1 jsp部分
<input id="uploadPoto" style="display: none" class ="upload-btn" type="file" />
<div id="uploadBase" class="layui-btn" lay-submit=""><i class="layui-icon layui-icon-upload-drag"></i>上传</div>
1.2 js部分
// 文件改变时 将文件名称显示到p元素中
$('#uploadPoto').on('change', function(event) {
if (this.files[0] == undefined || '' == this.files[0]) {
return false;
}
var fileType = $("#fileType").val();
var docType = $("#docType").val();
var imagSize = document.getElementById("uploadPoto").files[0].size;
if(imagSize>1024*1024*10){
alert("文件大小要求在10M以内");
$("#uploadPoto").val("");
return false;
}
var formData = new FormData();
formData.append('file',this.files[0]); //添加图片信息的参数
formData.append("scriptId", "");
formData.append("vendorCode", "");
formData.append("fileType", fileType);
formData.append("docType", docType);
formData.append("vendorName", "");
$.ajax({
url: "${ctx}/knowledgeBase/fileManage/uploadFile",
type: "post",
dataType: "json",
cache: false,
data: formData,
processData: false,// 不处理数据
contentType: false, // 不设置内容类型
success: function (data) {
if(data.success == true){
layer.alert("上传成功");
reladTable();
}else{
$.msgAlert("系统提示", "上传失败", "error");
}
},complete: function () {
initDownload();
//setTimeout(function(){removeProcess()},1000);
},
})
})
2 后台部分
2.1 nginx配置
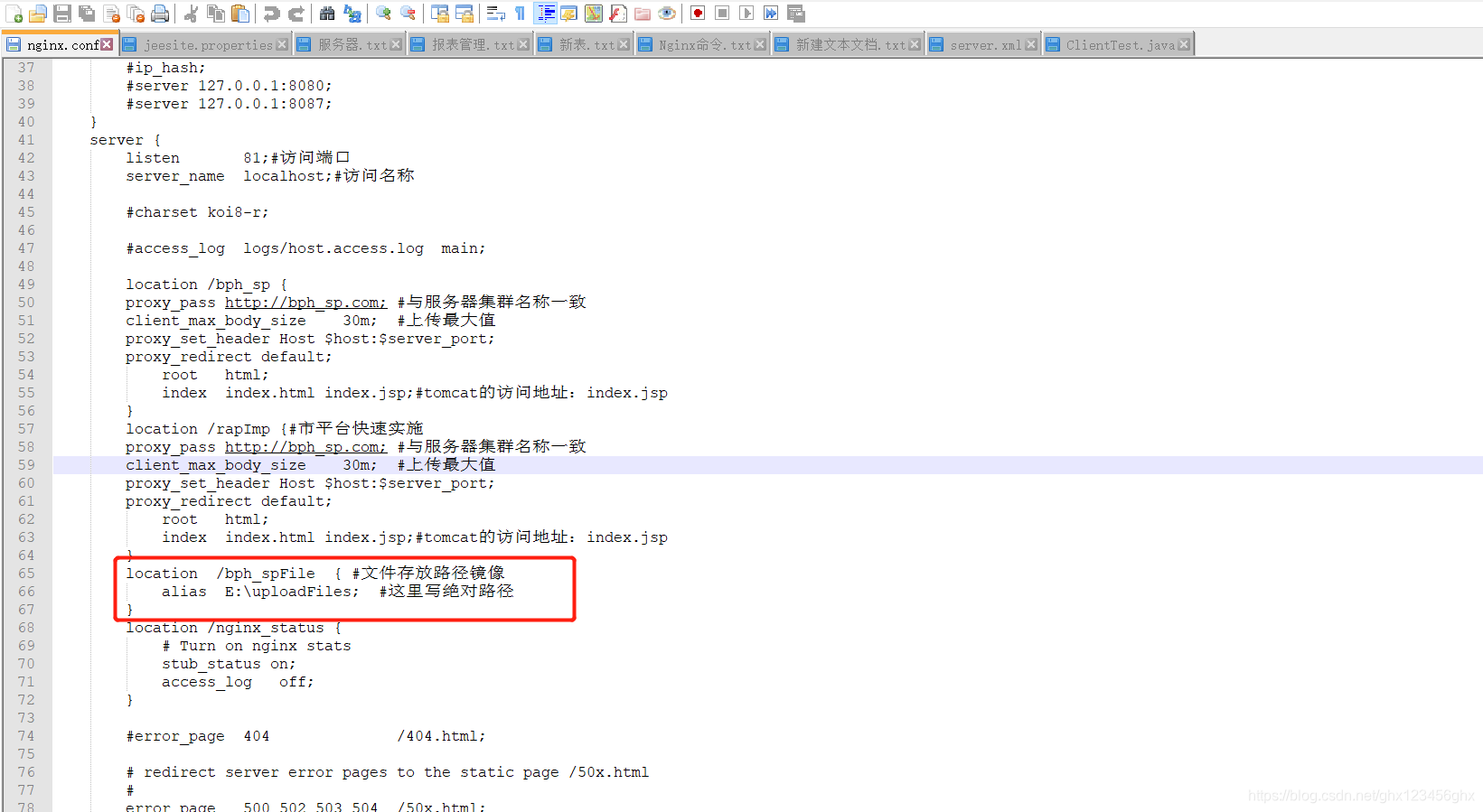
2.2 文件配置
LOCATION_PATH=E\:\\uploadFiles\\
MIRROR_PATH=/bph_spFile(此路径随便写,和nginx配置的映射一致)
IMG_PATH=img/
2.3 获取配置信息
<util:properties id="propertiesReader" location="classpath:jeesite.properties"/>
@Value("#{propertiesReader['MIRROR_PATH']}")
private String MIRROR_PATH;//nginx中镜像文件访问路径
@Value("#{propertiesReader['LOCATION_PATH']}")
private String LOCATION_PATH;
@Value("#{propertiesReader['IMG_PATH']}")
private String IMG_PATH;
3 后台:
/**
* 上传文件
*
* @param file
* @param path
* @return
*/
@RequestMapping(value = "/uploadFile", produces = "text/json;charset=UTF-8")
public @ResponseBody String uploadWangImg(@RequestParam("file") MultipartFile[] myFiles,
@RequestParam(required = true, value = "scriptId") String scriptId,
@RequestParam(required = true, value = "vendorCode") String vendorCode,
@RequestParam(required = true, value = "fileType") String fileType,
@RequestParam(required = true, value = "vendorName") String vendorName,
@RequestParam(required = false, value = "docType") String docType,
HttpServletRequest request, HttpSession session) {
String pathurl = MIRROR_PATH;
String basePathurl = request.getScheme() + "://" + request.getServerName() + ":"
+ request.getServerPort() + pathurl + "/";
String HTTP_URL = basePathurl + "upload/" +fileType + "/";
String folderPath = LOCATION_PATH;
folderPath = folderPath + "upload/"+fileType+"/";
List<Map<String, String>> fileRandomNameList = null;
Map<String,Object> result = new HashMap<String,Object>();
String imgUrl = "";
// 创建图片保存路径
String path = folderPath + IMG_PATH;
ObjectMapper objectMapper = new ObjectMapper();
try {
fileRandomNameList = FileUtils.uploadImages(path, myFiles);
if (fileRandomNameList.size() > 0) {
