XML文件解析
NSXMLParser
-(instancetype)init{
self = [super init];
if (self) {
NSBundle *b = [NSBundle mainBundle];
NSString *path = [b pathForResource:@"customers" ofType:@".xml"];
NSData *data = [NSData dataWithContentsOfFile:path];
self.parser = [[NSXMLParser alloc]initWithData:data];
self.parser.delegate = self;
self.list = [NSMutableArray arrayWithCapacity:5];
}
return self;
}
- (void)parser:(NSXMLParser *)parser didStartElement:(NSString *)elementName namespaceURI:(nullable NSString *)namespaceURI qualifiedName:(nullable NSString *)qName attributes:(NSDictionary<NSString *, NSString *> *)attributeDict{
NSLog(@"didStartElement... %@",elementName);
self.currentElement = elementName;
if([self.currentElement isEqualToString:@"customer"]){
self.customer = [[Customer alloc]init];
}
}
- (void)parser:(NSXMLParser *)parser foundCharacters:(NSString *)string{
NSLog(@"foundCharacters... %@",string);
if([self.currentElement isEqualToString:@"id"]){
int cid = [string intValue];
[self.customer setCid:cid];
}else if([self.currentElement isEqualToString:@"name"]){
[self.customer setName:string];
}else if([self.currentElement isEqualToString:@"name"]){
int age = [string intValue];
[self.customer setAge:age];
}
}
- (void)parser:(NSXMLParser *)parser didEndElement:(NSString *)elementName namespaceURI:(nullable NSString *)namespaceURI qualifiedName:(nullable NSString *)qName{
