一、HTML页面代码如下:
登录界面
<!DOCTYPE html>
<html lang="zh-cn">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>登陆界面</title>
<link rel="stylesheet" type="text/css" href="login.css" />
<script type="text/javascript" src="login.js"></script>
</head>
<body class="body">
<div class="login_frame">
<div class="box_0">
<div class="box_1">
<img id="logo_image" src="images/OIP.png" alt="网站logo" />
</div>
<form method="POST" action="date.jsp">
<div class="box_2">
<p><label style="font-size: 14px ;" class="label_input">账号:</label><input type="text" id="username" class="text_field" placeholder="请输入账号" autofocus="autofocus" /></p>
<p><label style="font-size: 14px ;" class="label_input">密码:</label><input type="password" id="password" class="text_field" placeholder="请输入密码" /></p>
</div>
<div class="box_3">
<input type="button" id="btn_login" value="登录" onclick="login()" />
<input type="button" id="btn_login" value="注册" onclick="register()" />
</div>
<div class="box_4"> 欢迎您</div>
</form>
</div>
</div>
</body>
</html>
注册界面
<!DOCTYPE html>
<html lang="zh-cn">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>注册界面</title>
<link rel="stylesheet" type="text/css" href="register.css" />
<script type="text/javascript" src="register.js"></script>
</head>
<body>
<div class="frame">
<div class="box_1">
<img src="images/OIP.png" alt="网站logo" width="120px" height="60px" />
</div>
<div class="box_2">
<h1 class="title">注册个人账号</h1>
</div>
<div class="box_3">
<form class="register-form" action="#" name="myform" method="POST">
<h3>账号</h3>
<input onkeyup="value=value.replace(/[^\w\.\/]/ig,'')" type="text" name="account" placeholder="请输入账号" maxlength="11">
<h3>设置密码</h3>
<input type="password" name="password1" placeholder="请输入密码">
<h3>确认密码</h3>
<input type="password" name="password2" placeholder="请确认密码"><br/>
<input type="button" value="立即注册" class="submit" onclick="checksub()">
</form>
</div>
</div>
</body>
</html>
二、CSS代码如下:
登录界面CSS:
.body {
background-attachment: fixed;
background-image: url(images/background.jpg);
background-repeat: no-repeat;
background-size: cover;
}
.login_frame {
width: 480px;
background-attachment: fixed;
margin: 300px auto;
padding: 20px 0 20px;
border-radius: 20px 20px 20px 20px;
}
.box_0 {
width: 320px;
border: 1px solid #deb068;
background: rgba(228,210,216,0.6);
margin: 0 auto;
border-radius: 20px 20px 20px 20px;
}
.box_1 {
width: 320px;
height: 50px;
text-align: center
}
.box_2 {
width: 320px;
height: 60px;
padding: 0 0 0 55px;
}
.box_3 {
width: 320px;
height: 30px;
text-align: center;
margin-top: 10px;
}
.box_4 {
width: 320px;
height: 25px;
border: 1px solid #deb068;
border-style: solid none none none;
padding: auto;
text-align: center;
font-size: 16px;
}
#logo_image {
width: 120px;
height: 60px;
}
注册界面CSS:
body {
background: #F9F9F9;
}
.frame {
width: 330px;
margin: 0 auto;
}
.box_1 {
text-align: center;
width: 330px;
height: 60px;
margin: 0 auto;
}
.box_2 {
text-align: center;
width: 330px;
height: 50px;
margin: 0 auto;
}
.box_3 {
text-align: center;
width: 330px;
margin: 0 auto;
}
.box_1 a {
width: 100%;
height: 100%;
background-image: url('images/OIP.png');
}
.title {
font-size: 24px;
}
.register-form {
width: 330px;
height: 450px;
margin: 5px auto 0;
}
.register-form h3 {
font-size: 14px;
line-height: 5px;
text-align: left;
}
.register-form input {
width: 330px;
height: 43px;
box-sizing: border-box;
border: 1px solid #E8E8E8;
font-size: 14px;
padding: 5px;
}
.register-form input:first-of-type {
margin-bottom: 5px;
}
.register-form .submit {
border: none;
background: #FF6700;
color: white;
margin-top: 30px;
cursor: pointer;
}
三、JavaScript代码如下:
登录界面JavaScript:
function login() {
var username = document.getElementById("username");
var pass = document.getElementById("password");
if (username.value == "") {
alert("请输入用户名");
} else if (pass.value == "") {
alert("请输入密码");
} else {
alert("请输入正确的用户名和密码!")