for (Map<String, String> fileMap : fileRandomNameList) {
String thefileName = fileMap.get("newFileName");
String oldFileName = fileMap.get("oldFileName");
if (null != thefileName) {
FileRecord fileRecord = new FileRecord();
fileRecord.setId(IdGen.uuid());
//服务器存放文件路径
String inputFile = path + thefileName;
String ext = GetFileExt(thefileName);//xlsx
//图片类文件
if (ext.endsWith("jpg") || ext.endsWith("png") || ext.endsWith("jpeg")) {
fileRecord.setType("1");
imgUrl = HTTP_URL + IMG_PATH + thefileName;//数据库存储地址路径
// htmlUrl = imgUrl;
}
//文档类文件
else if (ext.endsWith("docx") || ext.endsWith("doc") || ext.endsWith("xls")
|| ext.endsWith("xlsx") || ext.endsWith("pdf")) {
fileRecord.setType("2");
}
//压缩类文件
else if (ext.endsWith("rar") || ext.endsWith("zip")) {
fileRecord.setType("3");
}else {
fileRecord.setType("4");
}
//保存數據庫
fileRecord.setScriptId(scriptId);
fileRecord.setFileName(oldFileName);
fileRecord.setFilePath(imgUrl);
fileRecord.setRealPath(inputFile);
fileRecord.setDocType(docType);
fileRecord.setFileType(fileType);
fileRecordService.insert(fileRecord);
}
}
result.put("success", true);
result.put("msg", "保存成功");
result.put("scriptId", scriptId);
} else {
result.put("success", false);
result.put("msg", "保存失败,文件格式错误");
}
} catch (Exception e) {
result.put("success", false);
result.put("msg", "保存失败");
}
String json;
try {
json = objectMapper.writeValueAsString(result);
return json;
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
public static String GetFileExt(String name) {
String ext = null;
int i = name.lastIndexOf('.');
if (i > 0 && i < name.length() - 1) {
ext = name.substring(i + 1).toLowerCase();
}
return ext;
}
4 FileUtils
package com.msunsoft.common.utils.file;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Random;
import org.apache.commons.collections.CollectionUtils;
import org.apache.commons.lang3.time.DateFormatUtils;
import org.springframework.web.multipart.MultipartFile;
import com.msunsoft.common.utils.Constants;
import com.msunsoft.common.utils.ConvertStringUtil;
public class FileUtils {
public static final String data_root_dir = FileUtils.getWebrootPath("upload");
public static final String data_download_dir = FileUtils.getWebrootPath("download");
public static String getExtention(String fileName) {
int index = fileName.lastIndexOf(".");
if (index != -1 && index < fileName.length() - 1) {
return fileName.substring(index + 1);
} else {
return "";
}
}
public static void copy(String sourceFilePath, String targetFilePath) {
try {
FileInputStream fileInputStream = new FileInputStream(sourceFilePath);
copy(fileInputStream, targetFilePath);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public static void copy(File inputFile, String targetFilePath) {
try {
FileInputStream fileInputStream = new FileInputStream(inputFile);
copy(fileInputStream, targetFilePath);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public static void copy(InputStream inputStream, String targetChildFilePath) {
try {
BufferedInputStream input = new BufferedInputStream(inputStream);