NSLog(@"didEndElement... %@",elementName);
if([elementName isEqualToString:@"customer"]){
[self.list addObject:self.customer];
}
self.currentElement = nil;
}
- (void)parserDidStartDocument:(NSXMLParser *)parser{
NSLog(@"parserDidStartDocument...");
}
- (void)parserDidEndDocument:(NSXMLParser *)parser{
NSLog(@"parserDidEndDocument...");
}
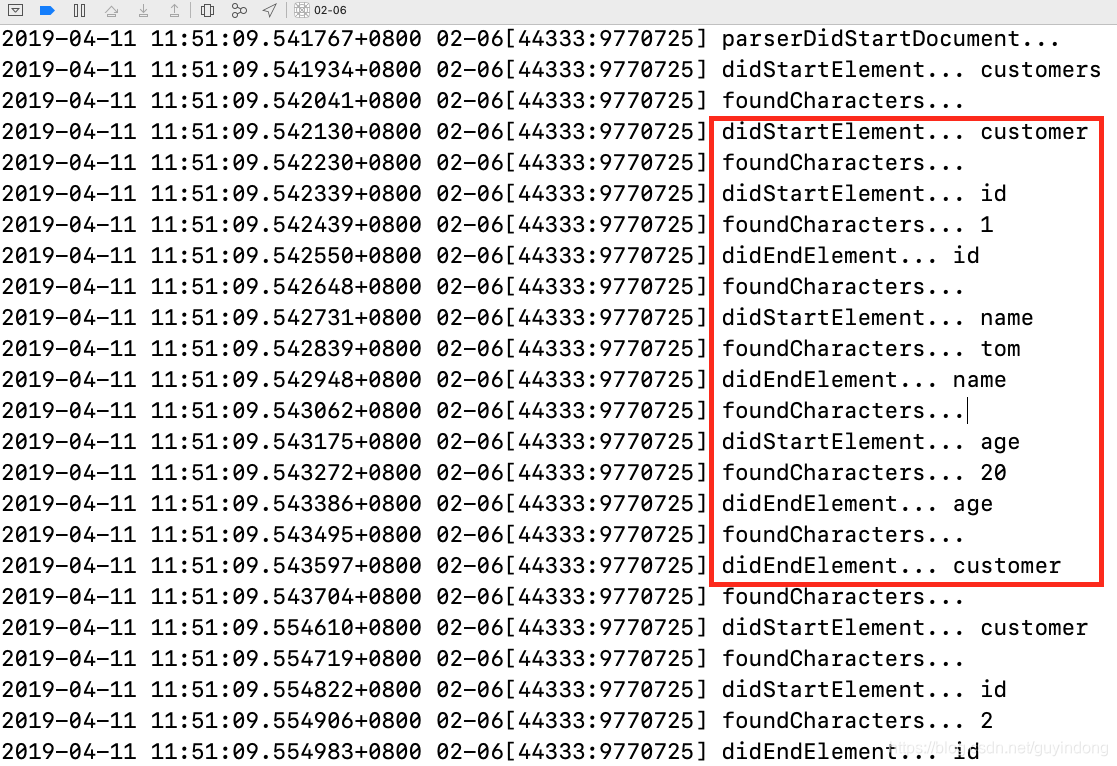
GDataXML
NSString *filePath = [[NSBundle mainBundle]pathForResource:@"customers" ofType:@"xml"];
NSData *xmlData = [[NSData alloc]initWithContentsOfFile:filePath];
GDataXMLDocument *doc = [[GDataXMLDocument alloc]initWithData:xmlData options:0 error:nil];
GDataXMLElement *rootElement = [doc rootElement];
NSArray *users = [rootElement elementsForName:@"customer"];
for (GDataXMLElement *user in users) {
GDataXMLElement *idElement = [[user elementsForName:@"id"] objectAtIndex:0];
NSString *cid = [idElement stringValue];
NSLog(@"Customer id is:%@",cid);
GDataXMLElement *nameElement = [[user elementsForName:@"name"] objectAtIndex:0];
NSString *name = [nameElement stringValue];
NSLog(@"Customer name is:%@",name);
GDataXMLElement *ageElement = [[user elementsForName:@"age"] objectAtIndex:0];
NSString *age = [ageElement stringValue];
NSLog(@"Customer age is:%@",age);