}
}
function register() {
window.location = "register.html"
}
注册界面JavaScript:
function checksub() {
if (!myform.account.value) {
alert("请输入账号!");
myform.account.focus();
return false;
} else if (!myform.password1.value) {
alert("请输入密码!");
myform.password1.focus();
} else if (myform.password1.value != myform.password2.value) {
alert("密码不一致!");
myform.password2.focus();
}else
myform.submit();
}
四、效果图:
登录界面:
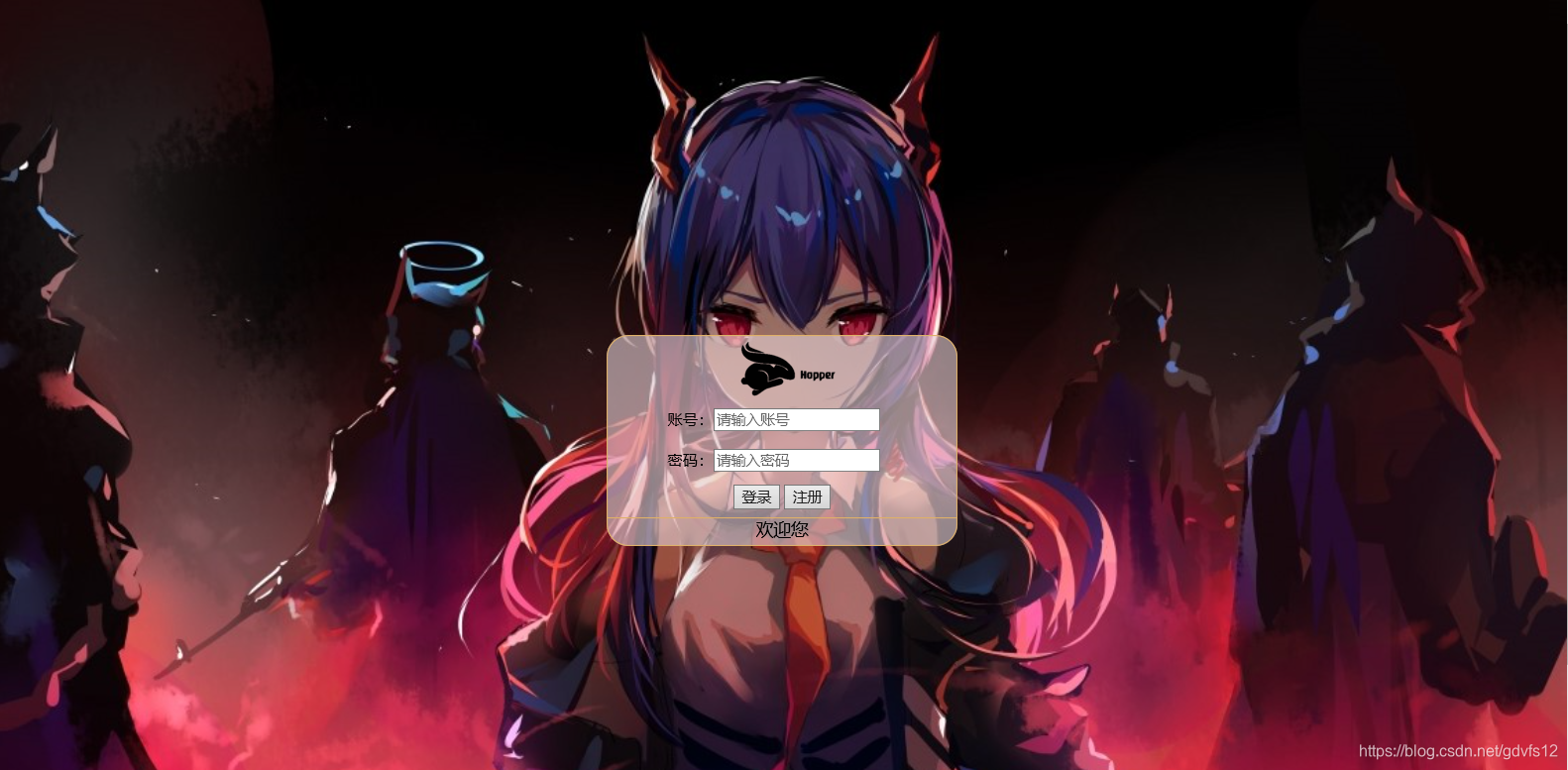
注册界面:
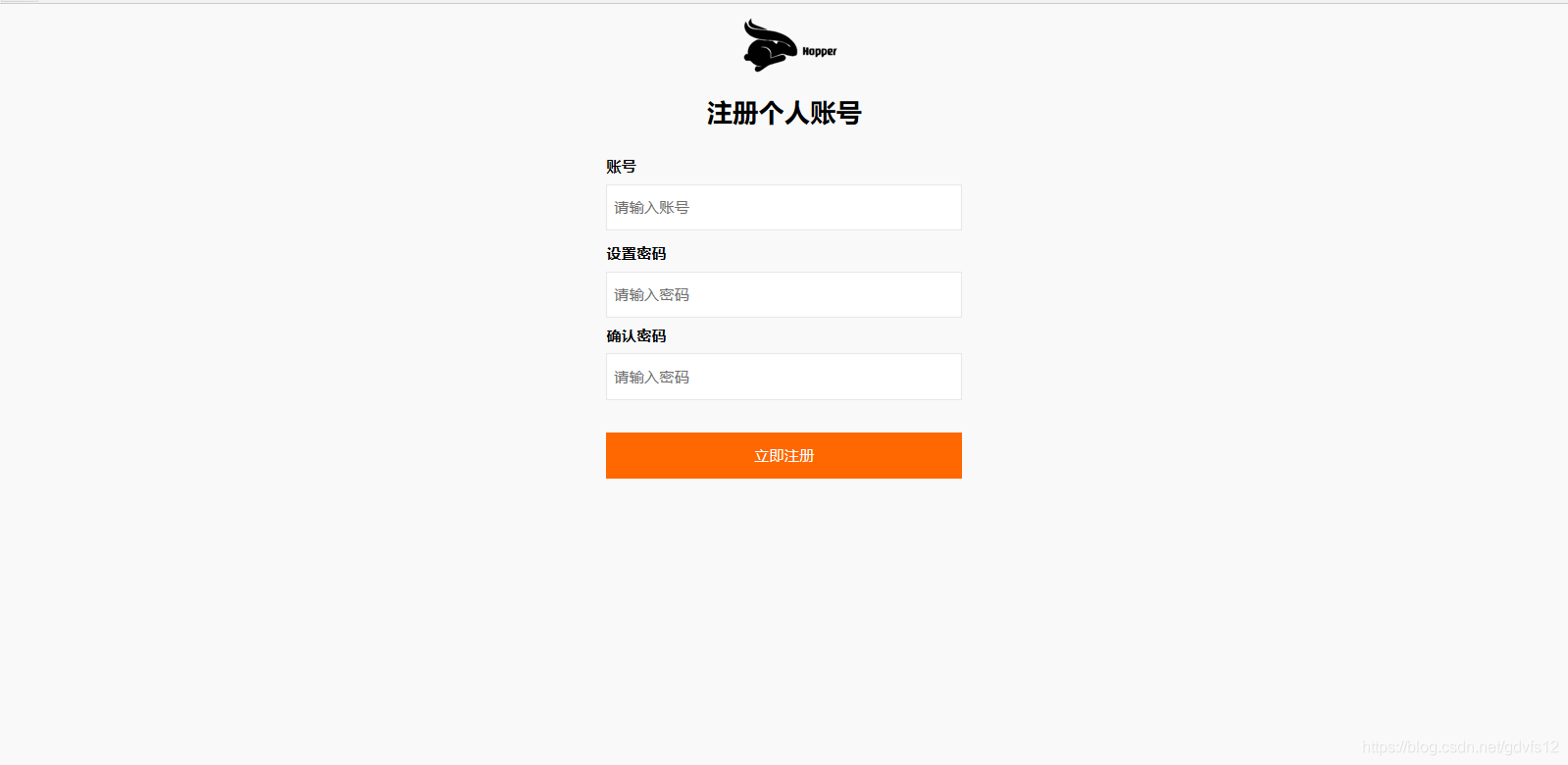