File outputFile = new File(targetChildFilePath);
if (outputFile.exists()) {
outputFile.delete();
}
File parentFile = outputFile.getParentFile();
if (!parentFile.exists()) {
parentFile.mkdirs();
}
BufferedOutputStream output = new BufferedOutputStream(new FileOutputStream(outputFile));
byte[] b = new byte[1024 * 5];
int len;
while ((len = input.read(b)) != -1) {
output.write(b, 0, len);
}
output.flush();
output.close();
input.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public static String getFileType(File file) {
String fileName = file.getName();
return getFileType(fileName);
}
public static String getFileType(String fileName) {
int lastDotIndex = fileName.lastIndexOf(".");
if (lastDotIndex > -1) {
return fileName.substring(lastDotIndex + 1).toLowerCase();
}
return "";
}
public static void close(InputStream stream) {
if (stream != null) {
try {
stream.close();
} catch (IOException e) {
// ignore
}
}
}
public static void deleteFile(String file) {
new File(file).delete();
}
public static String readFileStream(String filePath, String encode) throws IOException {
StringBuilder sb = new StringBuilder();
BufferedReader br = new BufferedReader(new InputStreamReader(new FileInputStream(filePath),
encode));
try {
String temp = null;
while ((temp = br.readLine()) != null) {
sb.append(temp).append("\n");
}
} finally {
if (br != null) {
br.close();
}
}
return sb.toString();
}
public static void write(String content, File file, String encoding) {
try {
file.getParentFile().mkdirs();
OutputStreamWriter fileWriter = new OutputStreamWriter(new FileOutputStream(file),
encoding);
fileWriter.write(content);
fileWriter.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public static String getEncodeName(String unpackEncode, String targetName) {
return targetName + "_" + unpackEncode;
}
/**
* 判断文件是否存在
*
* @param fileName
* @param dir
* @return
*/
public static boolean isFileExist(String fileName, String dir) {
File files = new File(dir + fileName);
return (files.exists()) ? true : false;
}
/**/
public static String randomRename(String fileName, String dir) {
String[] split = fileName.split("\\.");// 将文件名已.的形式拆分
String extendFile = "." + split[split.length - 1].toLowerCase(); // 获文件的有效后缀
Random random = new Random();
int add = random.nextInt(1000000); // 产生随机数10000以内
String ret = add + extendFile;
while (isFileExist(ret, dir)) {
add = random.nextInt(1000000);
ret = fileName + add + extendFile;
}
File file = new File(dir + fileName);
File reFile = new File(dir + ret);
file.renameTo(reFile);
String name = reFile.getName();
file = null;
reFile = null;
return name;
}
/**
* 获得随机文件名,保证在同一个文件夹下不同名
*
* @param fileName
* @param dir
* @return
*/
public static String getRandomName(String fileName, String dir) {
String[] split = fileName.split("\\.");// 将文件名已.的形式拆分
String extendFile = "." + split[split.length - 1].toLowerCase(); // 获文件的有效后缀
Random random = new Random();
int add = random.nextInt(1000000); // 产生随机数10000以内
String ret = add + extendFile;