NSLog(@"----------------------");
}
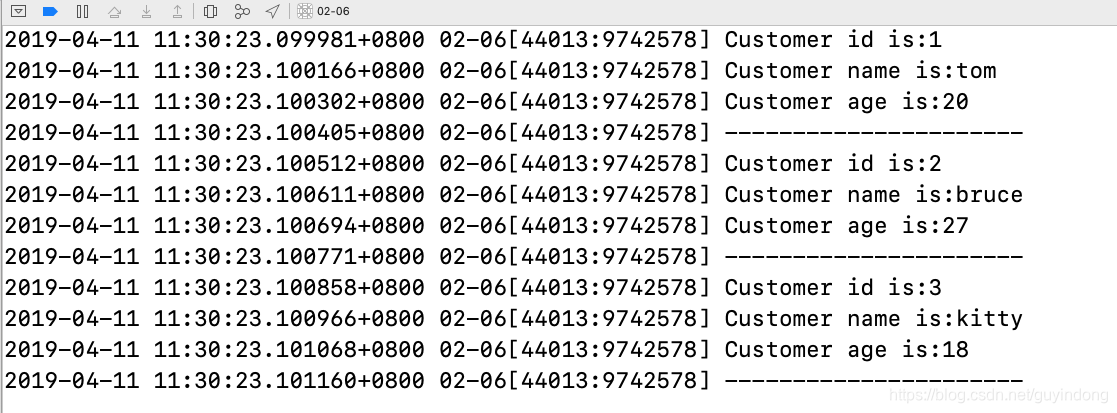
JSON数据解析
NSJSONSerialization
{
"name":"中国",
"province":[
{
"name":"黑龙江",
"cities":{
"city":["哈尔滨","大庆"]
}
},
{
"name":"浙江",
"cities":{
"city":["杭州","宁波"]
}
},
{
"name":"江苏",
"cities":{
"city":["南京","苏州"]
}
}
]
}
NSBundle *b = [NSBundle mainBundle];
NSString *path = [b pathForResource:@"json" ofType:@".json"];
NSString *content = [NSString stringWithContentsOfFile:path encoding:NSUTF8StringEncoding error:nil];
NSLog(@"content=%@",content);
NSDictionary *dic = [NSJSONSerialization JSONObjectWithData:[content dataUsingEncoding:NSUTF8StringEncoding] options:NSJSONReadingAllowFragments error:nil];
NSLog(@"dic=%@",dic);
NSString *name = [dic objectForKey:@"name"];
NSLog(@"name=%@",name);
NSArray *array = [dic objectForKey:@"province"];
for(NSDictionary *dic in array){
NSLog(@"dic=%@", dic);
}
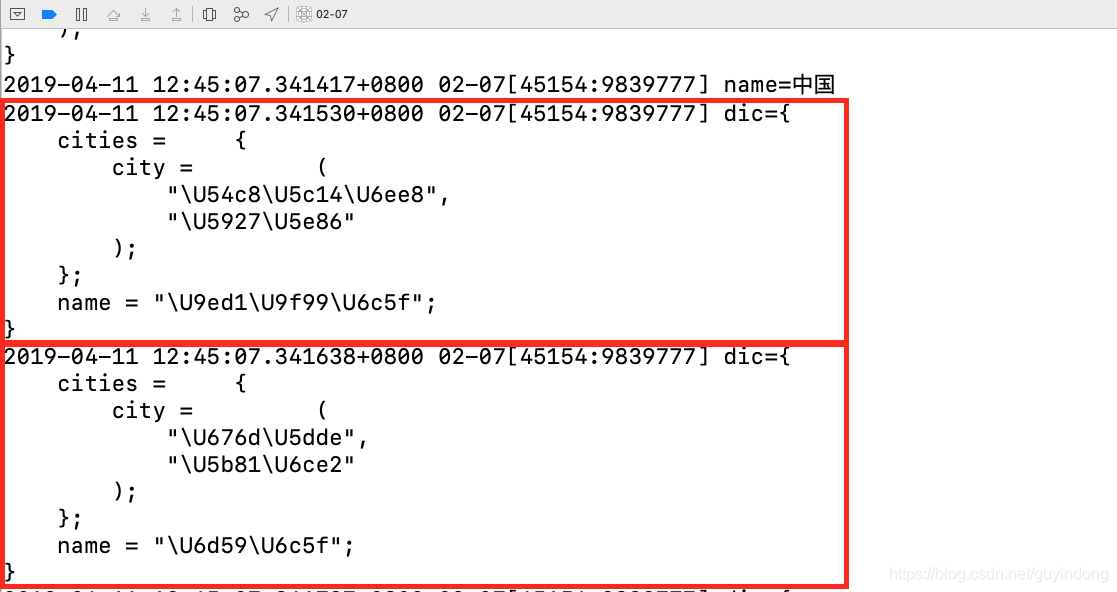
SBJSON
NSBundle *b = [NSBundle mainBundle];
NSString *path = [b pathForResource:@"json" ofType:@".json"];
NSString *content = [NSString stringWithContentsOfFile:path encoding:NSUTF8StringEncoding error:nil];
NSLog(@"content=%@",content);
SBJsonParser *parser = [[SBJsonParser alloc]init];
NSDictionary *dic = [parser objectWithString:content];
NSLog(@"dic=%@",dic);
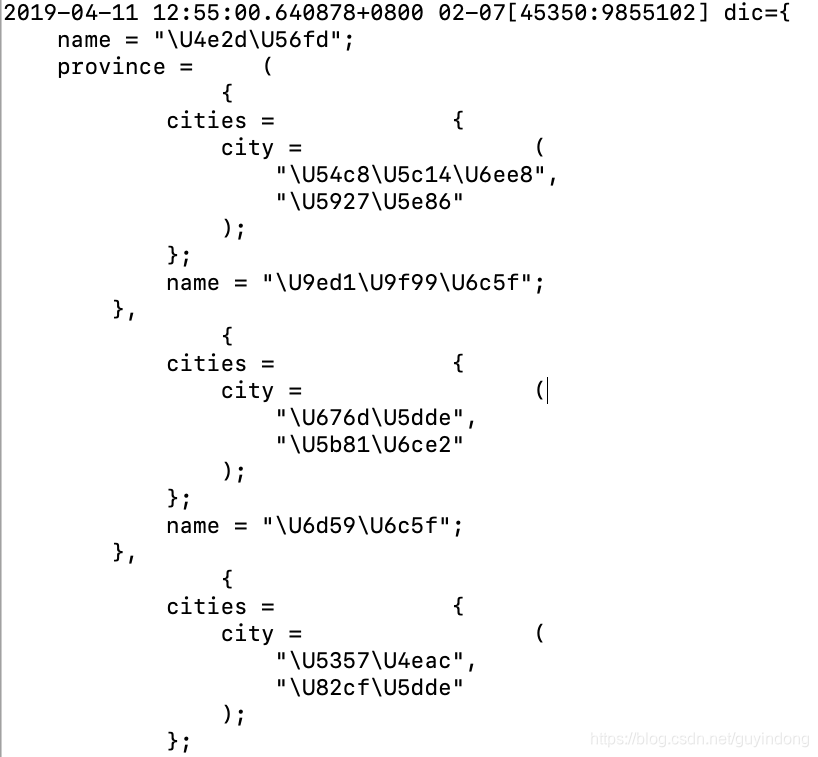