1. 成员函数
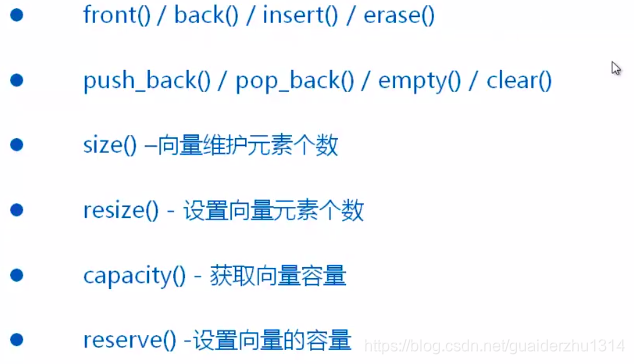
- 向量只提供了尾部增加和删除
- 容量就是最多可以放多少个。容量反应的是 向量容器维护了多少内存,大小反应的是已经占据了多少内存。
2. 初始化
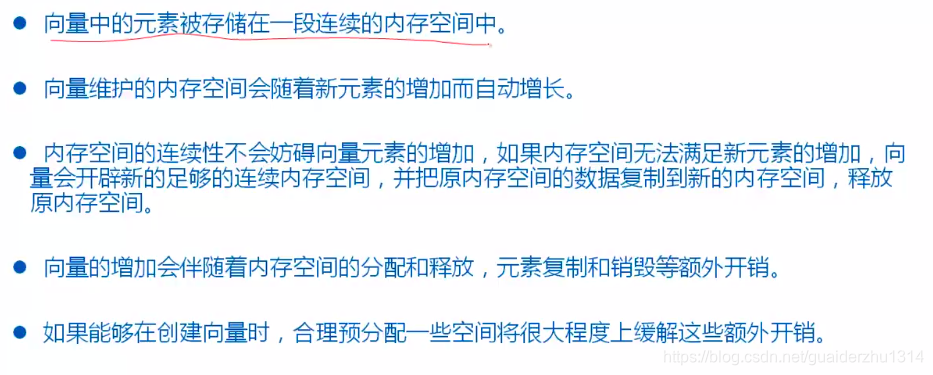
- 给向量后面添加新元素时,如果向量维护的内存空间满载数据,而且其后的内存空间也已经被占用,那么就会自动开辟新的足够大的连续内存空间,并把原来的数据拷贝过来(副本造副本),然后释放掉原内存空间。
- 这个问题可以通过reserve() 提前设置向量容量来解决。
#include <iostream>
#include <vector>
#include <cstdio>
using namespace std;
class Student{
public:
Student(string const& name = ""):m_name(name){
cout << "缺省构造了: " << m_name << "(" << this << ")" << endl;
}
Student(Student const& that):m_name(that.m_name){
cout << "用: " << that.m_name << "(" << &that << ")" << "拷贝构造了" << m_name
<< "(" << this << ")" << endl;
}
~Student(){
cout << "析构了: " << m_name << "(" << this << ")" << endl;
}
private:
string m_name;
};
int main(){
vector<Student> vs;
vs.push_back(Student("张三"));
vs.push_back(Student("李四"));
getchar();
}
$ ./a.out
缺省构造了: 张三(0x7ffc78ad00c0)
用: 张三(0x7ffc78ad00c0)拷贝构造了张三(0xe8d030)
析构了: 张三(0x7ffc78ad00c0)
缺省构造了: 李四(0x7ffc78ad0100)
用: 李四(0x7ffc78ad0100)拷贝构造了李四(0xe8d080)
用: 张三(0xe8d030)拷贝构造了张三(0xe8d060)
析构了: 张三(0xe8d030)
析构了: 李四(0x7ffc78ad0100)
析构了: 张三(0xe8d060)
析构了: 李四(0xe8d080)
3. 迭代器的使用
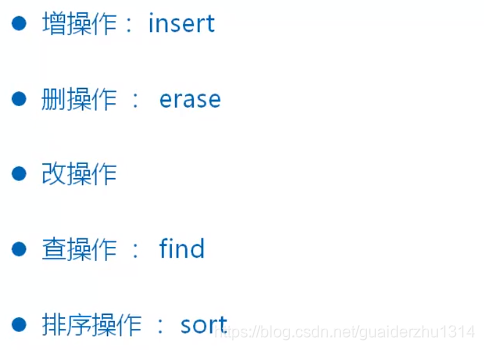
#include <iostream>
#include <vector>
#include <cstdio>
#include<algorithm>
using namespace std;
class Student{
public:
Student(string const& name = "", int age = 0):m_name(name), m_age(age){}
private:
string m_name;
int m_age;
friend ostream& operator<<(ostream& os, const Student& that);
friend bool operator==(const Student& a, const Student& b);
friend bool operator<(const Student& a, const Student& b);
friend bool operator>(const Student& a, const Student& b);
};
ostream& operator<<(ostream& os, const Student& that){
os << that.m_name << ": " << that.m_age;
}
bool operator==(const Student& a, const Student& b){
return(a.m_age == b.m_age && a.m_name == b.m_name);
}
bool operator<(const Student& a, const Student& b){
return(a.m_age < b.m_age);
}
bool operator>(const Student& a, const Student& b){
return(a.m_age > b.m_age);
}
void print(string const& str, vector<Student>& v){
cout << str << endl;
typedef vector<Student>::iterator IT;
for(IT it = v.begin(); it != v.end(); it++){
cout << *it<<", ";
}
cout << endl << "-------------------------"<<endl;
}
class CMP{
public:
bool operator()(Student const& a, Student const& b){
return a > b;
}
};
int main(){
vector<Student> vs;
vs.reserve(10);
vs.push_back(Student("张三", 1));
vs.push_back(Student("李四", 2));
vs.push_back(Student("王五", 3));
print("添加节点以后: ", vs);
vs.insert(vs.begin(), Student("xuehui", 4));
print("插入节点以后: ", vs);
vs.erase(vs.begin());
print("删除节点以后: ", vs);
typedef vector<Student>::iterator IT;
IT it = vs.begin();
*it = Student("赵六", 5);
print("修改节点以后", vs);
IT fit = find(vs.begin(), vs.end(), Student("李四", 2));
if(fit != vs.end()){
cout << "找到李四" << endl;
}
sort(vs.begin(), vs.end());
print("按年龄升序排列", vs);
CMP cmp;
sort(vs.begin(), vs.end(), cmp);
print("按年龄降序排列", vs);
}
$ ./a.out
添加节点以后:
张三: 1, 李四: 2, 王五: 3,
-------------------------
插入节点以后:
xuehui: 4, 张三: 1, 李四: 2, 王五: 3,
-------------------------
删除节点以后:
张三: 1, 李四: 2, 王五: 3,
-------------------------
修改节点以后
赵六: 5, 李四: 2, 王五: 3,
-------------------------
找到李四
按年龄升序排列
李四: 2, 王五: 3, 赵六: 5,
-------------------------
按年龄降序排列
赵六: 5, 王五: 3, 李四: 2,
-------------------------