while (isFileExist(ret, dir)) {
add = random.nextInt(1000000);
ret = fileName + add + extendFile;
}
return ret;
}
/**
* 以时间戳命名
*
* @return
*/
public static String getTimeStampName(String fileName, String dir) {
String[] split = fileName.split("\\.");// 将文件名已.的形式拆分
String extendFile = "." + split[split.length - 1].toLowerCase(); // 获文件的有效后缀
long add = System.nanoTime();
String ret = add + extendFile;
while (isFileExist(ret, dir)) {
add = System.nanoTime();
ret = fileName + add + extendFile;
}
return ret;
}
public static String getAddTimeStampName(String fileName) {
String[] split = fileName.split("\\.");// 将文件名已.的形式拆分
String extendFile = "." + split[split.length - 1].toLowerCase(); // 获文件的有效后缀
long add = System.nanoTime();
String ret = split[0] + "("+add+")"+extendFile;
return ret;
}
/**
* 上传文件
*
* @param uploadFileName 被上传的文件名称
* @param savePath 文件的保存路径
* @param uploadFile 被上传的文件
* @return newFileName
*/
public static String upload(String uploadFileName, String savePath, File uploadFile) {
String newFileName = getRandomName(uploadFileName, savePath);
FileOutputStream fos = null;
FileInputStream fis = null;
try {
fos = new FileOutputStream(savePath + newFileName);
fis = new FileInputStream(uploadFile);
byte[] buffer = new byte[1024];
int len = 0;
while ((len = fis.read(buffer)) > 0) {
fos.write(buffer, 0, len);
}
} catch (FileNotFoundException e) {
Constants.msunsoftlog.error("Woyundata Exception:"
+ ConvertStringUtil.exceptionToString(e));
} catch (IOException e) {
Constants.msunsoftlog.error("Woyundata Exception:"
+ ConvertStringUtil.exceptionToString(e));
} finally {
// 关流
if (fos != null) {
try {
fos.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
if (fis != null) {
try {
fis.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
return newFileName;
}
/**
* 上传的图片
*
* @param uploadFileName 被上传的文件名称
* @param savePath 文件的保存路径
* @param imageData 被上传的图片二进制流
* @return newFileName
*/
public static String uploadImage(String uploadFileName, String savePath, byte[] imageData) {
BufferedOutputStream bos = null;
FileOutputStream fos = null;
File file = null;
String newFileName = null;
String todayFolder = getDailyFilePath();
try {
String pathStr = savePath + todayFolder;
File path = new File(pathStr);
if (!path.exists()) {
path.mkdirs();
}
newFileName = getTimeStampName(uploadFileName, pathStr);
// fos = new FileOutputStream(savePath + newFileName);
file = new File(pathStr + newFileName);
// if(!file.exists()){//判断文件是否存在
// file.mkdirs();
// }
// else {
// return null;
// }
// file = new File(filePath+"\\"+fileName);
fos = new FileOutputStream(file);
bos = new BufferedOutputStream(fos);
bos.write(imageData);
} catch (Exception e) {
Constants.msunsoftlog.error("Woyundata Exception:"
+ ConvertStringUtil.exceptionToString(e));
} finally {
if (bos != null) {
try {
bos.close();
} catch (IOException e1) {
}
}
if (fos != null) {
try {
fos.close();
} catch (IOException e1) {
Constants.msunsoftlog.error("Woyundata Exception:"
+ ConvertStringUtil.exceptionToString(e1));
}
}
}
