h1> <br> <router-link to="/"></router-link> </div> </template> <script setup lang="ts"> </script> <style scoped></style>
- 效果
- about
- aboutChildren1
- aboutChildren2
- about
- 核心代码
vue-router 路由跳转、带参动态路由匹配、404路由和嵌套路由
于 2024-03-19 14:52:16 首次发布
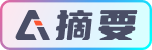