return todayFolder + newFileName;
}
/**
* 上传的多个图片
*
* @param savePath 文件的保存路径
* @param myFiles 文件
*/
public static List<Map<String, String>> uploadImages(String savePath, MultipartFile[] myFiles) {
List<Map<String, String>> list = new ArrayList<>();
BufferedOutputStream bos = null;
FileOutputStream fos = null;
File file = null;
String newFileName = null;
String todayFolder = getDailyFilePath();
Map<String, String> map = null;
for (MultipartFile multipartFile : myFiles) {
map = new HashMap<String, String>();
String uploadFileName = multipartFile.getOriginalFilename();
// if (checkSuffix(suffix, contentType)) {
try {
byte[] imageData = multipartFile.getBytes();
String pathStr = savePath + todayFolder;
File path = new File(pathStr);
if (!path.exists()) {
path.mkdirs();
}
newFileName = getTimeStampName(uploadFileName, pathStr);
// newFileName =new String(newFileName.getBytes("utf-8"),"8859_1");
// fos = new FileOutputStream(savePath + newFileName);
file = new File(pathStr + newFileName);
fos = new FileOutputStream(file);
bos = new BufferedOutputStream(fos);
bos.write(imageData);
} catch (Exception e) {
Constants.msunsoftlog.error("Woyundata Exception:"
+ ConvertStringUtil.exceptionToString(e));
} finally {
if (bos != null) {
try {
bos.close();
} catch (IOException e1) {
Constants.msunsoftlog.error("Woyundata Exception:"
+ ConvertStringUtil.exceptionToString(e1));
}
}
if (fos != null) {
try {
fos.close();
} catch (IOException e1) {
Constants.msunsoftlog.error("Woyundata Exception:"
+ ConvertStringUtil.exceptionToString(e1));
}
}
}
map.put("newFileName", todayFolder + newFileName);
map.put("oldFileName",getAddTimeStampName(uploadFileName) );
list.add(map);
}
// }
return list;
}
/**
* 根据路径创建一系列的目录
*
* @param path
*/
public static void mkDirectory(String path) {
File file;
try {
file = new File(path);
if (!file.exists()) {
file.mkdirs();
}
} catch (RuntimeException e) {
Constants.msunsoftlog.error("Woyundata Exception:"
+ ConvertStringUtil.exceptionToString(e));
} finally {
file = null;
}
}
/**
* 将对象数组的每一个元素分别添加到指定集合中,调用Apache commons collections 中的方法
*
* @param collection 目标集合对象
* @param arr 对象数组
*/
public static void addToCollection(Collection<Object> collection, Object[] arr) {
if (null != collection && null != arr) {
CollectionUtils.addAll(collection, arr);
}
}
/**
* 根据文件路径获取企业定制的文件
*
* @param customFilePath
* @return
*/
public static File getCustomFile(String customFilePath) {
String url = FileUtils.class.getResource("").getPath().replaceAll("%20", " ");
String path = url.substring(0, url.indexOf("customFile")) + "customFile/" + customFilePath;
File file = new File(path);
return file;
}
public static String getWebrootPath(String floder) {
String root = FileUtils.class.getResource("/").getFile();
try {
root = new File(root).getParentFile().getParentFile().getCanonicalPath();
root += File.separator + floder + File.separator;
} catch (IOException e) {
throw new RuntimeException(e);
}
mkDirectory(root);
return root;
}
/**
* 上传文件
*
* @param uploadFileName 被上传的文件名称
* @param rootDir 保存文件目录
* @param inputStream 上传文件流
* @return
*/
public static String upload(String uploadFileName, String rootDir, InputStream inputStream) {
String newFileName = null;
String daiLyFolder = "";
try {
BufferedInputStream input = new BufferedInputStream(inputStream);
daiLyFolder = getDailyFilePath();
String pathStr = rootDir + daiLyFolder;
File path = new File(pathStr);
if (!path.exists()) {
path.mkdirs();
}
newFileName = getTimeStampName(uploadFileName, pathStr);
File outputFile = new File(pathStr + newFileName);
BufferedOutputStream output = new BufferedOutputStream(new FileOutputStream(outputFile));
byte[] b = new byte[1024 * 5];
int len;
while ((len = input.read(b)) != -1) {
output.write(b, 0, len);
}
output.flush();
output.close();
input.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
return daiLyFolder + newFileName;
}
/**
* 随机产生一串字符
*
* @param length 几位数的字符
* @return
*/
private static long b = 1180000000000L;
private static long seedUniquifier = System.currentTimeMillis() - b;
private static final int DEFAULT_RADIX = 36;
public static String getUniqueId(int length) {
seedUniquifier++;
String uniqueId = Long.toString(seedUniquifier, DEFAULT_RADIX);
int initialLength = uniqueId.length();
int leftLength = initialLength - length;
if (leftLength > 0) {
uniqueId = uniqueId.substring(leftLength, initialLength);
} else if (leftLength < 0) {
StringBuilder buffer = new StringBuilder();
while (leftLength < 0) {
buffer.append('0');
leftLength++;
}
buffer.append(uniqueId);
uniqueId = buffer.toString();
}
return uniqueId;
}
/**
* 获取每日的一日起命名的文件夹
*
* @return
*/
public static String getDailyFilePath() {
return DateFormatUtils.ISO_DATE_FORMAT.format(new Date()) + "/";
}
public static boolean checkSuffix(String suffix, String contentType) {
boolean checkFlag = false;
if (".jpg".equals(suffix) || ".JPG".equals(suffix) || ".JPEG".equals(suffix)
|| ".jpeg".equals(suffix)) {
if ("image/jpeg".equals(contentType)) {
checkFlag = true;
}
} else if (".png".equals(suffix)) {
if ("image/png".equals(contentType)) {
checkFlag = true;
}
} else if (".docx".equals(suffix)) {
if ("application/vnd.openxmlformats-officedocument.wordprocessingml.document"
.equals(contentType)) {
checkFlag = true;
}
} else if (".doc".equals(suffix)) {
if ("application/msword".equals(contentType)) {
checkFlag = true;
}
} else if (".xlsx".equals(suffix)) {
if ("application/vnd.openxmlformats-officedocument.spreadsheetml.sheet"
.equals(contentType)) {
checkFlag = true;
}
} else if (".xls".equals(suffix)) {
if ("application/vnd.ms-excel".equals(contentType)) {
checkFlag = true;
}
} else if (".pdf".equals(suffix)) {
if ("application/pdf".equals(contentType)) {
checkFlag = true;
}
} else if (".zip".equals(suffix)) {
if ("application/x-zip-compressed".equals(contentType)) {
checkFlag = true;
}else if("application/zip".equals(contentType)) {
checkFlag = true;
}
} else if (".rar".equals(suffix)) {
if ("application/octet-stream".equals(contentType)) {
checkFlag = true;
}
}
return checkFlag;
}
}